Android Bluetooth framework
Based on Android 9 0 AMLOGIC code analysis, Bluetooth applications communicate with Bluetooth processes through Binder. The Bluetooth process uses JNI to communicate with the Bluetooth stack and provides developers with access to various Bluetooth configuration files. The following figure shows the general structure of the Bluetooth stack:
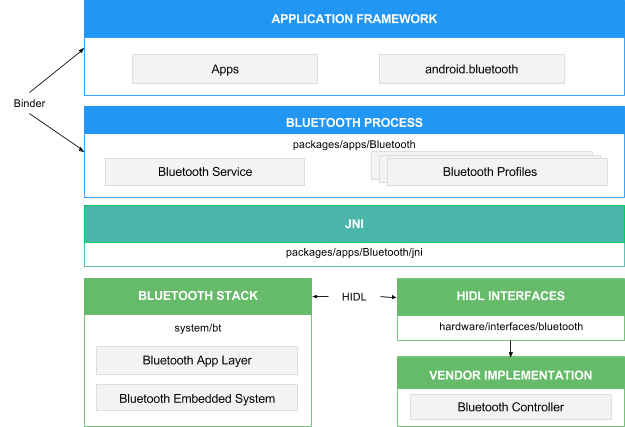
fluoride_architecture.png
Application framework client: frameworks/base/core/java/android/bluetooth/* servie: framework/base/services/core/java/com/android/server/BluetoothService.java framework/base/services/core/java/com/android/server/BluetoothManagerService.java
This part mainly provides APIs for app calls. Like other system services, it is divided into client side and service side, which communicate through binder
Bluetooth system service Bluetooth system services (located in packages/apps/Bluetooth) are packaged as Android applications, and Bluetooth services and configuration files are implemented in the Android framework layer. This application calls the native Bluetooth stack through JNI.
JNI With Android The JNI code associated with Bluetooth is located in packages/apps/Bluetooth/jni. When a specific Bluetooth operation occurs (for example, when a device is discovered), the JNI code will call the Bluetooth stack.
Bluetooth stack A default Bluetooth stack (located in system/bt) is provided in AOSP. This stack implements conventional Bluetooth HAL and customizes it through extenders and configuration changes
Supplier implementation Vendor devices interact with the Bluetooth stack using hardware interface design language (HIDL).
framework and bluetoothk app
The android Bluetooth framework layer is a little different from other system services. The services in the framework layer are mainly bound to the service of the bluetooth app, and most of the functional logic is implemented in the bluetooth app
When opening Bluetooth, mManagerService will indirectly call the handleEnable method. The dobind of handleEnable will bind Bluetooth service, call back to the Bluetooth serviceconnection method, and assign the service to mBluetooth, so that we can get the service(AdapterService) in the Bluetooth app for operation
//When Bluetooth is turned on, the handleEnable method will be called indirectly private void handleEnable(boolean quietMode) { mQuietEnable = quietMode; try { mBluetoothLock.writeLock().lock(); if ((mBluetooth == null) && (!mBinding)) { //Bind bluetooth app Service if (!doBind(i, mConnection, Context.BIND_AUTO_CREATE | Context.BIND_IMPORTANT, UserHandle.CURRENT)) { mHandler.removeMessages(MESSAGE_TIMEOUT_BIND); } else { mBinding = true; } } else if (mBluetooth != null) { } } finally { mBluetoothLock.writeLock().unlock(); } } boolean doBind(Intent intent, ServiceConnection conn, int flags, UserHandle user) { ComponentName comp = intent.resolveSystemService(mContext.getPackageManager(), 0); intent.setComponent(comp); if (comp == null || !mContext.bindServiceAsUser(intent, conn, flags, user)) { Slog.e(TAG, "Fail to bind to: " + intent); return false; } return true; } private BluetoothServiceConnection mConnection = new BluetoothServiceConnection(); private class BluetoothServiceConnection implements ServiceConnection { public void onServiceConnected(ComponentName componentName, IBinder service) { String name = componentName.getClassName(); if (DBG) { Slog.d(TAG, "BluetoothServiceConnection: " + name); } //After the service is bound successfully, it will call back. The first step is to bind AdapterService first, //Then start the GattService through the AdapterService, and Message msg = mHandler.obtainMessage(MESSAGE_BLUETOOTH_SERVICE_CONNECTED); if (name.equals("com.android.bluetooth.btservice.AdapterService")) { msg.arg1 = SERVICE_IBLUETOOTH; } else if (name.equals("com.android.bluetooth.gatt.GattService")) { msg.arg1 = SERVICE_IBLUETOOTHGATT; } else { Slog.e(TAG, "Unknown service connected: " + name); return; } msg.obj = service; mHandler.sendMessage(msg); } public void onServiceDisconnected(ComponentName componentName) { } } case MESSAGE_BLUETOOTH_SERVICE_CONNECTED: { if (DBG) { Slog.d(TAG, "MESSAGE_BLUETOOTH_SERVICE_CONNECTED: " + msg.arg1); } IBinder service = (IBinder) msg.obj; try { mBluetoothLock.writeLock().lock(); if (msg.arg1 == SERVICE_IBLUETOOTHGATT) { mBluetoothGatt = IBluetoothGatt.Stub.asInterface(Binder.allowBlocking(service)); continueFromBleOnState(); break; } // else must be SERVICE_IBLUETOOTH //Remove timeout mHandler.removeMessages(MESSAGE_TIMEOUT_BIND); mBinding = false; mBluetoothBinder = service; mBluetooth = IBluetooth.Stub.asInterface(Binder.allowBlocking(service)); //Do enable request try { if (!mQuietEnable) { if (!mBluetooth.enable()) { Slog.e(TAG, "IBluetooth.enable() returned false"); } } else { if (!mBluetooth.enableNoAutoConnect()) { Slog.e(TAG, "IBluetooth.enableNoAutoConnect() returned false"); } } }
After successfully binding with the bluetooth app service, the Bluetooth managerservice will obtain two service binder objects of the bluetooth app mBluetooth is bound with AdapterService mBluetoothGatt is bound to GattService
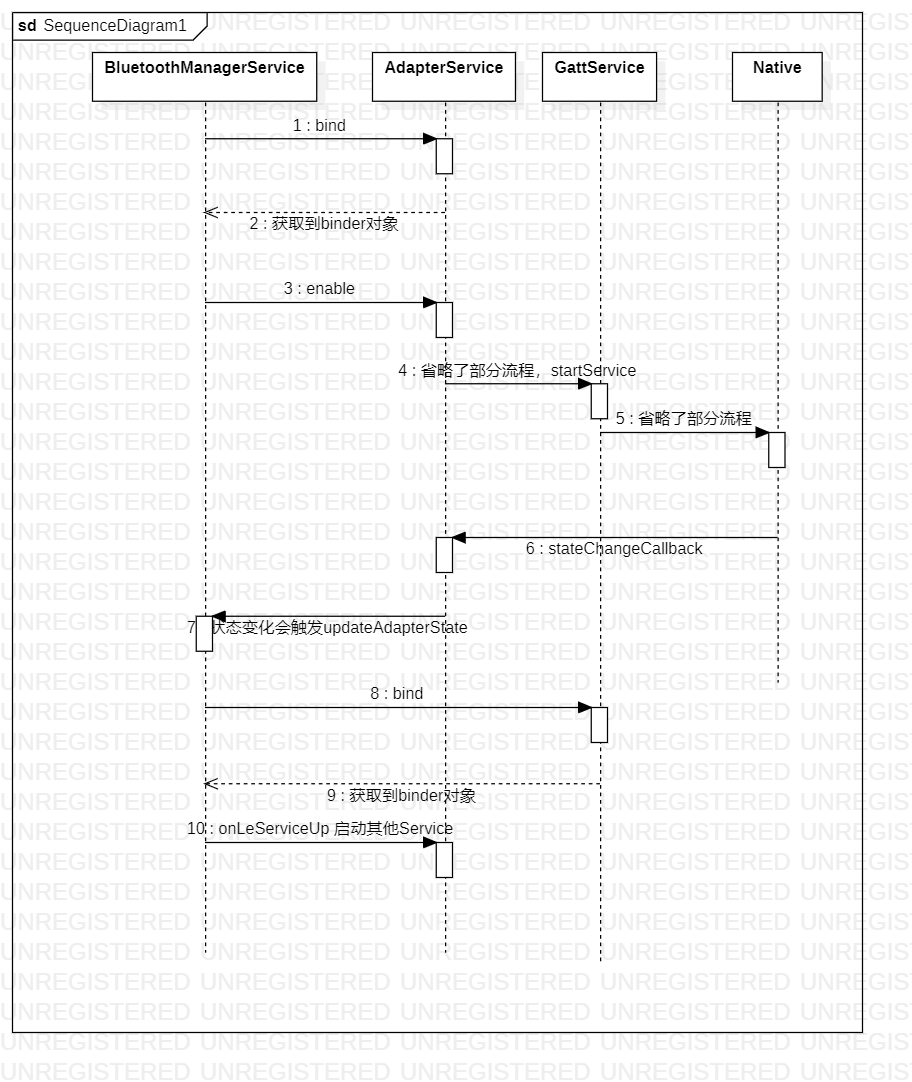
frame_app.png
Summary:
- Bluetooth managerservice binds AdapterService through bind. After the connection is successful, it obtains the binder object in AdapterService through the callback onServiceConnected
- Call AdapterService enable to trigger the enable process. In the enable process, GattService will be started to open Bluetooth and Ble.
- After Bluetooth is successfully opened, the native will call back the AdapterService. The AdapterService will continue to call the state machine for processing, and the AdapterService will call back bluetoothmanagerservice bluetoothstatechangehandler every time the state machine changes
- After receiving the callback, Bluetooth managerservice will bind GattService through bind again
- After successful binding, continue from bleonstate() -- > onleserviceup process will be followed to start other services in bluetooth app
See the next section for a relatively detailed process
bluetooth app
Bluetooth apk is the specific implementation of Bluetooth function logic. The general open / close function is implemented at the AdapterService portal. These methods will eventually be traced to the native method
enable process
When the upper layer or the enable interface is set to open Bluetooth, it will call adapterservice The general sequence diagram of java interface enable is as follows:
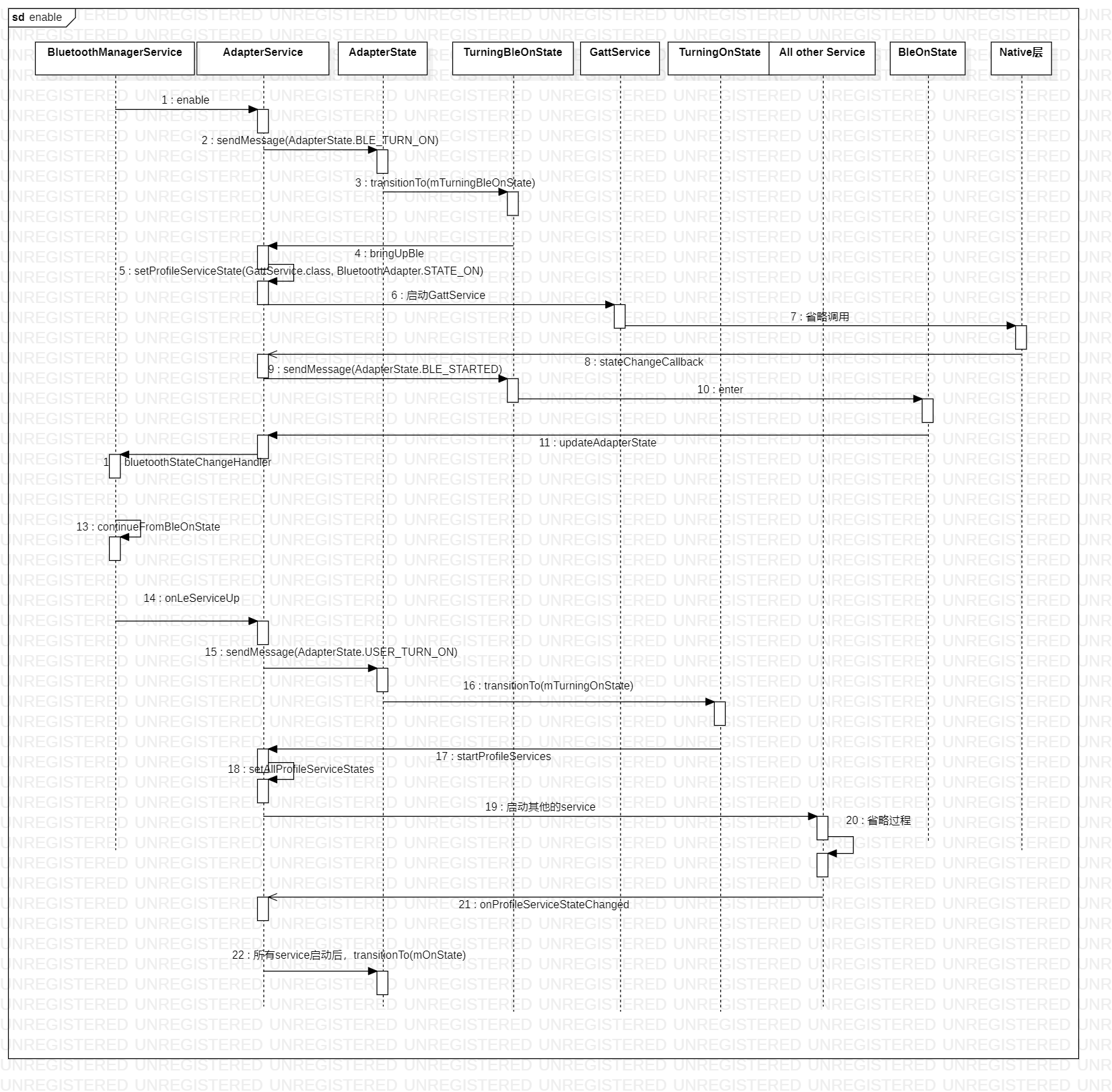
SequenceDiagram1.png
AdapterService performs state transition through AdapterState
private AdapterState mAdapterStateMachine; public synchronized boolean enable(boolean quietMode) { ... mAdapterStateMachine.sendMessage(AdapterState.BLE_TURN_ON); }
AdapterState.java code snippet
private TurningOnState mTurningOnState = new TurningOnState(); private TurningBleOnState mTurningBleOnState = new TurningBleOnState(); private TurningOffState mTurningOffState = new TurningOffState(); private TurningBleOffState mTurningBleOffState = new TurningBleOffState(); private OnState mOnState = new OnState(); private OffState mOffState = new OffState(); private BleOnState mBleOnState = new BleOnState(); private AdapterState(AdapterService service) { super(TAG); addState(mOnState); addState(mBleOnState); addState(mOffState); addState(mTurningOnState); addState(mTurningOffState); addState(mTurningBleOnState); addState(mTurningBleOffState); mAdapterService = service; setInitialState(mOffState); }
AdapterState is a state machine. When the state machine changes the state, it will execute some behaviors of the class. The constructor defaults to mOffState and receives BLE_TURN_ON message. The first place is the processMessage processing ble of the OffState_ TURN_ On, the message is also delivered directly to the TurningBleOnState
AdapterState.java code snippet
private class OffState extends BaseAdapterState { ... @Override public boolean processMessage(Message msg) { switch (msg.what) { case BLE_TURN_ON: transitionTo(mTurningBleOnState); break; } }
private class TurningBleOnState extends BaseAdapterState { ... @Override public void enter() { super.enter(); sendMessageDelayed(BLE_START_TIMEOUT, BLE_START_TIMEOUT_DELAY); mAdapterService.bringUpBle(); }
Here, the AdapterService will be called to start the service
void bringUpBle() { ... //Start Gatt service setProfileServiceState(GattService.class, BluetoothAdapter.STATE_ON); } private void setProfileServiceState(Class service, int state) { Log.e(TAG, "setProfileServiceStat :" + service.getSimpleName()); Intent intent = new Intent(this, service); intent.putExtra(EXTRA_ACTION, ACTION_SERVICE_STATE_CHANGED); intent.putExtra(BluetoothAdapter.EXTRA_STATE, state); startService(intent); }
Many services in bluetooth apk inherit from ProfileService. Here, start startService and call onStartCommand of ProfileService. After the service is started, it will call back to AdapterService
ProfileService.java code snippet
private void doStart() { // Callback to AdapterService MESSAGE_PROFILE_SERVICE_REGISTERED mAdapterService.addProfile(this); //Call the start method of the corresponding subclass mProfileStarted = start(); if (!mProfileStarted) { Log.e(mName, "Error starting profile. start() returned false."); return; } // Callback to AdapterService MESSAGE_PROFILE_SERVICE_REGISTERED mAdapterService.onProfileServiceStateChanged(this, BluetoothAdapter.STATE_ON); }
This is the first step. Start GattService first.
Start other services
After Bluetooth is turned on successfully, the JNI layer will call back the message to the AdapterService for processing through the stateChangeCallback method of JniCallbacks, and the AdapterService will continue to send the message to the state machine for processing:
AdapterService.java code snippet:
void stateChangeCallback(int status) { if (status == AbstractionLayer.BT_STATE_OFF) { debugLog("stateChangeCallback: disableNative() completed"); mAdapterStateMachine.sendMessage(AdapterState.BLE_STOPPED); } else if (status == AbstractionLayer.BT_STATE_ON) { mAdapterStateMachine.sendMessage(AdapterState.BLE_STARTED); } else { Log.e(TAG, "Incorrect status " + status + " in stateChangeCallback"); } }
At this time, the AdapterState is in the TurningBleOnState state, and the TurningBleOnState receives ble_ After the started message, move to the BleOnState state:
AdapterState.java code snippet:
private class TurningBleOnState extends BaseAdapterState { ....... @Override public boolean processMessage(Message msg) { switch (msg.what) { case BLE_STARTED: transitionTo(mBleOnState); break; ........ }
Each state migration will trigger updateAdapterState of AdapterService. This method will call back the state change to the remote listener:
AdapterState.java code snippet:
private abstract class BaseAdapterState extends State { abstract int getStateValue(); @Override public void enter() { int currState = getStateValue(); infoLog("entered "); mAdapterService.updateAdapterState(mPrevState, currState); mPrevState = currState; }
AdapterService.java code snippet:
void updateAdapterState(int prevState, int newState) { mAdapterProperties.setState(newState); if (mCallbacks != null) { int n = mCallbacks.beginBroadcast(); debugLog("updateAdapterState() - Broadcasting state " + BluetoothAdapter.nameForState( newState) + " to " + n + " receivers."); for (int i = 0; i < n; i++) { try { mCallbacks.getBroadcastItem(i).onBluetoothStateChange(prevState, newState); //Remote callback } catch (RemoteException e) { debugLog("updateAdapterState() - Callback #" + i + " failed (" + e + ")"); } } mCallbacks.finishBroadcast(); }
Here, a remote callback will be made to the listening interface in BluetoothManagerService, and the bluetoothStateChangeHandler method will be called through the handler message processing mechanism:
BluetoothManagerService.java code snippet
private class BluetoothHandler extends Handler { ... @Override public void handleMessage(Message msg) { case MESSAGE_BLUETOOTH_SERVICE_CONNECTED: { IBinder service = (IBinder) msg.obj; try { mBluetoothLock.writeLock().lock(); if (msg.arg1 == SERVICE_IBLUETOOTHGATT) { mBluetoothGatt = IBluetoothGatt.Stub.asInterface(Binder.allowBlocking(service)); continueFromBleOnState(); break; } private void continueFromBleOnState() { ... mBluetooth.onLeServiceUp(); }
mBluetooth.onLeServiceUp() called AdapterService
AdapterService.java code snippet
void onLeServiceUp() { mAdapterStateMachine.sendMessage(AdapterState.USER_TURN_ON); }
Next, it is similar to the above process of starting gattService. Through state machine switching, call AdapterService and start
AdapterState.java code snippet
private class TurningOnState extends BaseAdapterState { @Override public void enter() { ... mAdapterService.startProfileServices(); }
AdapterService.java code snippet
void startProfileServices() { Class[] supportedProfileServices = Config.getSupportedProfiles(); ... setAllProfileServiceStates(supportedProfileServices, BluetoothAdapter.STATE_ON); } } private void setAllProfileServiceStates(Class[] services, int state) { for (Class service : services) { if (GattService.class.getSimpleName().equals(service.getSimpleName())) { continue; } setProfileServiceState(service, state); } } private void setProfileServiceState(Class service, int state) { Intent intent = new Intent(this, service); intent.putExtra(EXTRA_ACTION, ACTION_SERVICE_STATE_CHANGED); intent.putExtra(BluetoothAdapter.EXTRA_STATE, state); startService(intent); }
Here, use the for loop to start all services in supportedProfileServices. The services here inherit from ProfileService. After each service is started, it will call back to AdapterService. After all services are started, it will go to the following else if process
private void processProfileServiceStateChanged(ProfileService profile, int state) { switch (state) { case BluetoothAdapter.STATE_ON: if (GattService.class.getSimpleName().equals(profile.getName())) { enableNativeWithGuestFlag(); } else if (mRegisteredProfiles.size() == Config.getSupportedProfiles().length - 1 && mRegisteredProfiles.size() == mRunningProfiles.size()) { mAdapterProperties.onBluetoothReady(); updateUuids(); setBluetoothClassFromConfig(); mAdapterStateMachine.sendMessage(AdapterState.BREDR_STARTED); } break;
app startup summary:
Picture from https://blog.csdn.net/yus201120/article/details/119038589
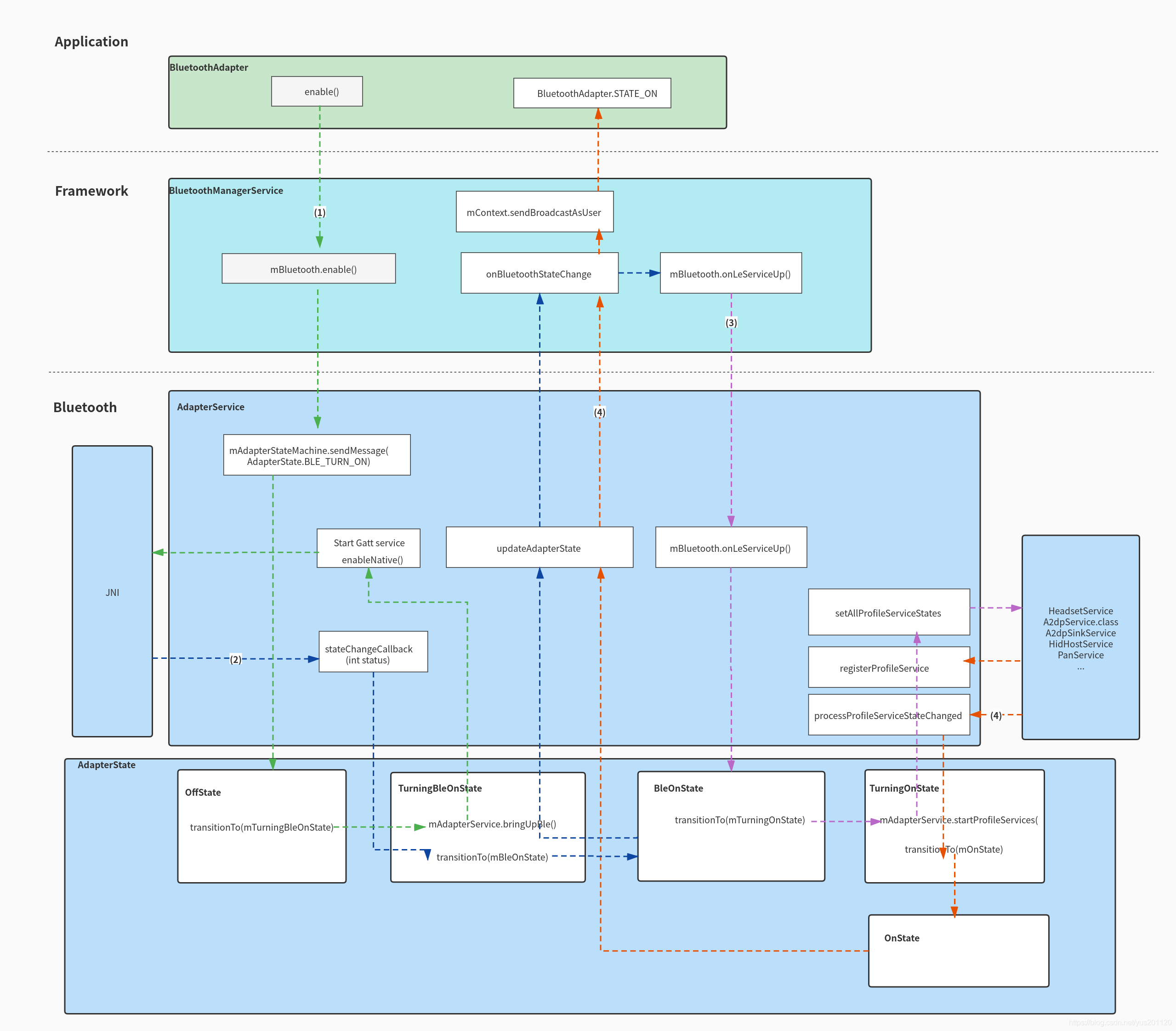
beac4f61a8a842d08d0ecc0eb70207cd.png
reference resources: https://source.android.google.cn/devices/bluetooth?hl=zh-cn https://www.jianshu.com/p/b15314d91df0 https://blog.csdn.net/yus201120/article/details/119038589