Character array
- definition
- An array used to store character data. An element in a character array holds a character.
- General form
- char c [10];
- Character arrays can also be two-dimensional or multi-dimensional arrays
- For example: char c[5][10]; It is a two-dimensional character array.
Character array initialization
- Assign each element in the array character by character.
- For example: char c [10] = {a ',' B ',' 2 ',' H ',' a ',' B ',' C ','d'};
- If the number of initial values provided in curly braces is greater than the length of the array, there is a syntax error;
- If the number of initial values is less than the length of the array, this character is only assigned to the first elements in the array, and the rest are '\ 0';
- If the number of initial values is the same as the predetermined array length, the length of each array can be omitted during definition, and the system will automatically determine the array length according to the number of initial values.
- For example: char c [] = {'a', 'B', '2', 'H', 'a', 'B', 'C','d '};
example
Output a string.
#include <stdio.h> void main() { char c[15] = { 'M','y',' ','n','a','m','e',' ','i','s',' ','X','G','G','M'}; for (int i = 0; i < 15; i++) printf("%c", c[i]); printf(" \n"); }
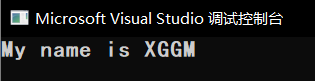
String and string end flag
- There is usually no special character string in the C language array.
- Sometimes people are concerned about the length of a valid string rather than the length of a character array.
- For example, define a character array with a length of 100, while the actual valid characters are only 40. In order to determine the actual length of a string, C language specifies a "string bundle flag", represented by the character '\ 0'.
- If there is a string in which the 10th character is' \ 0 ', the valid characters of this string are 9. That is, when the character '\ 0' is encountered, it indicates the end of the string and consists of the characters in front of it.
- You can directly assign a string to the variable represented by a character array to initialize the character array.
- For example: char c[]={"l am happy"};
- You can also omit the flower bracket and write it directly as char c [] = "l am happy";
- Assignment in string mode takes up one byte more than assignment in character by character, which is used to store the end of string flag '\ 0'.
Note: no single character is used as the initial value here. Instead, use a string as the initial value. The length of array c is not 10, but 11, because the system adds a '\ 0' at the end of the string constant.
Therefore, initialize char c[]="I am happy"; And char c [] = {'I', 'a','m ',' H ',' a ',' p ',' p ',' y ',' 0 '}; Equivalent.
char c[10]={"China"};

Input and output of character array
- Input and output character by character. Enter or output a character with format character '% c'.
- Input or output the whole string at once. Enter or output a string with the character "% s".
- be careful:
- When outputting a string with the format character "% s", the output item in the printf function is the character array name, not the array element name, or &c.
- If the array length is greater than the actual length of the string, it will only be output to the end of '\ 0'.
- The output character does not include the terminator '\ 0'.
- If a character array contains more than one '\ 0', the output ends when the first '\ 0' is encountered.
- You can use the scanf function to enter a string. For example: char c[20]; scanf("%s",c);
- If multiple strings are entered using a scanf function, they are separated by spaces.
- For example:
#include <stdio.h> #pragma warning(disable:4996) void main() { char str1[5], str2[5], str3[5]; scanf("%s%s%s", str1, str2, str3); printf("%s %s %s\n", str1, str2, str3); }
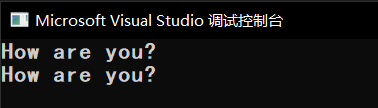
Array header address
- As mentioned earlier, the input items of scanf must appear in the form of address, such as & A, & B, etc. But in the previous example, it appears as an array name. Why?
- This is because it is stipulated in C language that the array name represents the first address of the array. The entire array is a contiguous memory unit that begins with the first address.
String handler
- C language provides rich string processing functions, which can be roughly divided into string input, output, merge, modify, compare, convert, copy and search.
- Using these functions can greatly reduce the burden of programming
- The string function used for input and output should include the header file "stdio.h" before use.
- If other string functions are used, the header file "string. h" should be included.
puts(str)
- Function: output a string (character sequence ending with '0') to the terminal and wrap it automatically.
- For example:
#include <stdio.h> #include <string.h> void main() { char str[] = { "China\nBeijin" }; puts(str); }
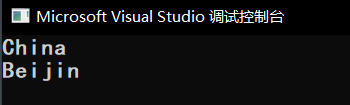
gets(str)
- Function: input a string from the terminal to the character array, and get a function value. The function value is the starting address of the character array.
- For example:
#include <stdio.h> #include <string.h> void main() { char str[10]; gets(str); puts(str); }
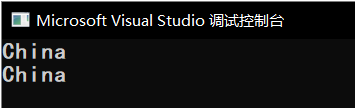
- Note: only one string can be input or output with the get and put functions Cannot be written as: puts (str1, str2); Or gets(str1, str2);
- When the input string contains spaces, the output is still all strings. Note that the function does not end with a space as the sign of string input, but only with carriage return as the input. This is different from the scanf function.
strcat()
- Function: connect the string in character array 2 to the back of the string in character array 1, delete the string flag "0" after string 1, and put the result in character array 1.
- The return value of this function is the first address of character array 1.
- General form: strcat (character array name 1, character array name 2);
- It should be noted that the character array 1 should be defined with sufficient length, otherwise it cannot load all the connected strings.
- For example:
#include <stdio.h> #include <string.h> #pragma warning(disable:4996) void main() { char str1[10],str2[5]; printf("Enter the value of array 1:\n"); gets(str1); printf("Enter the value of array 2:\n"); gets(str2); strcat(str1, str2); puts(str1); }
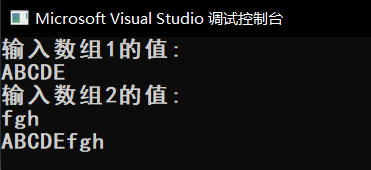
strcpy()
- Function: copy the string in character array 2 to character array 1. The string end flag "\ 0" is also copied. Character array 2 can also be a string constant.
- This is equivalent to assigning a string to a character array.
- General form: strcpy (character array name 1, character array name 2);
- You can use the strcpy function to copy the first few characters in string 2 into character array 1.
- For example: strncpy (STR1, str2, 2); Copy the first two characters of str2 and '\ O'.
- It should be noted that the value of character array 1 will be overwritten. Character array 1 should be defined with sufficient length, otherwise it cannot load all connected strings.
- str1="China" ; str1=str2; It is wrong to assign a string to a character array. You can only use the strcpy function.
- For example:
#include <stdio.h> #include <string.h> #pragma warning(disable:4996) void main() { char str1[10], str2[]="China"; strcpy(str1, str2); puts(str1); }
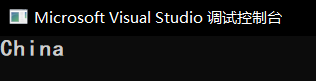
strcmp()
- Function: compare the characters in two arrays one by one from left to right according to ASCII code until different characters or '\ 0' appear, and return the comparison result by the return value of the function.
- String 1 = string 2, return value = 0;
- String 1 > string 2, return value > 0;
- String 1 < word string 2, return value < 0;
- General form: StrCmp (character array name 1, character array name 2);
- Note: for the comparison of two strings, the following form cannot be used:
- if(str1>str2) printf("yes");
- You can only use if(strcmp(str1, STR2) > o) printf ("STR1 > STR2");
- For example:
#include <stdio.h> #include <string.h> void main() { int a; char str1[10], str2[]="China"; gets(str1); a = strcmp(str1, str2); if (a == 0) printf("str1 = str2\n"); if (a > 0) printf("str1 > str2\n"); if (a < 0) printf("str1 < str2\n"); }
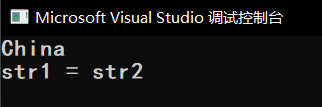
strlen()
- Function: measure the actual length of the string (excluding the string end flag "10") and return the value as a function.
- General form: strlen (character array name);
- For example:
#include <stdio.h> #include <string.h> void main() { int a; char str[] = "China"; a = strlen(str); printf("The length of the string is %d\n", a); }
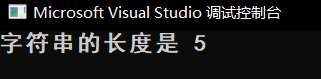
strlwr()
- Format: strlwr (string);
- Function: change uppercase letters into lowercase letters in string.
strupr()
- Format: strupr (Ning et al.);
- Function: change lowercase letters into uppercase letters in string.
example
Enter a string of characters to count the number of numbers.
#include <stdio.h> #include <string.h> void main() { char str[10],c; int i, num=0; gets(str); for (i = 0; (c = str[i]) != '\0'; i++) if (c >= '0'&&c <= '9') num++; printf("%d\n", num); }
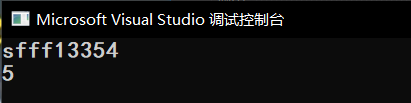
Enter a line of characters, count how many words there are, and separate the words with spaces.
#include <stdio.h> #include <string.h> void main() { int i,word=0,num=0; char c,str[80]; gets(str); printf("i\t,str[i]\t,word\t,num\n"); for (i = 0; (c = str[i]) != '\0'; i++) { if (c == ' ') word=0; else if (word == 0) { word = 1; num++; } printf("%d\t,%c\t,%d\t,%d\n", i, str[i], word, num); } printf("The number of words is %d\n", num); }
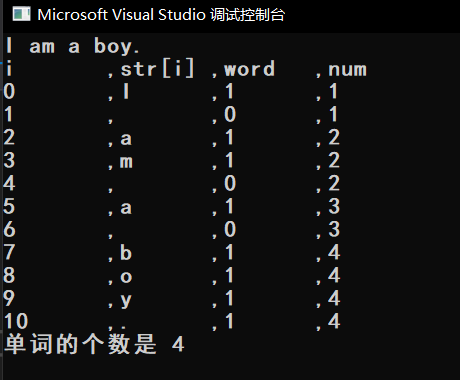