Code branch management
After splitting the microservices, the number of Application projects and some second-party packages will inevitably increase. I think it should be easy to exceed 10 + projects. Then these fine-grained splits will lead to trouble when publishing versions.
Show us a branch flow chart of git at this stage for reference only.
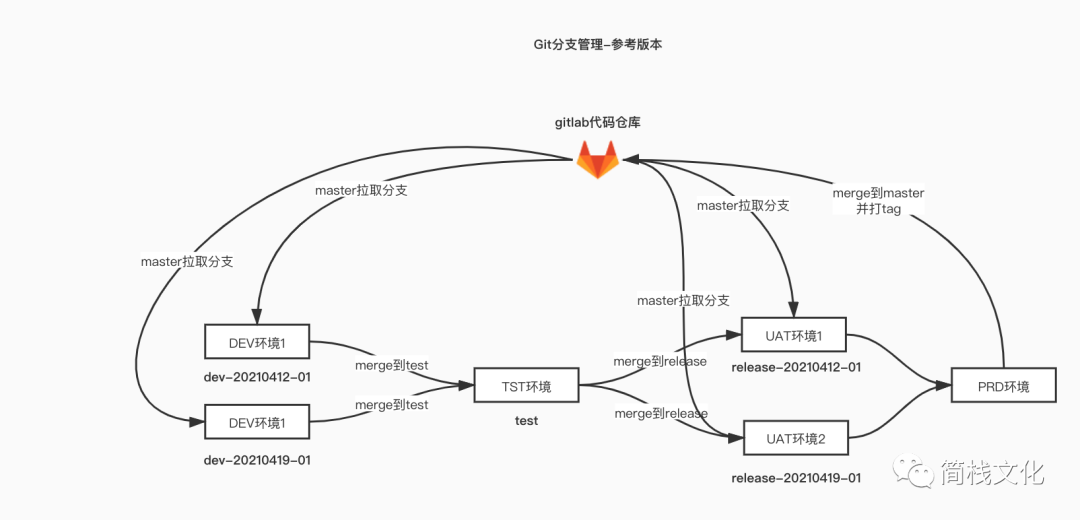
http://static.cyblogs.com/git_flow_publish_20210415.jpg
Briefly explain:
- Each time, the dev environment pulls a branch from the master, named dev - {release date} - {sequenceId}, and the sequenceId is superimposed from 01 to avoid repeated pulling of a version.
- At the test stage, it is also for convergence (be more strict in the early stage). When testing, the code needs to be merged into the test branch.
- At the UAT stage, you still need to pull branches from the master. If you want to pull branches again, you still need to strictly pull the code of the master branch. The main purpose is to keep consistent with the master and avoid overwriting others. It is called release - {release date} - {sequenceId}. The rules are the same as above.
- After production, after verification, you need to merge the code into the master branch and package the tag.
However, in this process, you need to pull new branches, merge branches, delete branches in batch, hit tag and other cumbersome operations. If there are many projects, it would be better to have a batch script. Here are some of the most commonly used ones for reference only:
Batch script
01 - batch pull new branches
For example, when a new iteration is about to start, you need to pull the dev branch from the master.
#! /bin/bash # Directory space HOME_DIR="/Users/chenyuan" WORK_DIR=$HOME_DIR"/Workspaces/xxx" // Replace with your working directory # Filter directory EXCLUDE_DIR_OR_FILE=("cr-test-demo" "git_batch_co_brach.sh" "git_batch_co_push_remote.sh" "git_batch_co_merge_push_remote.sh") # Branch name OLD_BR_NAME="$1" NEW_BR_NAME="$2" # Calibration parameters if [ -f $OLD_BR_NAME ]; then echo "Please input your old branch name." exit 0 fi if [ -f $NEW_BR_NAME ]; then echo "Please input your new branch name." exit 0 fi # Switch to a branch function gitCheckoutBrachAndPushRemote() { echo "-------- $1 --------" cd "$1" pwd git checkout $OLD_BR_NAME git fetch git pull git checkout -b $NEW_BR_NAME $OLD_BR_NAME git push --set-upstream origin $NEW_BR_NAME echo "success push branch " $NEW_BR_NAME " to remote" } ## get all folder in "$WORK_DIR" for i in `ls "$WORK_DIR"`;do cd "$WORK_DIR" if [[ -d $i ]] && [[ ! "${EXCLUDE_DIR_OR_FILE[@]}" =~ "${i}" ]];then gitCheckoutBrachAndPushRemote "$i" fi done
For specific use, for example, pull a new branch based on the master
./git_batch_co_push_remote.sh master dev-20210419-01
02 - batch switch branch
When making different requirements, you need to switch branches back and forth.
#! /bin/bash # Directory space HOME_DIR="/Users/chenyuan" WORK_DIR=$HOME_DIR"/Workspaces/xxx" // Replace with your working directory # Filter directory EXCLUDE_DIR_OR_FILE=("cr-test-demo" "git_batch_co_brach.sh" "git_batch_co_push_remote.sh" "git_batch_co_merge_push_remote.sh") # Branch name BR_NAME="$1" # Calibration parameters if [ -f $BR_NAME ]; then echo "Please input your branch name." exit 0 fi # Switch to a branch function gitCheckoutBrach() { cd "$1" pwd git fetch git checkout $BR_NAME echo "success checkout to " $BR_NAME } ## get all folder in "$WORK_DIR" for i in `ls "$WORK_DIR"`;do cd "$WORK_DIR" if [[ -d $i ]] && [[ ! "${EXCLUDE_DIR_OR_FILE[@]}" =~ "${i}" ]];then gitCheckoutBrach "$i" fi done
For specific use, first switch to the master branch.
./git_batch_co_brach.sh dev-20210412-01
03 - Delete remote branches in batch
Sometimes you accidentally write the wrong branch name, or there are too many branches. You need to delete remote branches in batch
#! /bin/bash # Directory space HOME_DIR="/Users/chenyuan" WORK_DIR=$HOME_DIR"/Workspaces/xxx" // Replace with your working directory # Filter directory EXCLUDE_DIR_OR_FILE=("cr-test-demo" "git_batch_co_brach.sh" "git_batch_co_push_remote.sh" "git_batch_co_merge_push_remote.sh") # Branch name BR_NAME="$1" # Calibration parameters if [ -f $BR_NAME ]; then echo "Please input your branch name." exit 0 fi # Switch to a branch function doSomething() { cd "$1" pwd git fetch git push origin --delete $BR_NAME echo "success delete remote branch: " $BR_NAME } ## get all folder in "$WORK_DIR" for i in `ls "$WORK_DIR"`;do cd "$WORK_DIR" if [[ -d $i ]] && [[ ! "${EXCLUDE_DIR_OR_FILE[@]}" =~ "${i}" ]];then doSomething "$i" fi done
Specific use
./git_batch_delete_remote.sh dev-20210412-01
As a result, the remote branch dev-20210412-01 can be deleted all at once.
04 - batch merge branch code
From one environment to another, it requires a lot of merge operations. In fact, Git's merge operation is much better than SVN's.
#! /bin/bash # Directory space HOME_DIR="/Users/chenyuan" WORK_DIR=$HOME_DIR"/Workspaces/xxx" // Replace with your working directory # Filter directory EXCLUDE_DIR_OR_FILE=("cr-test-demo" "git_batch_co_brach.sh" "git_batch_co_push_remote.sh" "git_batch_co_merge_push_remote.sh") # Branch name BR_NAME="$1" # Calibration parameters if [ -f $BR_NAME ]; then echo "Please input your branch name." exit 0 fi # Switch to a branch function gitCheckoutBrach() { cd "$1" pwd git merge $BR_NAME git push echo "success merge from branch" $BR_NAME " and push remote" } ## get all folder in "$WORK_DIR" for i in `ls "$WORK_DIR"`;do cd "$WORK_DIR" if [[ -d $i ]] && [[ ! "${EXCLUDE_DIR_OR_FILE[@]}" =~ "${i}" ]];then gitCheckoutBrach "$i" fi done
For specific use, remember to update the relevant code first~
For example, if you merge dev into test, update the corresponding code first, and then co to the test directory.
./git_batch_co_merge_push_remote.sh dev-20210412-01
05 - tag branches in batch
For example, our process is to put a new tag for the master after production
#! /bin/bash # Directory space HOME_DIR="/Users/chenyuan" WORK_DIR=$HOME_DIR"/Workspaces/xxx" // Replace with your working directory # Filter directory EXCLUDE_DIR_OR_FILE=("cr-test-demo" "git_batch_co_brach.sh" "git_batch_co_push_remote.sh" "git_batch_co_merge_push_remote.sh") # Branch name TAG_NAME=$1 # Calibration parameters if [ -f $TAG_NAME ]; then echo "Please input your tag name." exit 0 fi # Switch to a branch function doSomething() { cd "$1" pwd git fetch git checkout master git tag -a $TAG_NAME -m "Create branch $TAG_NAME" echo "success create tag " $TAG_NAME git push origin --tags echo "success push tag to remote" $TAG_NAME } ## get all folder in "$WORK_DIR" for i in `ls "$WORK_DIR"`;do cd "$WORK_DIR" if [[ -d $i ]] && [[ ! "${EXCLUDE_DIR_OR_FILE[@]}" =~ "${i}" ]];then doSomething "$i" fi done
For specific use, first switch to the master branch.
./git_batch_tag_push.sh v1.0.0
06 batch mvn packaging
#! /bin/bash # Directory space HOME_DIR="/Users/chenyuan" WORK_DIR=$HOME_DIR"/Workspaces/xxx" // Replace with your working directory # Projects requiring deploy NEED_DEPLOY_DIR_OR_FILE=("xxxx") // Add the name of the project you want to package here, separated by spaces ## get all folder in "$WORK_DIR" for i in "${NEED_DEPLOY_DIR_OR_FILE[@]}";do #cd "$WORK_DIR" echo "$WORK_DIR/$i"; cd "$WORK_DIR/$i"; git pull mvn install deploy -Dpmd.skip=true -Dcheckstyle.skip=true -Dmaven.test.skip=true done
Specific use
./git_batch_deploy_snapshot.sh
The above scripts were also released at that time. It took 20~30mins to write the scripts, and the release time was only 30mins. If I didn't write this script that day, I would spend 60+mins on business every time, and other unfamiliar people would spend more time on publishing. Development should standardize and produce those repetitive things. Can it really be sustainable~