Hello, everyone. I'm safe and sound.
catalogue
4. Input and output of birth date
5.2 calculation to the nth power of
6. Input and exchange output according to format
8. Calculate the value of the expression
9. Calculate division with remainder
11. Calculate the perimeter and area of the triangle
12. Calculate the volume of the sphere
Conclusion: meet Enron meet you, not negative code, not negative Qing!
[preface]
The title is relatively simple, 130 to the title, two brush, because many solutions written before are too cumbersome, so it takes a few days to go over it again.
1. Judgment letter
Original title link: Judgment letter_ Niuke Tiba_ Niuke network
Title Description:
Code execution:
Code 1:
#include<stdio.h> int main() { int ch = 0; //Multi group input while((ch=getchar())!=EOF) { if(ch>='A' && ch<='Z' || ch>='a' && ch<='z') { printf("YES\n"); } else { printf("NO\n"); } getchar();//Be sure to clear the buffer } return 0; }
Code 2:
//isplpha - is a library function designed to determine whether a character is a letter //If it is a letter, it returns a value other than 0, and if it is not a letter, it returns 0 //Header file #include < ctype. Required h> #include<stdio.h> #include<ctype.h> int main() { int ch = 0; //Multi group input while((ch = getchar())!=EOF) { //Judge and output if(isalpha(ch)) { printf("YES\n"); } else { printf("NO\n"); } getchar();//Clear buffer \ n } return 0; }
2. Character Christmas tree
Original title link: Character Christmas tree_ Niuke Tiba_ Niuke network
Title Description:
Example:
Input: 1 Output: 1 1 1 1 1 1 1 1 1 1 1 1 1 1 1
Code execution:
#include<stdio.h> int main() { char ch = 0; ch = getchar(); int i = 0; //Print one line per cycle //Each line consists of two parts: spaces and characters for (i = 0; i < 5; i++) { //Print spaces int j = 0; for (j = 0; j < 5 - 1 - i; j++) { printf(" "); } //Print character for (j = 0; j <= i; j++) { printf("%c ", ch); } printf("\n"); } return 0; }
3.ASCII code
Original title link: ASCII code_ Niuke Tiba_ Niuke network
Title Description:
Code execution:
#include<stdio.h> int main() { char arr[] = {73,32,99,97,110,32,100,111,32,105,116,33}; //arr is an array that is accessed using subscripts //Calculate the number of array elements int sz = sizeof(arr) / sizeof(arr[0]); int i = 0; for(i = 0; i < sz; i++) { printf("%c", arr[i]); } return 0; }
4. Input and output of birth date
Original title link: Date of birth input / output_ Niuke Tiba_ Niuke network
[knocking on the blackboard]: pay attention to the input and output format.
Title Description:
Example:
Input: 20130225 Output: year=2013 month=02 date=25
Note:
Through the%m format control of scanf function, you can specify the input field width and the input data field width (number of columns), and intercept the required data according to this width; Through the% 0 format controller of printf function, specify the empty position not used on the left when outputting the value, and fill in 0 automatically.
Code execution:
#include<stdio.h> int main() { int year = 0; int month = 0; int date = 0; //Enter in format scanf("%4d%2d%2d", &year, &month, &date); //output printf("year=%4d\n", year); printf("month=%02d\n", month); printf("date=%02d\n", date); return 0; }
5.2 calculation to the nth power of
Original title link: Calculation to the nth power of 2_ Niuke Tiba_ Niuke network
Title Description:
If you want to learn more, you can take a look at my article:
Code execution:
#include<stdio.h> int main() { int n = 0; //Multi group input while(scanf("%d", &n)!=EOF) { printf("%d\n", 1<<n); } return 0; }
6. Input and exchange output according to format
Original title link: Input and exchange output in format_ Niuke Tiba_ Niuke network
Title Description:
Example:
Input: a=1,b=2 Output: a=2,b=1
Code execution:
#include<stdio.h> int main() { int a = 0; int b = 0; int c = 0; scanf("a=%d,b=%d", &a, &b); //exchange //Imagine a bottle of soy sauce and a bottle of vinegar, and then exchange an empty bottle c = a; a = b; b = c; //output printf("a=%d,b=%d\n", a, b); return 0; }
Add a abnormal written test question:
You cannot create a temporary variable (the third variable) to exchange two numbers:
Method 1: addition and subtraction
a = a + b;
b = a - b;
a = a - b;
This solves the problem, but there are some disadvantages. If a and B are large, it may lead to overflow, so another method is to use XOR
Method 2: XOR method
a = a ^ b;
b = a ^ b;
a = a ^ b;
In this way, the exchange is completed, but in practical application, it is customary to define the third variable to realize the exchange of two numbers, because the readability of the above code is not high!
7. Character to ASCII
Original title link: Character to ASCII_ Niuke Tiba_ Niuke network
Title Description:
Example:
Input: a Output: 97
Code execution:
#include<stdio.h> int main() { char ch = 0; ch = getchar(); printf("%d\n",ch); return 0; }
8. Calculate the value of the expression
Original title link: Evaluate the value of the expression_ Niuke Tiba_ Niuke network
Title Description:
Code execution:
#include<stdio.h> int main() { int a = 40; int c = 212; printf("%d\n", (-8+22)*a - 10 + c / 2); return 0; }
9. Calculate division with remainder
Original title link: Calculating division with remainder_ Niuke Tiba_ Niuke network
Title Description:
Example:
Input: 15 2 Output: 7 1
Code execution:
#include<stdio.h> int main() { int a = 0; int b = 0; scanf("%d %d", &a, &b); // /The division operator gets the quotient // %The remainder (modulo) operator gets the remainder int c = a/ b; int d = a % b; printf("%d %d\n", c, d); return 0; }
10. Calculate body mass index
Original title link: Calculate body mass index_ Niuke Tiba_ Niuke network
Title Description:
Example:
Input: 70 170 Output: 24.22
Code execution:
#include<stdio.h> int main() { int weight = 0; int height = 0; double bmi = 0.0; //input scanf("%d %d", &weight, &height); //Calculate BMI //The reason for dividing by 100.0 is that both sides of the division sign are integers. To get a decimal, you must ensure that one on both sides is a floating point number bmi = weight / ((height / 100.0) * (height / 100.0)); //output printf("%.2lf\n", bmi); return 0; }
11. Calculate the perimeter and area of the triangle
Original title link: Calculate the perimeter and area of the triangle_ Niuke Tiba_ Niuke network
Title Description:
Example:
Input: 3 Output: circumference=9.00 area=3.90
Note: given the three sides of the triangle, let's calculate the area of the triangle, then we need to use Helen's formula, and the problem will be solved easily.
Code execution:
#include<stdio.h> #include<math.h> int main() { double a = 0.0; double b = 0.0; double c = 0.0; double circumference = 0.0;//Perimeter double area = 0.0;//the measure of area //input scanf("%lf %lf %lf", &a,&b,&c); //calculation circumference = a + b + c; double p = (a + b + c) / 2; area = sqrt(p * (p - a) * (p - b) * (p - c)); printf("circumference=%.2lf area=%.2lf\n",circumference, area); return 0; }
12. Calculate the volume of the sphere
Original title link: Calculate the volume of the sphere_ Niuke Tiba_ Niuke network
Title Description:
Code execution:
#include<stdio.h> #include<math.h> #include<math.h> #define PI 3.1415926 int main() { double r = 0.0; double v = 0.0; //input scanf("%lf", &r); //calculation v = 4.0 / 3 * PI * pow(r, 3); //output printf("%.3lf\n", v); return 0; }
Conclusion: meet Enron meet you, not negative code, not negative Qing!
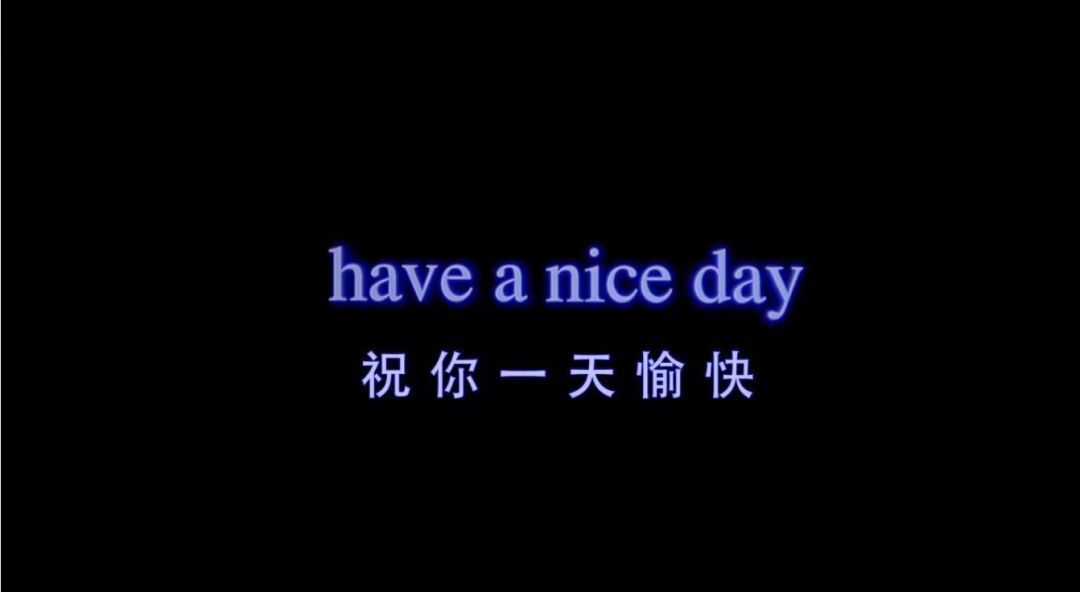