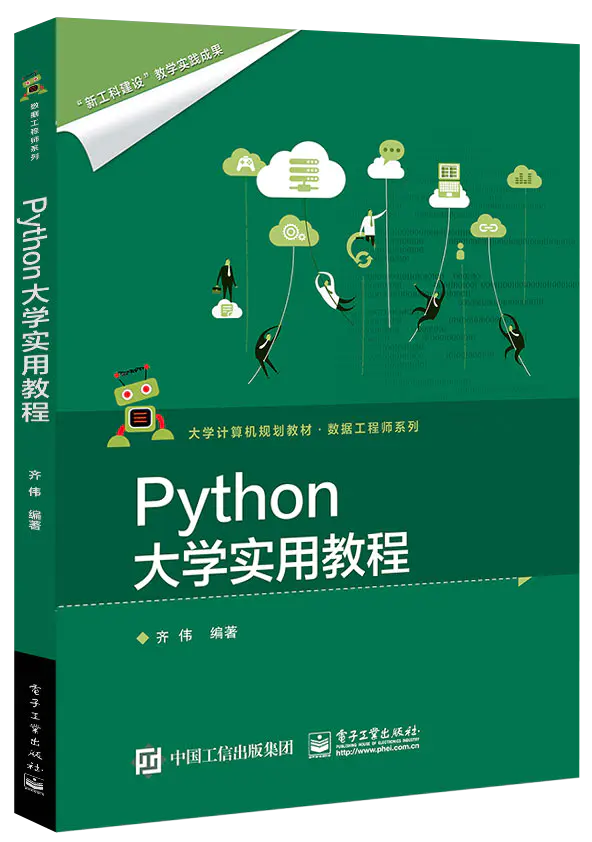
Compared with other programming languages, Python has more diversified operation types.
In particular, the asterisk (*), which is a widely used operator in Python, is not only used in the multiplication of two numbers. In this article, we will discuss the various uses of asterisks.
The application scenarios of four kinds of asterisks are summarized here:
- Operators as multiplication and power
- Represents the repetition of elements in a sequence
- Used to collect parameters (which can be called "packaging")
- Unpacking for container class objects
It is described one by one below. Note: many people will encounter all kinds of problems in the process of learning python. No one can easily give up. For this reason, I have built a python full stack for free answer. Skirt: seven clothes, 977 bars and five (homophony of numbers) can be found under conversion. The problems that I don't understand are solved by the old driver and the latest Python practical course. We can supervise each other and make progress together!
Operator of multiplication or power
You must be familiar with this. Like multiplication, Python also has a built-in multiplier operator.
>>> 2 * 3 6 >>> 2 ** 3 8 >>> 1.414 * 1.414 1.9993959999999997 >>> 1.414 ** 1.414 1.6320575353248798 Copy code
Container element of duplicate class list
Python also supports the multiplication of container class objects (i.e. sequences) of class lists by integers, that is, the number of elements repeated by integer implementation.
# Initialize the zero-valued list with 100 length
zeros_list = [0] * 100
# Declare the zero-valued tuple with 100 length
zeros_tuple = (0,) * 100
# Extending the "vector_list" by 3 times
vector_list = [[1, 2, 3]]
for i, vector in enumerate(vector_list * 3):
print("{0} scalar product of vector: {1}".format((i + 1), [(i + 1) * e for e in vector]))
# 1 scalar product of vector: [1, 2, 3]
# 2 scalar product of vector: [2, 4, 6]
# 3 scalar product of vector: [3, 6, 9]
Copy code
Parameter collection
In many functions, there are uncertain parameters. For example, if we don't know how many parameters to provide, or why we have to pass any parameters, etc.
In Python, there are two types of parameters, one is the location parameter, the other is the keyword parameter. The former determines the corresponding value according to the location, and the latter is determined according to the parameter name.
Before studying any position / keyword parameter, we first discuss how to determine the number of position parameters and keyword parameters.
# A function that shows the results of running competitions consisting of 2 to 4 runners.
def save_ranking(first, second, third=None, fourth=None):
rank = {}
rank[1], rank[2] = first, second
rank[3] = third if third is not None else 'Nobody'
rank[4] = fourth if fourth is not None else 'Nobody'
print(rank)
# Pass the 2 positional arguments
save_ranking('ming', 'alice')
# Pass the 2 positional arguments and 1 keyword argument
save_ranking('alice', 'ming', third='mike')
# Pass the 2 positional arguments and 2 keyword arguments (But, one of them was passed as like positional argument)
save_ranking('alice', 'ming', 'mike', fourth='jim')
Copy code
The function in the above code has two position parameters: first and second, and two keyword parameters: third and fourth. Position parameters cannot be omitted. All position parameters must be passed values according to their correct positions. However, for keyword parameters, you can set the default value when defining a function. If you omit the corresponding argument when calling a function, the default value will be used as the argument, that is, keyword parameters can be omitted.
As you can see, keyword parameters can be omitted, so they cannot be declared before unknown parameters. If parameters are declared in the following way, an exception will be thrown.
def save_ranking(first, second=None, third, fourth=None):
...
Copy code
However, in the save [ranking ('Alice ',' Ming ',' mike ', fourth ='Jim') call, three position arguments and a keyword parameter are provided. Yes, for keyword parameters, you can also transfer values in the way of location parameters. The corresponding keywords can accept the data transferred by location. According to the call method here, 'mike' is automatically passed to third.
We have discussed the basic meaning of parameters above. From the above example, we can see that the function defined above cannot receive any number of parameters, because the parameters of the function are fixed. Therefore, the function needs to be modified to receive any parameter, whether position parameter or keyword parameter. See the following example:
Collect location parameters
def save_ranking(*args):
print(args)
save_ranking('ming', 'alice', 'tom', 'wilson', 'roy')
# ('ming', 'alice', 'tom', 'wilson', 'roy')
Copy code
Collect location and keyword parameters
def save_ranking(*args, **kwargs):
print(args)
print(kwargs)
save_ranking('ming', 'alice', 'tom', fourth='wilson', fifth='roy')
# ('ming', 'alice', 'tom')
# {'fourth': 'wilson', 'fifth': 'roy'}
Copy code
In the above example, * args means to collect any number of location parameters, * * kwargs means to collect any number of keyword parameters. Here * args and * * kwargs can be called packing.
As you can see, we have passed any number of parameters according to the position or keyword above. Parameters passed by location are collected into tuples and referenced by variable args; parameters passed by keyword are referenced by variable kwargs as dictionary type.
As mentioned earlier, keyword parameters cannot be written in front of position parameters. Therefore, the following definitions are wrong:
def save_ranking(**kwargs, *args): ... Copy code
Any number of parameters is very valuable. It can be seen in many open source projects. Generally, * args or * * kwargs are used as the name of collecting any parameter. Of course, you can use other names, such as * requested or * * optional. Just for open source projects, we are used to using * args and * * kwargs.
Unpacking
The asterisk can also be used to unpack the container, which is similar to the previous parameter collection. For example, there is a list, tuple or dictionary containing data, and a function to collect any parameter:
from functools import reduce
primes = [2, 3, 5, 7, 11, 13]
def product(*numbers):
p = reduce(lambda x, y: x * y, numbers)
return p
product(*primes)
# 30030
product(primes)
# [2, 3, 5, 7, 11, 13]
Copy code
Because product() can take any parameter, we need to take out the elements in the list and pass them to this function. But here, if the primes list data is provided to the function in the way of * primes, the list referenced by primes will be unpacked, and each prime number in it will be passed to the function, and then it will be referenced with the variable numbers after collection. If you pass the list primes to a function, you cannot unpack it. There is only one primes list in the tuple referenced by numbers.
This is also true for tuples. For dictionaries, you need to use * * instead of *.
headers = {
'Accept': 'text/plain',
'Content-Length': 348,
'Host': 'http://mingrammer.com'
}
def pre_process(**headers):
content_length = headers['Content-Length']
print('content length: ', content_length)
host = headers['Host']
if 'https' not in host:
raise ValueError('You must use SSL for http communication')
pre_process(**headers)
# content length: 348
# Traceback (most recent call last):
# File "<stdin>", line 1, in <module>
# File "<stdin>", line 7, in pre_process
# ValueError: You must use SSL for http communication
Copy code
There are many ways to unpack, not even for functions, but to extract data from lists and tuples, and reference them with dynamic variables.
numbers = [1, 2, 3, 4, 5, 6]
# The left side of unpacking should be list or tuple.
*a, = numbers
# a = [1, 2, 3, 4, 5, 6]
*a, b = numbers
# a = [1, 2, 3, 4, 5]
# b = 6
a, *b, = numbers
# a = 1
# b = [2, 3, 4, 5, 6]
a, *b, c = numbers
# a = 1
# b = [2, 3, 4, 5]
# c = 6
Copy code
In the above operation, it is explained that the data obtained from unpacking can be unpacked from the list or tuple respectively, and the corresponding value can be obtained. Then * A and * b are used to reference the unpacked data and package it as a list. This is the same concept as any number of parameters mentioned above.
conclusion
The above briefly introduces the asterisk (*) in Python language. As an operator, it has many uses, especially in "collect parameters", which is very important. However, beginners are easy to be confused here, so if you are a beginner, please take a look at the above content carefully.
Note: many people will encounter all kinds of problems in the process of learning python. No one can easily give up. For this reason, I have built a python full stack for free answer. Skirt: seven clothes, nine seven seven bars and five (homophony of numbers) can be found under conversion. The problems that I don't understand are solved by the old driver and the latest Python practical course. We can supervise each other and make progress together!
The text and pictures of this article come from the Internet and my own ideas. They are only for learning and communication. They have no commercial use. The copyright belongs to the original author. If you have any questions, please contact us in time for handling.