What is a function?
Library function
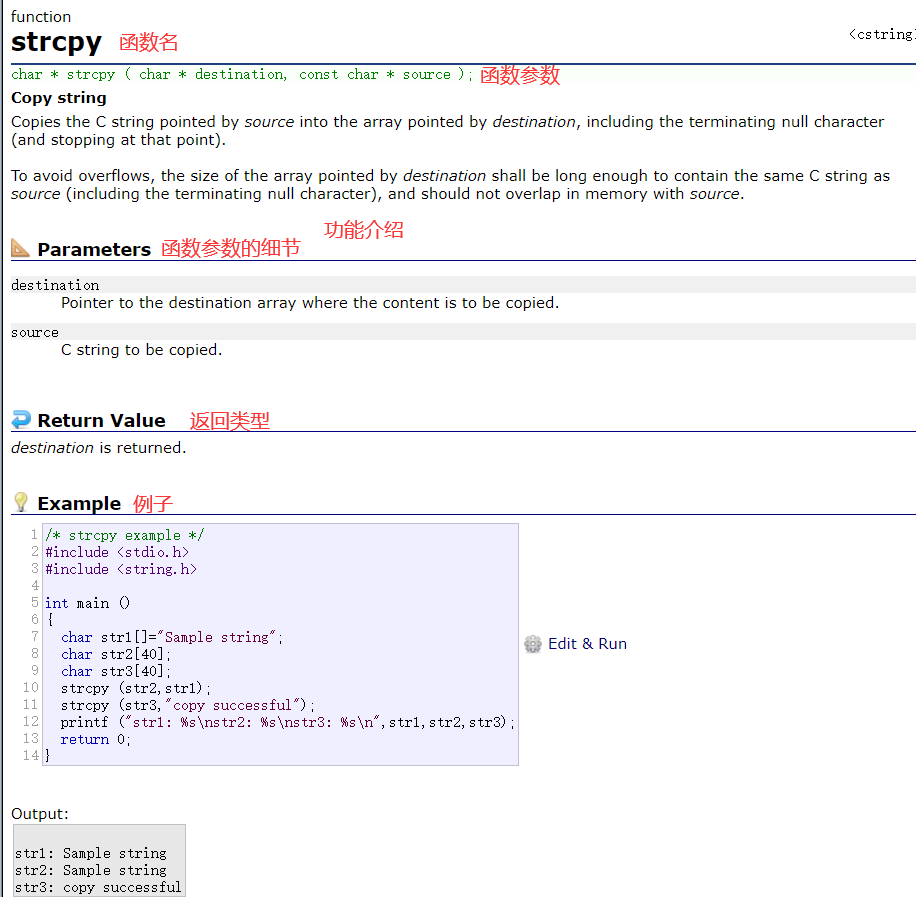
strcpy
char * strcpy ( char * destination, const char * source );
#include <stdio.h> #include <string. h> / / when calling strcpy function, you need to refer to the corresponding header file int main() { char arr1[20] = "xxxxxxxxx";//Target space char arr2[] = "hello";//Copy the contents of arr2 into arr1 char* ret = strcpy(arr1, arr2); printf("%s\n", arr1);//The result is hello printf("%s\n", ret);//The result is hello return 0; }
Why is the print result of arr1 not hello ####? Because strcpy will copy the original \ 0 when copying, there is no printing after # 0.
memset
void * memset ( void * ptr, int value, size_t num );
#include <stdio.h> #include <string.h> int main() { char arr[] = "hello bit"; char *ret = (char*)memset(arr, 'x', 5); printf("%s\n", ret);//The output result is xxxxx bit return 0; }
Here, the side 5 refers to the space size of 5 bytes, not the first five letters.
Custom function
ret_type fun_name(para1,para2,,) { statement;//Statement item } ret_type Return type fun_name Function name para Function parameters
for instance
#include <stdio.h> int get_max(int x, int y) //x. y is a formal parameter { if(x > y) return x; else return y; } int main() { int a = 0; int b = 0; scanf("%d%d", &a, &b); //Find the larger value of 2 numbers int max = get_max(a,b);//a. b is an argument printf("max = %d\n", max); return 0; }
Another example
#include<stdio.h> void Swap(int x, int y) { int z = 0; z = x; x = y; y = z; } int main() { int a = 10; int b = 20; printf("Before exchange: a=%d b=%d\n", a, b); //function Swap(a, b); printf("After exchange: a=%d b=%d\n", a, b); return 0; }
Output results
Why didn't the exchange succeed??? In fact, when the arguments a and b are passed to the formal parameters X and y, the formal parameter is a temporary copy of the argument. Changing the formal parameter variables X and y will not affect the arguments a and b.
How can we change it to realize exchange? The above program cannot be implemented because there is no connection between the internal and external functions. To establish a connection, we can pass the addresses of a and b.
#include<stdio.h> void Swap(int* px, int* py) { int z = 0; z = *px; *px = *py; *py = z; } int main() { int a = 10; int b = 20; printf("Before exchange: a=%d b=%d\n", a, b); Swap(&a, &b);//Address to receive a and b printf("After exchange: a=%d b=%d\n", a, b); return 0; }
Output results
Actual parameters
The parameters really passed to the function are called arguments.
Arguments can be constants, variables, expressions, functions, etc.
Formal parameters
function call
In fact, the first code of the exchange value function just written is a value transfer call, while the second code passes the address to the function by address transfer call.
Value passing call
Address call
My level is limited, please criticize and correct! If you like me, just pay attention!