This paper mainly describes the architecture and implementation principle of G-sensor related software and Hardware on Android 2.3 platform, which are introduced respectively according to the five levels of Applications, Framework, HAL, Driver and Hardware.
1. System architecture
1.1 Android architecture diagram
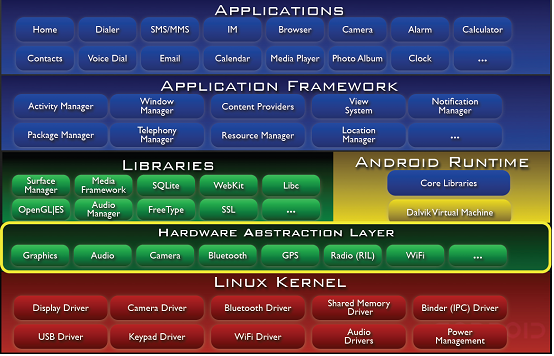
1.2 Sensor subsystem architecture diagram
· Application Framework
The Sensor application obtains Sensor data through the Sensor application framework, and the Sensor Manager of the application framework layer communicates with the C + + layer through JNI.
· Sensor Libraries
The Sensor middle layer is mainly composed of Sensor Manager, Sensor service and Sensor hardware abstraction layer.
· Input Subsystem
The general Linux input framework is designed for input devices such as keyboard, mouse and touch screen, and defines a set of standard event sets. The Sensor input subsystem adopts a general Linux input framework, which interacts with the user space through the / sys/class/input node.
· Event Dev
Evdev provides a common way to access / dev/input/eventX input device events.
· AccelerometerDriver
The driver communicates with MMA7660 module through SIRQ and I2C bus. SIRQ is used to generate sensor event interrupts.
2 applications
two point one Five steps of application development
(1) Obtain the sensor manager object;
mSensorManager =(SensorManager) getSystemService(SENSOR_SERVICE);
(2) Acquiring sensor objects;
mSensor = mSensorManager.getDefaultSensor(Sensor.TYPE_ACCELEROMETER);
(3) Define event listeners;
mEventListener =new SensorEventListener() {
@Override publicvoid onSensorChanged (SensorEvent event) { float[] values = event.values; mTextView.setText( "Accelerometer:" + values[ 0] + ", " + values[ 1] + ", " + values[ 2]); } @Override publicvoidonAccuracyChanged(Sensor sensor, int accuracy) { } };
(4) Register event listeners;
protectedvoid onResume() {
super.onResume(); mSensorManager.registerListener(mEventListener, mSensor, SensorManager.SENSOR_DELAY_NORMAL); }
(5) Uninstall the event listener;
protectedvoid onPause() {
super.onPause(); mSensorManager.unregisterListener(mEventListener); }
3 framework
3.1 working model
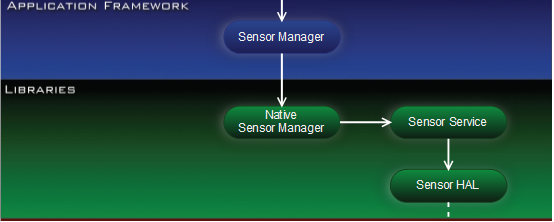
3.1.1 creation of sensormanager
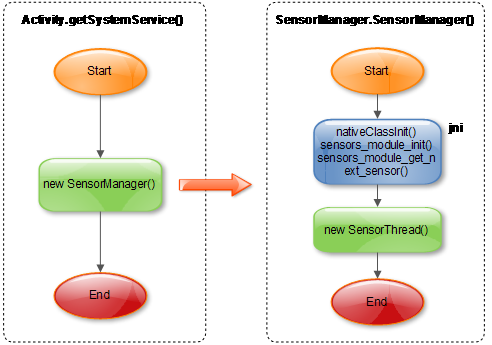
sensors_module_init(): create a Native SensorManager instance and read the Sensor device list from SensorService;
sensors_module_get_next_sensor(): read the next sensor device from SensorService;
3.1.2 SensorThread data receiving and processing
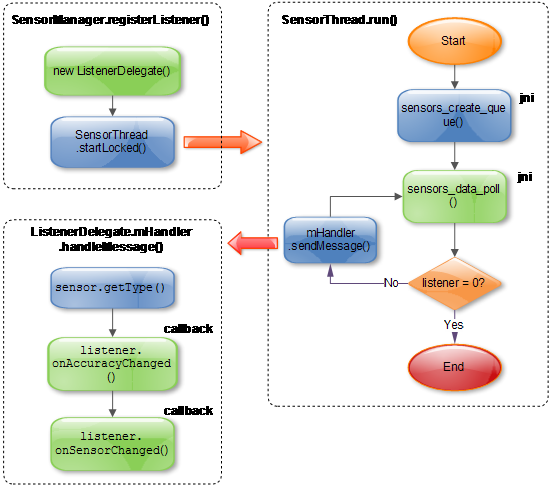
sensors_data_poll(): read the message sent by SensorService from the message queue;
3.1.3 working principle of sensorservice
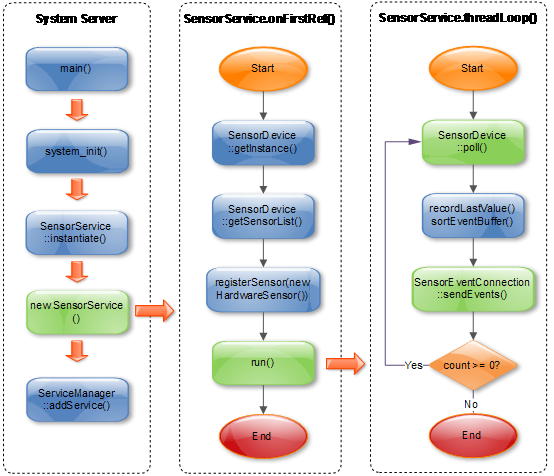
SensorEventConnection::sendEvents(): write a message to the message queue, and SensorThread will read the message later;
3.1.4 SensorDevice access to HAL
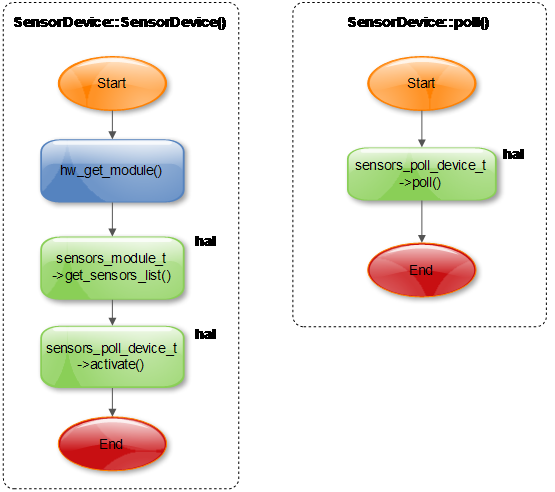
4 hardware abstraction layer (HAL)
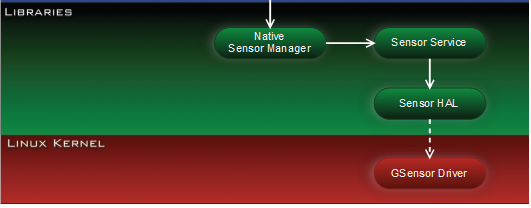
four point one Sensors HAL key processes
4.1.1 Turn on the Sensor device
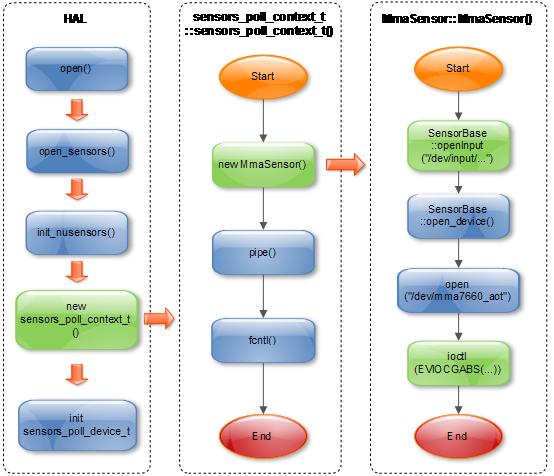
IOCTL (evocgabs (...): get ABS_ X/ABS_ Y/ABS_ Acceleration of Z;
4.1.2 Round robin Sensor event
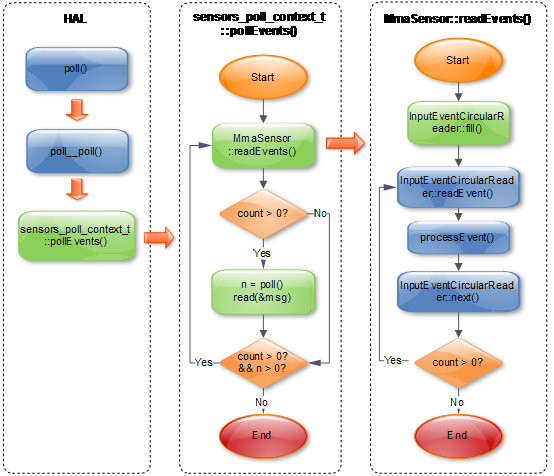
InputEventCircularReader::readEvent(): reads events from the ring buffer;
InputEventCircularReader::next(): move the current pointer of the ring buffer;
5.2.1 sensors_module_t
struct sensors_module_t { struct hw_module_t common; /** * Enumerate all available sensors. The list is returned in "list". * @return number of sensors in the list */ int (*get_sensors_list)( struct sensors_module_t* module, struct sensor_t const** list); };
hw_get_module() will load the Hal module and return the Hal entry data structure (hw_module_t). HAL_MODULE_INFO_SYM defaults to "Hal" in hw_get_module is obtained with dlsym.
const struct sensors_module_t HAL_MODULE_INFO_SYM = { .common = { .tag = HARDWARE_MODULE_TAG, .version_major = 1, .version_minor = 0, .id = SENSORS_HARDWARE_MODULE_ID, .name = "MMA7660 Sensors Module", .author = "The Android Open Source Project", .methods = &sensors_module_methods, }, .get_sensors_list = sensors__get_sensors_list };
5.2.2 hw_module_methods_t
.open = open_sensors };
5.2.3 sensors_poll_context_t
struct sensors_poll_context_t { struct sensors_poll_device_t device; // must be first sensors_poll_context_t(); ~ sensors_poll_context_t(); int activate(int handle, int enabled); int setDelay(int handle, int64_t ns); int pollEvents(sensors_event_t* data, int count); int handleToDriver(int handle); };
5.2.4 sensors_poll_device_t
struct sensors_poll_device_t { struct hw_device_t common; int (*activate)( struct sensors_poll_device_t *dev, int handle, int enabled); int (*setDelay)( struct sensors_poll_device_t *dev, int handle, int64_t ns); int (*poll)( struct sensors_poll_device_t *dev, sensors_event_t* data, int count); };
5.2.5 sensor_t
static const struct sensor_t sSensorList[] = { { "MMA7660 3-axis Accelerometer", "Freescale Semiconductor", 1, SENSORS_HANDLE_BASE+ID_A, SENSOR_TYPE_ACCELEROMETER, 3.0f* 9.81f, ( 3.0f* 9.81f)/ 64.0f, 0.35f, 0, { } }, };
struct sensor_t { const char* name; const char* vendor; int version; int handle; int type; float maxRange; float resolution; float power; int32_t minDelay; void* reserved[ 8]; };
6 driver
six point one Mm7660 drive frame
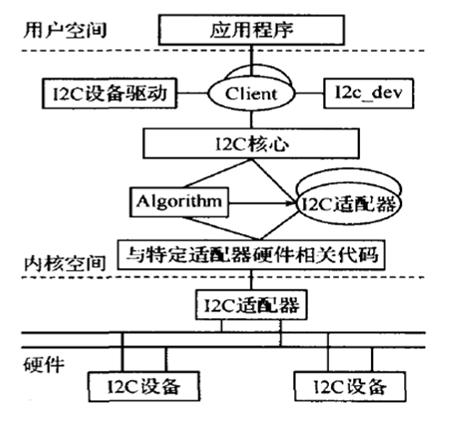
(1) I2C core
I2C core provides the registration and cancellation methods of I2C bus driver and device driver, the upper code of I2C communication method (i.e. "algorithm") independent of specific adapter, and the upper code of detection device and detection device address. This part is platform independent.
This part is implemented in the I2C driver of the Linux kernel. The mma7660 driver uses the functional interface it provides to register the device driver.
(2) I2C bus driver
I2C bus driver is the implementation of adapter in I2C hardware architecture. I2C bus driver mainly includes I2C adapter data structure_ algorithm data structure of adapter and I2C adapter_ algorithm and function controlling I2C adapter to generate communication signal. Through the I2C bus driven code, we can control the I2C adapter to generate start bit, stop bit and read-write cycle in the master mode, and to be read-write and generate ACK in the slave mode. Different CPU platforms correspond to different I2C bus drivers.
This part is implemented in the I2C driver of the Linux kernel. The mma7660 driver directly obtains the adapter provided by it and calls the interface of the I2C core to register.
(3) I2C device driver
I2C device driver is the implementation of device end in I2C hardware architecture. The device is generally connected to the I2C adapter controlled by the CPU, and exchanges data with the CPU through the I2C adapter. I2C device driver mainly includes data structure i2c_driver and I2C_ The client and mma7660 drivers need to implement the member functions.
I2C in the drivers directory in the Linux kernel source code_ Dev.c file, which realizes the function of I2C adapter device file. The application program accesses the device through "i2c-%d" file name and using file operation interfaces open(), write(), read(), ioctl() and close(). The application layer can use these interfaces to access the storage space or registers of the I2C device attached to the adapter and control the working mode of the I2C device.
six point two Mm7660 operation flow
6.2.1 initialization
6.2.2 Detection equipment
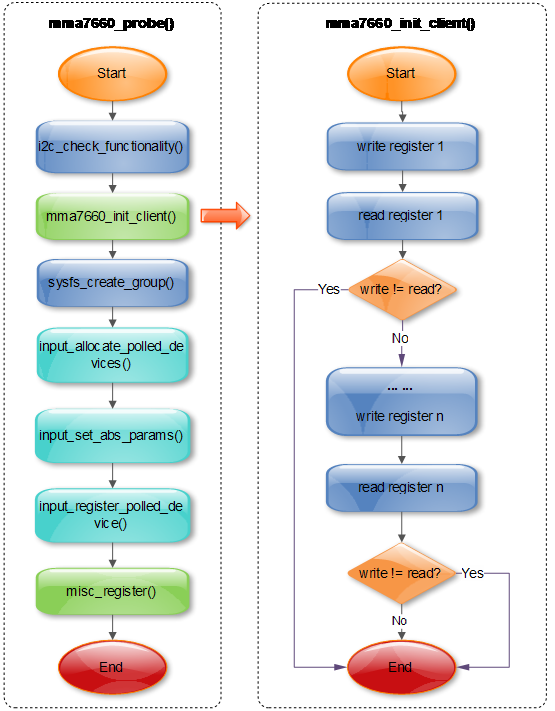
6.2.3 Remove device
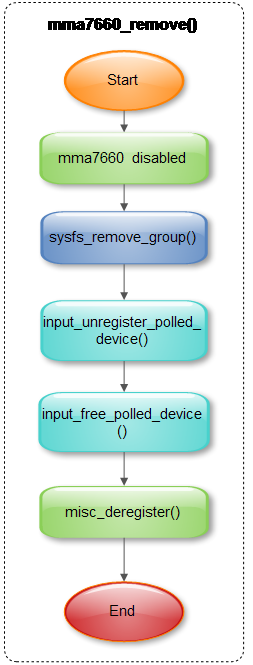
6.2.4 Collect data
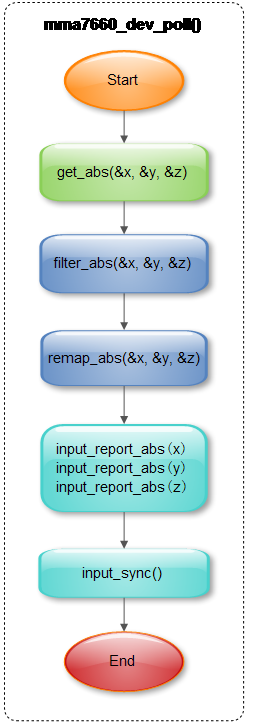
6.2.5 Sleep and wake
Suspend processing: close mma7660 module;Resume processing: enable mma7660 module;
static int mma7660_suspend(struct i2c_client *client, pm_message_t mesg) { int result; result = i2c_smbus_write_byte_data(client, MMA7660_MODE, MK_MMA7660_MODE( 0, 0, 0, 0, 0, 0, 0)); assert(result== 0); return result; } static int mma7660_resume(struct i2c_client *client) { int result; result = i2c_smbus_write_byte_data(client, MMA7660_MODE, MK_MMA7660_MODE( 0, 1, 0, 0, 0, 0, 1)); assert(result== 0); return result; } static struct i2c_driver mma7660_driver = { .driver = { .name = MMA7660_DRV_NAME, .owner = THIS_MODULE, }, . class = I2C_CLASS_HWMON, .suspend = mma7660_suspend, .resume = mma7660_resume, .probe = mma7660_probe, .detect = mma7660_detect, // .address_data = &addr_data, .remove = __devexit_p(mma7660_remove), .id_table = mma7660_id, };
six point three Command line debugging
6.3.1 sysfs debug interface
(1) Define data structures related to sysfs attribute;
static SENSOR_DEVICE_ATTR(all_axis_force, S_IRUGO, show_xyz_force, NULL, 0); static SENSOR_DEVICE_ATTR(x_axis_force, S_IRUGO, show_axis_force, NULL, 0); static SENSOR_DEVICE_ATTR(y_axis_force, S_IRUGO, show_axis_force, NULL, 1); static SENSOR_DEVICE_ATTR(z_axis_force, S_IRUGO, show_axis_force, NULL, 2); static SENSOR_DEVICE_ATTR(orientation, S_IRUGO, show_orientation, NULL, 0); static struct attribute* mma7660_attrs[] = { &sensor_dev_attr_all_axis_force.dev_attr.attr, &sensor_dev_attr_x_axis_force.dev_attr.attr, &sensor_dev_attr_y_axis_force.dev_attr.attr, &sensor_dev_attr_z_axis_force.dev_attr.attr, &sensor_dev_attr_orientation.dev_attr.attr, NULL }; static const struct attribute_group mma7660_group = { .attrs = mma7660_attrs, };
(2) Create sysfs file system in probe function;
result = sysfs_create_group(&client->dev.kobj, &mma7660_group); if (result != 0) { ERR( "sysfs_create_group err\n"); goto exit_sysfs_creat_failed; }
(3) Implement the read-write function related to sysfs attribute;
ssize_t show_orientation(struct device *dev, struct device_attribute *attr, char *buf) { int result; u8 tilt, new_orientation; mma7660_read_tilt(&tilt); DBG( "tilt [0x%x]\n", tilt); new_orientation = tilt & 0x1f; if (orientation!=new_orientation) orientation = new_orientation; switch ((orientation>> 2)& 0x07) { case 1: result = sprintf(buf, "Left\n"); break; case 2: result = sprintf(buf, "Right\n"); break; case 5: result = sprintf(buf, "Downward\n"); break; case 6: result = sprintf(buf, "Upward\n"); break; default: switch(orientation & 0x03) { case 1: result = sprintf(buf, "Front\n"); break; case 2: result = sprintf(buf, "Back\n"); break; default: result = sprintf(buf, "Unknown\n"); } } return result; }
ssize_t show_xyz_force(struct device *dev, struct device_attribute *attr, char *buf) { int i; s8 xyz[ 3]; for (i= 0; i< 3; i++) mma7660_read_xyz(i, &xyz[i]); return sprintf(buf, "(%d,%d,%d)\n", xyz[ 0], xyz[ 1], xyz[ 2]); } ssize_t show_axis_force(struct device *dev, struct device_attribute *attr, char *buf) { s8 force; int n = to_sensor_dev_attr(attr)->index; mma7660_read_xyz(n, &force); return sprintf(buf, "%d\n", force); }
6.3.2 Gsensor debugging instance
/sys/devices/platform/gl5201-i2c.1/i2c-1/1-004c # ls uevent name modalias subsystem power driver all_axis_force x_axis_force y_axis_force z_axis_force orientation input /sys/devices/platform/gl5201-i2c.1/i2c-1/1-004c # cat all_axis_force (-1,0,22)
7 Hardware
seven point one Mm7660 module
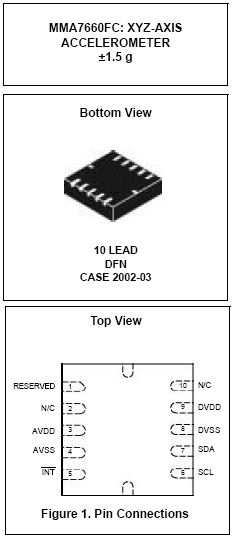
- Sampling Resolution: 6bit
- Digital Output (I2C)
- 3mm x 3mm x 0.9mm DFN Package
- Low Power Current Consumption:
Standby Mode: 2 μA,
Active Mode: 47 μA at 1 ODR
- Configurable Samples per Second from 1 to 120 samples
- Low Voltage Operation:
Digital Voltage: 1.71 V - 3.6 V
- Auto-Wake/Sleep Feature for Low Power Consumption
- Tilt Orientation Detection for Portrait/Landscape Capability
- Gesture Detection Including Shake Detection and Tap Detection
7.2.1 Functional module diagram
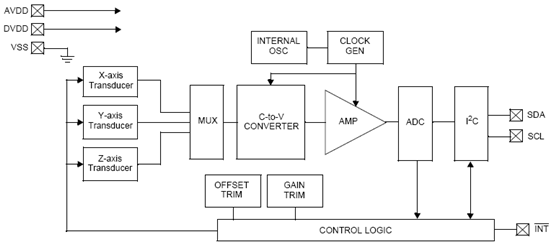
7.2.2 Hardware connection diagram
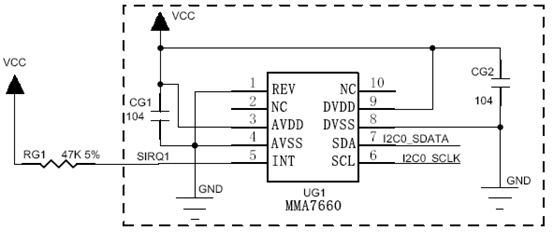
7.2.3 Motion detection principle
The simple physical model is shown as follows:
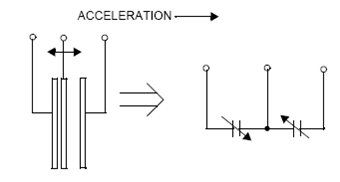
7.2.4 I2C read / write timing
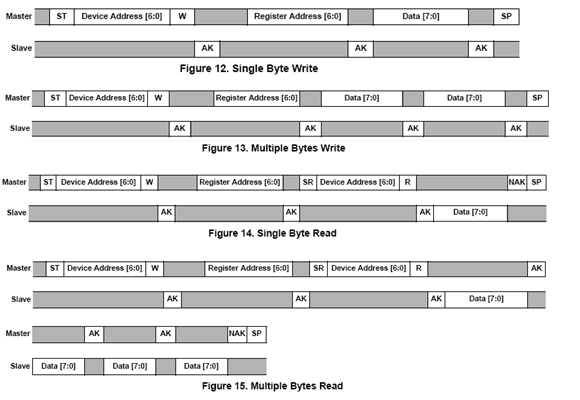
7.2.5 Working state machine
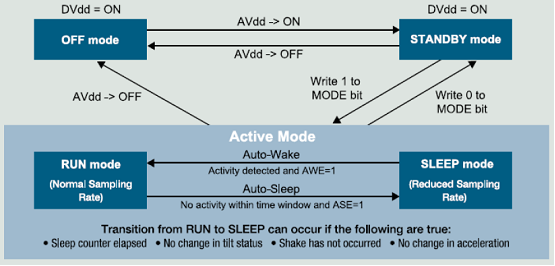
7.2.6 Register definition
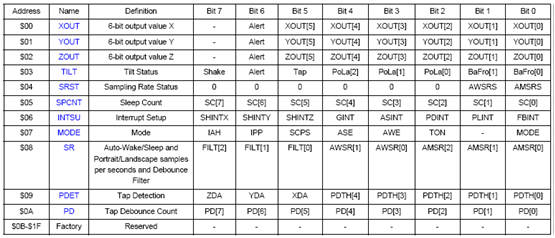
7.2.7 event detection
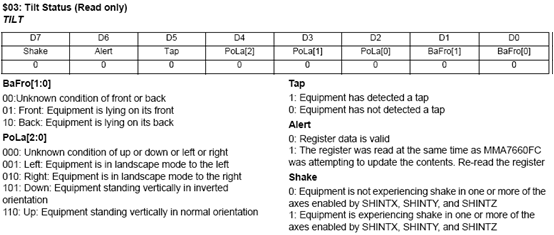
- Direction and shake detection
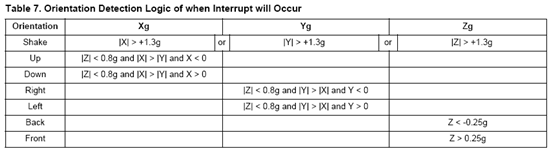
- Tap or tilt detection
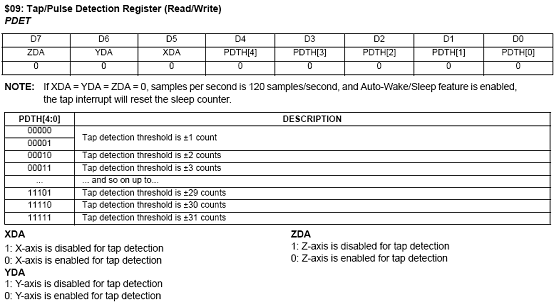