preface:
This article explains the use of branch statements and loop statements to clarify the branches and loops.
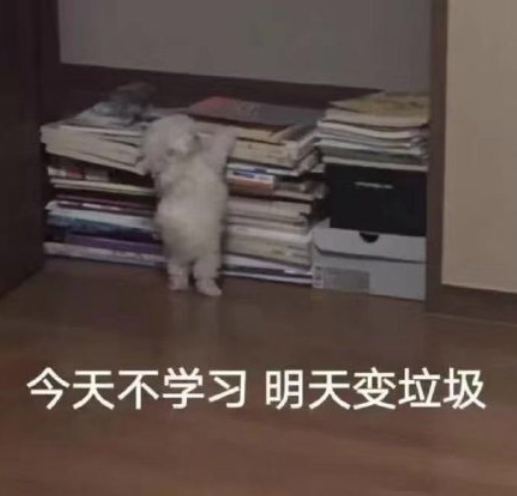
1, Statement
c language is a structured programming language. There are three structures in c language:
- Sequential structure (from top to bottom)
- Select structure (if, switch)
- Loop structure (while, for, do... While)
What is a sentence?
It is stipulated in C language that a semicolon is added after a sentence is written; Is a statement, that is to say, in C language, there is a semicolon; Separated is a statement.
For example:
int main() { int a = 5;//This is a statement printf("%d", a);//This is also a statement ;//This is still a statement. This is an empty statement return 0; }
2, Branch statement
1. Branch statement (select structure)
What is a branch? It is to separate parts from the whole or a system, and selecting a structure is to get different results after you perform some operation.
For example, if you go to college and study hard, you can get a good offer after graduation; If you don't study hard, you will honestly go home to raise pigs after graduation.
This way:
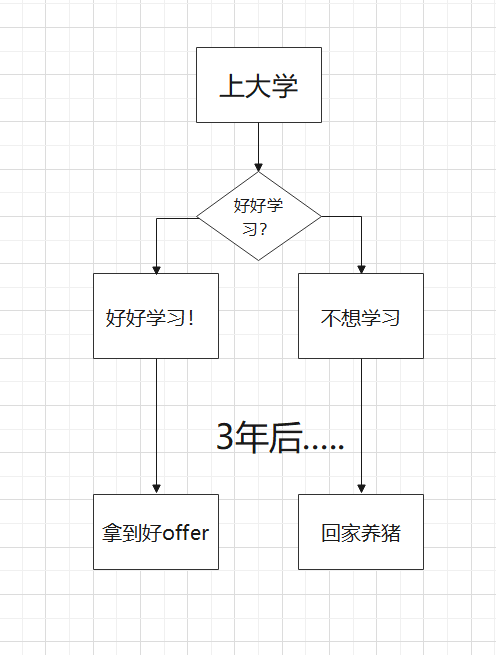
Or:
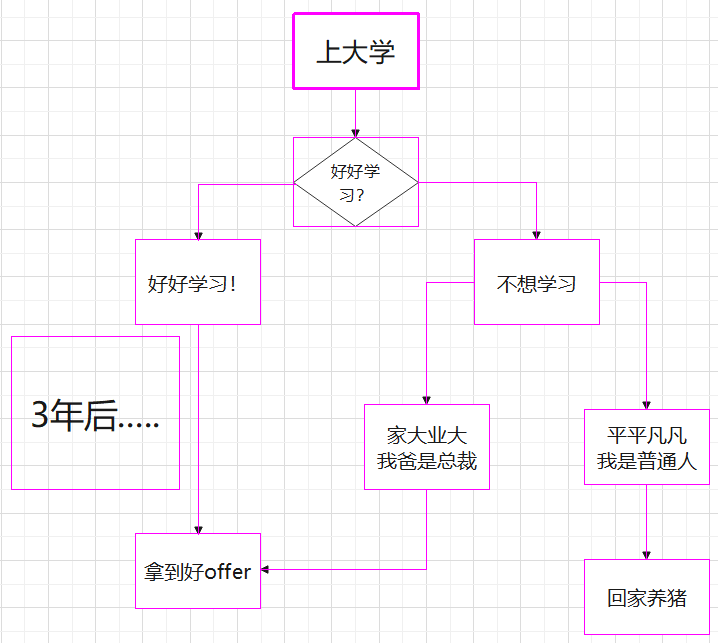
2.if statement
If statement is used to judge whether something is true. If it is true, perform the following operations. It can also be combined with else, else and if, so that several judgments can be expressed.
Syntax structure of if statement:
1. if(expression) sentence; 2. if(expression) Statement 1; else Statement 2; 3. if(Expression 1)//Multi branch Statement 1; else if(Expression 2) Statement 2; else Statement 3;
The first is the most basic if statement:
Basically judge whether a thing is true or false. In fact, the expression in the if statement really determines the value of the expression, such as money > = price. If it is true, the expression value is 1. If it is not true, the expression value is 0. In c language, 0 means false and non-0 means true, but when a true event is converted to a number, it is converted to 1 by default.
int main() { int price = 39;//Price int money = 50;//balance if (money >= price)//Judge whether the balance is greater than the price { printf("Sufficient balance, you can buy!");//If true, output this statement } return 0; }
The second is an if else statement that can branch:
The if else statement is just like its English interpretation. If... Other is a result of the if statement judging something, and then the else statement is executed for other results.
int main() { int age = 18; if (age >= 18)//Greater than or equal to 18, the expression is 1 { printf("You are an adult"); } else//In addition to the above possibilities, the other results are minors { printf("You are a minor"); } return 0; }
The third if else statement:
if else if else is the combination of the first two. It can judge a variety of possibilities and other possibilities and realize multiple branch selection.
int main() { float rec = 12.91;//Olympic records of 110 meter hurdles float your_ach = 13.22;//Olympic achievements if(your_ach<rec)//Judge whether the score is lower than the Olympic record { printf("Your result broke the Olympic record!\n"); } if (your_ach <= 13.02)//Gold medal achievement { printf("Congratulations on winning the gold medal!"); } else if (your_ach <= 13.20 && your_ach > rec)//Top three achievement range { printf("Congratulations on winning the medal!"); } else//Other achievements { printf("Unfortunately, I didn't get a medal, but it's great!"); } return 0; }
Note here:
In the if... else if statement, if the if is judged to be true, the else statement will not be executed. The relationship between the two is one of two, and if is false, the if statement in else will be judged again.
<>
If... If... Statements are executed sequentially. After executing the above if statements, whether true or false, continue to execute the following if statements.
Other codes may look like this:
int main() { int a = 5; if (a > 10) { printf("yes"); } else printf("no");//It's better to add a {} }
int main() { int a = 15; if (a > 10) printf("yes"); return 0;//Plus else {} is better }
These codes can also be run, but the whole is not beautiful. On the basis of these codes, curly brackets are added to display symmetrically, so that the logic is clearer and not easy to make mistakes.
For example, the following code makes it easy to think that 1 is printed:
int main() { int a = 1; int b = 2; if (a == 0) if (b == 2) printf("1"); else printf("0"); return 0; }
Finally, after understanding the if statement, can you write the flow chart of the first branch?
int main() { char ch = 0; printf("You went to college. Do you want to study hard?"); printf("Please enter Y/N:>\n"); scanf("%c", &ch); if (ch == 'Y') { printf("I want to study hard!\n"); printf("Three years later...\n"); printf("You got it okay offer!\n"); } else if (ch == 'N') { printf("I don't want to study hard\n"); printf("Three years later...\n"); int a = 0; printf("Big family and big business/Ordinary people: 1/2:>\n"); scanf("%d", &a); if (a == 1) { printf("You still got it offer\n"); } else if (a == 2) { printf("Go home and raise pigs\n"); } } return 0; }
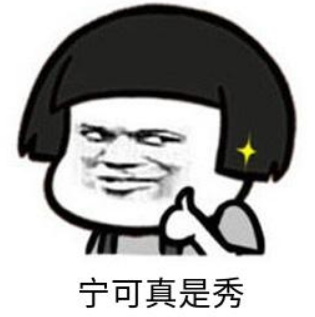
3.switch statement
Next, let's turn to our switch statement, which is also a branch statement. It is often used in the case of multiple branches.
Why is there another switch with the if branch? Let's use a code to explain:
//Suppose I want to print the day of the week today int main() { int day = 0; scanf("%d", &day); if (day == 1) { printf("Today is Monday"); } else if (day == 2) { printf("Today is Tuesday"); } else if (day == 3) { printf("Today is Wednesday"); } //.......... How many more return 0; }
Isn't the code a little sloppy? switch should be used at this time:
Syntax structure of switch statement:
switch(Shaping expression) { case Integer constant expression: sentence; } //Attached products: break; //Jump out: when a statement is executed, jump out of the whole loop default //If there is no switch shaping expression in the branch, execute the default clause
You can more clearly and simply multi branch with switch statement:
int main() { int day = 0;//plastic scanf("%d", &day); switch(day)//day must be an integer expression { case 1: //The case entry also needs to be an integer constant expression printf("Monday\n"); break;//Jump out //case is like a branch entrance, and break is a branch exit case 2: printf("Tuesday\n"); break; case 3: printf("Wednesday\n"); break; case 4: printf("Thursday\n"); break; case 5: printf("Friday\n"); break; case 6: printf("Saturday\n"); break; case 7: printf("Sunday\n"); break; default: printf("Input error, unrecognized"); //Execute this statement when the number entered is not 1-7 } return 0; }
In the above code, swith (day) is equivalent to the mate selection requirements of if you are the one. If day is 1, I will go to case 1 and execute the contents of case 1. If it is 2, you choose to go to case 2. The break is equivalent to the exit, that is, after executing the content of case 1, it jumps out. If case 1 does not break at last, it will automatically execute case 2. But not all switch statements use break, which depends on the logic of the code.
For example, I want to know if I need class today:
int main() { int day = 0; scanf("%d", &day); switch (day) { case 1: case 2: case 3: case 4: case 5: printf("Today is school day, come on!\n"); break; case 6: case 7: printf("Today is a rest day. Have a good rest!\n"); break; default: printf("Input error, unrecognized\n"); break; }
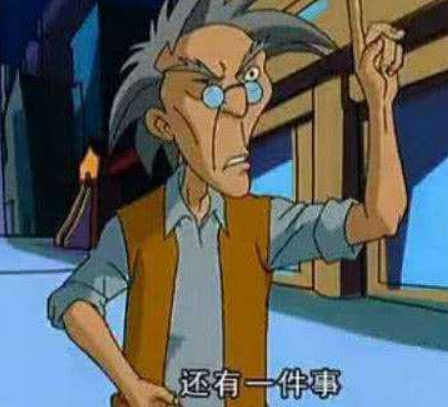
be careful:
if and switch statements can be nested. You can branch after the branch enters to complete more complex judgment. In the switch statement, add a break statement after the last case statement. The reason for this is to avoid forgetting to add a break statement after the last previous case statement.
3, Circular statement
1. Circulation
But in the above selection statements, let's understand the circular statements. In an IF statement, when the conditions are met, the statement after the if statement is executed; otherwise, it is not executed. But this statement will be executed only once. So we need to judge many times? We need our loop statement.
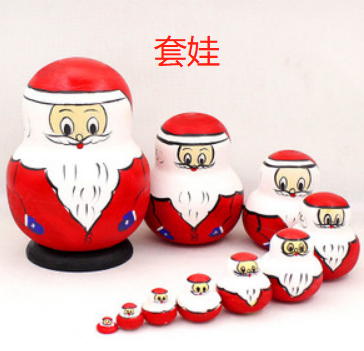
2.while statement
Syntax structure of while statement:
while(expression) Loop statement; //Bonus continue; //The code used to terminate this cycle, that is, the code after continue, will not be executed this time
In the while statement, if the expression result is true, it will enter the loop, execute it once, and then come back to judge the expression result. If the expression is 1, the code will enter the infinite loop.
For example:
Then he will enter the behavior of crazy praise me and can't extricate himself.
To get back to business, when is the while statement used? For example, when you want to print numbers from 1 to 10, you can't say 10 in printf(). What about 10000? So if we continue to execute something before it is completed, we can use the while statement:
int main() { int count = 1; while (count<10) { printf("I'm so handsome! The first%d Second shout\n",count); count++; } return 0; }
Why do we shout here 9 times? We need to see that our conditional expression says count < 10, so when count is added to 10, I won't go in again. Because the while statement is executed after judgment.
1. Difference between break and continue
There is a break out in front and a continue out here. What's the difference between the two?
We can compare the following code:
//Version 1.break int main() { int count = 1; while (count<10) { if (count == 5) { break; } printf("I'm so handsome! The first%d Second shout\n",count); count++; } printf("Shout over\n"); return 0; } //2.continue version int main() { int count = 1; while (count<10) { if (count == 5) { continue; } printf("I'm so handsome! The first%d Second shout\n",count); count++; } printf("Shout over\n"); return 0; }
The code is only different in if statements. What is the result of running?
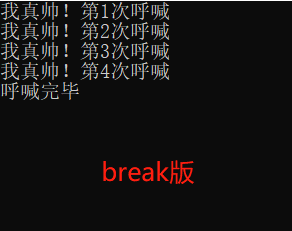
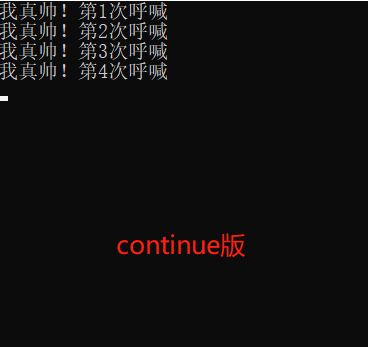
We can see that when the break version should have cycled 9 times in the while cycle, it only shouted 4 times, and then jumped out to shout the end, while the continue version did not shout and say the end after shouting 4 times.
Let's first understand the definitions of break and continue:
The role of break in the while loop:
In fact, as long as a break is encountered in the cycle, all the later cycles will be stopped and the cycle will be terminated directly. So: break in while is used to permanently terminate the loop.
The function of continue in the while loop is:
Continue is used to terminate this cycle, that is, the code behind continue in this cycle will not be executed, but directly jump to the judgment part of the while statement. Determine the inlet of the next cycle.
Therefore, when the above code count==5 meets a break in the while statement, it directly jumps out of the while loop and doesn't come in. When you encounter continue, you just don't execute the following code this time, and then go back to judge the expression of while. When count==5, you encounter continue, go back to judge, count==5, go back to judge again... Which leads to an endless loop.
So when we need to jump out of the cycle, we should pay attention to distinguish the effects of the two. Don't use it wrong. We want to stop talking when calling ourselves handsome.
3.for statement
Syntax structure of for statement:
for(Expression 1; Expression 2; Expression 3) Loop statement;
Among them, expression 1 is the initialization part, which is used to initialize the loop variable. Expression 2 expression 2 is a condition judgment part, which is used to judge the termination of the cycle. Expression 3 is the adjustment part, which is used to adjust the loop conditions.
for loop statement executes expression 1 first, and then judges expression 2. If it is true, enter the loop statement, and if it is false, exit the loop. If it enters the loop statement, execute expression 3 to adjust the variable. Then enter expression 2 again to judge and cycle.
Let's first compare the differences and details between the while loop and the for loop. Next, I want to print 1-10 numbers with for:
//1.while loop int main() { int i = 1;//Initialization part while (i <= 10)//Judgment part { printf("%d\n", i); i++;//Adjustment part } return 0; } //2.for loop int main() { for(i=1; i<=10; i++) //Three in one { printf("%d\n",i); } }
It can be found that there are still three necessary conditions for the loop in the while loop, but due to the style problem, the three parts are likely to deviate far, so the search and modification is not centralized and convenient. Therefore, the style of for loop is better, and for is used the most frequently.
The for loop statement is also easy to understand:
int main() { int i = 0; for (i = 0; i <= 10; i++) //Single expression; Conditional expression; End loop //Initialize assignment; Judgment conditions; Circular statement { printf("%d", i);//Circulatory body } return 0; }
For statement, that is, in the parentheses of for, the first; The former is the initial assignment statement as a variable, which is used to give initial values to the loop control variables. The second is to determine the sentence to determine whether to satisfy the conditions to enter the loop. The third is to adjust the loop variables after the cycle and avoid the infinite loop.
The break in the for statement is the same as that in the while statement, but the continue is different:
Continue in the for loop skips the code behind continue, goes to the adjustment part, and adjusts the loop variables, which is not easy to cause an endless loop.
In the while loop, continue skips the code behind continue and goes directly to the judgment part.
4.do while statement
Syntax structure of do statement:
do { Circular statement }while(expression);
The do... While loop is similar to the while loop, but the difference is that the do... While loop will be executed directly once. No matter whether the expression is true or false, it will be executed first and then judged at the while, that is, it will be executed first and then judged. Judging whether it is true or false will be used as the basis for entering the loop again.
For example:
int main() { int i = 0; do { i++; } while (i <= 10); // The same judgment conditions as the while loop, but the result is more than 1, which proves that under the same conditions //The do while statement executes the loop body one more time than the while statement printf("%d", i);//i=11 return 0; }
The do statement is executed at least once in the loop. The use scenario is limited and is not often used.
5. Small exercises
Knowing the above, let's write an exercise:
1. Write code to demonstrate that multiple characters move from both ends and converge to the middle. For example:
//I want to print hello, so print it like this: h o he lo hello //From both sides to the middle
code:
#include <windows.h> #include <string.h> #include <stdlib.h> int main() { char arr1[] = "hello! today is a good day!"; //What you want to print char arr2[] = " "; //Empty screen int right = strlen(arr1)-1; //strlen calculates the length of the string, minus 1 to indicate the subscript of the last character of the string int left = 0; //The first character is marked in the table below while (left <= right)//Cycle conditions: the leftmost lower table and the rightmost lower table are different { arr2[left] = arr1[left];//Exchange characters with an empty screen and print arr2[right] = arr1[right]; left++;//The leftmost switch shifts one bit to the right after printing right--;//Shift the rightmost switch one bit to the left after printing printf("%s\n", arr2);//Print Sleep(1000); //The execution is suspended for a period of time, which is the pause time. The unit is milliseconds, so here is 1s system("cls"); //System is a library function used to execute system commands. cls means clear screen //So the above print will be cleared before printing the next content } printf("%s\n", arr1); //The screen will be cleared for the last time, so I'll print it again after jumping out of the cycle. return 0; }
4, goto statement
In theory, goto statement is not necessary. In practice, it is easy to write code without goto statement. However, goto statements are still useful in some situations. The most common usage is to terminate the processing process of the program in some deeply nested structures, such as jumping out of two or more layers of loops at a time. In this case, using break will not achieve the goal. It can only exit from the innermost loop to the upper loop, which is equivalent to an arbitrary door.
We can write a program to shut down the computer:
#include <windows.h> #include <string.h> #include <stdlib.h> int main() { char input[10] = { 0 }; //Set a string for you to enter system("shutdown -s -t 60"); //System is a library function used to execute system commands //shutdown -s is shutdown, followed by - t seconds. What is the number of seconds before shutdown again: printf("The computer will shut down within 1 minute. If you enter: I am Han PI, you will cancel the shutdown!\n Please enter:>"); //Prompt you to enter scanf("%s", input);//input if (strcmp(input, "I'm a pig") ==0 ) //strcmp string comparison function returns 0 if it is the same { system("shutdown -a"); //shutdown -a is to cancel shutdown } else//If the input is different { goto again;//Jump back and let you enter it. It will be turned off in 1 minute } return 0; }
This is the purpose of goto, to return to a marked place. But without goto statements, we can also use while statements to achieve this function. In fact, the real application of goto statements lies in the deep loop nesting, such as
int main() { for () { for () { for () { if () { goto here; } } } } here: //See the light again return 0; }
5, Guess numbers games
According to the content of this article, you can write a number guessing game. The following is a link to the number guessing game. Interested partners can have a look:
[C language] guessing numbers games
Well, that's all for the content of this article. Xiaobai's production is not easy. Please xdm correct the mistakes, pay attention to each other and make common progress.
There is another thing: