In this article:
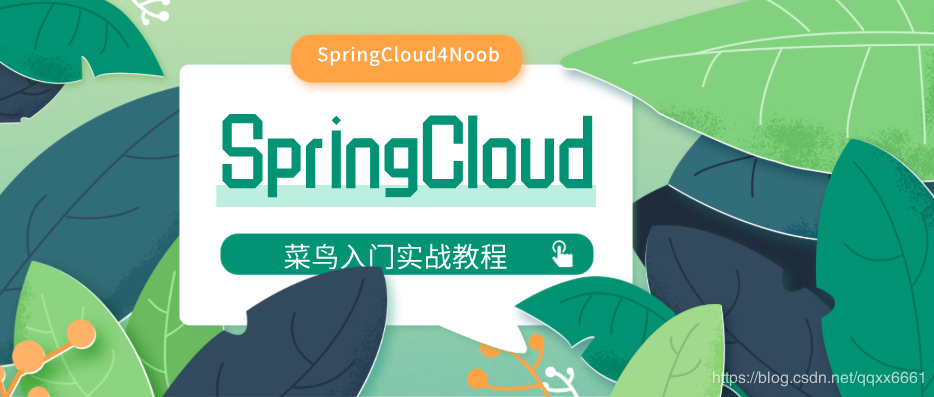
Preface
Welcome to the Starter Spring Cloud for Noob series, which learns and understands Spring Cloud step by step from a progressive practical perspective.
This series is suitable for students with a certain Java and SpringBook foundation.
At the end of each article, the corresponding Github source code is attached for students to debug.
Github Warehouse Address:
https://github.com/qqxx6661/springcloud_for_noob
Starter Spring Cloud Practical Series
You can also view the Starter Spring Cloud Series in the following two ways:
- Pay attention to my public number: Rude3Knife click below the public number: Technology Twitter - Spring Cloud
- Rookie Spring Cloud Practical Column (CSDN)
Actual version
- SpringBoot: 2.0.3.RELEASE
- SpringCloud: Finchley.RELEASE
------ The text begins------------------------------------------------------------------------------------------------------
Constructing multi-module Maven project + creating registry Eureka sub-module
Building a multi-module Maven project
Because there are many components in Spring Cloud, each component needs to be developed and maintained independently. In order to facilitate uniform version maintenance and management, we often use Maven's multi-module mode.
The structure is as follows:
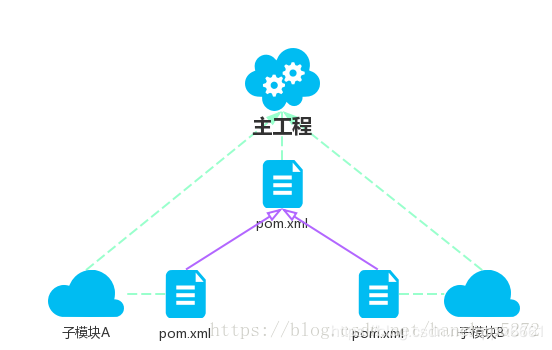
Creating Main Project
First, create a Maven project as the main project, the type does not matter. Here we recommend the use of the maven-archetype-quickstart skeleton, the creation process is as follows:
File–>New–>Project –>Maven–>Create from archetype–>maven-archetype-quickstart-Next –>GroupId={Your GroupId}–>AritifactId={Your ArtifactId} –>Next–>Next–>Finish–>New Whindow
Create submodules
New module in new-module:
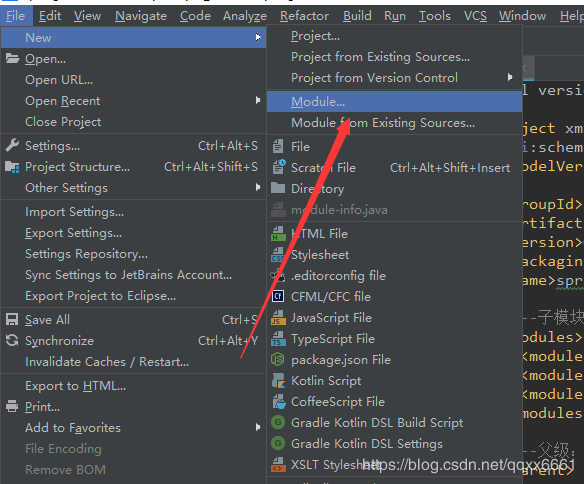
The next step along the way is:
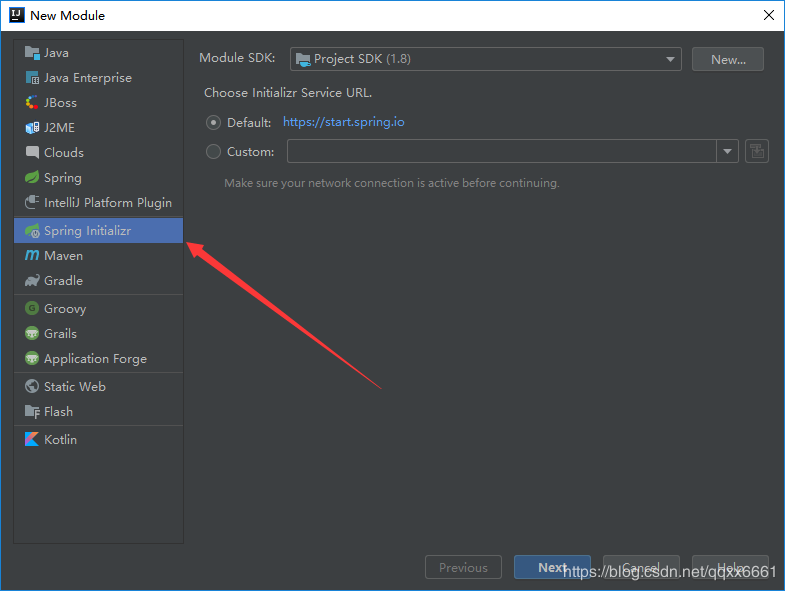
Here we create a new sub-module called eureka, which is used to put the Eureka module into use. After the new sub-module is built, the directory of the sub-module is as follows:
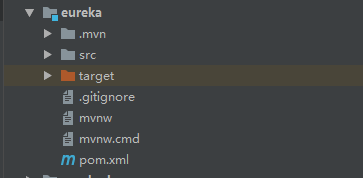
Delete more main projects than directories
Since no code development is required in the main project, the src directory is deleted.
You can see our directory structure now:
Editing the main project pom.xml
What is done in the pom.xml of the main project:
- Configure Springboot and SpringCloud infrastructure components
- Configuration sub-module: You can see that there is now a sub-module `eureka', and new sub-modules need to be added here after they are created.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.pricemonitor</groupId>
<artifactId>springcloud</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>pom</packaging>
<name>springcloud</name>
<! - Submodule - >
<modules>
<module>eureka</module>
</modules>
<! - Parent: Spring Boot ->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.3.RELEASE</version>
</parent>
<! - Parameters - >
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<! - Spring Cloud Version Sequence Configuration ->
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-dependencies</artifactId>
<version>Finchley.RELEASE</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<! - Spring Boot Actuator Component -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<!-Spring Cloud Foundation-
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter</artifactId>
</dependency>
<!-Spring Cloud Service Registration Component-
<dependency>
<groupId>org.springframework.cloud</groupId>
<! - The dependencies here are dedicated after SpringBoot 2.0. If you use SpringBoot version less than 2.0, use spring-cloud-starter-eureka-server ->.
<artifactId>spring-cloud-starter-netflix-eureka-server</artifactId>
</dependency>
<! - Spring Boot Web Component -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<! --- Spring Book Test Component --> ___________
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Create registry Eureka sub-module
Introduction to Eureka
Service center, also known as registry, manages various service functions, including service registration, discovery, fuse, load, downgrade, etc., such as dubbo admin background functions.
Spring Cloud encapsulates the Eureka module developed by Netflix to implement service registration and discovery. Eureka uses the C-S design architecture. Eureka Server serves as a service registration function, and it is a service registry. Other micro services in the system use Eureka clients to connect to Eureka Server and maintain heartbeat connections. In this way, the maintainer of the system can monitor the normal operation of each micro-service through Eureka Server. Some other modules of Spring Cloud, such as Zuul, can use Eureka Server to discover other micro services in the system and execute related logic.
Eureka consists of two components: the Eureka server and the Eureka client. The Eureka server serves as a service registration server. The Eureka client is a java client that simplifies interaction with the server and acts as polling load balancing It also provides service failover support. Netflix uses another client in its production environment, which provides weighted load balancing based on traffic, resource utilization, and error status.
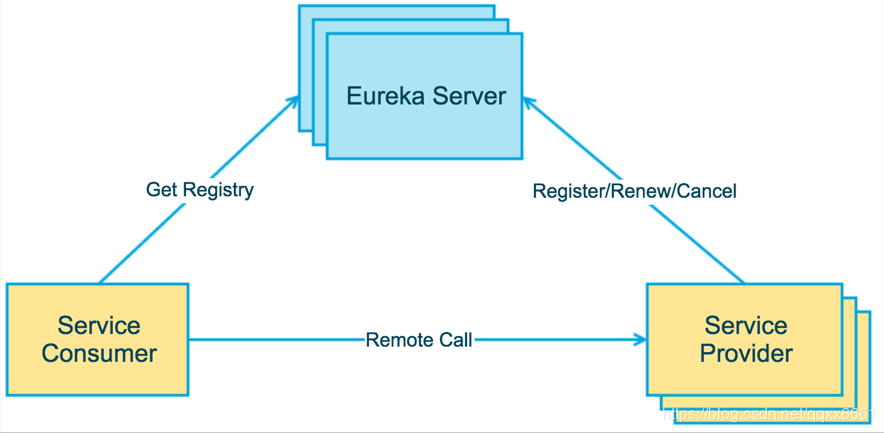
The figure above briefly describes the basic architecture of Eureka, which consists of three roles:
1,Eureka Server
- Providing service registration and discovery
2,Service Provider
- service provider
- Register your own services in Eureka so that service consumers can find them
3,Service Consumer
- Service consumer
- Get a list of registered services from Eureka so that services can be consumed
Editing sub-module Eureka's pom.xml
Next, we create a Spring Cloud registration center Eureka in the sub-module Eureka.
Code emphasis:
- Inherited the parent pom.xml
- As you can see, there is no new dependency introduced here, so how to introduce Eureka component dependency? Back to the main pom.xml above, you will find that I introduced spring-cloud-starter-netflix-eureka-server in it, because in most of the later modules, we will use Eureka-server, so we put it in the main. POM for sharing with other sub-modules
- Submodules can be packaged to run jar: <packaging>jar</packaging>
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion><span class="token operator"><</span>artifactId<span class="token operator">></span>eureka<span class="token operator"><</span><span class="token operator">/</span>artifactId<span class="token operator">></span> <span class="token operator"><</span>version<span class="token operator">></span><span class="token number">0.0</span><span class="token punctuation">.</span><span class="token number">1</span><span class="token operator">-</span>SNAPSHOT<span class="token operator"><</span><span class="token operator">/</span>version<span class="token operator">></span> <span class="token operator"><</span>name<span class="token operator">></span>eureka<span class="token operator"><</span><span class="token operator">/</span>name<span class="token operator">></span> <span class="token operator"><</span>packaging<span class="token operator">></span>jar<span class="token operator"><</span><span class="token operator">/</span>packaging<span class="token operator">></span> <span class="token operator"><</span>description<span class="token operator">></span>Demo project <span class="token keyword">for</span> Spring Boot<span class="token operator"><</span><span class="token operator">/</span>description<span class="token operator">></span> <span class="token operator"><</span><span class="token operator">!</span><span class="token operator">--</span>Dependence on Parent Engineering<span class="token operator">--</span><span class="token operator">></span> <span class="token operator"><</span>parent<span class="token operator">></span> <span class="token operator"><</span>groupId<span class="token operator">></span>com<span class="token punctuation">.</span>pricemonitor<span class="token operator"><</span><span class="token operator">/</span>groupId<span class="token operator">></span> <span class="token operator"><</span>artifactId<span class="token operator">></span>springcloud<span class="token operator"><</span><span class="token operator">/</span>artifactId<span class="token operator">></span> <span class="token operator"><</span>version<span class="token operator">></span><span class="token number">0.0</span><span class="token punctuation">.</span><span class="token number">1</span><span class="token operator">-</span>SNAPSHOT<span class="token operator"><</span><span class="token operator">/</span>version<span class="token operator">></span> <span class="token operator"><</span><span class="token operator">/</span>parent<span class="token operator">></span> <span class="token operator"><</span>dependencies<span class="token operator">></span> <span class="token operator"><</span><span class="token operator">/</span>dependencies<span class="token operator">></span>
</project>
Add the @EnableEurekaServer annotation to the startup code
EurekaApplication.java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.server.EnableEurekaServer;
@SpringBootApplication
@EnableEurekaServer
public class EurekaApplication {
<span class="token keyword">public</span> <span class="token keyword">static</span> <span class="token keyword">void</span> <span class="token function">main</span><span class="token punctuation">(</span>String<span class="token punctuation">[</span><span class="token punctuation">]</span> args<span class="token punctuation">)</span> <span class="token punctuation">{</span> SpringApplication<span class="token punctuation">.</span><span class="token function">run</span><span class="token punctuation">(</span>EurekaApplication<span class="token punctuation">.</span><span class="token keyword">class</span><span class="token punctuation">,</span> args<span class="token punctuation">)</span><span class="token punctuation">;</span> <span class="token punctuation">}</span>
}
If you find that @Enable Eureka Server cannot be introduced, check that your Spring Cloud version is compatible with Spring Book version:
springboot cannot introduce @enableeurekaserver and Spring Cloud matching with Spring Boot versions:
https://blog.csdn.net/zhang53141/article/details/83091032
configuration file
The default generated configuration file is application.properties, which can also be written in application.yml format. The configuration is as follows:
# Port number server: port: 8761 # Service registration related configuration eureka: # Service instance hostname instance: hostname: localhost # Service Provider Configuration client: # No registration (configuration when the service registry is single rather than highly available) registerWithEureka: false # Not getting registration information (configuration when the service registry is single rather than highly available) fetchRegistry: false # Service Registry Address serviceUrl: defaultZone: http://${eureka.instance.hostname}:${server.port}/eureka/
Be careful:
- RegiserWithEureka: false indicates that the current service registry does not register in other service registries.
- fetchRegistry: false indicates that the current service registry does not obtain registration information from other service registries.
- The above two configurations are configurable only when the service registry is a single point configuration, because these two values are necessarily false in a single point.
Function
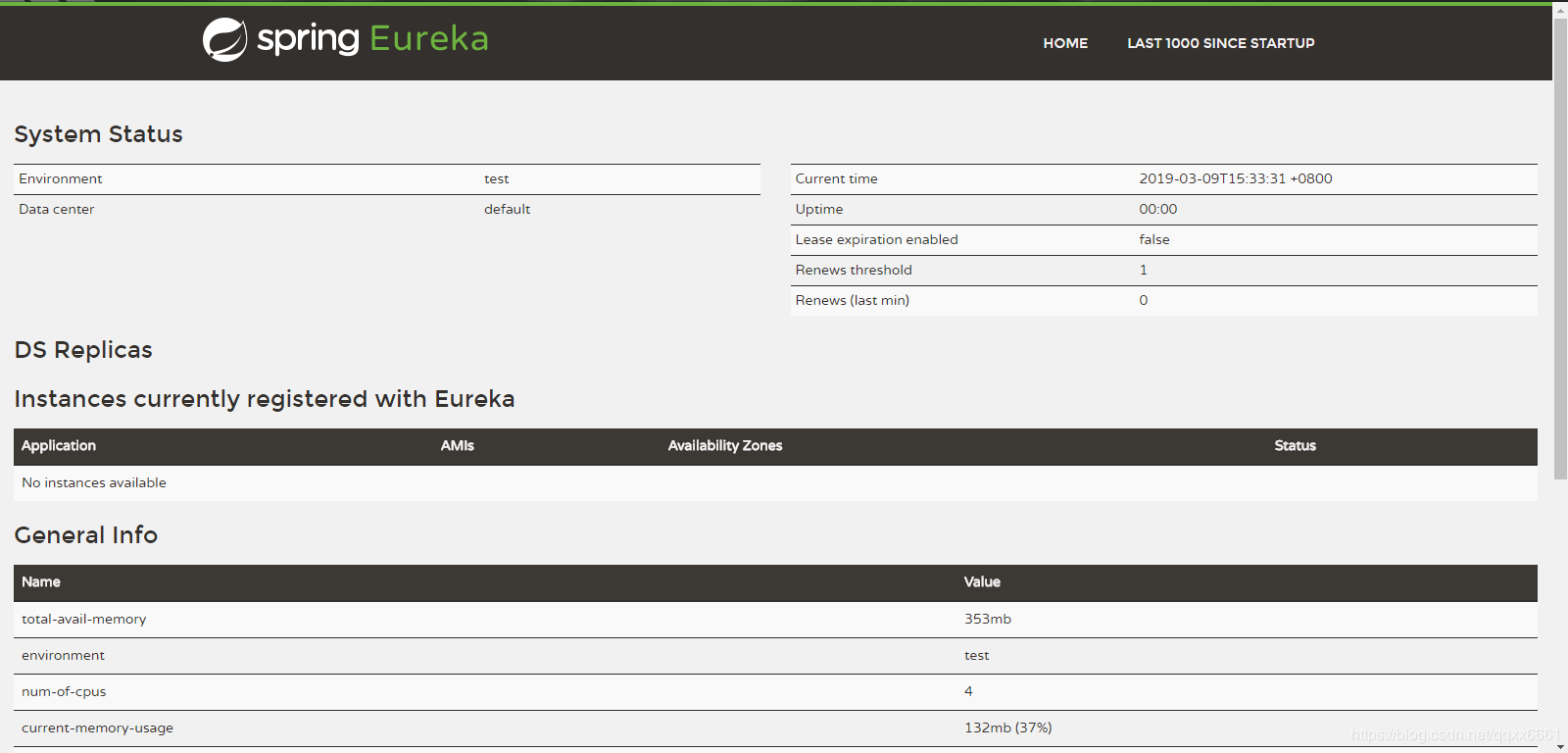
You can see the following page, which hasn't found any services yet. It's just a lone eureka-server.
In the next tutorial, we will create a service provider and register in Eureka.
The corresponding engineering code for this tutorial
https://github.com/qqxx6661/springcloud_for_noob/tree/master/01-eureka