1, Method
Binary image geometry extraction and separation is one of the key points in machine vision. It is widely used in CT image analysis and robot visual perception. OpenCV provides the most effective tool for binary image geometric feature description and analysis - SimpleBlobDetector class, which can realize the separation and analysis of binary image geometry. The reason why it is powerful is that it integrates the functions of some other API s in OpenCV, mainly including three:
- Automatic image grayscale and binarization, and the radius is obtained according to the input step size and threshold
- The contour search function is realized, and all contours can be found,
- Then, based on the calculation of geometric moment, various filtering based on geometric features are realized
Before learning the correlation function of Blob feature detector, let's first look at the geometric features used in Blob geometric feature filtering
the measure of area
The BLOB feature detector can filter the results according to the area size. Only the geometry with the area within the specified range will be detected and marked by the BLOB feature. In this way, it can realize size classification based on geometric area. It should be noted that the area here is based on pixel units, which is mainly conducive to the calculation of geometric moments.
Roundness
The formula of roundness can be expressed as

- When C equals 1, the shape represents a perfect circle
- When C approaches 0, the shape represents a polygon or rectangle close to a straight line.
- When the C value is between 0.75 and 0.85, most of the time it means that polygons with rectangular or equilateral edges appear.
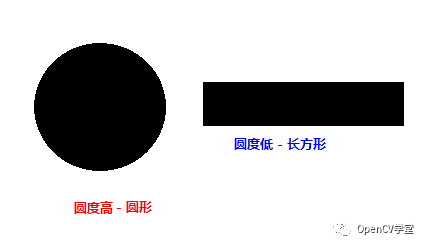
Inertia rate
The inertia rate is the same as the eccentricity. The eccentricity of the circle is equal to 0, the eccentricity of the ellipse is between 0 and 1, and the eccentricity of the straight line is close to 0. It is easier to calculate the inertia rate based on the geometric moment than to calculate the eccentricity. Therefore, OpenCV selects the characteristic value of the inertia rate, and the eccentricity can be calculated according to the inertia rate. The relationship between the eccentricity and the inertia rate is shown as follows

Convexity
A measure that indicates whether the geometry is convex or concave. To put it bluntly, you can filter convex polygons or concave polygons according to parameters. The input parameters are generally between 0 and 1, with a minimum of 0 and a maximum of 1. Generally, the circle will be more than 0.5
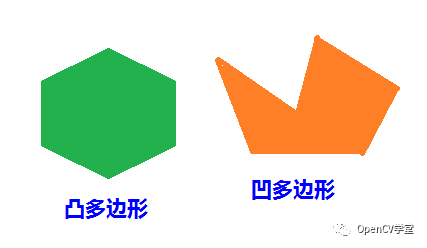
2, Demonstration
In the demonstration part, two examples are used to illustrate the different uses of BLOB feature. The first example detects the sunflower disc through BLOB feature, and the second example filters and classifies the geometry through BLOB feature detection.
Example 1: original drawing
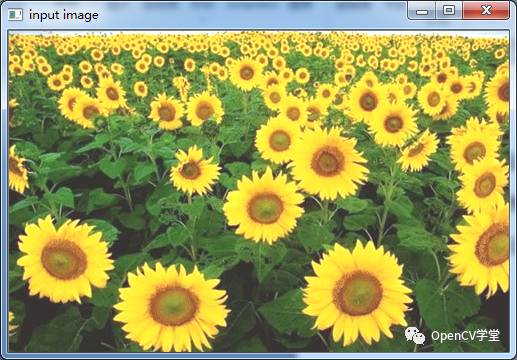
BLOB test results
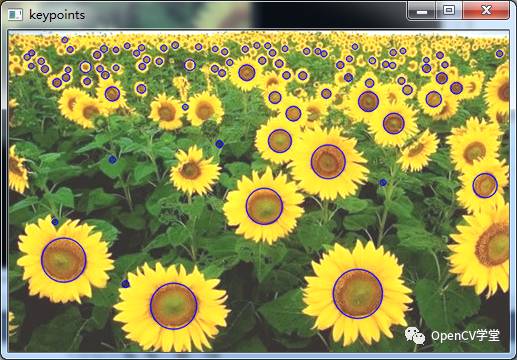
The relevant codes are as follows:
// Initialize BLOB parameters SimpleBlobDetector::Params params; params.minDistBetweenBlobs = 0.0f; params.filterByInertia = false; params.filterByConvexity = false; params.filterByColor = false; params.filterByCircularity = false; params.filterByArea = false; // Declare to filter by area and set the maximum and minimum area params.filterByArea = true; params.minArea = 20.0f; params.maxArea = 2000.0f; // Declare to set the maximum and minimum roundness based on roundness filtering params.filterByCircularity = true; params.minCircularity = 0.5; params.maxCircularity = 1.0; // Convex hull shape analysis - filtering concave hull params.filterByConvexity = true; params.minConvexity = 0.5; params.minConvexity = 1.0; // Parameter initializes the BLOB detector, Ptr<SimpleBlobDetector> detector = SimpleBlobDetector::create(params); vector<KeyPoint> keypoints; // Detected features and drawn features detector->detect(src, keypoints, Mat()); Mat kp_image; drawKeypoints(src, keypoints, kp_image, Scalar(255, 0, 0), DrawMatchesFlags::DRAW_RICH_KEYPOINTS); imshow("keypoints", kp_image);
Example 2: original drawing
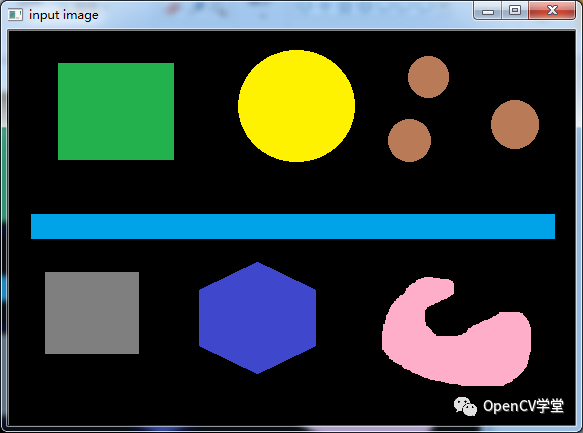
Filter operation results with different parameters
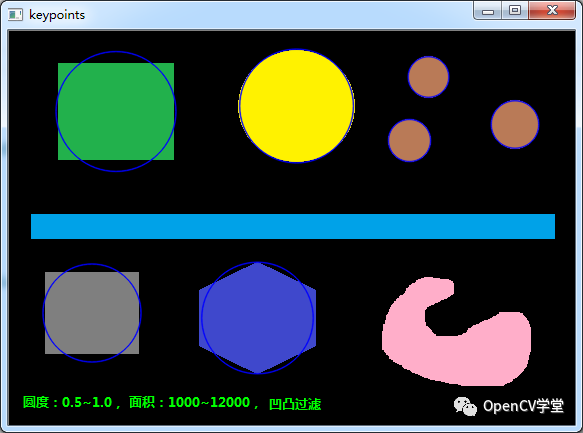
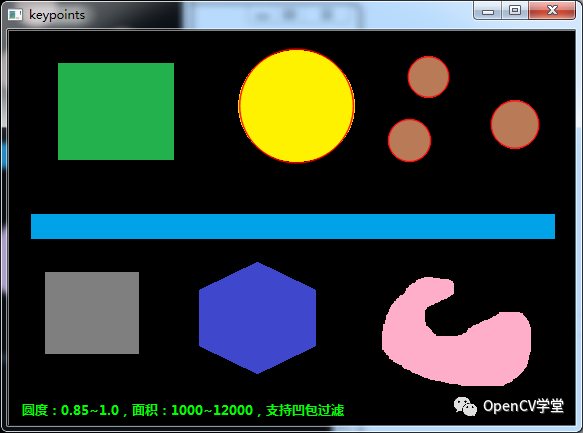
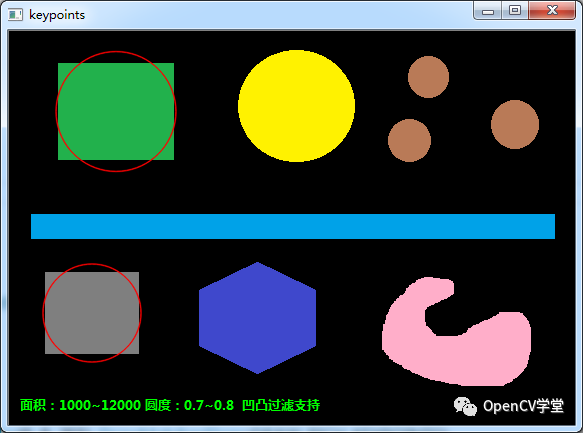
Blue and red are the test results
The relevant codes are as follows
// Geometry filtering // Declare to filter by area and set the maximum and minimum area params.filterByArea = true; params.minArea = 1000.0f; params.maxArea = 12000.0f; // Declare to set the maximum and minimum roundness based on roundness filtering params.filterByCircularity = true; params.minCircularity = 0.7; params.maxCircularity = 0.8; // Convex hull shape analysis - filtering concave hull params.filterByConvexity = true; params.minConvexity = 0.0; params.minConvexity = 0.5; // Parameter initializes the BLOB detector, Ptr<SimpleBlobDetector> detector = SimpleBlobDetector::create(params); vector<KeyPoint> keypoints; // Detected features and drawn features detector->detect(src, keypoints, Mat()); Mat kp_image; drawKeypoints(src, keypoints, kp_image, Scalar(0, 0, 255), DrawMatchesFlags::DRAW_RICH_KEYPOINTS); imshow("keypoints", kp_image);
Summary:
In addition to using SimpleBlobDetector class, BLOB feature extraction and analysis of images can also be realized by combining findContours with geometric moment calculation. The latter investigates the familiarity with OpenCV related API functions and the mastery of image processing related knowledge.