Preface
I didn't pay much attention to this part of knowledge when I read Layer's related content before. Recently, I just used similar functions. First, I wrote Demo Warm-up.
That's probably what happens when you're done.
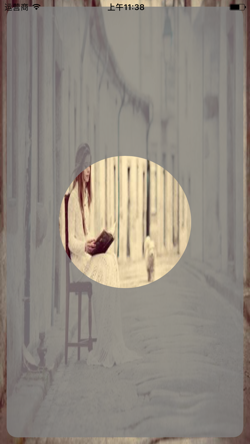
The general idea is to draw a mask with a Bessel curve and then add it to the Layer layer of the translucent View.
//Create a View UIView *maskView = [[UIView alloc] initWithFrame:self.view.bounds]; maskView.backgroundColor = [UIColor grayColor]; maskView.alpha = 0.8; [self.view addSubview:maskView]; //Bessel curve draws a rectangle with rounded corners UIBezierPath *bpath = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(10, 10, SCREEN_WIDTH - 20, SCREEN_HEIGHT - 20) cornerRadius:15]; //Bessel curve draws a circle [bpath appendPath:[UIBezierPath bezierPathWithArcCenter:maskView.center radius:100 startAngle:0 endAngle:2*M_PI clockwise:NO]]; //Create a CAShapeLayer layer CAShapeLayer *shapeLayer = [CAShapeLayer layer]; shapeLayer.path = bpath.CGPath; //Add Layer Mask maskView.layer.mask = shapeLayer;
Several points
- How to use the Bessel curve?
- What is CAShapeLayer?
- What does a layer mask do?
Use of Bessel Curve
There are not too many introductions here. Let's summarize some common methods.
Specific reference
Introduction to UIBezierPath This article
// Create basic paths + (instancetype)bezierPath; // Create a rectangular path + (instancetype)bezierPathWithRect:(CGRect)rect; // Create an elliptic path + (instancetype)bezierPathWithOvalInRect:(CGRect)rect; // Create rounded rectangle + (instancetype)bezierPathWithRoundedRect:(CGRect)rect cornerRadius:(CGFloat)cornerRadius; // rounds all corners with the same horizontal and vertical radius // Create a rectangular path with rounded corners at the specified location + (instancetype)bezierPathWithRoundedRect:(CGRect)rect byRoundingCorners:(UIRectCorner)corners cornerRadii:(CGSize)cornerRadii; // Creating Arc Path + (instancetype)bezierPathWithArcCenter:(CGPoint)center radius:(CGFloat)radius startAngle:(CGFloat)startAngle endAngle:(CGFloat)endAngle clockwise:(BOOL)clockwise; // Create through CGPath + (instancetype)bezierPathWithCGPath:(CGPathRef)CGPath;
What is CAShapeLayer
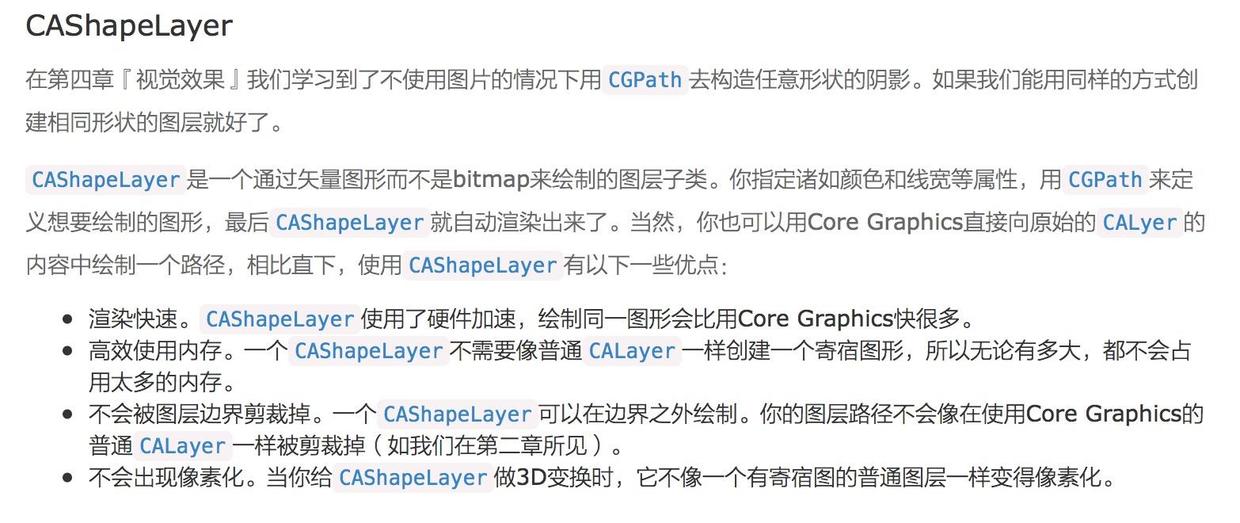
What I understand is that the CGPath attribute of this layer can be used to define the graph you want to draw.
That is to say, through this layer and Bessel curve, I can get any shape I want.
The code above, change a few places to feel it
//The outermost layer is covered with a large rounded rectangle UIBezierPath *bpath = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(10, 10, SCREEN_WIDTH - 20, SCREEN_HEIGHT - 20) cornerRadius:15]; //Add a circle in the middle [bpath appendPath:[UIBezierPath bezierPathWithArcCenter:maskView.center radius:100 startAngle:0 endAngle:2*M_PI clockwise:NO]]; CAShapeLayer *shapeLayer = [CAShapeLayer layer]; shapeLayer.path = bpath.CGPath; shapeLayer.strokeColor = [UIColor redColor].CGColor; //maskView.layer.mask = shapeLayer; [self.view.layer addSublayer:shapeLayer];
The black area in the picture is the shape of the layer created.
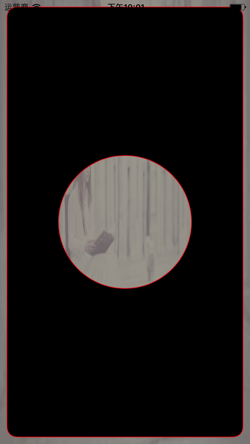
It should be noted that if you want to preserve the part outside the circle, clockwise (clockwise) should be chosen as NO. If you want to preserve the part outside the rectangle, the rectangle needs to draw the path in the opposite direction.
UIBezierPath *bpath = [UIBezierPath bezierPathWithRoundedRect:CGRectMake(10, 10, SCREEN_WIDTH - 20, SCREEN_HEIGHT - 20) cornerRadius:15]; [bpath appendPath:[UIBezierPath bezierPathWithArcCenter:CGPointMake(SCREEN_WIDTH/2, 150) radius:100 startAngle:0 endAngle:2*M_PI clockwise:NO]]; // - Bezier Path ByReversing Path, plotting the path in the opposite direction [bpath appendPath:[[UIBezierPath bezierPathWithRoundedRect:CGRectMake(50, 350, SCREEN_WIDTH - 100, 100) cornerRadius:20] bezierPathByReversingPath]]; CAShapeLayer *shapeLayer = [CAShapeLayer layer]; shapeLayer.path = bpath.CGPath; shapeLayer.strokeColor = [UIColor redColor].CGColor; //maskView.layer.mask = shapeLayer; [self.view.layer addSublayer:shapeLayer];
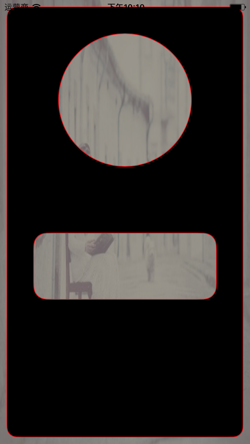
layer mask
Let's borrow the concept from the core animation. The biscuit cutter is very vivid.
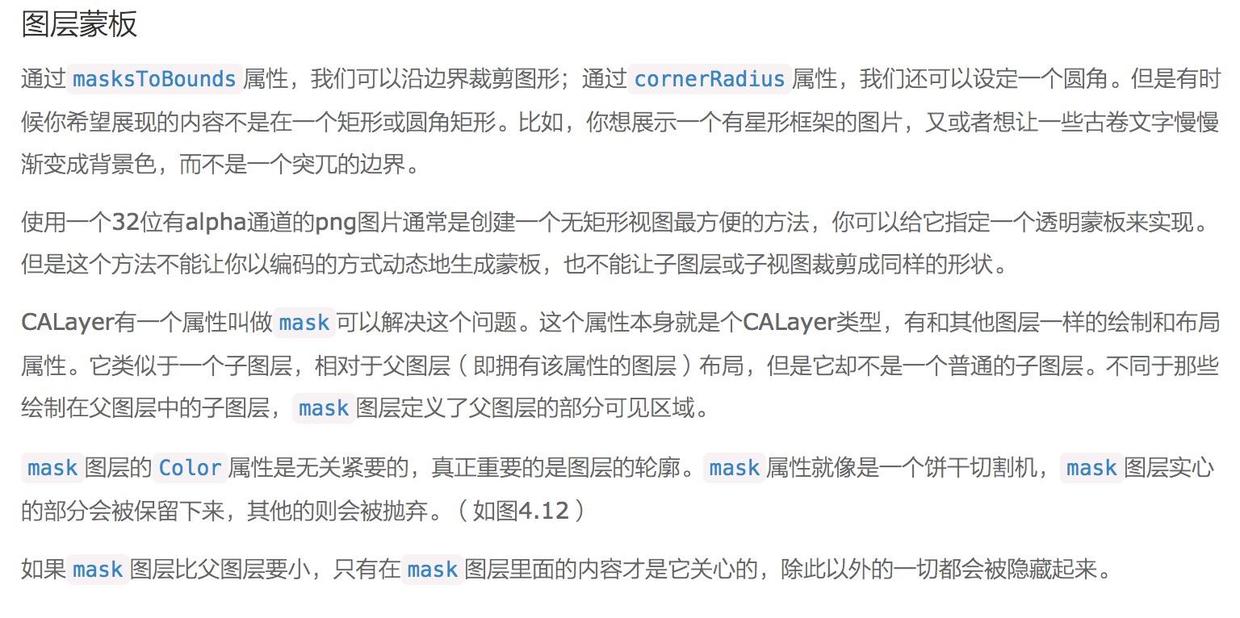
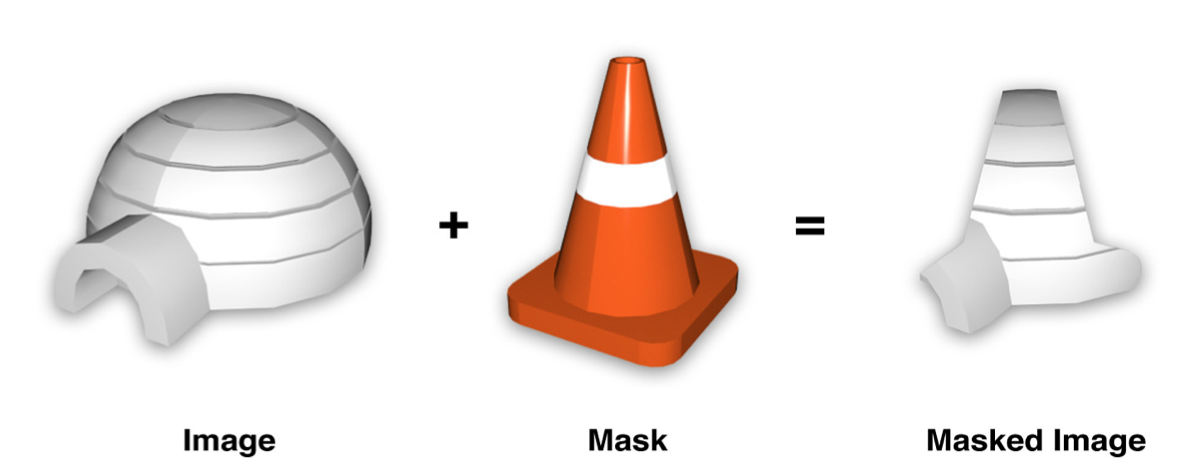
Author: Light jun
Link: http://www.jianshu.com/p/50c46c72e3dd
Source: Brief Book
Copyright belongs to the author. For commercial reprints, please contact the author for authorization. For non-commercial reprints, please indicate the source.