preface | ❤️ As long as the next day can be expected, today is worth rejoicing ❤️ |
---|
1, Variable
(1) Variable overview
1.1 what are variables
Vernacular: a variable is a box of things.
Popular: variables are containers for storing data. We get the data through the variable name, and even the data can be modified.
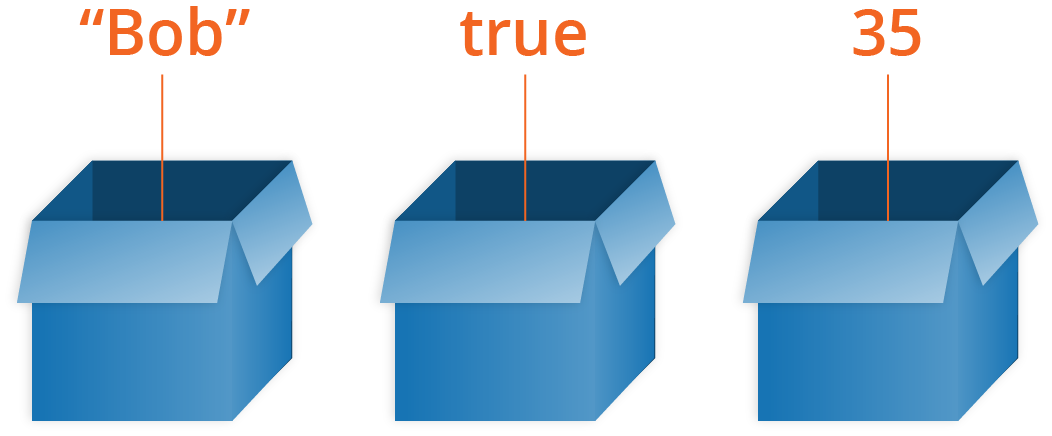
1.2 storage of variables in memory
Essence: a variable is a piece of space applied by a program in memory for storing data.
Similar to our hotel room, a room can be regarded as a variable.
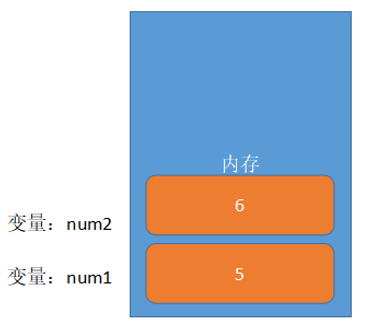
(2) Use of variables
Variables can be used in two steps: 1 Declare variable 2 assignment
2.1 declared variables
The code is shown as follows:
// Declare variable var age; // Declare a variable named age
- var is a JS keyword used to declare variables (the meaning of variable variable). After the variable is declared with this keyword, the computer will automatically allocate memory space for the variable, which does not need to be managed by the programmer;
- age is the variable name defined by the programmer. We need to access the allocated space in memory through the variable name;
2.2 assignment
The code is shown as follows:
age = 10; // Assign a value of 10 to this variable
- =It is used to assign the value on the right to the variable space on the left, which represents the meaning of assignment;
- Variable value is the value stored in the variable space by the programmer;
2.3 initialization of variables
The code is shown as follows:
var age = 18; // The declared variable is assigned 18 at the same time
- Declaring and assigning a variable is called variable initialization.
(3) Variable syntax extension
3.1 update variables
After a variable is re assigned, its original value will be overwritten, and the value of the variable will be subject to the last assigned value.
The code is shown as follows:
var age = 18; age = 81; // The end result is 81 because 18 is covered
3.2 declare multiple variables at the same time
When declaring multiple variables at the same time, you only need to write a var, and the names of multiple variables are separated by English commas.
The code is shown as follows:
var age = 23, name = 'battledao', sex = 'male';
3.3 special cases of declared variables
situation | explain | result |
---|---|---|
var age; console.log(age); | Only declaration without assignment | undefined |
console.log(age); | No declaration, no assignment, direct use | report errors |
age = 10; console.log(age); | No declaration, only assignment | 10 |
(4) Variable naming convention
- Consists of letters (A-Za-z), numbers (0-9), underscores () Dollar sign ($), for example: usrage, num01_ name
- Strictly case sensitive. For example: var App; And var App; Are two variables
- Cannot start with a number. For example: 18age is wrong
- Cannot be keyword or reserved word. For example: var, for, while
- Variable names must be meaningful. For example, MMD and BBD are wrong
- Follow the hump nomenclature. The first letter is lowercase, and the first letter of the following word needs to be capitalized. For example: myFirstName
😆 Case requirements 😆: Exchange the values of two variables (implementation idea: use a temporary variable as intermediate storage)
The code is shown as follows:
<script> // js is a programming language with strong logic: the idea of realizing this requirement is how to do it first and then how to do it // 1. We need a temporary variable to help us // 2. Give us the temporary variable temp from apple1 // 3. Give the apple in apple2 to apple1 // 4. Give the value in the temporary variable to apple2 var temp; // A temporary variable was declared null var apple1 = 'green apple'; var apple2 = 'Red apple'; temp = apple1; // Give the right to the left apple1 = apple2; apple2 = temp; console.log(apple1); console.log(apple2); </script>
2, Data type
(1) Introduction to data types
1.1 why do you need data types
one ️⃣ In the computer, the storage space occupied by different data is different. In order to divide the data into data with different memory sizes and make full use of the storage space, different data types are defined.
two ️⃣ Simply put, data type is the category and model of data. For example, the name "Zhang San" and age are 18. The types of these data are different.
1.2 data types of variables
Variables are where values are stored. They have names and data types. The data type of the variable determines how the bits representing these values are stored in the computer's memory.
JavaScript is a weakly typed or dynamic language. This means that there is no need to declare the type of variable in advance, and the type will be determined automatically during the operation of the program.
The code is shown as follows:
var age = 10; // This is a numeric type var areYouOk = 'yes'; // This is a string
When the code is running, the data type of the variable is determined by the JS engine according to the data type of the variable value on the right. After running, the variable determines the data type.
JavaScript has dynamic types, which also means that the same variables can be used as different types:
The code is shown as follows:
var x = 6; // x is a number var x = "Bill"; // x is a string
1.3 classification of data types
JS divides data types into two categories:
- Simple data type (Number,String,Boolean,Undefined,Null)
- Complex data type (object)
(2) Simple data type
2.1 simple data type (basic data type)
Simple data types in JavaScript and their descriptions are as follows:
Simple data type | explain | Default value |
---|---|---|
Number | Numeric type, including integer value and floating-point value, such as 30 and 0.35 | 0 |
Boolean | Boolean value | false |
String | String type, such as "library". Note that in js, strings are quoted | " " |
Undefined | var a; Variable a is declared, but no value is given. At this time, a = undefined | undefined |
Null | var a = null; Declared that variable a is null | null |
2.2 digital Number
The JavaScript number type can be used to hold both integer values and decimals (floating point numbers).
The code is shown as follows:
var age = 21; // integer var Age = 21.3747; // decimal
2.2.1 digital hexadecimal
Binary and hexadecimal are the most common.
The code is shown as follows:
// 1. Octal digit sequence range: 0 ~ 7 var num1 = 07; // Corresponding to decimal 7 var num2 = 012; // 10 corresponding to decimal var num3 = 017; // 15 corresponding to decimal // 2. Hexadecimal digit sequence range: 0 ~ 9 and A~F var num = 0xA; // 10 corresponding to decimal
At this stage, we only need to remember: add 0 before octal and 0x before hexadecimal in JS;
2.2.2 digital range
Maximum and minimum values of values in JavaScript:
// 1. Maximum value of digital type console.log(Number.MAX_VALUE); // 1.7976931348623157e+308 // 2. Minimum value of digital type console.log(Number.MIN_VALUE); // 5e-324
- Maximum value: number MAX_ Value: 1.7976931348623157e+308
- Minimum value: number MIN_ Value: 5e-324
2.2.3 three special values of digital type
// 1. Infinity console.log(Number.MAX_VALUE * 2); // Infinity infinity // 2. Infinitesimal console.log(-Number.MAX_VALUE * 2); // -Infinity infinity // 3. Non numeric console.log('Curry' - 100); // NaN
- Infinity, representing infinity, greater than any value;
- -Infinity, representing infinitesimal, less than any value;
- NaN, Not a number, represents a non numeric value;
2.2.4 isNaN()
It is used to judge whether a variable is of non numeric type and return true or false;

The code is shown as follows:
var userAge = 21; var isOk = isNaN(userAge); console.log(isOk); // false, 21 is not a non number var userName = "andy"; console.log(isNaN(userName)); // true, "andy" is a non number
2.3 String type String
String type can be any text in quotation marks, and its syntax is double quotation mark "" and single quotation mark '
The code is shown as follows:
var strMsg = "My idol is curry"; // Use double quotation marks to represent strings var strMsg2 = 'My idol is curry'; // Use single quotation marks to represent strings // Common errors var strMsg3 = My idol is curry; // If an error is reported and quotation marks are not used, it will be considered as js code, but js does not have these syntax
Because the attributes in HTML tags use double quotation marks, we prefer to use single quotation marks in JS.
2.3.1 string quotation mark nesting
JS can nest double quotation marks with single quotation marks, or nest single quotation marks with double quotation marks (outer double inner single, outer single inner double)
The code is shown as follows:
var strMsg = 'I am"Gao Shuaifu"Cheng xuape'; // You can include '' with '' var strMsg2 = "I am'Gao Shuaifu'Cheng xuape"; // You can also include '' with '' // Common errors var badQuotes = 'What on earth?"; // Error reporting, cannot match single and double quotation marks
2.3.2 string escape character
Similar to the special characters in HTML, there are also special characters in the string, which we call escape characters.
Escape characters start with \. Common escape characters and their descriptions are as follows:
Escape character | interpretative statement |
---|---|
\n | Newline, n means newline |
\ \ | Slash\ |
\ ' | ’Single quotation mark |
\ " | ”Double quotation mark |
\ t | tab indent |
\ b | b means blank |
2.3.3 string length
A string is composed of several characters. The number of these characters is the length of the string. The length of the whole string can be obtained through the length attribute of the string.
// Detects the length of the obtained string var str = 'my name is battledao'; console.log(str.length); // 20
2.3.4 string splicing
one ️⃣ Multiple strings can be spliced with + in the form of string + any type = new string after splicing;
two ️⃣ Before splicing, any type added with the string will be converted into a string, and then spliced into a new string;
The code is shown as follows:
//1.1 string "addition" alert('hello' + ' ' + 'world'); // hello world //1.2 numeric string "addition" alert('100' + '100'); // 100100 //1.3 numeric string + numeric value alert('11' + 12); // 1112
😆+ No. summary formula 😆: Numerical values are added and characters are connected;
2.3.5 string splicing reinforcement
console.log('My age is' + 18); // As long as there are characters, they will be connected var age = 18; // console.log('My age is age ')// That won't work console.log('My age is' + age); // My age is 18 console.log('My age is' + age + 'Years old'); // My age is 18
- We often splice strings and variables, because variables can easily modify their values;
- Variables cannot be quoted, because quoted variables will become strings;
- If there is string splicing on both sides of the variable, the formula "quote plus", delete the number, and write the variable in the middle;
2.4 Boolean
Boolean type has two values: true and false, where true represents true (right) and false represents false (wrong);
When Boolean and numeric types are added, the value of true is 1 and the value of false is 0;
The code is shown as follows:
console.log(true + 1); // 2 console.log(false + 1); // 1
2.5 Undefined and Null
one ️⃣ A variable that has not been assigned a value after declaration will have a default value of undefined (pay attention to the result when connecting or adding)
The code is shown as follows:
var variable; console.log(variable); // undefined console.log('Hello' + variable); // Hello, undefined console.log(11 + variable); // NaN console.log(true + variable); // NaN
two ️⃣ A declared variable is given a null value, and the value stored in it is null (I will continue to explain null when explaining the object)
The code is shown as follows:
var vari = null; console.log('Hello' + vari); // Hello, null console.log(11 + vari); // 11 console.log(true + vari); // 1
(3) Get variable data type
3.1 get the data type of the detection variable
typeof can be used to obtain the data type of the detected variable;
var num = 18; console.log(typeof num) // Result number
type | example | result |
---|---|---|
String | typeof "curry" | "string" |
Number | typeof 18 | "number" |
Boolean | typeof | "boolean" |
Undefined | typeof undefined | "undefined" |
Null | typeof null | "object" |
3.2 literal quantity
Literal quantity is the representation of a fixed value in the source code. Generally speaking, it means how to express this value.
- Number literal: 8, 9, 10
- String literal: 'Stephen curry', 'a silent struggle program ape'
- Boolean literal: true, false
(4) Data type conversion
4.1 what is data type conversion
The data obtained by using the form and prompt is of string type by default. At this time, you can't simply add directly, but need to convert the data type of the variable. Generally speaking, it is to convert a variable of one data type into another data type.
We usually realize the conversion in three ways:
- Convert to string type
- Convert to digital
- Convert to Boolean
4.2 convert to string
mode | explain | case |
---|---|---|
toString() | Convert to string | var num = 10; var str = num.toString(); |
String() cast | Convert to string | var num = 10; console.log(String(num)); |
Plus concatenated string | And string splicing results are strings | var num = 10; console.log(num + ' '); |
- toString() and String() are used differently;
- Among the three conversion methods, we prefer to use the third plus sign splicing string conversion method, which is also called implicit conversion;
4.3 conversion to digital type (key)
mode | explain | case |
---|---|---|
parseInt(string) function | Convert string type to integer numeric type | parseInt('78') |
parseFloat(string) function | Convert string type to floating-point numeric type | parseFloat('78.21') |
Number() cast function | Convert string type to numeric type | Number('30') |
js implicit conversion (- * /) | Implicit conversion to numerical type by arithmetic operation | '30' - 0 |
- Pay attention to the case of parseInt and parseFloat words, which are the key points;
- Implicit conversion is that JS automatically converts the data type when we perform arithmetic operations;
4.4 convert to Boolean
mode | explain | case |
---|---|---|
Boolean() function | Convert other types to Booleans | Boolean('true'); |
- Values representing null and negative will be converted to false, such as' ', 0, NaN, null, undefined;
- Other values will be converted to true;
The code is shown as follows:
console.log(Boolean('')); // false console.log(Boolean(0)); // false console.log(Boolean(NaN)); // false console.log(Boolean(null)); // false console.log(Boolean(undefined)); // false console.log(Boolean('Xiaobai')); // true console.log(Boolean(12)); // true
3, JS case study
JS case: ask and obtain the user's name, age and gender in turn, and print the user information;
The complete code is demonstrated as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JS case</title> <script> //Case: ask and obtain the user's name, age and gender at one time, and print the user information var name = prompt('Please enter your name'); var age = prompt('Please enter your age'); var sex = prompt('Please enter your gender'); alert("Your name is:" + name + '\n' + "What is your age:" + age + '\n' + "What is your age:" + sex); </script> </head> <body> </body> </html>
4, Summary
😝 Because there are many contents, I decided to write separately. I will keep updating! Like friends, remember to praise! 😝