This article was last updated on February 22, 2022, and has not been updated for more than 6 days. If the article content or picture resources fail, please leave a message and feedback. I will deal with it in time. Thank you!
Structure type
- The user establishes a combined data structure composed of different types of data, which is called structure.
- The general form of declaring a structure type is:
- struct structure name {member list};
explain:
- 1. Many types of structures can be designed
- For example:
- struct Teacher
- struct Worker
- struct Date and other struct types, each containing different members
- 2. Members can belong to another structure type
- For example:
struct Date { int month; int day; int year; }; struct Student{ int num; char name[20]; char sex; int age; struct Date birthday; };
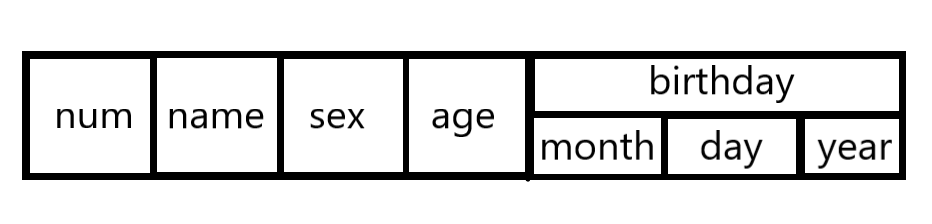
Structure type variable
- 1. Declare the structure type first, and then define the type variable.
- For example:
// Declare structure type struct Student{ int num; char name[20]; char sex; int age; }; // Define variables of this type // Structure type name structure variable name struct Student student1,student2;
- 2. Define variables while declaring types
- For example:
struct Student{ int num; char name[20]; char sex; int age; }student1,student2;
- 3. Directly define the structure type variable without specifying the type name
- The general form is:
- struct {member table column} variable name table column; An unnamed structure type was specified.
- The general form is:
- For example:
struct { int num; char name[20]; char sex; int age; }student1,student2;
explain:
- 1. Structure type and structure variable are different concepts and should not be confused.
- You can only assign, access, or operate on variables, not on a type.
- At compile time, no space is allocated to types, only space is allocated to variables. The storage space allocated by memory to structure variables is the sum of the storage space occupied by each member variable.
- 2. The member name in the structure type can be the same as the variable name in the program, but they do not represent the same object.
- 3. Structure variables can be initialized and assigned during definition. When initializing structural variables, the initial values assigned are placed in a pair of curly braces in order.
- For example:
struct Student{ int num; char name[20]; char sex; int age; }student1={2022160123,"Zhang san","male",24},student2;
A reference to a member of a structure variable
- Structure variables are construction type variables. There are two objects that can be accessed.
- 1. Members of structural variables;
- 2. The structure variable itself represents all the data members that make up the variable.
- Members that reference structural variables
- Access form of structure variable members:
- Structure variable name member name
- For example:
- student1.name
- Dot "." It is called member operator. It is a binary operator. The priority is the first level, and the associativity is from left to right.
- Access form of structure variable members:
- Members of structural variables can participate in various operations like ordinary variables
- For example:
- student2.num = student1.num++;
- For example:
explain:
- 1. If the member itself is a structure type, several member operators must be used to find the lowest level member level by level.
- For example:
- student2.birthday.day =18;
- For example:
- 2. The address of the structural variable member can be referenced, and "&" should be placed in front of the structural variable name.
- For example:
- scanf( "%d" ,&student2.num);
- For example:
- 3. "&" and "." When both occur, the operator "." High priority
- For example:
- Expression student1 Num + + is equivalent to (student1.num) + +.
- For example:
- 4. Structural variables of the same structural type can be directly assigned to each other.
- For example:
- Assign all member values of variable student1 to all member values of variable student2 one by one
- student2 = student1;
- For example:
- 5. Structural variables are different from basic type variables. They should not be directly used for arithmetic, relational and logical operations. They can only be compared one by one.
- 6. You cannot input / output structural variables as a whole. You can only input or output each member one by one.
- 7. The first address of a continuous memory cell occupied by the structure variable is called the address of the structure variable, The first address of several memory units occupied by each member is called the address of the member, and both addresses can be referenced.
example
Put a student's information (including student number, name, gender and age) into a structural variable, and then output the student's information.
#include <stdio.h> void main() { struct Student { long int num; char name[20]; char sex; int age; }std = { 10001,"Zhang san",'M',20 }; printf("Student number: NO.%ld\n full name:%s\n Gender:%c\n Age:%d\n", std.num,std.name,std.sex,std.age); }
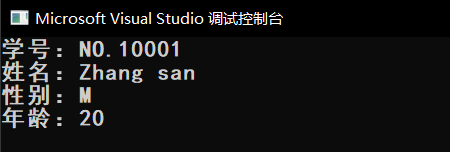
Input the student number, name and grade of two students, and output the student number, name and grade of students with higher grades
#include <stdio.h> void main() { struct Student { long int num; char name[20]; float score; }std1,std2; printf("Please enter student information:\n"); // For array input, scanf_s needs to define the buffer size, and the name is not preceded by &, and the name itself is the address scanf_s("%d%s%f", &std1.num, std1.name,21, &std1.score); scanf_s("%d%s%f", &std2.num, std2.name,21, &std2.score); if (std1.score > std2.score) { printf("Student number: NO.%ld\n full name:%s\n Achievements:%.2f\n", std1.num, std1.name, std1.score); } else if (std1.score < std2.score) { printf("Student number: NO.%ld\n full name:%s\n Achievements:%.2f\n", std2.num, std2.name, std2.score); } else { printf("Student number: NO.%ld\n full name:%s\n Achievements:%.2f\n", std1.num, std1.name, std1.score); printf("Student number: NO.%ld\n full name:%s\n Achievements:%.2f\n", std2.num, std2.name, std2.score); } }
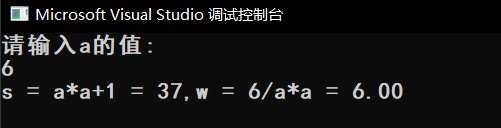