NSFontAttributeName (Font)
The value corresponding to this property is a UIFont object.This property is used to change the font of a piece of text.If this property is not specified, it defaults to 12-point Helvetica(Neue).
NSParagraph StyleAttributeName (Paragraph)
The value corresponding to this property is a NSParagraphStyle object.This attribute applies multiple attributes to a single piece of text.If this property is not specified, it defaults to the default paragraph property returned by the defaultParagraphStyle method of NSParagraphStyle.
NSMutableParagraphStyle *paragraph = [[NSMutableParagraphStyle alloc] init]; paragraph.alignment = NSTextAlignmentCenter;
NSForegroundColorAttributeName (font color)
The value corresponding to this property is a UIColor object.This property is used to specify the font color of a piece of text.If this property is not specified, it defaults to black.
// NSForegroundColorAttributeName NSDictionary *attrDict1 = @{ NSForegroundColorAttributeName: [UIColor redColor] }; NSDictionary *attrDict2 = @{ NSForegroundColorAttributeName: [UIColor blueColor] }; NSDictionary *attrDict3 = @{ NSForegroundColorAttributeName: [UIColor orangeColor] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3];
Be careful:
The color set by NSForegroundColorAttributeName is positionally equal to the color set by the UILabel's textColor attribute and to the NSBackgroundColorAttributeName's position. Whoever assigns the value last shows who the color is, but the textColor attribute can be overlaid with the NSBackgroundColorAttributeName attribute.
NSBackgroundColorAttributeName (Font Background Color)
The value corresponding to this property is a UIColor object.This property is used to specify the background color of a piece of text.If this property is not specified, no background color is defaulted.
NSLigatureAttributeName (hyphen)
The value corresponding to this property is a NSNumber object (integer).Hyphenated characters refer to certain hyphenated characters that use a single meta-symbol.0 means there are no hyphens.1 indicates that the default hyphenation character is used.2 means to use all the conjoined symbols.The default value is 1 (note that iOS does not support a value of 2).
NSString *ligatureStr = @"flush"; NSDictionary *attrDict1 = @{ NSLigatureAttributeName: [NSNumber numberWithInt: 0], NSFontAttributeName: [UIFont fontWithName: @"futura" size: 30] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: ligatureStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSLigatureAttributeName: @(1), NSFontAttributeName: [UIFont fontWithName: @"futura" size: 30] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: ligatureStr attributes: attrDict2];
To display hyphens, replace the previous string with Chinese characters with flush
NSLigatureAttributeName takes the value of a NSNumber object, so it cannot be assigned an integer value directly. There are many ways to create a NSNumber object, or it can be abbreviated as @(int)
Note the changes between the letters f and l.
Feeling like hyphenation is an art word function that really looks better when the characters f and L are drawn with a combination of symbols (so-called glyphs).But not all characters have combination symbols. In fact, only certain combinations of characters (such as characters f and l, f and i) in some fonts can have beautiful combination symbols.
NSKernAttributeName
NSKernAttributeName sets the character spacing to NSNumber objects (integers), widens the positive value spacing, and narrows the negative value spacing
NSDictionary *attrDict1 = @{ NSKernAttributeName: @(-3), NSFontAttributeName: [UIFont systemFontOfSize: 20] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSKernAttributeName: @(0), NSFontAttributeName: [UIFont systemFontOfSize: 20] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; NSDictionary *attrDict3 = @{ NSKernAttributeName: @(10), NSFontAttributeName: [UIFont systemFontOfSize: 20] }; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3];
NSStrikethrough StyleAttributeName (Strikeout)
NSStrikethroughStyleAttributeName sets the strikethrough, takes the value of the NSNumber object (integer), and enumerates the values in the constant NSUnderlineStyle:
- NSUnderlineStyleNone does not set strikethrough
- NSUnderlineStyleSingle sets the strikethrough to a thin solid line
- NSUnderlineStyleThick sets the strikethrough to a thick single solid line
- NSUnderlineStyleDouble sets the strikethrough to thin double solid
The default value is NSUnderlineStyleNone.


NSDictionary *attrDict1 = @{ NSStrikethroughStyleAttributeName: @(NSUnderlineStyleSingle), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSStrikethroughStyleAttributeName: @(NSUnderlineStyleThick), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; NSDictionary *attrDict3 = @{ NSStrikethroughStyleAttributeName: @(NSUnderlineStyleDouble), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3];
Be careful:
-
Although enumeration constants are used, they are integers in nature and must also be converted to NSNumber before they can be used
-
Strikeout and underscore use the same enumeration constant as their attribute values
-
Currently there are only 4 effects listed above in iOS. Although we can find more values in the header file, they have no effect after use.
You can see that the positions of the strikethroughs are different between Chinese and English
In addition, in addition to the above four values, in fact, you can also take other integer values. Interested people can experiment on their own. When the value is 0 - 7, the effect is a single solid line. As the value increases, the single solid line becomes thicker gradually. When the value is 9 - 15, the effect is a double solid line. The larger the value, the thicker the double solid line.
NSDictionary *attrDict1 = @{ NSStrikethroughStyleAttributeName: @(1), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSStrikethroughStyleAttributeName: @(3), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; NSDictionary *attrDict3 = @{ NSStrikethroughStyleAttributeName: @(7), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3];
NSStrikethroughColorAttributeName
NSStrikethroughColorAttributeName sets the strikethrough color, takes the UIColor object, and defaults to black
NSDictionary *attrDict1 = @{ NSStrikethroughColorAttributeName: [UIColor blueColor], NSStrikethroughStyleAttributeName: @(1), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSStrikethroughColorAttributeName: [UIColor orangeColor], NSStrikethroughStyleAttributeName: @(3), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; NSDictionary *attrDict3 = @{ NSStrikethroughColorAttributeName: [UIColor greenColor], NSStrikethroughStyleAttributeName: @(7), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3];
NSUnderlineStyleAttributeName
The value corresponding to this property is a NSNumber object (integer).The value specifies whether to underline the text, which refers to Underline Style Attributes.The default value is NSUnderlineStyleNone.
Underlines can refer entially to the strikethrough settings except for their position and strikethrough.


NSUnderlineColorAttributeName
NSUnderlineColorAttributeName sets the underline color, takes the UIColor object, and defaults to black
NSDictionary *attrDict1 = @{ NSUnderlineColorAttributeName: [UIColor blueColor], NSUnderlineStyleAttributeName: @(NSUnderlineStyleSingle), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSUnderlineColorAttributeName: [UIColor orangeColor], NSUnderlineStyleAttributeName: @(NSUnderlineStyleThick), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; NSDictionary *attrDict3 = @{ NSUnderlineColorAttributeName: [UIColor greenColor], NSUnderlineStyleAttributeName: @(NSUnderlineStyleDouble), NSFontAttributeName: [UIFont systemFontOfSize:20] }; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3];
NSStrokeColorAttributeName (edge color) and NSStrokeWidthAttributeName (edge width)
To set the text stroke color, you need to set the stroke width with NSStrokeWidthAttributeName to make the text hollow.
The value corresponding to the property NSStrokeWidthAttributeName is a NSNumber object (decimal).This value changes the stroke width (as a percentage of font size), negative fill effect, and positive hollow effect, which defaults to 0, i.e. does not change.Positive numbers only change the stroke width.Negative numbers change both the description edge and the fill width of the text.For example, for common hollow words, this value is usually 3.0.
When two hollow attributes are set and the NSStrokeWidthAttributeName attribute is set to an integer, the text foreground color is invalid
NSStrokeColorAttributeName fills part of the color, not the font color, with a value of UIColor object




NSDictionary *attrDict1 = @{ NSStrokeWidthAttributeName: @(-3), NSFontAttributeName: [UIFont systemFontOfSize:30] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSStrokeWidthAttributeName: @(0), NSFontAttributeName: [UIFont systemFontOfSize:30] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; NSDictionary *attrDict3 = @{ NSStrokeWidthAttributeName: @(3), NSFontAttributeName: [UIFont systemFontOfSize:30] }; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3]; NSDictionary *attrDict1 = @{ NSStrokeWidthAttributeName: @(-3), NSStrokeColorAttributeName: [UIColor orangeColor], NSFontAttributeName: [UIFont systemFontOfSize:30] }; _label01.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict1]; NSDictionary *attrDict2 = @{ NSStrokeWidthAttributeName: @(0), NSStrokeColorAttributeName: [UIColor blueColor], NSFontAttributeName: [UIFont systemFontOfSize:30] }; _label02.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict2]; NSDictionary *attrDict3 = @{ NSStrokeWidthAttributeName: @(3), NSStrokeColorAttributeName: [UIColor greenColor], NSFontAttributeName: [UIFont systemFontOfSize:30] }; _label03.attributedText = [[NSAttributedString alloc] initWithString: originStr attributes: attrDict3];
NSShadowAttributeName (Shadow)
The value corresponding to this property is a NSShadow object.The default is nil.Setting it alone is not good, and either of these three is good, NSVerticalGlyphFormAttributeName, NSObliquenessAttributeName, NSExpansionAttributeName
NSVerticalGlyphFormAttributeName (horizontal and vertical typesetting)
The value corresponding to this property is a NSNumber object (integer).0 means horizontal text.1 stands for vertical text.In iOS, horizontal text is always used, and values other than 0 are undefined.
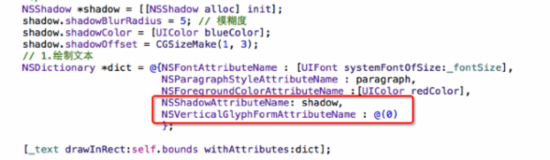

NSObliquenessAttributeName
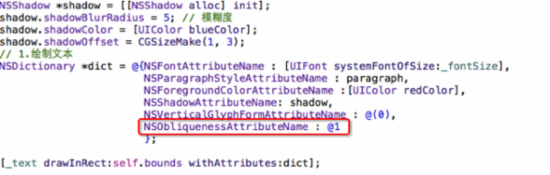

NSExpansionAttributeName (Flattened Text)
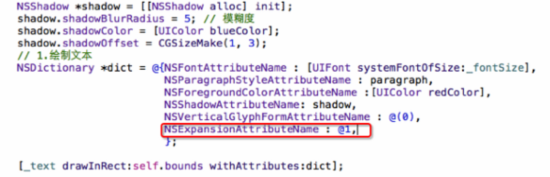

Author: Ammar
Link: http://www.jianshu.com/p/8f49c9c99b21
Source: Short Book
Copyright belongs to the author.For commercial reprinting, please contact the author for authorization. For non-commercial reprinting, please indicate the source.