Hello everyone, meet again. I'm Jun Quan. I wish every programmer can learn more languages.
java study Road of
0x00 Preface
I've been learning java for some time. Write an article to record the learning content.
0x01 java loading mechanism
When it comes to java, I have to mention the class loading mechanism of java. java is a development language that relies on the jvm (that is, the java virtual machine) to realize cross platform. All java codes will run in the jvm, and java is running XX The java source file will be compiled into a class suffix file (bytecode file) to run. java. java is called when the java class is initialized Lang.classloader loads bytecode files. Let's take a look at the relationship between jdk, jre and jvm.
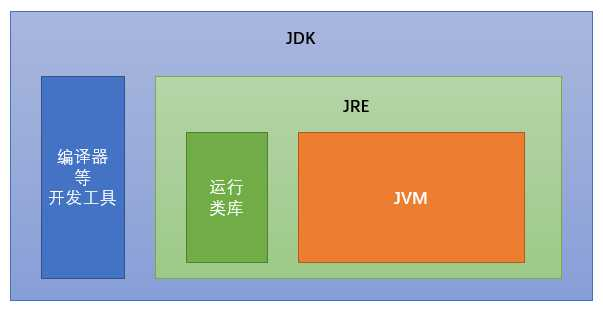
0x02 first program
Here's a direct copy of the code
public class test { public static void main(String[] args) { System.out.println("Hello wrorld!"); } }
Let's look down from the first code
public class test {}
A class is defined here. The class name here must be consistent with the file name, otherwise an error will be reported
public static void main(String[] args) {}
The second line of code represents its main function, and the entry point in the program is main.
System.out.println("Hello wrorld!");
The third line of code calls system out. Println's method prints things on the screen.
0x03 data type
Data type classification
The data types of java are divided into two categories:
Basic data types: including integer, floating point number, character and Boolean
Reference data types: including classes, arrays, and interfaces
Basic data type
data type | keyword |
---|---|
Byte type | byte |
Short | short |
integer | int |
Long integer | long |
Single precision floating point | float |
Double precision floating point | double |
character | char |
Boolean type | boolean |
The default type in java: integer is int and floating point number is double.
0x04 variable definition
The format of variable definition includes three elements: data type, variable name and data value.
Format:
Data type variable name = data value;
public class test1 { public static void main(String[] args) { int a = 1; float b= 5.5F; double c = 5.5; boolean d = true; char e ='A'; System.out.println(a); System.out.println(b); System.out.println(c); System.out.println(d); System.out.println(e); } }
long type data is represented by L.
float plus f.
Defined variables cannot be used without assignment.
0x04 type conversion
Automatic type conversion
public static void main(String[] args) { int a =1; byte b = 2; int c =a+b; System.out.println(c); }
The operation is successful, and the operation result is output 3
This can run successfully because of the automatic type conversion internally, which automatically converts the byte type of the b variable to the int type
Cast type
Conversion format:
Data type variable name = (converted data type) converted variable
int i = (int)1.5
Casting can result in loss of data precision
0x05 assignment operator
Assignment Operators
Assignment Operators | explain |
---|---|
= | assignment |
+= | Plus equals |
-= | Minus equals |
*= | Multiply equal |
/= | Division equals |
%= | Modulo equals |
Comparison operator
Comparison operator | explain |
---|---|
== | Equal ratio of the values of variables on both sides |
< | Compare whether the value on the left is less than the value on the right |
|Compare whether the value on the left is greater than the value on the right =| compare whether the value on the left is greater than or equal to the value on the right < = | compare whether the value on the left is less than or equal to the value on the right != | If the data on both sides are not equal, the result is true
For comparison operators, the result will only be false or true
Logical operator
Logical operator | explain |
---|---|
&& | If the results on both sides are true, then tue |
|| | As long as one result is true, the result is true |
! | ! True, the result is false,! The result of flash is true |
As for operators, I won't explain them much. Just record them and see them when they need to be used.
0x06 method definition
Method definition format:
Modifier return value type method name (parameter list){ code }
Return value type: void The type is no type, so if you define the return value type as void,reurn No need to fill in Method name: name the method we define, which meets the specification of identifier and is used to call the method. Parameter list: can be filled in. The parameter type must be carried in front of the incoming parameter. return: Method ended. The return value type is void,If other types are defined in the return value, return The returned result must also be the type filled in by the return value type
public static void main(String[] args) { show(); } public static void show(){ System.out.println("method"); }
After defining the method, the method will be loaded by default. We also need to call the method in the main method.
Definition method considerations
Methods must be defined outside of methods in a class
A method cannot be defined inside another method
0x07 process control statement
The essential thing in a program is process control. Process control is nothing more than judgment and loop. First, let's look at the judgment statement.
if judgment
Syntax:
if (Relational expression){ Statement body; }else{ Statement body; }
Execution process
First, it will judge whether the result of the expression is true or false. If it is true, the statement body will be executed. If it is false, the content in else will be executed.
public static void main(String[] args) { int a = 1; if (a==1){ System.out.println("a:"+a); }else{ System.out.println("a!=1"); } }
switch -- select statement
switch statement format:
switch(expression) { case Constant value 1: Statement body 1; break; case Constant value 2: Statement body 2; break; ... default: Statement body n+1; break; }
Execution process:
First, calculate the value of the expression, and then compare it with case in turn. If there is a corresponding value, execute the corresponding statement, and encounter the end of break when the execution is completed. Finally, if all case values do not match, execute the statement in default.
public static void main(String[] args) { int weekday = 6; switch(weekday) { case 1: System.out.println("Monday"); break; case 2: System.out.println("Tuesday"); break; case 3: System.out.println("Wednesday"); break; case 4: System.out.println("Thursday"); break; case 5: System.out.println("Friday"); break; case 6: System.out.println("Saturday"); break; case 7: System.out.println("Sunday"); break; default: System.out.println("The number you entered is wrong"); break; } }
Circular statement - for
A loop statement can repeatedly execute a piece of code when the loop condition is met. When a condition is reached, the loop condition must be changed to flash to end the loop, otherwise an endless loop will appear and continue to be executed.
for loop statement format:
for(Initialization expression;Boolean expression;Step expression){ Circulatory body; }
Execution process:
First initialize a variable, define an expression and step expression, and then execute the loop body. If not, continue the loop until the conditions of Boolean expression are met, and exit the loop.
public static void main(String[] args) { for (int i = 0; i < 20; i++) { System.out.println("Hello World"); } }
It has been printed 20 times, that is, it has been cycled 20 times. The cycle condition is that i is less than 20. Each time i is executed, 1 will be added. If i is added 20 times, the Boolean expression condition will be met and the cycle will be exited. Here, the step expression must be written, otherwise it will fall into an endless loop.
Loop statement 2 -- while
while loop statement format:
Initialization expression while(Boolean expression){ Circulatory body Step expression }
public static void main(String[] args) { //while loop to print HelloWorld 10 times //Define initialization variables int i = 1; //Cycle condition < = 10 while(i<=10){ System.out.println("HelloWorld"); //Stepping i++; } }
Loop statement 3 do while
Do while is very similar to while. It can be said that the basic usage is the same, but the only difference is that while will not be executed directly if the expression meets the conditions, while do while is to execute the loop body inside first, and then judge whether the expression meets the conditions.
do... while format:
Initialization expression do{ Circulatory body Step expression }while(Boolean expression);
public static void main(String[] args) { int x=1; do { System.out.println("HelloWorld"); x++; }while(x<=10); }
Characteristics of do... while loop: unconditionally execute the loop body once. Even if we directly write the loop condition as false, it will still loop once.
Here's the difference between a for loop and a while loop.
The variable controlled by the control condition statement can no longer be accessed after the for loop ends, and the while loop ends Continue to use. If you want to continue to use, use while. Otherwise, for is recommended. The reason is that when the for loop ends, the variable is erased from memory Loss, can improve the efficiency of memory use.
for is recommended when the number of cycles is known, and while is recommended when the number of cycles is unknown.
Jump out of loop statement
break
Usage scenario: Terminate the loop or select the switch structure
continue
Usage scenario: end this cycle and continue the next cycle
0x08 array
Array concept: array is a fixed container for storing data to ensure that multiple data types are consistent.
Container concept: multiple data are stored together, and each data is called the element of the container.
array define
Mode 1 format:
Data storage type [] array name = new array storage type [length];
Detailed explanation of array definition format: Data type of array storage: what data type can the created array container store. [] : Represents an array. Array name: name a variable for the defined array, which meets the identifier specification. You can use the name to operate the array. new: Keyword, the keyword used to create the array. Data type of array storage: what data type can the created array container store. [length]: The length of the array, indicating how many elements can be stored in the array container
The array has a specific length. Once the length is specified, it cannot be changed.
int[] arr = new int[3];
Mode 2 format:
Data type [] array name = new data type [] {element 1, element 2, element 3};
Mode 3 format:
Data type [] data name = {element 1, element 2, element 3};
Access array
Accessing array data will use the index to access the data in the array. Let's talk about the index.
Index: every element stored in the array will automatically have a number, starting from 0. This automatic number is called array index (index), you can access the elements in the array through the index of the array.
If you want to access the data, you can add brackets after the array variable, and then write the value to be indexed.
Array name [index]
Each array has a length and is fixed. java gives a property to the value, which can directly obtain the length of the array.
Array name length
If you want to index the maximum value of an array, you can use the array name directly length-1 gets that one is subtracted here because the index starts from zero.
As mentioned earlier, once the length of the array is defined, it cannot be changed, so there will be some problems, such as the index is out of access. If the index is out of range, a null pointer exception error will be burst.
Array traversal
Traversing the array can use the for loop to get the maximum value of the array as the loop condition, and then index the number of steps of the array.
public static void main(String[] args) { int arr[] = {1,20,13,64,45}; for (int i = 0; i < arr.length ; i++) { System.out.println(arr[i]); } }
In this way, you can traverse the values of all arrays one by one.
Array passing
Arrays can also be passed as parameters
public static void main(String[] args) { int[] arr = { 1, 3, 5, 7, 9 }; //Call method to pass array printArray(arr); } / * Create a method that receives parameters of array type Traverse the array */ public static void printArray(int[] arr) { for (int i = 0; i < arr.length; i++) { System.out.println(arr[i]); } }
When the parameter of the method is a basic type, the data value is passed When the parameter of the method is a reference type, the address value is passed.
0x09 end
The basic grammar article has been updated, and subsequent articles are continuously updated...
Publisher: full stack programmer, stack length, please indicate the source for Reprint: https://javaforall.cn/119959.html Original link: https://javaforall.cn