Teach yourself Python and record things that need special attention
Unit1 start
1.1 setting up programming environment
1.3 solving installation problems
1.4 running Python program from terminal
Method, just enter python
1.5 summary
Unit2 variables and simple data types
2.1 run hello_word.py
2.2 variables
2.2.1 naming and use of variables
1.2 build Python programming environment in different operating systems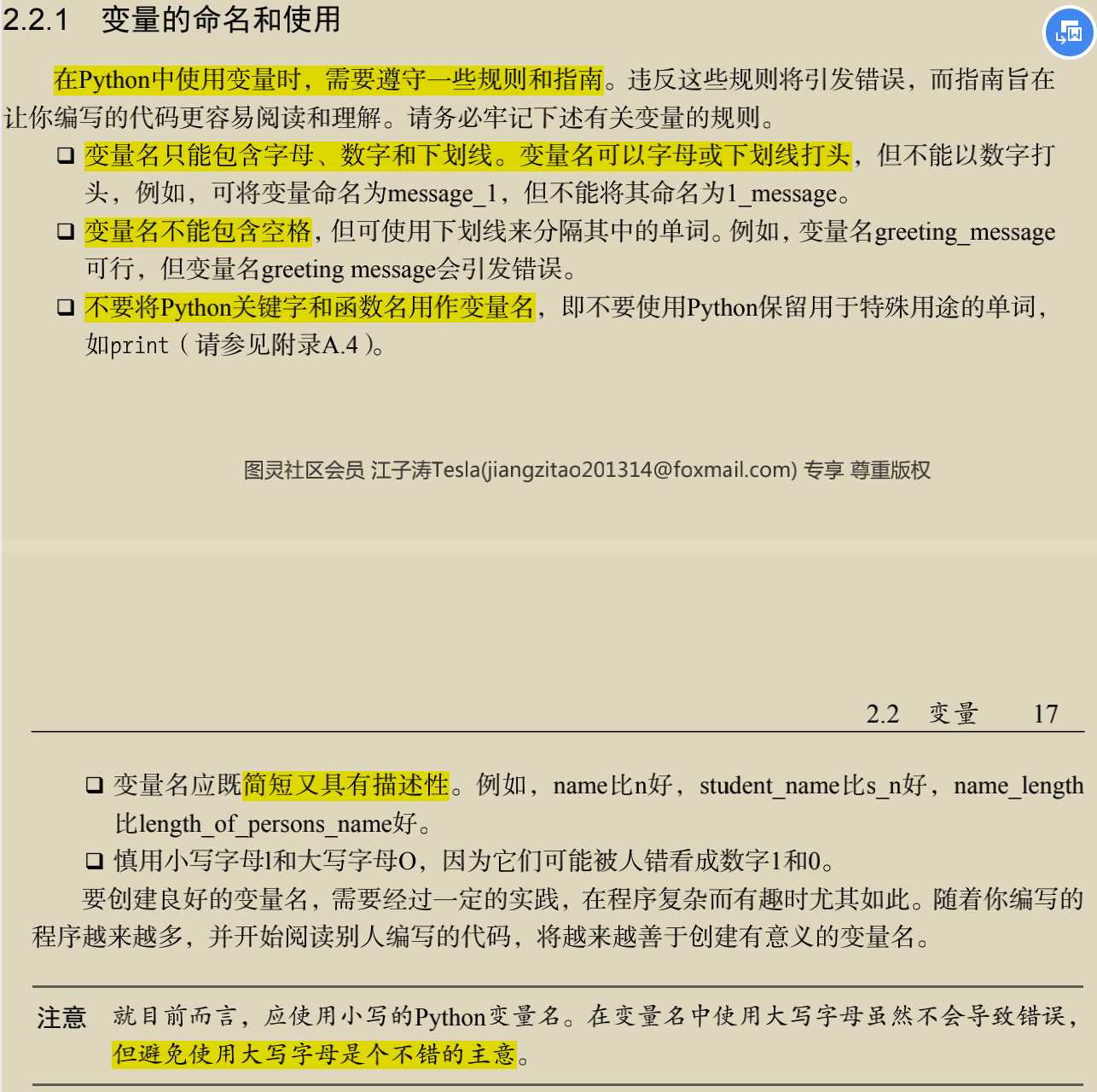
2.2.2 avoid naming errors when using variables
2.3 string
2.3.1 use the method to modify the case of the string
title() function
name = "ada lovelace" print(name.title())
Ada Lovelace
upper() and lower() functions
name = "Ada Lovelace" print(name.upper()) print(name.lower())
ADA LOVELACE
ada lovelace
2.3.2 merge (splice) strings
Direct use+
first_name = "ada" last_name = "lovelace" full_name = first_name + " " + last_name print(full_name)
ada lovelace
2.3.3 use tabs or line breaks to add white space
\t \n
2.3.4 delete blank
Temporarily delete blank
[1]>>> favorite_language = 'python ' [2]>>> favorite_language 'python ' [3]>>> favorite_language.rstrip() 'python' [4]>>> favorite_language 'python '
To permanently delete the whitespace in this string, you must save the result of the deletion operation back to the variable:
>>> favorite_language = 'python ' >>> favorite_language = favorite_language.rstrip() >>> favorite_language 'python'
Delete blank space elsewhere
You can also eliminate the whitespace at the beginning of the string or at both ends of the string. For this purpose, methods can be used separately
lstrip() and strip():
>>> favorite_language = ' python ' >>>> favorite_language.rstrip() ' python' >>> favorite_language.lstrip() 'python ' >>> favorite_language.strip() 'python'
2.3.5 avoid syntax errors when using strings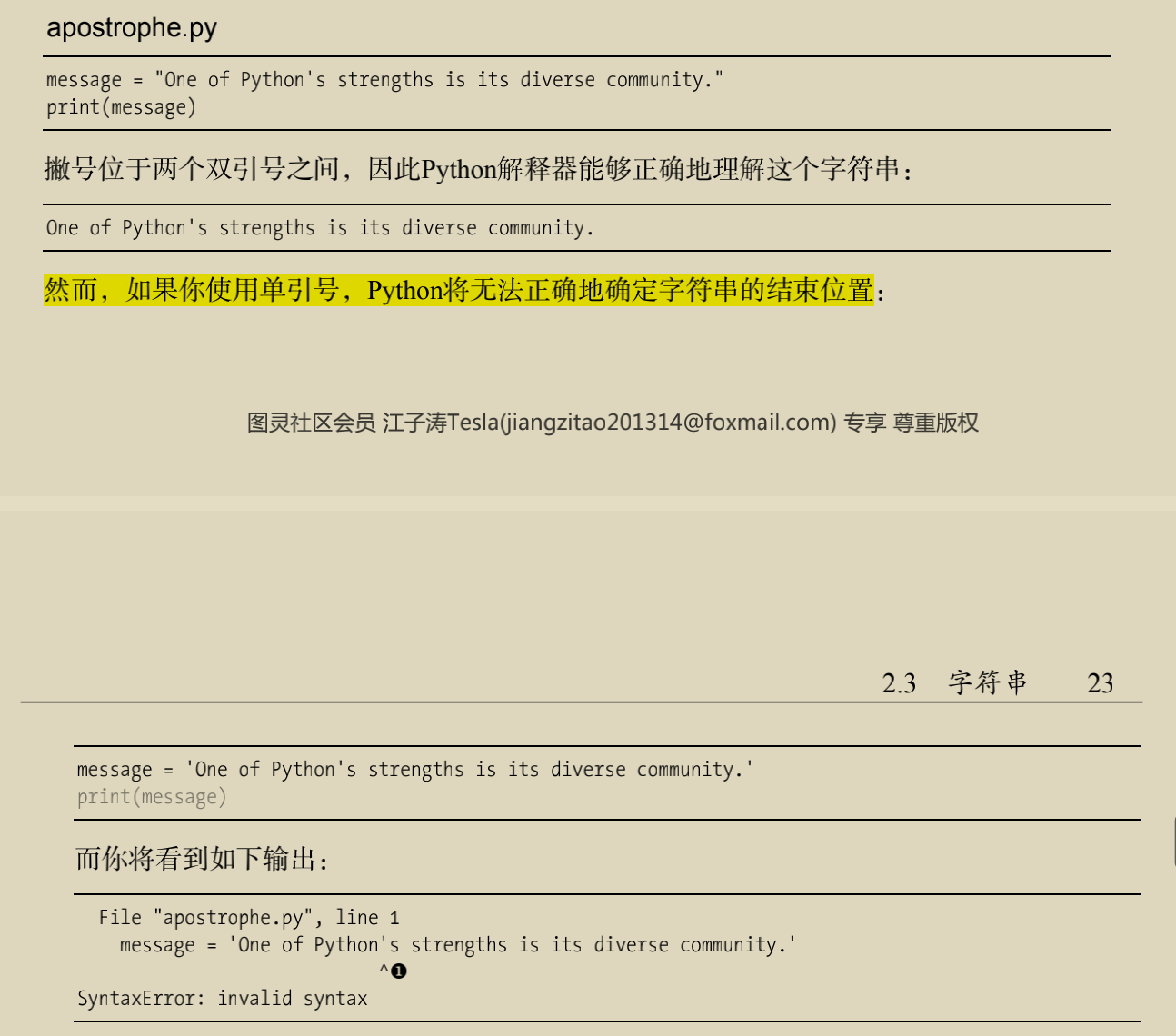
2.3.6 print statement in Python 2
There are no parentheses
>>> python2.7 >>> print "Hello Python 2.7 world!" Hello Python 2.7 world!
2.4 figures
2.4.1 integer
In Python, you can add (+) subtract (-) multiply (*) divide (/) integers.
Python uses two multiplier signs to represent a power operation:
>>> 3 ** 2 9 >>> 3 ** 3 27 >>> 10 ** 6 1000000
2.4.2 floating point number
Floating point numbers are numbers with decimal points
2.4.3 using the function str() to avoid type errors
age = 23 message = "Happy " + age + "rd Birthday!"
In this way, mixed types will report errors
age = 23 message = "Happy " + str(age) + "rd Birthday!"
Call the str() function to convert a non character type to a character type
2.4.4 integer in Python 2
>>> python2.7 >>> 3 / 2 1
In Python 2, the result of integer division only contains integer part and decimal part
The score was deleted. Please note that when calculating the integer result, the method is not rounding, but deleting the decimal part directly
>>> 3 / 2.0 1.5 >>> 3.0 / 2.0 1.5
2.5 notes
2.5.1 how to write notes
# Say hello to everyone print("Hello Python people!")
2.5.2 what kind of notes should be prepared
2.6 Zen of Python
Introduction to Unit3 list
3.1 what is the list
A list consists of a series of elements arranged in a specific order
In Python, lists are represented by square brackets ([]) and elements are separated by commas. Here is a simple example
List example, this list contains several bicycles:
bicycles = ['trek', 'cannondale', 'redline', 'specialized'] print(bicycles)
Output results
['trek', 'cannondale', 'redline', 'specialized']
3.1.1 accessing list elements
print(bicycles[0])
Output results
trek
3.1.2 index starts from 0 instead of 1
-1 can be used to represent the last element
Python provides a special syntax for accessing the last list element. By specifying an index of - 1, python returns the last list element:
print(bicycles[-1])
You can also return the penultimate, third, fourth, and so on
3.1.3 use the values in the list
Direct drinking, such as num[0]
3.2 modify, add and delete elements
3.2.1 modifying list elements
Direct call to change
motorcycles[0] = 'ducati'
3.2.2 adding elements from the list
Call the append() function to add to the end
motorcycles.append('ducati')
Call the insert() function to insert into the specified location
motorcycles.insert(0, 'ducati')
ducati is placed in position 0
3.2.3 removing elements from the list
- Delete with del statement
del motorcycles[0]
- The pop() function deletes the last element and returns it
- You can also pop(0) or pop(1)
popped_motorcycle = motorcycles.pop()
- Delete elements based on their values. Use remove()
motorcycles.remove('ducati')
3.3 organization list
3.3.1 sort() permanently sorts the list
- sort()
- sort(reverse=True)
3.3.2 sorted() temporarily sorts the list
At this time, cars does not change, but outputs the sorted values
print(sorted(cars))
3.3.3 print list upside down
print(cars) cars.reverse() print(cars)
3.3.4 determining the length of the list
len(cars)
3.4 avoid index errors when using lists
For example, if there are only three elements, the index of the last element is 2. If you reference 3, you will make an error