1. Overview of BOM
1.1 What is a BOM
The BOM (Browser Object Model), or==Browser Object Model, provides a content-independent object that interacts with the browser window==, and its core object is window.
BOM consists of a series of related objects, and each object provides many methods and properties.
BOM lacks standards, the standardization organization of JavaScript grammar is ECMA, the standardization organization of DOM is W3C, and BOM was originally Netscape liu
Part of the viewer standard.
Composition of 1.2 BOM
BOM is larger than DOM and contains DOM.
**The window object is the top level object of the browser,**It has a dual role.
-
It is an interface for JS to access browser windows.
-
It is a global object. Variables and functions defined in the global scope become properties and methods of window objects.
You can omit window when calling, and the dialog boxes you learned earlier belong to window object methods, such as alert(), prompt().
**Note: **A special property under window window.name
<body> <script> // window.document.querySelector() var num = 10; console.log(num); console.log(window.num); function fn() { console.log(11); } fn(); window.fn(); // alert(11); // window.alert(11) console.dir(window); // var name = 10; console.log(window.name); </script> </body>
2. Common events for windows objects
2.1 Window Load Events
window.onload = function(){} perhaps window.addEventListener("load",function(){});
window.onload is a window (page) loading event that is triggered when the document content is fully loaded (including images, script files, CSS)
File, etc.), on the handler called.
Be careful
- With window.onload, you can write the JS code on top of the page element, because onload is waiting for the page content to be fully loaded.
Then execute the processing function.
-
The window.onload traditional registration event method can only be written once, if there are more than one, the last window.onload will prevail.
-
There is no limit to using addEventListener
document.addEventListener('DOMContentLoaded',function(){})
When the DOMContentLoaded event is triggered, only when the DOM load is complete, excluding stylesheets, pictures, flash, and so on.
If there are a lot of pictures on the page, it may take a long time from user access to onload trigger, interaction effect cannot be achieved, it must affect the use of
User experience, it is appropriate to use the DOMContentLoaded event at this time.
// Case: Display order: 33 22 <script> // window.onload = function() { // var btn = document.querySelector('button'); // btn.addEventListener('click', function() { // alert('tap me'); // }) // } // window.onload = function() { // alert(22); // } window.addEventListener('load', function() { var btn = document.querySelector('button'); btn.addEventListener('click', function() { alert('Click on me'); }) }) window.addEventListener('load', function() { alert(22); }) document.addEventListener('DOMContentLoaded', function() { alert(33); }) // Page content such as load is fully loaded, including page dom element picture flash CSS and so on // DOMContentLoaded is loaded by DOM and can be performed faster than load without pictures such as falsh css </script>
2.2 Resize Window Event
window.onresize = function(){} window.addEventListener("resize",function(){});
window.onresize is a handler that is called when a window is resized to load events.
Be careful:
-
This event is triggered whenever the window size changes in pixels.
-
This event is often used to complete a responsive layout. window.innerWidth Current Screen Width
// Case study: div is hidden when window is less than 800px <body> <script> window.addEventListener('load', function() { var div = document.querySelector('div'); window.addEventListener('resize', function() { console.log(window.innerWidth); console.log('Changed'); if (window.innerWidth <= 800) { div.style.display = 'none'; } else { div.style.display = 'block'; } }) }) </script> <div></div> </body>
3. Timer
3.1 Two timers
The window object provides us with two very useful methods, timers.
-
setTimeout()
-
setInterval()
3.2 setTimeout() Timer
window.setTimeout(Call function, [Delayed milliseconds]);
The setTimeout() method is used to set a timer that executes the calling function after the timer expires.
Be careful:
-
Windows can be omitted.
-
This calling function can either write a function directly, or write a function name or take the form of a string ~'function name ()'~~ The third is not recommended
-
Delayed milliseconds omit defaults to 0, and must be milliseconds if written.
-
Because there may be many timers, we often assign an identifier to a timer.
// Case: call Timer1 after 3s, call timer2 after 5S // Note: function name is not followed by () <body> <script> // 1. setTimeout // Syntax specification: window.setTimeout (call function, delay time); // 1. This window can be omitted when called // 2. This delay time unit is in milliseconds but can be omitted if the default is 0 // 3. This calling function can write function directly or function name as well as a'function name ()' // 4. There may be a lot of timers on the page. We often give the timer an identifier (name) // setTimeout(function() { // console.log('time is up'); // }, 2000); function callback() { console.log('Exploded'); } var timer1 = setTimeout(callback, 3000); var timer2 = setTimeout(callback, 5000); // setTimeout('callback()', 3000); //We do not advocate this writing </script> </body>
3.3 clearTimeout() Stop Timer
window.clearTimeout(timeoutID)
The clearTimeout() method cancels the timer previously established by calling setTimeout().
Be careful:
-
Windows can be omitted.
-
The parameter inside is the identifier of the timer.
// Case study: Clicking the stop button within 5 seconds will not show'explosion'in the console <body> <button>Click Stop Timer</button> <script> var btn = document.querySelector('button'); var timer = setTimeout(function() { console.log('Exploded'); }, 5000); btn.addEventListener('click', function() { clearTimeout(timer); }) </script> </body>
3.4 setInterval() Timer
window.setInterval(callback, [Interval in milliseconds]);
The setInterval() method calls a function repeatedly, and every other time, it calls the callback function
Be careful:
-
Windows can be omitted.
-
This calling function can either write the function directly, or write the function name or take the form of a string'function name ()'.
-
Interval milliseconds omitted defaults to 0, if written, must be milliseconds, indicating how many milliseconds this function is called automatically.
4. Because there may be many timers, we often assign an identifier to the timer.
-
The first execution is also performed after an interval of milliseconds and then every millisecond.
// The console prints this Continue Output every second <body> <script> // 1. setInterval // Syntax specification: window.setInterval (call function, delay time); setInterval(function() { console.log('Continue Output'); }, 1000); // 2. When the setTimeout delay is reached, call the callback function and end the timer with only one call // 3. setInterval calls this callback function every other delay, and it calls it many times, repeatedly </script> </body>
3.5 Stop setInterval() Timer
window.clearInterval(intervalID);
The clearInterval() method cancels the timer previously established by calling setInterval().
Be careful:
-
Windows can be omitted.
-
The parameter inside is the identifier of the timer
// Note: You need to define a global variable timer=null <body> <button class="begin">Turn on the timer</button> <button class="stop">Stop Timer</button> <script> var begin = document.querySelector('.begin'); var stop = document.querySelector('.stop'); var timer = null; // The global variable null is an empty object begin.addEventListener('click', function() { timer = setInterval(function() { console.log('ni hao ma'); }, 1000); }) stop.addEventListener('click', function() { clearInterval(timer); }) </script> </body>
3.6 this pointing
The direction of this is not certain when a function is defined. Only when a function is executed can the direction of this be determined. Generally, this is
The end point is at the object that called it
At this stage, let's first look at a few this points
-
This points to the global object window in a global scope or function (note that this points to the window in the timer)
-
Who calls this in a method call to whom
-
Instance of this pointing to constructor in constructor
<body> <button>click</button> <script> // this points to the problem this usually ends up with the object that called it // 1. This points to the global object window in the global scope or in a normal function (note that this points to the window in the timer) console.log(this); // window function fn() { console.log(this); // window } window.fn(); window.setTimeout(function() { console.log(this); // window }, 1000); // 2. Who calls this in a method call points to whom var o = { sayHi: function() { console.log(this); // this is pointing to the o object } } o.sayHi(); var btn = document.querySelector('button'); // btn.onclick = function() { // console.log(this); // this is pointing to the btn button object // } btn.addEventListener('click', function() { console.log(this); // this is pointing to the btn button object }) // 3. Examples of this pointing to constructors in constructors function Fun() { console.log(this); // this points to a fun instance object } var fun = new Fun(); </script> </body>
4. JS Execution Mechanism
JS is single threaded
One of the main features of JavaScript is that it is single-threaded, that is, it can only do one thing at a time. This is because of the Javascript footer
The mission of the language was born - JavaScript was born to handle user interaction on pages and to manipulate DOM. For example, we're right
A DOM element cannot be added and deleted simultaneously. You should add it before deleting it.
A single thread means that all tasks need to be queued before the last one can be executed. The problem with this is that if
JS takes too long to execute, which results in inconsistent rendering of pages, resulting in a sense of load blocking for page rendering
4.1 A question
What is the result of executing the following code?
console.log(1); setTimeout(function () { console.log(3); }, 1000); console.log(2); // 1 2 3
So what happens when the following code executes?
console.log(1); setTimeout(function () { console.log(3); }, 0); console.log(2); // 1 2 3
4.2 Synchronization and Asynchronization
To solve this problem, using the computing power of multi-core CPU s, HTML5 proposes the Web Worker standard, which allows JavaScript scripts to create
Build multiple threads. As a result, synchronization and asynchronization occur in JS.
synchronization
The execution order of the program is consistent with the order of tasks. For example, simultaneous cooking
Method: We want to boil water and cook rice, wait for the water to boil (after 10 minutes), then cut and stir-fry vegetables.
asynchronous
When you do one thing, because it takes a long time, you can do other things at the same time. For example, do
In the asynchronous way of cooking rice, we use the 10 minutes to cut and stir-fry while boiling water.
The essential difference is that the processes on this pipeline are executed in different order.
4.3 Execution instructions for synchronous and asynchronous
Synchronize Tasks
Synchronization tasks are executed on the main thread, forming a ==execution stack==.
Asynchronous Tasks
Asynchronization of JS is achieved through callback functions.
In general, there are three types of asynchronous tasks:
1. Common events, such as click, resize, etc.
2. Resource loading, such as load, error, etc.
3. Timers, including setInterval, setTimeout, etc.
Asynchronous task-related callback functions are added to the ==task queue==task queue (task queue is also known as message queue).
4.4 JS Execution Mechanism
-
Execute the synchronization task in the execution stack first.
-
Asynchronous tasks (callback functions) are placed in the task queue.
-
Once all synchronous tasks in the execution stack are executed, the system reads the asynchronous tasks in the task queue in order, and the read asynchronous tasks
The transaction ends waiting, enters the execution stack, and begins execution.
This mechanism is called an event loop because the main thread repeatedly obtains, executes, retrieves, and executes tasks.
event loop).
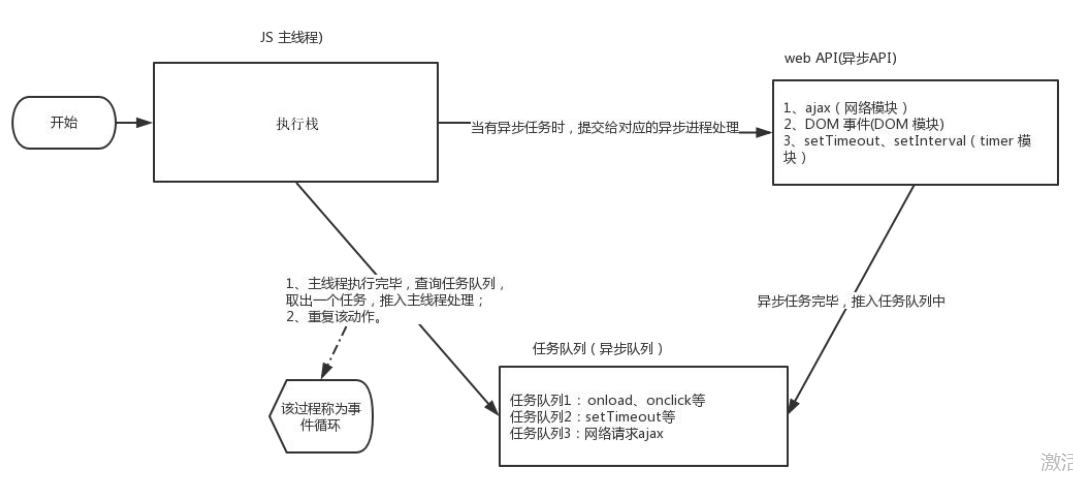
5. location object
5.1 What is a location object
The window object gives us a location property to get or set the form's URL, and it can be used to parse the URL because
This property returns an object, so we call it a location object.
5.2 URL
Uniform Resource Locator (URL) is the address of a standard resource on the Internet. Every file on the Internet has a
A unique URL that contains information about the location of the file and what the browser should do with it.
The general syntax format for URL s is:
protocol://host[:port]/path/[?query]#fragment http://www.itcast.cn/index.html?name=andy&age=18#link
5.3 Properties of the location object
- Keep in mind: href and search
Method of 5.4 location object
[External chain picture transfer failed, source station may have anti-theft chain mechanism, it is recommended to save the picture and upload it directly (img-49r50wVV-1638979307010)(img/image-20211208235416732.png)]
6. navigator object
The navigator object contains information about the browser and has many attributes, the most common of which is the userAgent, which can be returned by the client
The value of the user-agent header of the client sending server.
The following front-end code can tell which user's terminal opens the page and makes a jump
if((navigator.userAgent.match(/(phone|pad|pod|iPhone|iPod|ios|iPad|Android| Mobile|BlackBerry|IEMobile|MQQBrowser|JUC|Fennec|wOSBrowser|BrowserNG|WebOS |Symbian|Windows Phone)/i))) { window.location.href = ""; //Mobile phone } else { window.location.href = ""; //Computer }
7. history object
The window object provides us with a history object that interacts with the browser history. The object contains the user (in the browser window)
Visited URL.
- Hisry objects are rarely used in actual development, but they can be seen in some OA office systems.
8. Related Cases
3.2 Cases: Hidden Cases of Picture Advertising after 5s
// Case: Hidden Picture Advertising Cases after 5s <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> </head> <body> <img src="images/ad.jpg" alt="" class="ad"> <script> var ad = document.querySelector('.ad'); setTimeout(function() { ad.style.display = 'none'; }, 5000); </script> </body> </html>
3.4 Cases: Simple Countdown Case
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <style> div { margin: 200px; } span { display: inline-block; width: 40px; height: 40px; background-color: #333; font-size: 20px; color: #fff; text-align: center; line-height: 40px; } </style> </head> <body> <div> <span class="hour">1</span> <span class="minute">2</span> <span class="second">3</span> </div> <script> // 1. Get Elements var hour = document.querySelector('.hour'); // Black box for hours var minute = document.querySelector('.minute'); // Black box for minutes var second = document.querySelector('.second'); // Black box for seconds var inputTime = +new Date('2019-5-1 18:00:00'); // Returns the total number of milliseconds of user input time countDown(); // Let's call this function once to prevent the first page refresh from getting blank // 2. Turn on the timer setInterval(countDown, 1000); function countDown() { var nowTime = +new Date(); // Returns the total number of milliseconds in the current time var times = (inputTime - nowTime) / 1000; // times is the total number of seconds remaining var h = parseInt(times / 60 / 60 % 24); //time h = h < 10 ? '0' + h : h; hour.innerHTML = h; // Give the remaining hours to the hour black box var m = parseInt(times / 60 % 60); // branch m = m < 10 ? '0' + m : m; minute.innerHTML = m; var s = parseInt(times % 60); // Current seconds s = s < 10 ? '0' + s : s; second.innerHTML = s; } </script> </body> </html
3.5 Cases: Sending SMS Cases
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> </head> <body> Phone number: <input type="number"> <button>Send out</button> <script> // When the button is clicked, disabled is disabled to true // Notice that the contents of the button are modified through innerHTML // The number of seconds inside varies, so a timer is needed // Define a variable that decreases within the timer // If the variable 0 says it's time, we need to stop the timer and restore the initial state of the button var btn = document.querySelector('button'); var time = 3; // Define the number of seconds remaining btn.addEventListener('click', function() { btn.disabled = true; var timer = setInterval(function() { if (time == 0) { // Clear timer and restore button clearInterval(timer); btn.disabled = false; btn.innerHTML = 'Send out'; } else { btn.innerHTML = 'Remaining' + time + 'second'; time--; } }, 1000); }) </script> </body> </html>