1. Content outline
1. New attribute
- placeholder
- Calendar, date, time, email, url, search
- ContentEditable
- Draggable
- Hidden
- Content-menu
- Data val (custom attribute)
2. New label
- Semantic label
- canvas
- svg
- Audio (sound playback)
- Video (video playback)
3.API
- Mobile web development generally refers to h5
- Location required
- Gravity sensing (gyroscope in mobile phone (wechat shake, car turn))
- Request animation frame
- History interface (control the history of the current page)
- Local storage (local storage will be retained when the computer / browser is closed); SessionStorage (session storage: disappears when the window is closed). All stored information (such as historical records)
- WebSocket (online chat, chat room)
- FileReader (file reading, preview)
- WebWoker (file asynchrony, improving performance and interactive experience)
- Fetch (legendary replacement for AJAX)
2. Attribute_ input new type
1.placeholder
<input type="text" placeholder="user name/mobile phone/mailbox"> <input type="password" placeholder="Please input a password">
2.input type added
I learned before
<input type="radio"> <input type="checkbox"> <input type="file">
input new type
<form> <!-- Calendar class --> <input type="date"><!-- One of the reasons why it is not commonly used: chrome support, Safari,IE I won't support it --> <input type="time"><!-- One of the reasons why it is not commonly used: chrome support, Safari,IE I won't support it --> <input type="week"><!-- One of the reasons why it is not commonly used in the first few weeks: chrome support, Safari,IE I won't support it --> <input type="datetime-local"><!-- One of the reasons why it is not commonly used: chrome support, Safari,IE I won't support it --> <br> <input type="number"><!-- Limit input, only numbers can be. One of the reasons why it is not commonly used: chrome support, Safari,IE I won't support it--> <input type="email"><!-- One of the reasons why mailbox format is not commonly used: chrome,Firefox support, Safari,IE I won't support it--> <input type="color"><!-- One of the reasons why color selectors are not commonly used: chrome support, Safari,IE I won't support it--> <input type="range" min="1" max="100" name="range"><!-- chrome,Safar Support, Firefox, IE I won't support it--> <input type="search" name="search"><!-- Automatic prompt History Search.chrome support,Safar Support a little,IE I won't support it --> <input type="url"><!-- chrome,Firefox support,Safar,IE I won't support it --> <input type="submit"> </form>
3.ContentEditable
<div>Panda</div>
Want to modify the content
Native method: add click event modification
new method
<div contenteditable="true">Panda</div>
The default value is false. There is no compatibility problem. It can inherit (the child element of the package) and overwrite (the later setting overrides the previous setting)
Common misunderstandings:
<div contenteditable="true"> <span contenteditable="false">full name:</span>Panda<br> <span contenteditable="false">Last name:</span>male<br> <!-- Will result in deletion br Such label --> </div>
Summary: available attributes for actual combat development: contentable, placeholder
4.Drag dragged element
<div class="a" draggable="true"></div><!-- Google, safari Yes, Firefox, Ie I won't support it -->
The default value is false
Tags with the default value of true (tags with drag and drop function by default): a tag and img tag
Drag lifecycle
1. Drag starts, drag is in progress, and drag ends
2. Composition: dragged object, target area
Drag event
var oDragDiv = document.getElementsByClassName('a')[0]; oDragDiv.ondragstart = function (e) { console.log(e); }// The moment you press an object does not trigger an event, and only drag will trigger a start event oDragDiv.ondrag = function (e) {//Mobile event console.log(e); } oDragDiv.ondragend = function (e) { console.log(e); } // So we can figure out how many points to move
Small functions: a{position:absolute}
<div class="a" draggable="true"></div> <script> var oDragDiv = document.getElementsByClassName('a')[0]; var beginX = 0; var beginY = 0; oDragDiv.ondragstart = function (e) { beginX = e.clientX; beginY = e.clientY; console.log(e); } oDragDiv.ondragend = function (e) { var x = e.clientX - beginX; var y = e.clientY - beginY; oDragDiv.style.left = oDragDiv.offsetLeft + x + "px"; oDragDiv.style.top = oDragDiv.offsetTop + y + "px"; } </script>
5.Drag target element (target area)
.a { width: 100px; height: 100px; background-color: red; position: absolute; } .target{ width: 200px; height: 200px; border:1px solid; position: absolute; left: 600px; }
<div class="a" draggable="true"></div> <div class="target"></div> <script> var oDragDiv = document.getElementsByClassName('a')[0]; oDragDiv.ondragstart = function (e) { } oDragDiv.ondrag = function (e) { //As long as you move, you keep triggering } oDragDiv.ondragend = function (e) { } var oDragTarget = document.getElementsByClassName("target")[0]; oDragTarget.ondragenter = function (e) {//It is triggered not by the entry of element graphics, but by dragging the mouse // console.log(e); } oDragTarget.ondragover = function (e) { //Similar to ondrag, it is triggered as long as it moves in the area // console.log(e); // ondragover -- return to the original location / execute the drop event } oDragTarget.ondragleave = function (e) { console.log(e); // Trigger when you leave } oDragTarget.ondrop = function (e) { console.log(e); // For all tag elements, when the drag cycle ends, the default event is to return to the original location. To execute ondrop, you must add e.preventDefault() in ondragover; // An event is triggered by an action. An action can trigger more than one event // When lifting, there is an event that ondragover returns to its original location by default. As long as it is prevented from returning to its original location, the drop event can be executed // A - > b (blocking) - > c wants to block c, only the responsibility chain mode can be blocked on B } </script>
6. Drag the demo
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> * { padding: 0; margin: 0; } .box1 { position: absolute; width: 150px; height: auto; border: 1px solid; padding-bottom: 10px; } .box2 { position: absolute; width: 150px; left: 300px; height: auto; border: 1px solid; padding-bottom: 10px; } li { position: relative; width: 100px; height: 30px; background: #abcdef; margin: 10px auto 0px auto; /* Center, up and down 10px */ list-style: none; } </style> </head> <body> <div class="box1"> <ul> <li></li> <li></li> <li></li> </ul> </div> <div class="box2"></div> <script> var dragDom; var liList = document.getElementsByTagName('li'); for (var i = 0; i < liList.length; i++) { liList[i].setAttribute("draggable", true);//Assign class liList[i].ondragstart = function (e) { console.log(e.target); dragDom = e.target; } } var box2 = document.getElementsByClassName("box2")[0]; box2.ondragover = function (e) { e.preventDefault(); } box2.ondrop = function (e) { // console.log(dragDom); box2.appendChild(dragDom); dragDom = null; } // Drag it back var box1 = document.getElementsByClassName("box1")[0]; box1.ondragover = function (e) { e.preventDefault(); } box1.ondrop = function (e) { // console.log(dragDom); box1.appendChild(dragDom); dragDom = null; } </script> </body> </html>
7.dataTransfer supplementary properties
effectAllowed
oDragDiv.ondragstart = function (e) { e.dataTransfer.effectAllowed = "link";//What the pointer looks like can only be set in ondragstart } /Other cursors: link copy move copyMove linkMove all
dropEffect
oDragTarget.ondrop = function (e) { e.dataTransfer.dropEffect = "link";//The effect when dropping is only set in drop }
Test failed??
8. Label_ Semantic label
It's all div, just semantic
<header></header> <footer></footer> <nav></nav> <article></article><!--Articles can be directly cited and taken away--> <section></section><!--Paragraph structure, general section Put on article inside--> <aside></aside><!--sidebar -->
9.canvas line drawing
<canvas id="can" width="500px" height="300px"></canvas>
Note: the size can only be set in the inter line style, not through css
var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush
ctx.moveTo(100, 100);//starting point ctx.lineTo(200, 100);//End ctx.stroke();//Draw it ctx.closePath();// Continuous line, forming a closed ctx.fill();//fill ctx.lineWidth = 10;// Setting where the line is written is equivalent to writing after moveto???? Why doesn't it work
Want to achieve a thin, a thick
A figure, drawn by one stroke, can only be of one thickness. To achieve this, you must open a new image
ctx.beginPath();
closePath() is a closed graph, not a graph, and cannot be closed
10 canvas draw rectangle
ctx.rect(100, 100, 150, 100); ctx.stroke(); ctx.fill();
simplify
ctx.strokeRect(100, 100, 200, 100);//rectangle ctx.fillRect(100, 100, 200, 100); //Fill rectangle
11. Small square falling
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> * { padding: 0; margin: 0; } canvas { width: 500px; height: 300px; border: 1px solid; } </style> </head> <body> <canvas id="can" width="500px" height="300px"></canvas> <!-- --> <script> var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush var height = 100; var timer = setInterval(function () { ctx.clearRect(0, 0, 500, 300);//Eraser function, clear screen ctx.strokeRect(100, height, 50, 50); height += 5;//Every time I draw a new one, but the old one is not deleted, so I need to add a clear screen }, 1000 / 30); </script> </body> </html>
Operation: free fall
12.canvas drawing circle
<script> var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush // Center (x,y), radius (r), radian (start radian, end radian), direction ctx.arc(100, 100, 50, 0, Math.PI * 1.8, 0)//Clockwise 0; Counterclockwise 1 ctx.lineTo(100, 100); ctx.closePath(); ctx.stroke(); </script>
13. Draw rounded rectangle
<script> var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush // ABC is the end of the rectangle // B(x,y),C(x,y), fillet size (equivalent to border radius) ctx.moveTo(100, 110); ctx.arcTo(100, 200, 200, 200, 10); ctx.arcTo(200, 200, 200, 100, 10); ctx.arcTo(200, 100, 100, 100, 10); ctx.arcTo(100, 100, 100, 200, 10); ctx.stroke(); </script>
14.canvas Bezier curve
Bessel curve
var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush ctx.beginPath(); ctx.moveTo(100, 100); // ctx.quadraticCurveTo(200, 200, 300, 100); secondary // ctx.quadraticCurveTo(200, 200, 300, 100, 400 200); Three times ctx.stroke();
wave
Note: initialization
ctx.beginPath();
Wave demo
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> canvas { width: 500px; height: 300px; border: 1px solid; } </style> </head> <body> <canvas id="can" width="500px" height="300px"></canvas> <!-- --> <script> var width = 500; var height = 300; var offset = 0; var num = 0; var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush setInterval(function () { ctx.clearRect(0, 0, 500, 300); ctx.beginPath(); ctx.moveTo(0 + offset - 500, height / 2); ctx.quadraticCurveTo(width / 4 + offset - 500, height / 2 + Math.sin(num) * 120, width / 2 + offset - 500, height / 2); ctx.quadraticCurveTo(width / 4 * 3 + offset - 500, height / 2 - Math.sin(num) * 120, width + offset - 500, height / 2); // Shift the whole width to the left to form a complete connection ctx.moveTo(0 + offset, height / 2); ctx.quadraticCurveTo(width / 4 + offset, height / 2 + Math.sin(num) * 120, width / 2 + offset, height / 2); ctx.quadraticCurveTo(width / 4 * 3 + offset, height / 2 - Math.sin(num) * 120, width + offset, height / 2); ctx.stroke(); offset += 5; offset %= 500; num += 0.02; }, 1000 / 30) </script> </body> </html>
15. Coordinate translation, rotation and scaling
Rotation translation
<script> var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush ctx.beginPath(); ctx.rotate(Math.PI / 6);//Rotate according to the origin of the canvas // If you do not want to translate according to the canvas origin, translate the coordinate system // ctx.translate(100, 100); The coordinate origin is (100100), and the supporting rotation is at this time ctx.moveTo(0, 0); ctx.lineTo(100, 100); ctx.stroke(); </script>
zoom
<script> var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush ctx.beginPath(); ctx.scale(2, 2);//x times his coefficient, y times his coefficient ctx.strokeRect(100, 100, 100, 100); </script>
16. save and restore of canvas
I don't want others to be affected by the previous settings
<script> var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush ctx.save();//Save the translation data, scaling data and rotation data of the coordinate system ctx.beginPath(); ctx.translate(100, 100); ctx.rotate(Math.PI / 4); ctx.strokeRect(0, 0, 100, 50); ctx.beginPath(); ctx.restore();//Once restored, the state at the time of save will be restored ctx.fillRect(100, 0, 100, 50); </script>
17.canvas background filling
<script> var canvas = document.getElementById('can');//canvas var ctx = canvas.getContext("2d");//paint brush var img = new Image(); img.src = "file:///C:/Users/f1981/Desktop/source/pic3.jpeg"; img.onload = function () {//Because pictures are loaded asynchronously ctx.beginPath(); ctx.translate(100, 100);//Change the position of the coordinate system var bg = ctx.createPattern(img, "no-repeat"); // Picture filling starts with the origin of the coordinate system // ctx.fillStyle = "blue"; ctx.fillStyle = "bg"; ctx.fillRect(0, 0, 200, 100); } </script>
Picture problems
explore
open in live server can only open img in the same directory
18. Linear gradient
<script> var canvas = document.getElementById('can'); var ctx = canvas.getContext("2d"); ctx.beginPath(); var bg = ctx.createLinearGradient(0, 0, 200, 200); bg.addColorStop(0, "white");//The number is between 0 and 1 // bg.addColorStop(0.5, "blue"); bg.addColorStop(1, "black"); ctx.fillStyle = bg; ctx.translate(100, 100);//The starting point is still the origin of the coordinate system ctx.fillRect(0, 0, 200, 200); </script>
19.canvas radiation gradient
<script> var canvas = document.getElementById('can'); var ctx = canvas.getContext("2d"); ctx.beginPath(); // var bg = ctx.createRadialGradient(x1,y1,r1,x2,y2,r2); Start circle var bg = ctx.createRadialGradient(100, 100, 0, 100, 100, 100); // var bg = ctx.createRadialGradient(100, 100, 100, 100, 100, 100); The colors in the starting circle are all the starting colors bg.addColorStop(0, "red"); bg.addColorStop(0.5, "green"); bg.addColorStop(1, "blue"); ctx.fillStyle = bg; ctx.fillRect(0, 0, 200, 200); </script>
20.canvas shadow
<script> var canvas = document.getElementById('can'); var ctx = canvas.getContext("2d"); ctx.beginPath(); ctx.shadowColor = "blue"; ctx.shadowBlur = 20; ctx.shadowOffsetX = 15; ctx.shadowOffsetY = 15; ctx.strokeRect(0, 0, 200, 200); </script>
21.canvas rendered text
<script> var canvas = document.getElementById('can'); var ctx = canvas.getContext("2d"); ctx.beginPath(); ctx.strokeRect(0, 0, 200, 200); ctx.fillStyle = "red"; ctx.font = "30px Georgia";//It works for both stroke and fill ctx.strokeText("panda", 200, 100);//Text stroke ctx.fillText("monkey", 200, 250);//Text fill </script>
22.canvas line end style
<script> var canvas = document.getElementById('can'); var ctx = canvas.getContext("2d"); ctx.beginPath(); ctx.lineWidth = 15; ctx.moveTo(100, 100); ctx.lineTo(200, 100); ctx.lineTo(100, 130); ctx.lineCap = "square"//butt round ctx.lineJoin = "miter";//Line contact / / round bevel mitter (mitterlimit) ctx.miterLimit = 5; ctx.stroke(); </script>
23.SVG line drawing and rectangle
Difference between svg and canvas
svg: vector image. It will not be distorted when enlarged. It is suitable for large-area maps. Usually, there are fewer or simpler animations, labels and css drawings
Canvas: suitable for small area drawing, animation and js painting
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> .line1 { stroke: black; stroke-width: 3px; } .line2 { stroke: red; stroke-width: 5px; } </style> </head> <body> <svg width="500px" height="300px" style="border:1px solid"> <line x1="100" y1="100" x2="200" y2="100" class="line1"></line> <line x1="200" y1="100" x2="200" y2="200" class="line2"></line> <rect height="50" width="100" x="0" y="0"></rect><!-- All closed figures, in svg The default is that they are naturally full and drawn --> <rect height="50" width="100" x="0" y="0" rx="10" ry="20"></rect> </svg> </body> </html>
24.svg circle, ellipse, line
<style> polyline { fill: transparent; stroke: blueviolet; stroke-width: 3px; } </style>
<svg width="500px" height="300px" style="border:1px solid"> <circle r="50" cx="50" cy="220"></circle><!--circular--> <ellipse rx="100" ry="30" cx="400" cy="200"></ellipse><!-- ellipse --> <polyline points="0 0, 50 50, 50 100,100 100,100 50"></polyline><!-- curve:Default fill --> </svg>
25.svg draw polygons and text
<polygon points="0 0, 50 50, 50 100,100 100,100 50"> </polygon><!-- And polylkine Difference: polygons are automatically connected --> <text x="300" y="50">Brother Deng is in good health</text>
polygon { fill: transparent; stroke: black; stroke-width: 3px; } text { stroke: blue; stroke-width: 3px; }
26.SVG transparency and line style
transparent
stroke-opacity: 0.5;/* Translucent border */ fill-opacity: 0.3;/* Fill translucent */
Line style
stroke-linecap: butt;/* (round square is an extra length*/
stroke-linejoin:;/* bevel round miter when two lines intersect */
27. path tag of SVG
<path d="M 100 100 L 200 100"></path> <path d="M 100 100 L 200 100 L 200 200"></path><!-- Filled by default --> <path d="M 100 100 L 200 100 l 100 100"></path> <!-- Above: upper case letters represent absolute position and lower case letters represent relative position --> <path d="M 100 100 H 200 V 200"></path><!-- H level V vertical --> <path d="M 100 100 H 200 V 200 z"></path><!-- z Represents a closed interval, case insensitive -->
path { stroke: red; fill: transparent }
28.path draw arc
<path d="M 100 100 A 100 50 0 1 1 150 200"></path> <!-- A Represents the arc command to M100 100 150 is the starting point, 200 is the end point, radius 100, short radius 50, rotation angle 0, 1 large arc, 1 clockwise -->
29.svg linear gradient
<svg width="500px" height="300px" style="border:1px solid"> <defs> <!-- Define a gradient --> <linearGradient id="bg1" x1="0" y1="0" x2="0" y2="100%"> <stop offset="0%" style="stop-color:rgb(255,255,0)"></stop> <stop offset="100%" style="stop-color:rgb(255,0,0)"></stop> </linearGradient> </defs> <rect x="100" y="100" height="100" width="200" style="fill:url(#bg1)"></rect> </svg>
30.svg Gaussian blur
<svg width="500px" height="300px" style="border:1px solid"> <defs> <!-- Define a gradient --> <linearGradient id="bg1" x1="0" y1="0" x2="0" y2="100%"> <stop offset="0%" style="stop-color:rgb(255,255,0)"></stop> <stop offset="100%" style="stop-color:rgb(255,0,0)"></stop> </linearGradient> <filter id="Gaussian"> <feGaussianBlur in="SourceGraphic" stdDeviation="20"></feGaussianBlur> </filter> </defs> <rect x="100" y="100" height="100" width="200" style="fill:url(#bg1);filter:url(#Gaussian)"></rect> </svg>
31.SVG dotted line and simple animation
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <style> .line1 { stroke: black; stroke-width: 10px; /* stroke-dasharray: 10px; */ /* stroke-dasharray: 10px 20px;1,2,3,Take two values in sequence (array) */ /* stroke-dasharray: 10px 20px 30px;1,2,3,Take two values in sequence */ /* stroke-dashoffset: 10px;deviation */ /* stroke-dashoffset: 200px--->0;Can achieve full talk and empty feeling */ stroke-dashoffset: 200px; animation: move 2s linear infinite alternate-reverse; } @keyframes move { 0% { stroke-dashoffset: 200px; } 100% { stroke-dashoffset: 0px; } } </style> </head> <body> <svg width="500px" height="300px" style="border:1px solid"> <line x1="100" y1="100" x2="200" y2="100" class="line1"></line> </svg> </body> </html>
32.svg viewbox (scale bar)
<svg width="500px" height="300px" viewbox="0,0,250,150" style="border:1px solid"> <!-- viewbox It's half the width and half the height --> <line x1="100" y1="100" x2="200" y2="100" class="line1"></line> </svg>
Summary: not used in SVG development
33.audio and video player
<audio src="" controls></audio> <video src="" controls></video>
Above: too ugly, different browsers are not unified
34. Video player
Unable to load https://blog.csdn.net/qq_40340478/article/details/108309492
var express = require("express"); var app = new express(); app.use(express.static('./')); app.listen(12306); // If the progress bar cannot be changed // Section 36 black Technology
H5 advanced
1.geolocation
<script> // Get geographic information // Some systems do not support this function // GPS positioning. Almost all desktops have no GPS, most laptops have no GPS, and almost all smartphones have GPS // Network location to roughly estimate geographical location window.navigator.geolocation.getCurrentPosition(function(position){ console.log("======");//Successful callback function console.log(position); },function(){//Failed callback function console.log("++++++"); }); //Access methods: https protocol, file protocol and http protocol cannot be obtained // Longitude Max 180, latitude max 90 </script>
https is still inaccessible:
- Google browser opens Google maps, unable to locate
- It can be realized by climbing over the wall
2. Write a server in four lines
Mobile phone access computer
sever.js
npm init
npm i express
var express = require('express'); var app = new express(); app.use(express.static("./page")); app.listen(12306);//The port number is greater than 8000 or equal to 80 // Port 80 is accessed by default, and index. Is accessed by express by default html Want to visit the inside hello.html 127.0.0.1:12306 127.0.0.1:12306/hello.html
test.7z
Command box or vscode client, enter the project path, node server js
3.deviceorientation
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <title>document</title> <link rel="stylesheet" href=""> </head> <body> <div id="main"></div> <script> // Gyroscope, only the equipment supporting gyroscope can support body feeling // The pages of Apple devices can only use these interfaces under the https protocol // 11.1.X and before, can be used. Wechat browser // alpha: point north (Compass) [0360) point north when it is 0.180 Guide // Beta: the beta value is 0 when it is laid flat. When the mobile phone stands up (the short side contacts the desktop), the beta is 90; // Gamma: the gamma value is zero when placed flat. When the mobile phone stands up (the long side touches the desktop), the gamma value is 90 window.addEventListener("deviceorientation",function(event){ // console.log(event); document.getElementById("main").innerHTML = "alpha:" + event.alpha + "<br/>" + "beta:" + event.beta + "<br/>" + "gamma:" + event.gamma }); </script> </body> </html>
4. Mobile phone access to computer
1. The mobile phone and computer are under the same LAN
2. Get the IP address of the computer
windows get ip: terminal input ipconfig
3. Enter the corresponding IP and port on the mobile phone for access
5.devicemotion
<script> // Shake it window.addEventListener("devicemotion",function(event){ document.getElementById("main").innerHTML = event.accelertion.x + "<br/>" + event.accelertion.y + "<br/>" + event.accelertion.z; if(Math.abs(event.accelertion.x) > 9 || Math.abs(event.accelertion.y) > 9|| Math.abs(event.accelertion.z) > 9){ alert("Shaking"); } }); </script>
6.requestAnimationFrame
/* function move(){ var square = document.getElementById("main"); if(square.offsetLeft > 700){ return; } square.style.left = square.offsetLeft + 20 +"px"; } setInterval(move, 10); */ // Screen refresh rate: 60 times per second // If it changes more than 60 times a second, the animation needle will be lost // To achieve uniform movement, use requestAnimationFrame, which is 60 stitches per second // Interval 60 / 1000; Will it achieve the same effect // If 1 pin is less than 1 / 60 of a second, the requestAnimationFrame can execute each frame on time // requestAnimationFrame(move);// Move once var timer = null; function move(){ var square = document.getElementById("main"); if(square.offsetLeft > 700){ cancelAnimationFrame(timer); return; } square.style.left = square.offsetLeft + 20 +"px"; timer = requestAnimationFrame(move); } move(); // cancelAnimationFrame is basically equivalent to clearTimeout // requestAnimationFrame has poor compatibility
Compatibility is very poor. What if you want to use it?
window.cancelAnimationFrame = (function(){ return window.cancelAnimationFrame || window.webkitCancelAnimationFrame || window.mozCancelAnimationFrame || function(id){ window.clearTimeOut(id); } })(); window.requestAnimationFrame = (function(){ return window.requestAnimationFrame || window.webkitRequestAnimationFrame || window.mozRequestAnimationFrame || function(id){ window.setTimeOut(id, 1000 / 60); } })();
7.localStrorage
cookie: every request may send a lot of useless information to the back end
localStroage: stored in the browser for a long time, regardless of whether the window is closed or not
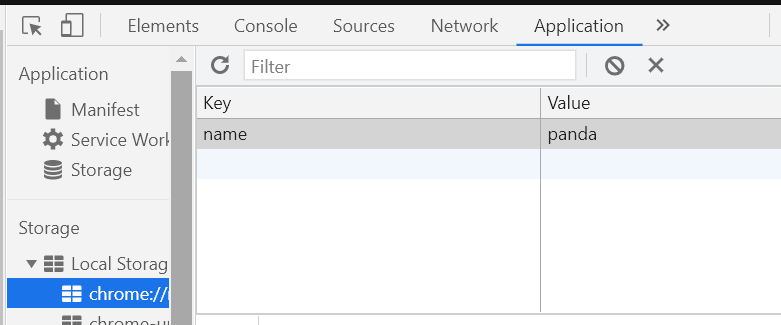
localStorage.name = "panda"; // localStroage.arr = [1, 2, 3]; // console.log(localStroage.arr); // localStroage can only store strings, To store an array localStorage.arr = JSON.stringify([1, 2, 3]); // console.log(localStorage.arr); console.log(JSON.parse(localStorage.arr)); localStorage.obj = JSON.stringify({ name: "panda", age: 18 }); console.log(JSON.parse(localStorage.obj));
sessionStroage: the variable that needs to be stored temporarily for this reply. Every time the window closes, the secionstroage is automatically cleared
sessionStorage.name = "panda";
localStorage and cookie s
1.localStorage will not send the data when sending the request. Cookies will bring all the data out
2. The contents stored in cookies are relatively (4k), and localStroage can store more contents (5M)
Another way of writing
localStorage.setItem("name", "monkey"); localStorage.getItem("name"); localStorage.removeItem("name");
The same protocol, the same domain name and the same port are called a domain
be careful:
www.baidu.com is not a domain.
http://www.baidu.com https://www.baidu.com , is a domain. This is a different domain
8.history
<!doctype html> <html lang="en"> <head> <meta charset="UTF-8"> <title>document</title> <style type="text/css"> </style> <script> // A -> B - C // For web page performance, single page operation var data = [{ name: "HTML" }, { name: "CSS" }, { name: "JS" }, { name: "panda" }, { name: "dengge" }]; function search() { var value = document.getElementById("search").value; var result = data.filter(function (obj) { if (obj.name.indexOf(value) > -1) { return obj; } }); render(result); history.pushState({ inpVal: value }, null, "#" + value); } function render(renderData) { var content = ""; for (var i = 0; i < renderData.length; i++) { content += "<div>" + renderData[i].name + "</div>"; } document.getElementById("main").innerHTML = content; } window.addEventListener("popstate", function (e) { // console.log(e); document.getElementById("search").value = e.state.inpVal ? e.state.inpVal : ""; var value = document.getElementById("search").value; var result = data.filter(function (obj) { if (obj.name.indexOf(value) > -1) { return obj; } }); render(result); }); // About pop state and hashchange // As soon as the url changes, the pop state will be triggered // If the anchor point changes (the hash value changes), hashchange will be triggered window.addEventListener("hashchange", function (e) { console.log(e); }) </script> </head> <body> <input type="text" id="search"><button onclick="search()">search</button> <div id="main"></div> <script> render(data); </script> </body> </html>
9.worker
<script> // js are single threaded // worker is multithreaded, real multithreading, not pseudo multithreading // worker cannot operate DOM, has no window object, and cannot read local files. Can send ajax, can calculate // Can I create a worker in worker? // In theory, it can, but there is no browser support /* console.log("======="); console.log("======="); var a = 1000; var result = 0; for (var i = 0; i < a; i++) { result += i; } console.log(result); console.log("======="); console.log("======="); */ // The last two equal signs are executed only after the calculation is completed var beginTime = Data.now(); console.log("======="); console.log("======="); var a = 1000; var worker = new Worker("./worker.js"); worker.postMessage({ num: a }); worker.onmessage = function (e) { console.log(e.data); } console.log("======="); console.log("======="); var endTime = Data.now(); console.log(endTime - beginTime); worker.terminate(); //stop it this.close();//Stop yourself </script>
worker.js
this.onmessage = function(e){//Accept message // console.log(e); var result = 0; for (var i = 0; i < e.data.num; i++) { result += i; } this.postMessage(result); }
worker.js, you can import external js files through importScripts("./index.js")