1. overview
This article explains how to use Maven to build Spring Security applications. Specific use cases using Spring Security dependencies will be discussed. The latest version of Spring Security can be found on Maven Central.
This is a follow-up to the previous Spring and Maven articles, so for non-secure Spring dependencies, this is where you start.
2. Maven and Spring Security
2.1. spring-security-core
Core Spring Security supports - spring-security-core - including authentication and access control functions. It also supports stand-alone (non-Web) applications, method-level security and JDBC:
<properties> <spring-security.version>5.0.6.RELEASE</spring-security.version> <spring.version>5.0.6.RELEASE</spring.version> </properties> <dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-core</artifactId> <version>${spring-security.version}</version> </dependency>
Note that Spring and Spring Security are in different release plans, so version numbers do not always match 1:1.
If you're using the old version of Spring - it's important to understand that, fortunately, Spring Security 3.1.x does not depend on Spring 3.1.x! This is because Spring Security 3.1.x was released before Spring 3.1. The plan is to align these dependencies more closely in future releases.
2.2. spring-security-web
To add Web support to Spring Security, you need spring-security-web dependencies
<dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-web</artifactId> <version>${spring-security.version}</version> </dependency>
It contains filters and associated Web security infrastructure that enables URL access control in a Servlet environment.
2.3. Spring Security and Older Spring Core Dependency Problems
This new dependency also presents a problem with the Maven dependency graph. As mentioned above, Spring Security jar does not depend on the latest Spring Core jar (but in previous versions). This may lead to these older dependencies taking advantage of the classpath rather than the updated 4.x Spring component.
To understand why this happens, we need to see how Maven can solve these problems. If version conflicts occur, Maven will choose the jar closest to the root. In our example, spring-core is defined by spring-orm (using version 4.x.RELEASE), but also by spring-security-core (using the old version 3.2.8.RELEASE), so in both cases, spring-jdbc is defined at a depth of 1 from the root pom of our project.
In fact, it's important to define the order of spring-orm and spring-security-core in our own pom.
The first one will take precedence, so we may eventually get any version on our classpath
To solve this problem, we must define some Spring dependencies explicitly in our pom, independent of the implicit Maven dependency parsing mechanism. Doing so places a particular dependency at the depth of 0 of our POM (because it is defined in the POM itself), so it will take precedence. All of the following belong to the same category and need to be defined directly or explicitly for multi-module projects, in the dependency management element of the parent.
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-jdbc</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-aop</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-tx</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-expression</artifactId> <version>${spring-version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-web</artifactId> <version>${spring-version}</version> </dependency>
2.4. spring-security-config and others
To use rich Spring Security XML namespaces, spring-security-config dependencies are required:
<dependency> <groupId>org.springframework.security</groupId> <artifactId>spring-security-config</artifactId> <version>${spring-security.version}</version> <scope>runtime</scope> </dependency>
No application code should be compiled for this dependency, so it should be considered a runtime scope.
Finally, LDAP, ACL, CAS and OpenID support have their own dependencies in Spring Security.
spring-security-ldap, spring-security-acl, spring-security-cas and spring-security-openid.
3. Use snapshots and milestones
Spring provides Spring Security milestones and snapshots in the custom Maven repository provided by Spring
For additional details on how to configure these contents, see How to Use snapshots and milestones.
4. conclusion
This article discusses the practical details of using Spring Security and Maven. The Maven dependencies introduced here are, of course, some of the major ones. There are a few other things worth mentioning, but here are some deletions, but this should be a good starting point for using Spring in Maven-enabled projects.
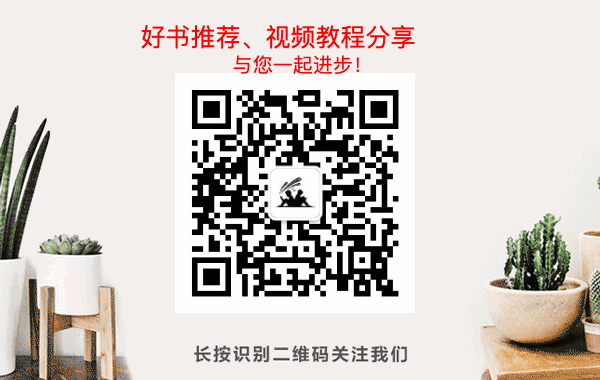