Recently, the step counting module was studied in the project. It began to record the steps of the day at 0 o'clock every day, which is similar to the Wechat movement. Many pits have been encountered. Today, I have time to sort them out for you to see.
Before doing this, we searched google, baidu, github and found no good ones. Most of them need to survive in the background, and need backstage Service.
Alert Manager, android.intent.action.BOOT_COMPLETED and back-end Service are basically eliminated for major mobile phone manufacturers to improve battery life (power saving).
Background survival strategy Service is basically useless. It's only a matter of time before the mobile phone system kills it. So I think it's better not to do it. Even if the backstage survives, users will see that the app is very power-intensive and will be deleted.
Project address: https://github.com/jiahongfei/TodayStepCounter
At present, there are two ways of android step-counting
System Stepper Chip
After Android 4.4, some models have implemented Sensor.TYPE_STEP_COUNTER sensors to record the number of steps users walk. Starting from the start of the mobile phone record, when the mobile phone shutdown reset to 0.
The stepping chip is system-level. Compared with previous versions of sensor stepping, the performance of the chip has some optimization.
App won't consume extra power because it uses step-taking alone.
The system chip step-taking is continuous and can optimize the problem of some models without step-taking in the background.
Calculating Method of Acceleration Sensor
Acceleration sensors consume a lot of power, which leads to high power consumption of App and affects user experience.
Background real-time operation is required to achieve the function of footnote. If the App process is killed by the system or security software, the function of footnote can not be used.
Project address: https://github.com/jiahongfei/TodayStepCounter
According to the above two ways to achieve step counting, mobile phones provide step counting sensors using Sensor.TYPE_STEP_COUNTER mode (app backstage shutdown can also be step counting), if not, use SensorManager.SENSOR_DELAY_UI mode (app needs to keep the background running).
Project structure:
Step-counting Service uses a separate process, so it uses inter-process communication aidl, todaystep counterlib as a library file to implement step-counting algorithm in a separate process, and app relies on the todaystep counterlib project to obtain the current step-counting display.
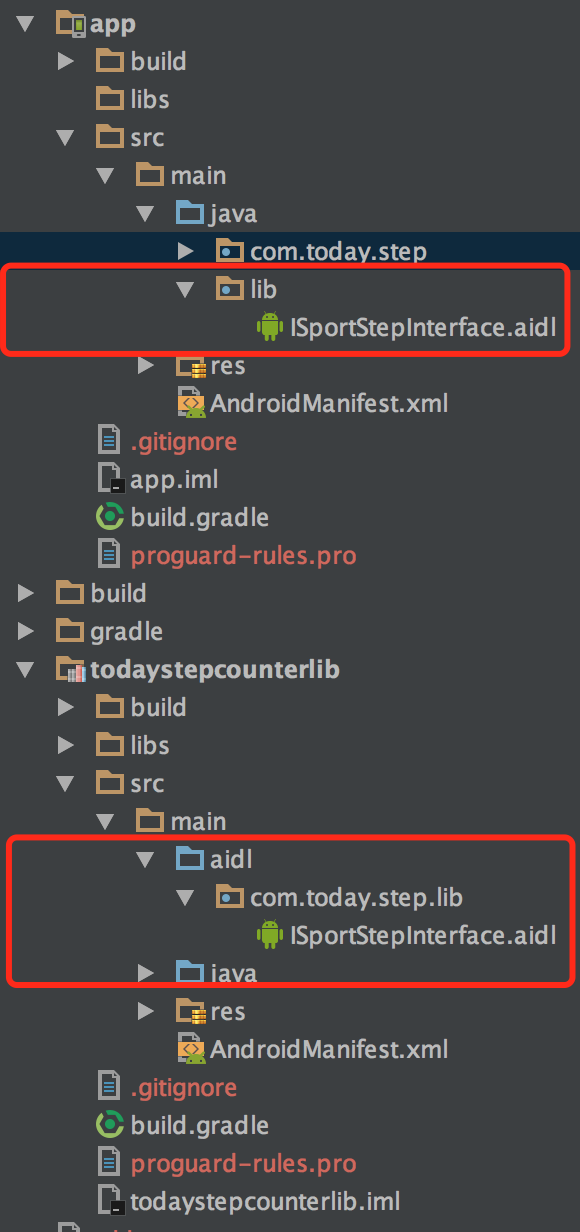
Access mode:
How to use the step-counting module in the project structure app is shown in the following code
public class MainActivity extends AppCompatActivity { private static String TAG = "MainActivity"; private static final int REFRESH_STEP_WHAT = 0; //The interval between the steps of the current time taken by the loop private long TIME_INTERVAL_REFRESH = 500; private Handler mDelayHandler = new Handler(new TodayStepCounterCall()); private int mStepSum; private ISportStepInterface iSportStepInterface; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Intent intent = new Intent(this, VitalityStepService.class); startService(intent); bindService(intent, new ServiceConnection() { @Override public void onServiceConnected(ComponentName name, IBinder service) { iSportStepInterface = ISportStepInterface.Stub.asInterface(service); try { mStepSum = iSportStepInterface.getCurrTimeSportStep(); updateStepCount(); } catch (RemoteException e) { e.printStackTrace(); } mDelayHandler.sendEmptyMessageDelayed(REFRESH_STEP_WHAT, TIME_INTERVAL_REFRESH); } @Override public void onServiceDisconnected(ComponentName name) { } }, Context.BIND_AUTO_CREATE); } class TodayStepCounterCall implements Handler.Callback{ @Override public boolean handleMessage(Message msg) { switch (msg.what) { case REFRESH_STEP_WHAT: { if (null != iSportStepInterface) { int step = 0; try { step = iSportStepInterface.getCurrTimeSportStep(); } catch (RemoteException e) { e.printStackTrace(); } if (mStepSum != step) { mStepSum = step; updateStepCount(); } } mDelayHandler.sendEmptyMessageDelayed(REFRESH_STEP_WHAT, TIME_INTERVAL_REFRESH); break; } } return false; } } private void updateStepCount() { Log.e(TAG,"updateStepCount : " + mStepSum); TextView stepTextView = (TextView)findViewById(R.id.stepTextView); stepTextView.setText(mStepSum + "step"); } }
Step-by-step strategy:
1. If the accelerometer step is used, the app must survive in the background before the step can be counted. '
2. The most important thing is to use the step-counting sensor to realize the step-counting, and the app can also do the step-counting when it closes in the background.
The following is a step-counting strategy using Sensor.TYPE_STEP_COUNTER sensor:
1. Users install new apps, starting with the first time they open App, and it doesn't cross the sky on that day.

2. The user keeps opening the app counting step and does not close the app across 0.

3. The user opens an App backend and closes it once. Alert Manager can't boot itself across 0 and separated by 0 (most mobile phones can't boot at present).

4. The user opens an app and closes the background once, spanning more than 0 points, and the Alertmanager 0-point separation can be started.

5. Users open an app once and restart the phone on the same day (self-startup is not good, many phones are not good)

6. The user opens the app once, and the switch crosses 0 points.

7. The user opens the app once, and the switch crosses 0 points.

defect
1. Plan three spans 0 points open app steps to calculate the previous day, if spanning more than one day will lead to a very large number of steps the previous day.
2. The number of steps before 0 is lost in solution 4 (since 0-point-separated Alert Manager can call back, so data before 0 can be processed, and later versions can be repaired).
3. Frequently calling SharePreference in the step callback
Be careful:
1. Opening App every morning can improve the accuracy of several steps, which is almost the same as the number of steps in Wechat.
2. Open app every time you restart your mobile phone. It will merge steps.