Android Month 2_Day2_Menu menu and Opup Window
1. System menu Options Menu
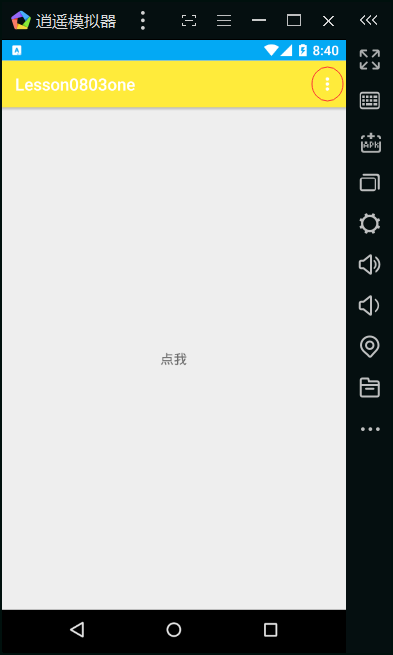
Step flow
1. Create menu folders
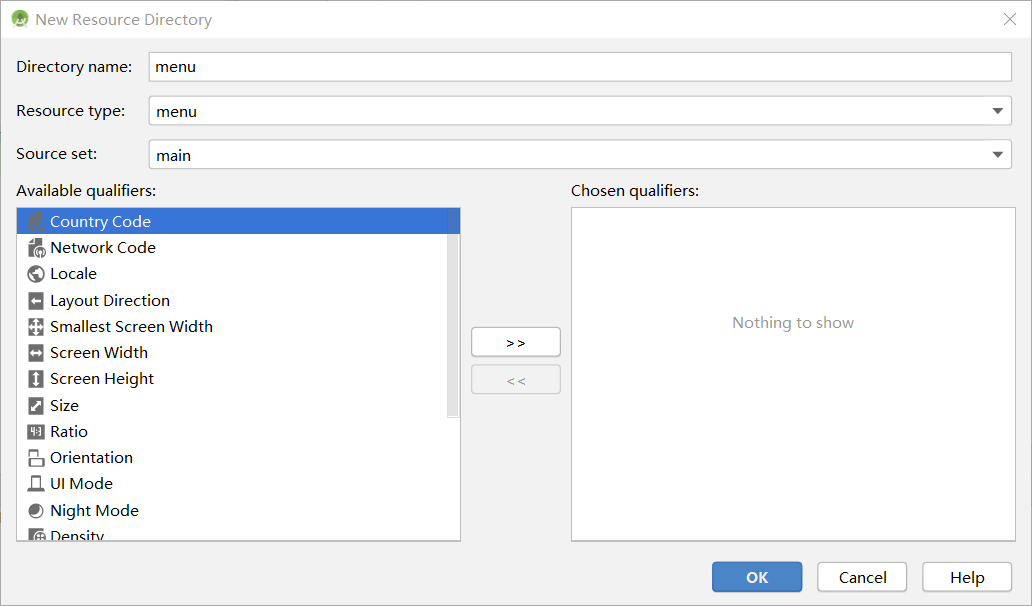
2. Create. xml file under menu folder
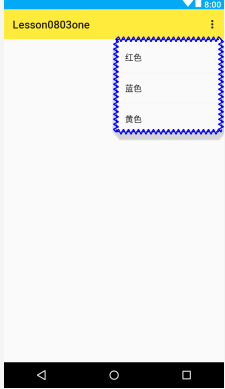
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/red" android:title="gules"/>
<item android:id="@+id/blue" android:title="blue"/>
<item android:id="@+id/yello" android:title="yellow"/>
</menu>
3.Activity rewrites onCreateOptionsMenu load resource files
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu,menu);
return super.onCreateOptionsMenu(menu);
}
4.Activity rewrites onOptions ItemSelected plus event listening
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
switch (id){
case R.id.red:
Toast.makeText(this, "gules", Toast.LENGTH_SHORT).show();
break;
case R.id.blue:
Toast.makeText(this, "blue", Toast.LENGTH_SHORT).show();
break;
case R.id.yello:
Toast.makeText(this, "yellow", Toast.LENGTH_SHORT).show();
break;
}
return super.onOptionsItemSelected(item);
}
Note: An Activity has only one system menu
Context Menu
Step flow:
1. Create a menu folder under res and create an xml file as the layout file of ContextMenu. We reuse the menu layout above.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/red" android:title="gules"/>
<item android:id="@+id/blue" android:title="blue"/>
<item android:id="@+id/yello" android:title="yellow"/>
</menu>
2.Activity rewrites onCreateConextMenu load resource files
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenu.ContextMenuInfo menuInfo) {
getMenuInflater().inflate(R.menu.menu,menu);
super.onCreateContextMenu(menu, v, menuInfo);
}
3.Activity rewrites onConextItemSelected to set up event listening
@Override
public boolean onContextItemSelected(MenuItem item) {
int id = item.getItemId();
switch (id){
case R.id.red:
Toast.makeText(this, "gules", Toast.LENGTH_SHORT).show();
break;
case R.id.blue:
Toast.makeText(this, "blue", Toast.LENGTH_SHORT).show();
break;
case R.id.yello:
Toast.makeText(this, "yellow", Toast.LENGTH_SHORT).show();
break;
}
return super.onContextItemSelected(item);
}
3. Pop-up menu
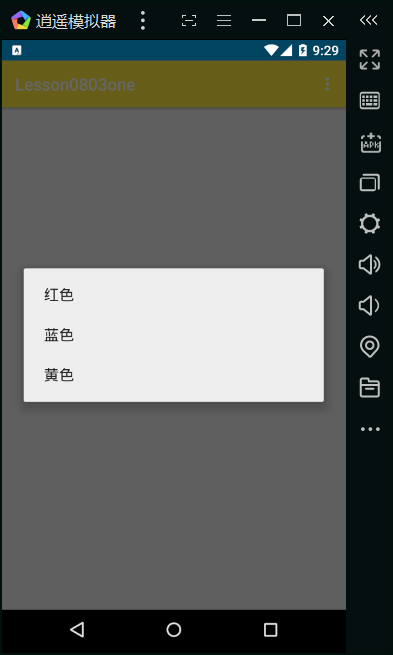
1. Implementation process:
Step 1: Create a menu folder under res and create a new xml file as the layout file for PoupMenu.
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<item android:id="@+id/red" android:title="gules"/>
<item android:id="@+id/blue" android:title="blue"/>
<item android:id="@+id/yello" android:title="yellow"/>
</menu>
Step 2: Encapsulate PopupMenu related logic into showPopupMenu() method, including instantiation of PopupMenu, layout settings, display, click monitoring and response by adding MenuItem, etc.
public void popupMenu(){
PopupMenu popupMenu = new PopupMenu(MainActivity.this, tv);
popupMenu.inflate(R.menu.menu);
popupMenu.setOnMenuItemClickListener(new PopupMenu.OnMenuItemClickListener() {
@Override
public boolean onMenuItemClick(MenuItem item) {
int id = item.getItemId();
switch (id){
case R.id.red:
Toast.makeText(MainActivity.this, "gules", Toast.LENGTH_SHORT).show();
break;
case R.id.blue:
Toast.makeText(MainActivity.this, "blue", Toast.LENGTH_SHORT).show();
break;
case R.id.yello:
Toast.makeText(MainActivity.this, "yellow", Toast.LENGTH_SHORT).show();
break;
}
return false;
}
});
popupMenu.show();
}
Step 3: Set up event listening for the control and call the showPopupMenu() method directly
tv.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
popupMenu();
}
});