There are many built-in methods of data types. You can view the built-in methods of data types through the built-in reminder of programming software. In python, it is viewed through the period character
Built in methods for shaping and floating point data
Type conversion
type conversion refers to the method of converting other different types of data into other types of data. Here, it refers to converting other types of data (only pure digital data) into integer with int keyword.
info = input('age is:') # The contents obtained by input will be converted into string type print(info, type(info)) # Data type of output info print(int(info), type(int(info))) # Data type of output int (info)
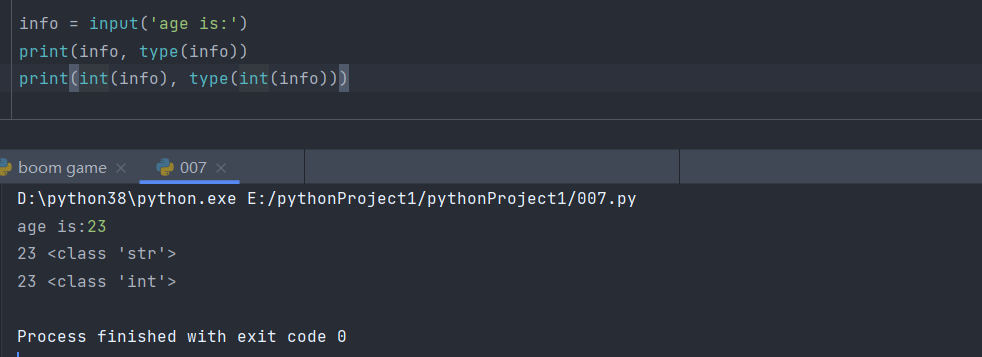
Binary conversion
base conversion is to convert the data of a base number into another data type. int can convert the digital data of other base numbers into decimal
print(bin(444)) # Convert decimal 444 to binary 0b110111100 print(oct(444)) # Convert decimal 444 to octal 0o674 print(hex(444)) # Convert decimal 444 to hexadecimal 0x1bc print(int(0b110111100)) # Convert binary 444 to decimal print(int(0o674)) # Convert octal 444 to decimal print(int(0x1bc)) # Convert hexadecimal 444 to decimal
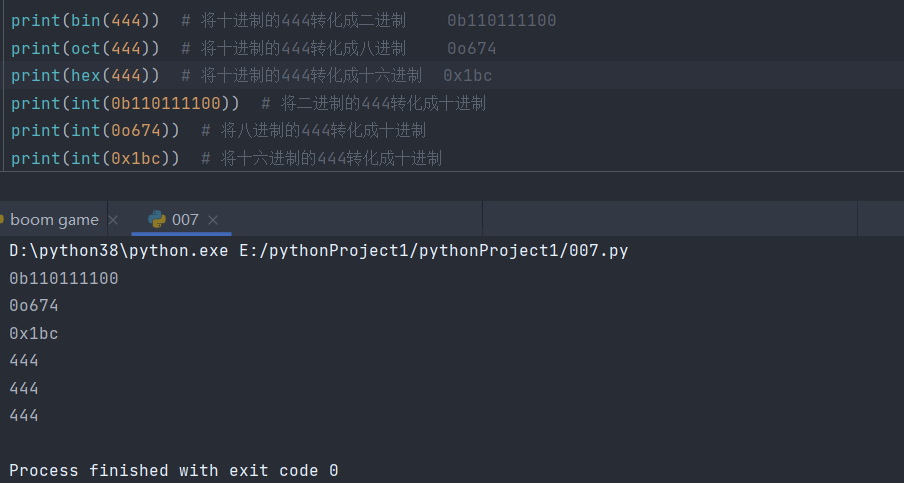
float
Type conversion
type conversion refers to the method of converting other different types of data into other types of data. Here, it refers to converting other types of data (only digital data) into floating-point type with float keyword.
info = input('age is:') # The contents obtained by input will be converted into string type print(info, type(info)) # Data type of output info print(float(info), type(float(info))) # Data type of output float (info)
integer
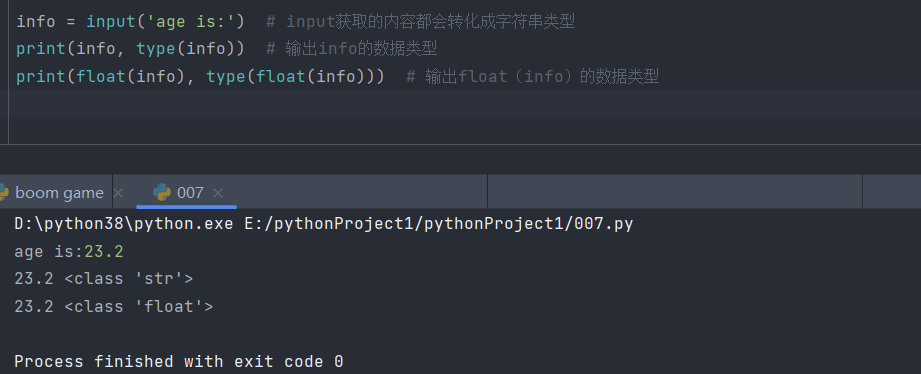
decimal
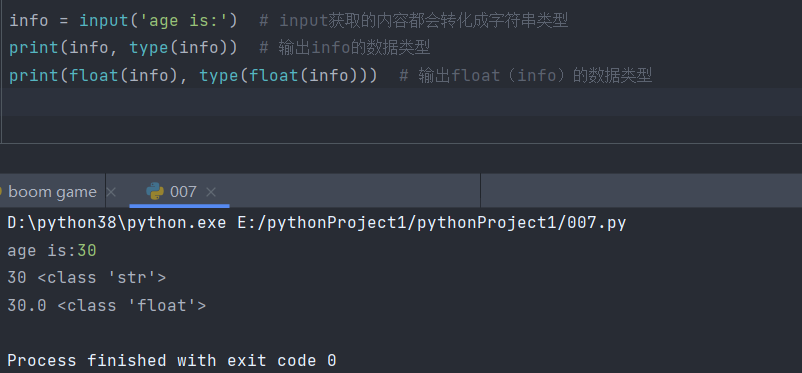
Built in method of string
Type conversion
refers to converting any other type of data into a string with the str keyword.
print(str(23), type(str(23))) # Shaping conversion print(str(23.38), type(str(23.38))) # Floating point conversion print(str([1, 2, 3, 4]), type(str([1, 2, 3, 4]))) # List conversion print(str({'hobby': 'sport'}), type(str({'hobby': 'sport'}))) # Dictionary conversion print(str((1, 2, 3, 4)), type(str((1, 2, 3, 4)))) # tuple print(str({1, 2, 3, 4}), type(str({1, 2, 3, 4}))) # aggregate print(str(False), type(str(False))) # Boolean value
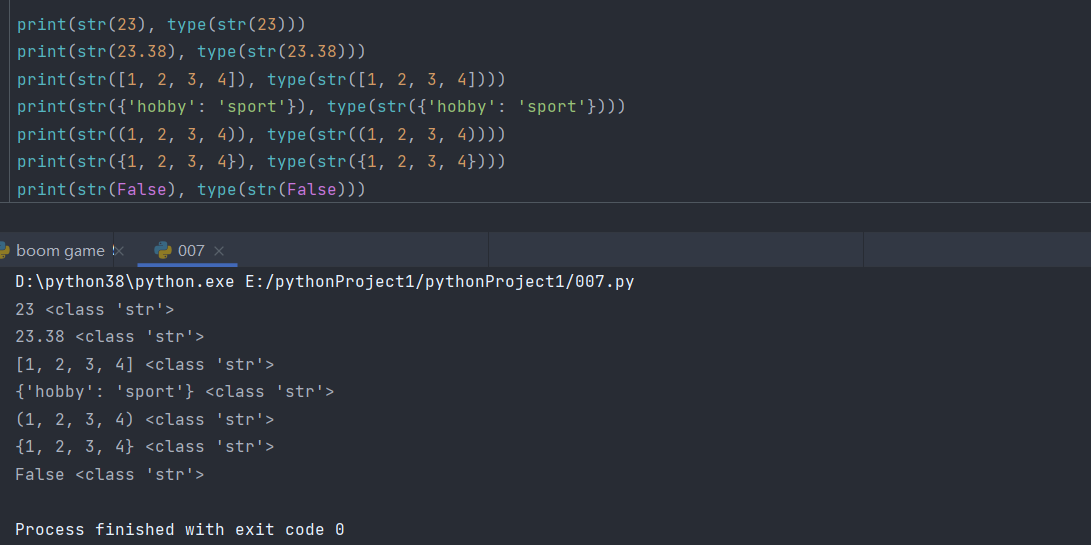
Other methods
str_name= 'oliver chance'
- Index value: similar to the list index value, from left to right, starting from 0 to extract the characters of the string
print(str_name[0]) - Slicing operation: extract all characters between two numbers (closed on the left and open on the right) according to the index value
print(str_name[2:4]) - Step size: measure the number of characters in the string. Note that spaces are also characters
print(str_name[2:9:1]) # the third parameter is the step size, which is 1 by default
print(str_name[2:9:2]) # interval one by one
Index value slice expansion
- Get the following characters: from right to left, decrease from - 1, and extract the string
print(str_name[-1]) - Control the direction of the index: get the last four strings in turn from right to left, with the start bit instead of the end bit
print(str_name[-1:-5:-1])
- Count the number of characters in the string, using the len keyword
print(len(str_name)) - Member operation
print('li' in str_name)
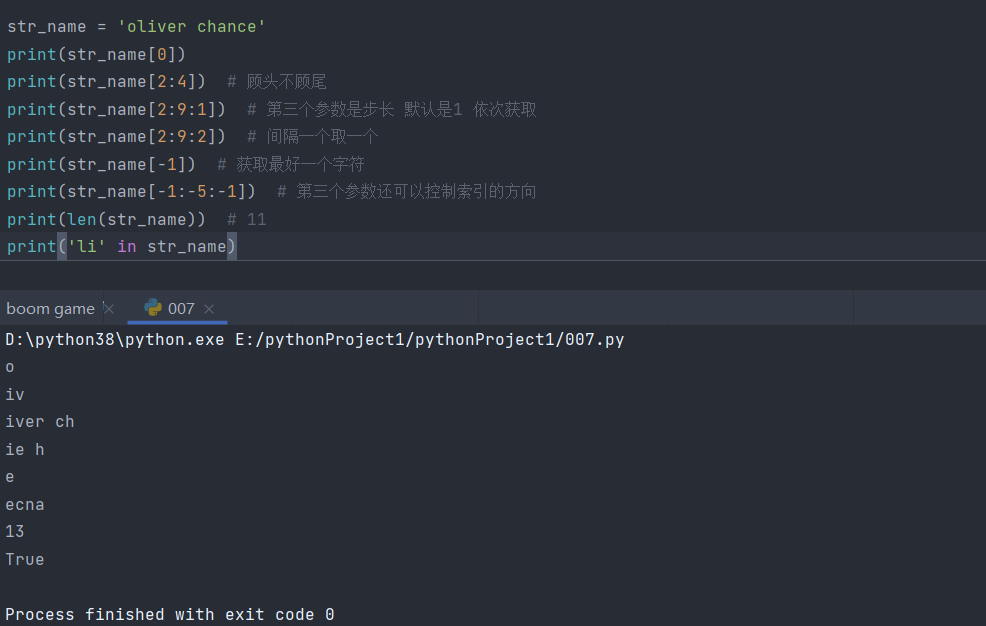
- Removes the specified character at the beginning of the string
name = ' oliver chance ' print(len(name)) res = name.strip() # By default, spaces at the beginning and end of a string are removed print(res, len(res)) name1 = '####oliver####' print(name1.strip('#')) # Removes the character specified at the beginning and end of the string
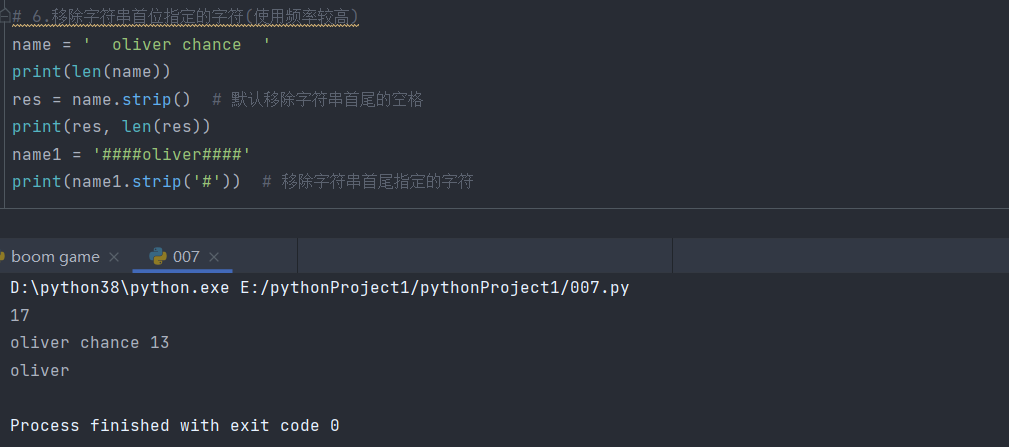
- Cut the string according to the specified characters, and the cutting results generate a list
data = 'oliver,23,fight' print(data.split(',')) name, pwd, hobby = data.split(',') # Decompression assignment print(data.split(',', maxsplit=1)) # Cut the first one and leave the rest uncut print(data.rsplit(',', maxsplit=1)) # The last one left will not be cut, and the rest will be cut completely

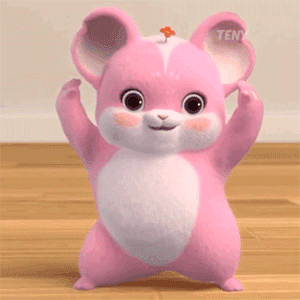