jQuery
Different versions of jQuery
1.x
- Compatible with old IE
- Larger file
2.x
- Some IE8 and below are not supported
- Smaller files, more efficient execution
3.x
- IE8 and below are no longer supported at all
- Some new API s are provided
- Provides a version that does not contain the ajax / animation API
jQuery local import
- This method requires downloading jQuery local files in advance. JQuery's official website: https://jquery.com/
<script type="text/javascript" src="./jquery-3.6.0.js"></script>
jQuery remote service introduction
- Load jQuery files through the remote CDN accelerator server
- When using, the computer must have a network
- Here is a free CDN acceleration service website: https://www.bootcdn.cn/
- The method of use is as follows:
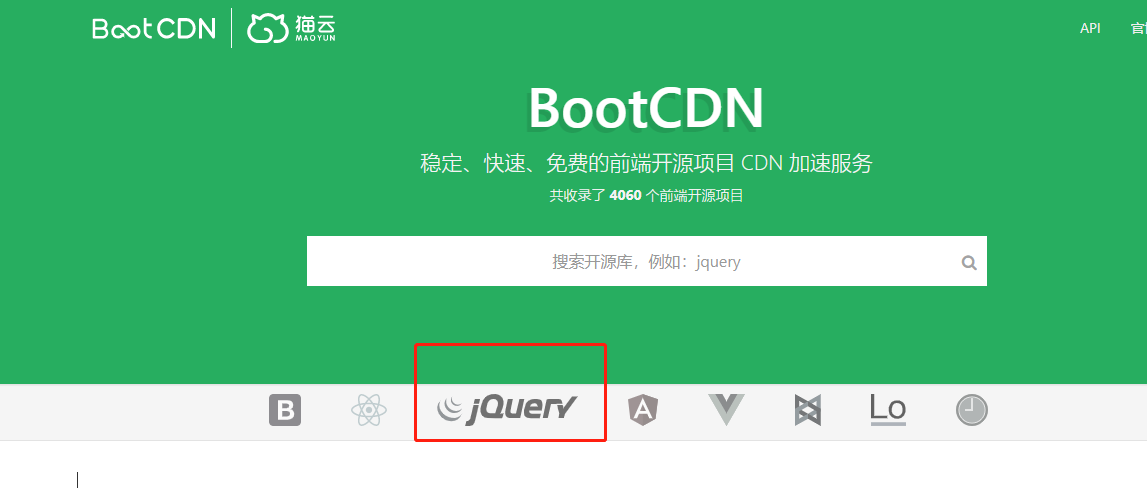
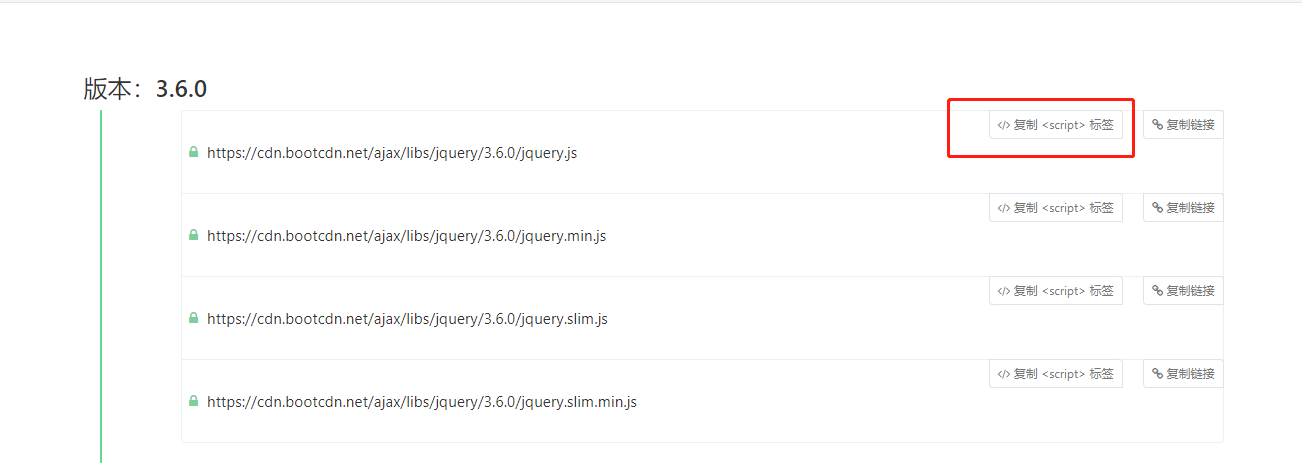
<script type="text/javascript" src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
The core of jQuery
jQuery core function
- JQuery function ($/ jQuery)
- What the jQuery library exposes is $/ jQuery
- After introducing the jQuery library, use $or jQuery directly
jQuery === $//true
jQuery core object
- Abbreviation: jQuery object
- Get the jQuery object: executing the jQuery function returns the jQuery object
- Using jQuery objects: Syntax: $obj xxx()
- The jQuery object is a pseudo element (possibly only one element) object that contains all dom element objects
Two uses of jQuery
Used as a general function: $(param)
- param parameter is a function: this callback function is executed after DOM loading is completed
- param parameter is selector string: find all matching tags and encapsulate them into jQuery objects
- param parameter is DOM object: encapsulate DOM object into jQuery object
- param parameter is html tag string: create a tag object and encapsulate it into a jQuery object
Here are four different parameters:
<!DOCTYPE html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script type="text/javascript" src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.js"></script>
<script type="text/javascript">
// 1). The parameter is function: after the DOM is loaded, this callback function is executed
$(function () {//Equivalent to window onlaod = function(){}
// 2). The argument is a selector string: find all matching tags and encapsulate them into a jQuery object
$('#btn').click(function() {/ / bind click event listening
// At this time, this is still the DOM element of the event (< button >)
// 3). The parameter is DOM object: encapsulate the DOM object into a jQuery object
// 4). The parameter is html tag string: create a tag object and encapsulate it into a jQuery object
$("<div>Xi'an, come on!</div>").appendTo("div");
})
})
</script>
<title>test</title>
</head>
<body>
<div>
<button id="btn">test</button>
</div>
</body>
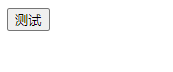
Use as object: $ xxx()
$.each()
- $. each() this method can be used to traverse any object (object, array)
- Syntax: $ each(object, [callback])
- The callback function has two parameters:
- The first parameter: (index) is the index of the member or array of the object
- The second parameter: (value) is the corresponding variable or content.
- If you need to exit each loop, you can make the callback function return false, and other return values will be ignored
let arr = ["waters","Heart ape","Wooden Dragon","medicine", "Yima"]
$.each(arr, function(index, value){
console.log(index + ":" + value);
})
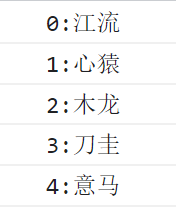
$.trim()
- $. trim(): remove spaces at both ends
let str = " Hello world ! ";
console.log("---"+$.trim(str)+"---");

jQuery basic behavior
length
- This attribute can get the number of DOM elements contained in the jQuery object
<body>
<div>
<button id="btn">test</button>
<button id="btn">test</button>
<button id="btn">test</button>
<button id="btn">test</button>
</div>
<script>
let $buttons = $("button");
console.log($buttons.length);//4
</script>
</body>
[index]
- Get the DOM element at the corresponding position
<script>
let $buttons = $("button");
console.log($buttons[2]);
</script>
each()
- Traverse all DOM elements contained
- Syntax: ` each(function)
<script>
let $buttons = $("button");
$buttons.each(function(index, domEle){
// this === domEle
console.log(index, domEle);
})
</script>
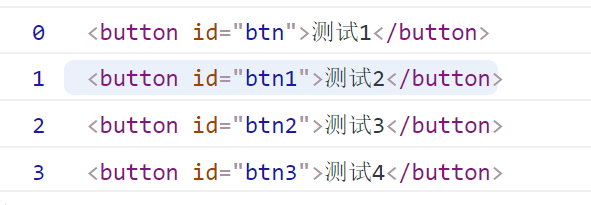
index()
- This method can get the subscript in the element