After the interactive interface design is completed, it will be followed by the writing of control functions and other functions,
The first thing to do is to write the functions of each control of the calculator. These functions can be generated automatically by double clicking the control directly in Visual Studio. This is very convenient and can greatly save our time in function development and programming.
Then we need to define some variables to receive the content sent when the corresponding control is triggered. These variables can receive numbers, operators, decimal points, results and other information when the calculator is used:
private string strOutput = ""; //Define output private double INumFormer = 0; //Defines the first number of inputs private double INumTemp = 0; //Define the second number of inputs private double IResult = 0; //Define results private string cOperation; //Define operator private bool DotCliked = false; //Define boolean type to judge decimal point double lastPrecisionNum = 1; //Defines the precision of the last decimal place as 1
=======================================================================
This function is used to clear all the entered contents and return to the initial interactive interface to facilitate the user's secondary input. The emptying function needs to be written under the function associated with the control. The idea of writing this function is to directly set all the previously defined receiving variables to the initial value. The specific codes are as follows:
//Empty button trigger function private void button_ce_Click(object sender, EventArgs e) { //Numeric variable emptying INumFormer = 0; INumTemp = 0; IResult = 0; strOutput = ""; cOperation = ""; DotCliked = false; lastPrecisionNum = 1; txtOutPut.Text = "Why is the screen clear again? Can't you count?"; }
Decimal point button click function
============================================================================
The decimal point button click function is used to distinguish between integer data and floating-point data after clicking the decimal point when the user performs double data operation. The writing idea is to add a decimal point after the string of the output content, and then display it in the text box. Since we first set the initial value of the DotCliked variable that determines the decimal point to false, after processing, we should set the value of DotCliked to true to remind the system to perform decimal operation at this time. The specific function code is as follows:
//Decimal point button click function private void button_dot_Click(object sender, EventArgs e) { if (!DotCliked) { strOutput += "."; txtOutPut.Text = strOutput; DotCliked = true; } }
Centralized processing button trigger event function
===============================================================================
This function is a function defined by ourselves, not a function type automatically generated by a control. The function receives the information triggered when the user clicks the button and judges the decimal. If the value entered by the user is a decimal, Then move the input data (click the data input after the decimal point) one digit to represent the value after the decimal point, and add the INumTemp data to get the input decimal. If it is not a decimal, it is worth noting that the previously input data should be multiplied by 10 and moved forward one digit,
At the same time, I give you a little suggestion. In order to judge whether the data overflows, you can add an exception throw judgment when judging the statement, so that when an error exception occurs, the program will not be unable to run, but an error exception reminder will be issued, so that you can easily know the problem of the program.
This is also a habit in many programming development. Often setting exception throwing can avoid program errors and failure to run.
The function implementation code is as follows:
//Centralized processing of button trigger events private void Numbers_Click(object sender, EventArgs e) { string strClickNum = ((Button)sender).Text; //Get button control text try { //Judge if you click decimal type if (DotCliked) { lastPrecisionNum *= 0.1; //Get the decimal value and judge whether there is abnormal overflow checked { INumTemp = INumTemp + long.Parse(strClickNum) * lastPrecisionNum; //Convert the obtained button string type to long type } } else { checked { INumTemp = INumTemp * 10 + long.Parse(strClickNum); } } txtOutPut.Text += INumTemp.ToString(); strOutput += strClickNum; txtOutPut.Text = strOutput; } catch (Exception) { MessageBox.Show("data overflow "); } }
Operator button click event function
==============================================================================
Like the button function for centralized processing of trigger events, this function is also a function defined by ourselves. The function is used to receive operations when controls such as addition, subtraction, multiplication and division are typed. The writing idea of this function is that when an operator is entered, it indicates that the previous data has been entered.
At this time, we need to assign the value of INumTemp to INumFormer to indicate that this is the first data we type, and then assign the value of INumTemp to 0 to facilitate the input of the second value. At the same time, we need to continue to increase the variables that define the output on the original basis, and output the information at this time through the text output box.
After that, the decimal point judgment variable and decimal number variable are assigned as initial values to facilitate the input of two data. The specific function implementation code is as follows:
//Operator button click event private void Opraters_Click(object sender, EventArgs e) { string strClickop = ((Button)sender).Text; //Get button value cOperation = strClickop; INumFormer = INumTemp; INumTemp = 0; strOutput += cOperation.ToString(); txtOutPut.Text = strOutput; DotCliked = false; lastPrecisionNum = 1; }
The equal sign button triggers the event function
=============================================================================
As the name suggests, the function is used to output the typed results. The function is automatically generated by double clicking the equal sign control. We don't need to define it ourselves. When we click the equal sign button, the function will be triggered.
The writing idea of the equal sign button trigger function is: use the switch statement to judge the type of operator you type, and then compare the numerical values INumFormer and INumTemp you type for the first and second time according to different operators you type; Perform the corresponding addition, subtraction, multiplication and division, assign the result to IResult, and output the operation result through the text output box.
There are two operation modes:
One is to re-enter two numbers for operation. After the result is output, all the variables originally defined can be given initial values,
The other is to continue to click the operator for serial operation after obtaining the operation result. After outputting the operation result, assign the operation result to INumFormer and INumTemp to store the operation result and continue the operation. After that, all variables previously defined are given initial values.
The specific implementation code of the equal sign button trigger function is as follows:
//Equal sign button trigger function private void button_enter_Click(object sender, EventArgs e) { try { switch (cOperation) { case "+": { checked //chexked keyword checks whether an exception overflow occurs { IResult = INumFormer + INumTemp; } break; } case "-": { checked //chexked keyword checks whether an exception overflow occurs { IResult = INumFormer - INumTemp; } break; } case "*": { checked //chexked keyword checks whether an exception overflow occurs { IResult = INumFormer * INumTemp; } break; } case "/": { checked //chexked keyword checks whether an exception overflow occurs { # Write at the end As a programmer about to apply for a job, where will your employment opportunities and outlets appear in 2019, which may be very different from recent years? In this new environment, should I choose a large factory or a small company? How should veterans with several years of work experience maintain and enhance their competitiveness and turn passive into active? **In terms of the current environment, the difficulty of job hopping success is much higher than in previous years. An obvious feeling: this year's interview, both on one side and on the other, is a test Java Programmer's technical skills.** Recently, I sorted out a review interview questions and high-frequency interview questions and technical points into one“**Java Classic interview questions (including answer analysis).pdf**And an online collection“**Java Programmer interview written test real question bank.pdf**"(Actually, it took a lot more energy than expected),**Including distributed architecture, high scalability, high performance, high concurrency Jvm Performance tuning Spring,MyBatis,Nginx Source code analysis, Redis,ActiveMQ,Mycat,Netty,Kafka,Mysql,Zookeeper,Tomcat,Docker,Dubbo,Nginx Wait for multiple knowledge points, advanced dry goods!** **Due to the limited space, in order to facilitate you to watch, here we show you some directories and answer screenshots in the form of pictures** 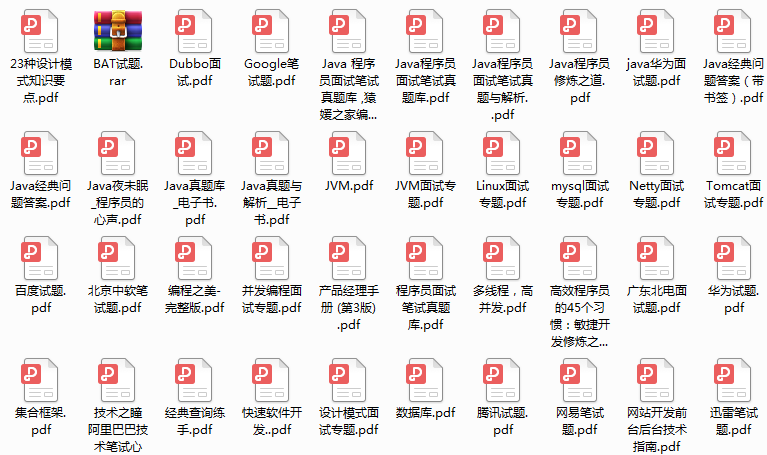 ### Java classic interview questions (including answer analysis) 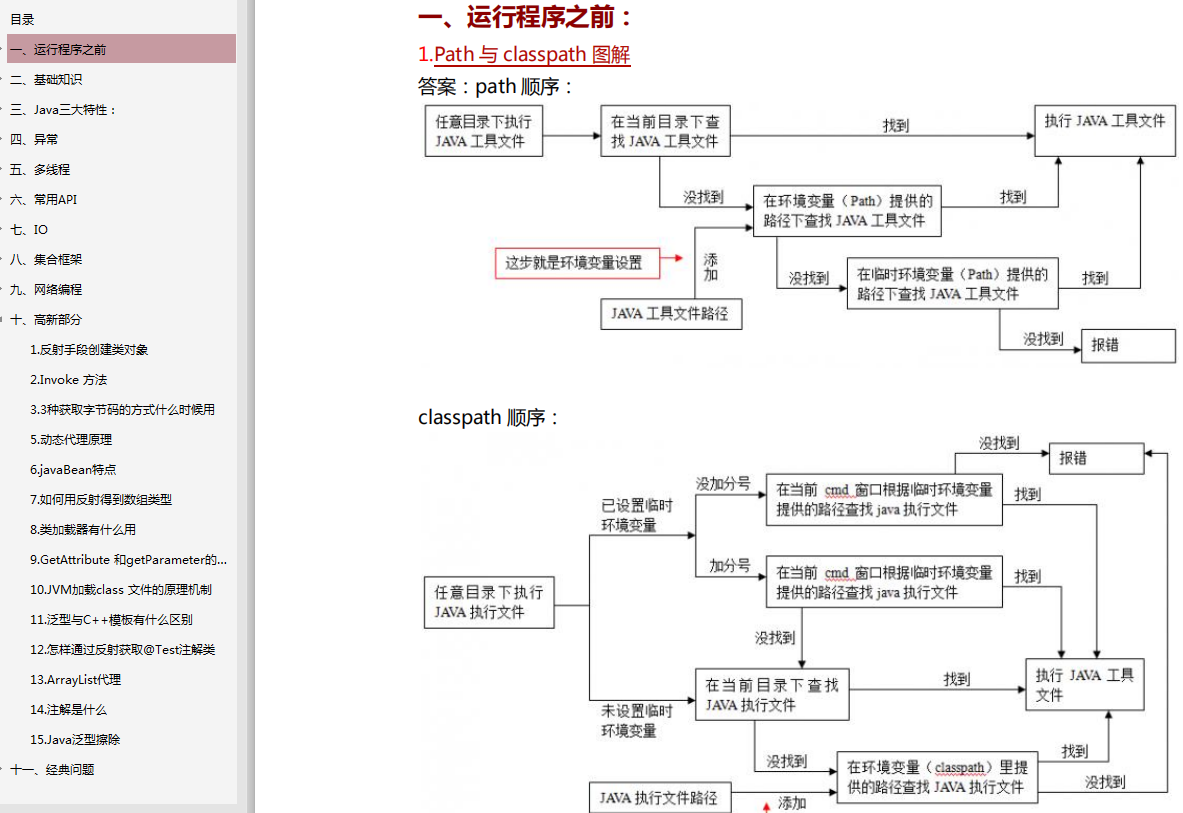 ### Alibaba technical written test experience 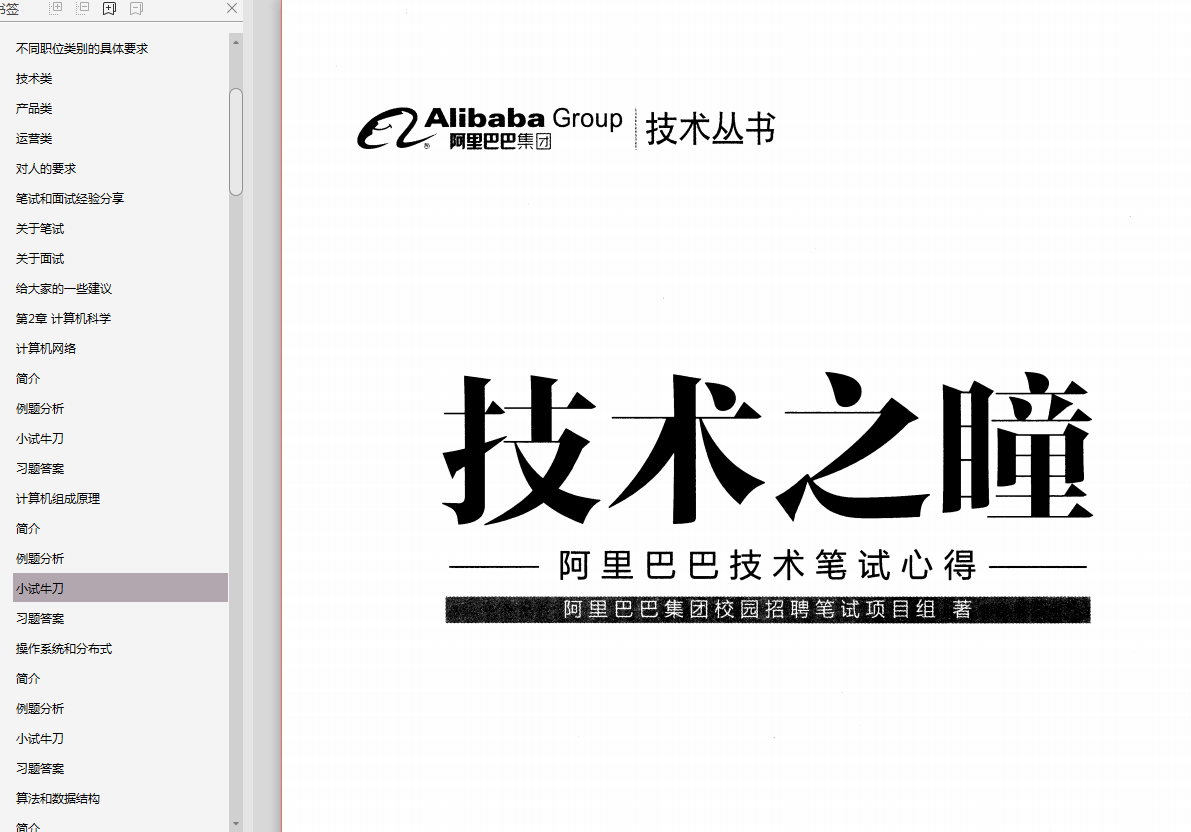 Analysis, Redis,ActiveMQ,Mycat,Netty,Kafka,Mysql,Zookeeper,Tomcat,Docker,Dubbo,Nginx Wait for multiple knowledge points, advanced dry goods!** **Due to the limited space, in order to facilitate you to watch, here we show you some directories and answer screenshots in the form of pictures** [External chain picture transfer...(img-uLUWYoo1-1628406912951)] ### Java classic interview questions (including answer analysis) [External chain picture transfer...(img-Ml5d2GB8-1628406912953)] ### Alibaba technical written test experience [External chain picture transfer...(img-SHQqKzLt-1628406912954)] #####