Brief Analysis of Several Port Scanning Methods
My Blog [My Blog]: New Zero Cloud Blog - Cloud Wing Campus Program
Everyone can learn!!!
Article Directory
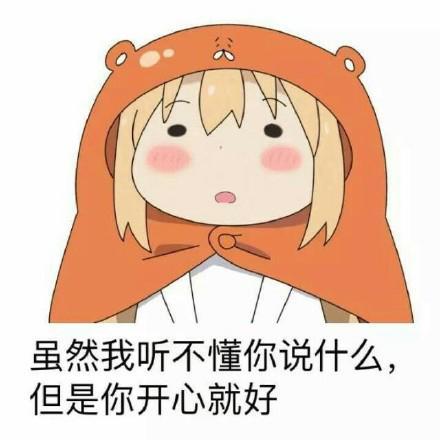
1.nmap probe port
nmap can set the parameter - min-hostgroup when scanning multiple hosts. Setting this parameter can scan multiple hosts in parallel
Divide the hosts into groups and scan one group at a time.Convenient and fast
Give an example:
- min-hostgroup 50 nmap is a group of 50 hosts, the results will not be displayed until 50 hosts are scanned. See nmap website for details.
#coding=utf-8 import nmap from queue import Queue from threading import Thread def portscan(ip): portlist = [] nm = nmap.PortScannerYield() for r in nm.scan(ip,ports='1-10000',arguments='-sS --min-hostgroup'): m = r[1]['scan'][ip]['tcp'] for p in m: temp = str(p) + "----" +m[p]['state'] portlist.append(temp) print(portlist) class Consumer(Thread): def __init__(self, q): Thread.__init__(self) self.q = q def run(self): while not self.q.empty(): ip = self.q.get() try: portscan(ip) except Exception as e: print(e) continue def producer(ip_list): num = 10 threads = [] q = Queue() for i in ip_list: print(i) q.put(i) threads = [Consumer(q) for i in range(0,int(num))] for t in threads: t.start() for t in threads: t.join() ip_list =['120.78.207.76', '120.78.207.231', '120.78.207.18', '120.78.207.233', '120.78.207.165', '120.78.207.48', '120.78.207.112', '120.78.207.27', '120.78.207.51', '120.78.207.8'] producer(ip_list)
2.masscan probe port
(1) Call python masscan
By default, masscan sends syn packets, and if the target host returns ack+syn, the port is open.The specific process is as follows
A:192.168.70.142
B:192.168.0.143 Open port 3306
(1)A->B syn
(2)B->A syn+ack
(3)A->B RST
Detect Open Ports
A->B syn
B->A rst
Give an example:
def portscan(ip): mas = masscan.PortScanner() mas.scan(ip,ports='1-65535') print(mas.scan_result)
Detect using system commands
Usage method
Scan class B subnet for port 443 Masscan 10.11.0.0/16 -p443 Scan Class B Subnets for Ports 80 or 443 Masscan 10.11.0.0/16 -p80,443 Scan Class B subnets for 100 common ports, 100,000 packets per second Masscan 10.11.0.0/16 --top-ports 100 -rate 100000 Output of Results -oX filename: XML output to filename. -oG filename: The grepable format output to filename. -oJ filename: Output to filename in JSON format.
3.socket detection port
The socket probe port does not send a complete three-time handshake package as follows.
A:192.168.70.142
B:192.168.0.143 Open port 3306
A discards data after receiving syn+ack packets returned by B.
Detect Open Port
A sends syn,B does not open port 33, so RST packets are returned.
ef portscan(ip,port): try: s = socket.socket(socket.AF_INET,socket.SOCK_STREAM) s.settimeout(0.2) status = s.connect_ex((ip,port)) if status == 0: temp_str = str(ip) + "---" + str(port) + "---open" port_list.append(temp_str) else: pass except Exception as e: pass finally: s.close()
4.telnet probe port
The telnet probe port uses a full three-time handshake connection, using the command telnet ip port, and the outgoing process is as follows
A:192.168.70.142
B:192.168.0.143 Open port 3306
telnet 192.168.0.143 3306
The process is as follows:
Connect using TCP three-time handshake: SYN -> SYN + ACK + ACK
Probe for non-existent ports, send SYN packets, and then discard RST packets
If there is a return value, the port is open, otherwise the port is closed.
def portscan(ip,port): try: t = telnetlib.Telnet(ip,port=port,timeout=0.2) if t: temp_str = str(ip) + '---' + str(port) port_list.append(temp_str) except Exception as e: print(e) pass
5.nc probe port
The NC probing port uses a full three-time handshake connection, using the command nc-v-w 1-z IP port, and the outgoing process is the same as telent probing.
Detect open port packets
Detect Packets on Unopened Ports
The port is open with a return value of 0, which can be used as a basis for judgment.
def portscan(ip,port): command = 'nc -v -w 1 -z {0} {1}'.format(ip,port) m = os.system(command) if m == 0: temp_str = str(ip) + "---" + str(port) port_list.append(temp_str) else: pass
6. Summary
The results of nmap as a scanner port are more detailed than those of several other methods.Slow
.
If efficiency is a concern, socket s are recommended.Not detailed
Each has its own merits and demerits
Follow the new Zero Cloud blog and get the original PDF!
More original tutorials to follow blog
I have sorted out my original technology series articles, self-learning experience summary into PDF with catalog, which makes it easy for everyone to download and learn, and keep updating. I can share the content on PDF with me, not only to learn technology, but also to communicate learning methods together!Keep growing beyond coding!Free tutorial video for everyone!