This article was last updated on February 20, 2022, and has not been updated for more than 7 days. If the article content or picture resources fail, please leave a message and feedback. I will deal with it in time. Thank you!
Reference method of string
- Strings are stored in character arrays.
- To reference a string, you can use the following two methods:
- Use a character array to store a string. You can output the string through the array name and format declaration "% s". You can also refer to a character in the string through the array name and subscript.
- Use the character pointer variable to point to a string constant, and reference the string constant through the character pointer variable.
- example
#include <stdio.h> void main() { char string[] = "I love China!"; printf("%s\n", string); printf("%c\n", string[7]); // Output the eighth character } //Output a string through a character pointer #include <stdio.h> void main() { char *string = "I love China!"; printf("%s\n", string); printf("%c\n", string[7]); // Output the eighth character }
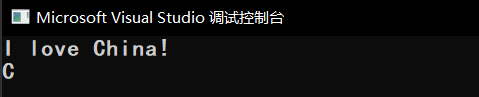
Copy a string array to b string array
#include <stdio.h> void main() { char a[] = "abcdefg"; char b[20]; int i; for (i = 0; *(a + i) != '\0'; i++) { *(b + i) = *(a + i); } *(b + i) = '\0'; printf("a = %s\n", a); // printf("b = %s\n", b); printf("b = "); for (i = 0; b[i] != '\0'; i++) { printf("%c", b[i]); } printf("\n"); }
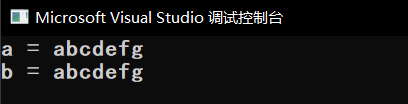
Handle with pointer variables
#include <stdio.h> void main() { char a[] = "abcdefg", b[20],*p1,*p2; p1 = a; p2 = b; for (; *p1 != '\0'; p1++) { *p2 = *p1; p2++; } *p2 = '\0'; printf("a = %s\n", a); printf("b = %s\n", b); }
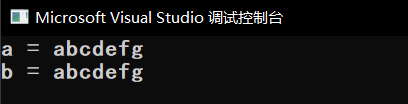
Comparison between character pointer variable and character array
- Character array and character pointer variable can be used to realize the storage and operation of string;
There are differences between them, mainly in the following points:
- 1. The character array is composed of several elements, with one character in each element; The character pointer variable stores the address (the address of the first character of the string), rather than putting the string into the character pointer variable.
- 2. The assignment methods are different. The character pointer variable can be assigned, but the array name cannot be assigned.
- char *a; a="I love Chinal"; correct
- char str[14]; str[0]='1'; correct
- char str[14]; str = "I love China!"; error
- 3. The contents of storage units are different. During compilation, several storage units are allocated to the character array to store the values of each element,
For character pointer variables, only one storage unit is allocated.
- char *a, str[10]; a=str; scanf ("%s",a); correct
- char *a; scanf("%s",a); error
- 4. The value of pointer variable can be changed, while the array name represents a fixed value (i.e. the address of the first element of the array) and cannot be changed.
- example
#include <stdio.h void main() { char *a = "I love China!"; a = a + 7; printf("%s\n", a); // Output from the eighth character }

- 5. The value of each element in the character array can be changed, but the content of the string constant pointed to by the character pointer variable cannot be replaced.
- char a[]="House", *b="House";
- a[2]='r'; correct
- b[2]='r'; error
- 6. Reference array element
- For the character array, the array elements can be referenced by subscript method and address method.
- For example: a[5], * (a+5)
- If the character pointer variable p=a, it can also be referenced in the form of pointer variable with subscript and address method.
- For example: p[5], * (p+5)
- For the character array, the array elements can be referenced by subscript method and address method.
- 7. Use pointer variable to point to a format string, which can be used to replace the format string in printf function.
- example
#include <stdio.h> void main() { char *format; int a = 10; float b = 3.6; format = "a = %d, b = %f \n"; printf(format, a, b); // amount to printf("a = %d, b = %f \n", a, b); }
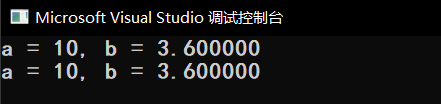
Character pointer as function parameter
- If you want to "pass" a string from one function to another, you can use address passing, That is, the character array name can be used as the parameter, and the character pointer variable can also be used as the parameter.
- The content of the string can be changed in the called function, and the changed string can be referenced in the calling function.
- example
Copy the string with function call.
#include <stdio.h> void main(){ // Character array name as parameter void string_copy(char from[], char to[]); char a[] = "abcdefg", b[20]; string_copy(a, b); printf("a = %s\n", a); printf("b = %s\n", b); } void string_copy(char from[], char to[]){ int i; for (i=0; from[i]!= '\0'; i++) { to[i] = from[i]; } to[i] = '\0'; }
#include <stdio.h> // Character pointer variables as parameters void main(){ void string_copy(char *from, char *to); char *a = "abcdefg", b[20]; char *to = b; string_copy(a, to); printf("a = %s\n", a); printf("b = %s\n", b); } void string_copy(char *from, char *to){ for (; *from != '\0'; from++,to++) { *to = *from; } *to = '\0'; }
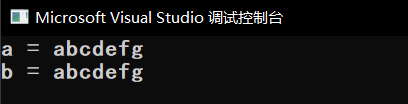
Pointer to function
- In C language, a function always occupies a continuous memory area, and the function name is the first address of the memory area occupied by the function.
- We can assign a pointer variable to the first address (or entry address) so that the pointer variable points to the function.
- Then you can find and call this function through the pointer variable. We call the pointer variable of this number "function pointer variable".
Calling a function with a function pointer variable
- Definition of function pointer variable
- The general form is:
- Type specifier (* pointer variable name) ()
- Type specifier: indicates the type of the return value of the specified function
- *Pointer variable name: the variable after "*" is the defined pointer variable
- (): pointer variable refers to a function
- The general form is:
- For example: int (*pf)(); Indicates that pf is a pointer variable pointing to the function entry, and (function value) is an integer.
- example
#include <stdio.h> // Character pointer variables as parameters void main(){ int max(int, int); int(*p)(int, int); int a, b, c; p = max; scanf_s("%d %d", &a, &b); c = (*p)(a, b); printf("max=%d\n", c); } int max(int x,int y){ int z; z = (x > y) ? x : y; return (z); }
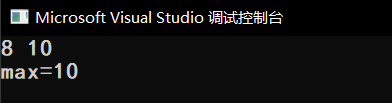