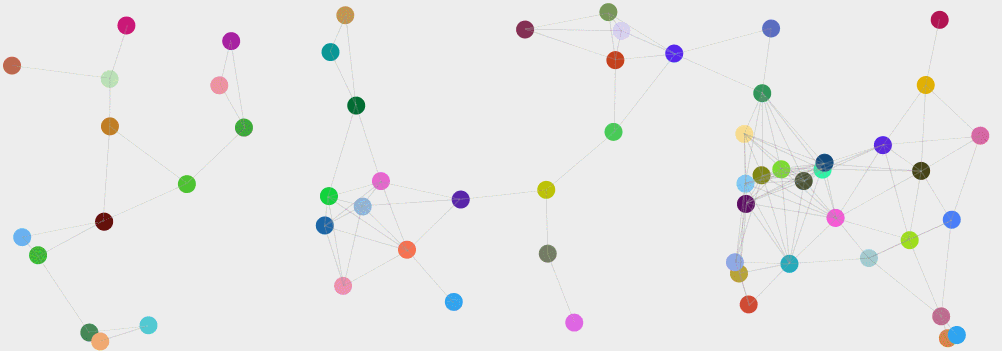
There are many moving balls in the background of the login interface of the knowledgeable web page, and there is a connection between the balls and the balls when they move. It gives us a three-dimensional transformation effect. It looks very good. So we try to make a similar effect with the background of the knowledgeable login interface with canvas. The above motion picture is a screenshot of the effect.
Ideas for Realization
First understand the animation principle in canvas? In fact, the animation in canvas is achieved through continuous redrawing. For example, when a small ball is initially recorded as a ball(x,y) in the X and Y coordinates of the canvas, the X and Y coordinates of the ball (x + 5, y + 5) are changed every 10 milliseconds, and the whole canvas is cleared and redrawn on the canvas. After adjusting the coordinates of the ball, because 10 milliseconds is very short, all the visual sense to us is that the ball is constantly moving. This is the basic principle of canvas drawing.
-
Define the sphere object
var ball = { xPointer: 100, //Initial x-coordinates of a sphere yPointer: 100, //Initial y coordinates of spheres vx: 1, //Velocity in the x direction vy: 0.1, //Velocity in the y direction x: 1, //The direction of motion of the x-axis (1 for positive direction and - 1 for negative direction) y: -1, //y-axis direction of motion color: "blue", //Ball colour radius: 10, //Spherical radius };
-
Generation of spheres
There are many balls in demo, so define an array to hold these balls. The starting coordinates, colors and moving directions of the balls are different, so these values need to be obtained randomly.var ballList = []; //Ball array var canvas, ctx; //Generate multiple balls function initBall() { canvas = document.getElementById("canvas"); ctx = canvas.getContext("2d"); //Cyclic generation of 60 balls for (var i = 0; i < 60; i++) { // console.log(getIndex() + " " + getIndex()) var ball = {}; ball.xPointer = getRandom(20, 980); //X-coordinates of random spheres ball.yPointer = getRandom(20, 340); //y coordinates of random spheres ball.x = getIndex(); //x-axis direction of motion of a random sphere ball.y = getIndex(); //y-axis direction of motion of random spheres ball.vx = Math.random(); //x-Axis Directional Velocity of Random Balls ball.vy = Math.random(); //y-Axis Directional Velocity of Random Balls ball.radius = 9; //Spherical radius ball.color = "#"+("00000"+((Math.random ()* 16777215 + 0.5) > 0). toString (16). slice (-6);//random ball color ballList.push(ball); } } //Random 1 or - 1 method function getIndex() { var arr = [0, 1]; var index = Math.floor((Math.random() * arr.length)); if (index == 0) { index = -1; } return index; } //A Method of Obtaining a Random Number Between Two Numbers function getRandom(first, last) { var choice = last - first + 1; return Math.floor(Math.random() * choice + first); }
-
Drawing balls through canvas
Page canvas tag<canvas id="canvas" width="1000" height="360" style='background-color: #EEEEEE;'></canvas>
canvas Draws Ball Code
function draw(ctx) { ctx.clearRect(0, 0, 1000, 360); //Clear the canvas before drawing for (var i = 0; i < ballList.length; i++) { ctx.save(); ctx.beginPath(); ctx.fillStyle = ballList[i].color; ctx.arc(ballList[i].xPointer, ballList[i].yPointer, ballList[i].radius, 0, Math.PI * 2, false); ctx.closePath(); ctx.fill(); ctx.restore(); } }
-
Exercise
Simple collision detection is used in the movement of the ball, which changes the direction of the ball every time it reaches the edge of the canvas.//Method of modifying the state of the ball to make it move function update(ballList, ctx) { for (var i = 0; i < ballList.length; i++) { ballList[i].xPointer += ballList[i].vx * ballList[i].x; ballList[i].yPointer += ballList[i].vy * ballList[i].y; //X-axis direction of collision detection if (ballList[i].xPointer + ballList[i].radius >= canvas.width || ballList[i].xPointer - ballList[i].radius <= 0) { ballList[i].x = ballList[i].x * -1; } //Y-axis direction of collision detection if (ballList[i].yPointer + ballList[i].radius >= canvas.height || ballList[i].yPointer - ballList[i].radius <= 0) { ballList[i].y = ballList[i].y * -1; } } }
-
Draw the line between the ball and the ball
//Connection between balls function drawLine(ballList, ctx) { for (var i = 0; i < ballList.length; i++) { for (var j = 0; j < ballList.length; j++) { var xx = Math.pow((ballList[i].xPointer - ballList[j].xPointer), 2); var yy = Math.pow((ballList[i].yPointer - ballList[j].yPointer), 2); var zz = Math.sqrt(xx + yy); //Judge that if the distance between two balls is between 20 and 100, draw a straight line. if (zz <= 100 && zz >= 20) { console.log(zz) ctx.save(); ctx.beginPath(); ctx.strokeStyle = "#999999"; ctx.lineWidth = 0.1; // ctx.strokeStyle= "#" + ("00000" + ((Math.random() * 16777215 + 0.5) >> 0).toString(16)).slice(-6); ctx.moveTo(ballList[i].xPointer, ballList[i].yPointer); ctx.lineTo(ballList[j].xPointer, ballList[j].yPointer); ctx.closePath(); ctx.stroke(); ctx.restore(); } } } }
-
Function
(function() { initBall(); //Generation of spheres //timer setInterval(function() { // console.log(selectfrom(0, 600) + " " + selectfrom(0, 600)); draw(ctx); //Draw update(ballList, ctx); //Modifying the Ball State drawLine(ballList, ctx); //Draw line }, 24) })();
Other
-
Because of the simplicity of the code, there is no encapsulation.
-
By modifying the radius of the sphere, another good display effect is obtained, as shown below.
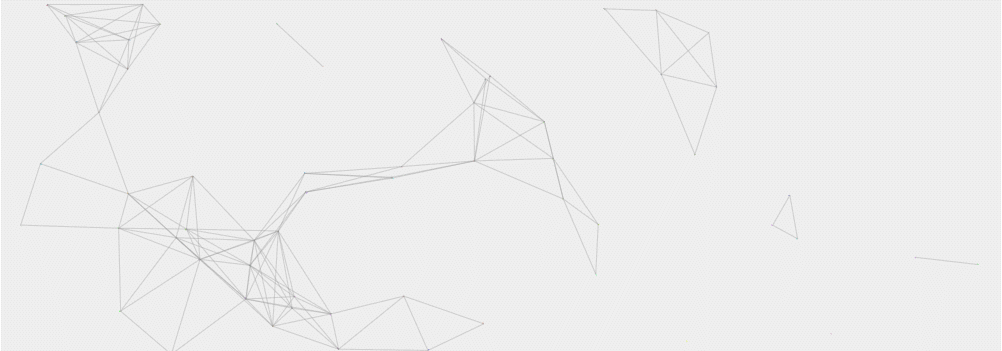
At this point, the demo of the canvas ball movement effect is finished, to see if there is a 3d transformation effect.
If the article is wrong, please don't hesitate to give advice.~