C + + Learning directory link:
C + + learning notes directory link (continuously updated)
1, while loop
The general form of the while loop statement is as follows:
While (expression) statement
An expression is generally a relational expression or a logical expression. The value of the expression should be a logical value true or false (true and false). When the value of the expression is true, the statement will be executed in a loop. When the value of the expression is false, the loop will be exited and the next item outside the loop will be executed
sentence. Each time the loop returns to the expression after executing the statement, starts judgment again, recalculates the value of the expression, exits the loop once the value of the expression is false, and continues to execute the statement when it is true. The while loop can use a process to demonstrate the execution process, as shown in the figure.
Statements can be compound statements, that is, multiple simple statements are enclosed in braces. Braces and the statements they contain are called loop body. Loop mainly refers to the content of loop execution loop body.
#include <iostream> using namespace std; void main() { int sum=0,i=1; while(i<=10) { sum=sum+i; i=i+1; } cout<<"sum:"<<sum<<endl; cout<<"i:"<<i<<endl; }
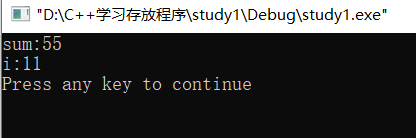
Precautions for using the while loop are as follows:
- (1) The expression cannot be empty. It is illegal for the expression to be empty.
- (2) Expressions can use non-0 to represent the logical value true (true) and 0 to represent the logical value false (false).
- (3) There must be a statement to change the value of the conditional expression in the loop body, otherwise it will become an endless loop.
2, do... while loop
The general form of do... while loop statement is as follows:
do
sentence
While (expression)
Do is the keyword and must be paired with while. The statement between do and while is called the loop body, which is also a compound statement enclosed by braces "{}". The expressions in the loop statement are the same as those in the while statement, and most of them are relational expressions or logical expressions. But it is worth noting that the do... While statement should be followed by a semicolon ";". The do... While loop can use a process to demonstrate the execution process, as shown in the figure.
The execution order of do... while loop is to execute the contents of the loop body first, and then judge the value of the expression. If the value of the expression is true, jump to the loop body and continue to execute the loop body until the value of the expression is false. When the value of the expression is false, jump out of the loop and execute the next statement.
#include <iostream> using namespace std; void main() { int sum=0,i=1; do { sum=sum+i; i=i+1; } while(i<=10);//Note the semicolon cout<<"sum:"<<sum<<endl; cout<<"i:"<<i<<endl; }
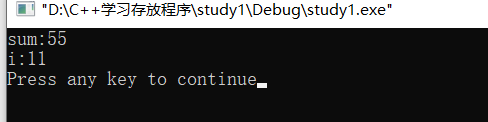
Precautions for do... while loop are as follows:
- (1) The loop executes the loop body first. If the loop condition is not tenable, the loop body has been executed - times. Pay attention to the change of variables when using.
- (2) The expression cannot be empty. It is illegal for the expression to be empty.
- (3) Expressions can use non-0 to represent the logical value true (true) and 0 to represent the logical value false (false).
- (4) There must be a statement to change the value of the conditional expression in the loop body, otherwise it will become an endless loop.
- (5) Semicolon ';' is required after loop statement.
3, do... What is the difference between a while loop and a while loop
The difference is as follows: when both the while expressions from 1 are true, the final execution results of the two are the same. When the while expression is false at first, the execution results are different.
#include <iostream> using namespace std; void main() { int i=0,j=0; cout<<"before do..,while,j="<<j<<endl; do { j++; } while(i>1);//Note the semicolon cout<<"after do..while,j="<<j<<endl; }
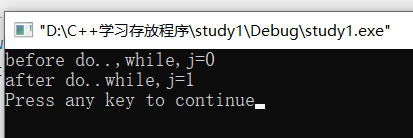
#include <iostream> using namespace std; void main() { int i=0,j=0; cout<<"before,while,j="<<j<<endl; while(i>1) { j++; } cout<<"after, while,j="<<j<<endl; }
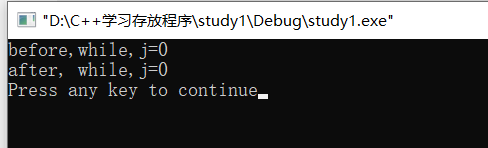
4, for loop
The general format of the for loop statement is as follows:
For (expression 1; expression 2; expression 3) statement
- Expression 1: this expression is usually an assignment expression, which is responsible for setting the starting value of the loop, that is, assigning the initial value to the variable controlling the loop.
- Expression 2: this expression is usually a relational expression, which compares the variables controlling the loop with the allowable range values of the loop variables.
- Expression 3: this expression is usually an assignment expression, which increases or decreases the variables of the control loop.
- Statement: the statement is still a compound statement.
The execution process of the for loop is shown below
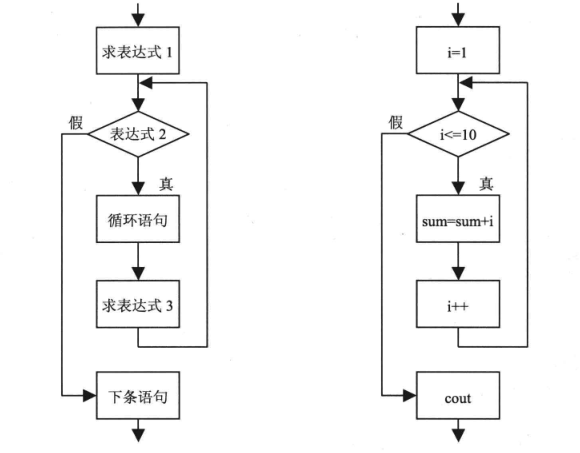
#include <iostream> using namespace std; void main() { int sum=0; int i; for(i=0;i<=10;i++) { sum=sum+i; } cout<<"sum is "<<sum<<endl; }
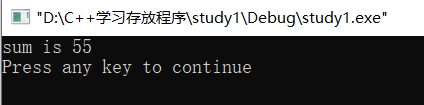
for loop considerations
- (1) The for statement can declare variables directly in expression 1.
#include <iostream> using namespace std; void main() { int sum=0; int i;//Declare variables outside expressions for(i=0;i<=10;i++) { sum=sum+i; } cout<<"sum is "<<sum<<endl; }
#include <iostream> using namespace std; void main() { int sum=0; for(int i=0;i<=10;i++)//Declare variables within expressions { sum=sum+i; } cout<<"sum is "<<sum<<endl; }
for declaring variables in a loop statement is also equivalent to declaring variables in a function. If two identical variables are declared in expression 1, the compiler will report an error. for example:
#include <iostream> using namespace std; void main() { for(int i=0,sum=0;i<=10;i++)//Declare variables within expressions { sum=sum+i; } for(int i=0,sum=0;i<=10;i++)//report errors { sum=sum+i; } cout<<"sum is "<<sum<<endl; }
- Expression 1, expression 2 and expression 3 in the for loop can be omitted.
If expression 1 is omitted and the control variable is declared and assigned an initial value outside the loop, the program can be compiled and run correctly. For example, the following code is 1
Expression 2 is omitted, that is, the loop judgment statement is omitted, that is, without the termination condition of the loop, the loop becomes an infinite loop.
After omitting expression 3, the loop is also an infinite loop, because the variables controlling the loop are always the initial values and always meet the loop conditions.
If the for loop statement omits expression 1 and expression 3, it is like a while loop -. For example, the following code 2
For loop statement if you omit three expressions, it will become an infinite loop. Infinite loop is a dead loop, which will paralyze the program. When using a loop, it is recommended to use count control, that is, when the loop is executed for a specified number of times, it will jump out of the loop. Example: Code 3
Code 1
#include <iostream> using namespace std; void main() { int i=0,sum=0; for(;i<=10;i++)//Take the cyclic variable out of the expression and give it an initial value { sum=sum+i; } cout<<"sum is "<<sum<<endl; }
Code 2
#include <iostream> using namespace std; void main() { int i=0,sum=0; for(;i<=10;) { sum=sum+i; i++; } cout<<"sum is "<<sum<<endl; }
Code 3
#include <iostream> using namespace std; void main() { int i=0,sum=0; for(;;) { sum=sum+i; i++; if (i>5)//Counting control { break;//Jump out of loop } } cout<<"sum is "<<sum<<endl; }
5, Cycle control
Loop control includes two aspects: one is to control the change mode of loop variables, and the other is to control the jump of loop. To control the jump of a loop, you need to use the keywords break and continue. The jump effects of these two jump statements are different. Break is to interrupt the loop and continue is to jump out of the execution of the loop body.
Cyclic variable control
There are two ways to change the loop control variables of the for loop, one is increasing and the other is decreasing. Whether to use increment or decrement is related to the comparison of the initial value and range value of the variable.
If the initial value is greater than the value in the limited range, expression 2 is an inequality determined by the greater than relationship (>), and the decreasing method is used.
If the initial value is less than the limited range value, expression 2 is an inequality determined by the less than relationship (<), and the incremental method is used.
Previously, the for loop is used to calculate the cumulative sum of 1 to 10. The incremental method can also be used to calculate the cumulative sum of 1 to 10. The code is as follows:
#include <iostream> using namespace std; void main() { int sum=0; for(int i=10;i>=0;i--) { sum=sum+i; } cout<<"sum is "<<sum<<endl; }
break statement
Use the break statement to jump out of the switch structure. In the loop structure, the break statement can also jump out of the current loop body to interrupt the current loop.
The form of break statement used in the three loop statements is shown in the figure.
#include <iostream> using namespace std; void main() { int i,n ,sum; sum=0; cout<<"Enter 10 integers"<<endl; for(i=1;i<=10;i++) { cin>>n; cout<<" current i yes"<<i<<"Entered n yes"<<n<<endl; if (n<0) break;//Jump out of loop sum=sum+n;//Sums the numbers that can be entered } cout<<"sum is "<<sum<<endl; }
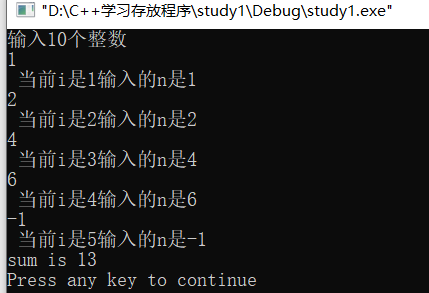
continue Statement
The continue statement is a supplement to the break statement. Continue does not immediately jump out of the loop body, but skips the statements before the end of the loop, returns to the conditional test part of the loop, and restarts the execution of the loop. When continue is encountered in the for loop statement, the incremental part of the loop is executed first, and then the condition test is performed. In the while and do... While loops, the continue statement returns control directly to the test part.
#include <iostream> using namespace std; void main() { int i,n ,sum; sum=0; cout<<"Enter 10 integers"<<endl; for(i=1;i<=10;i++) { cin>>n; cout<<" current i yes"<<i<<"Entered n yes"<<n<<endl; if (n<0)//Judge whether it is less than 0 continue;//Jump out of this cycle sum=sum+n;//Sums the numbers that can be entered } cout<<"sum is "<<sum<<endl; }
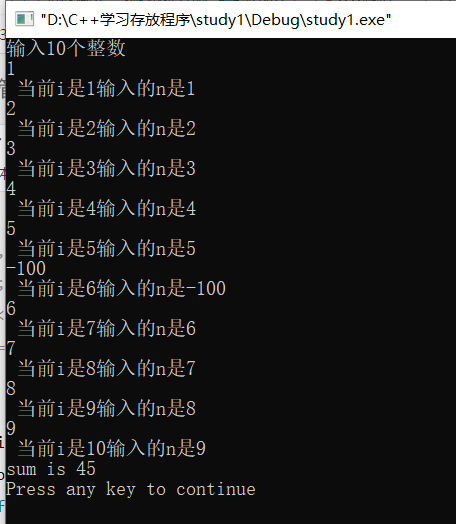
6, Loop nesting
There are three ways of loops: for, while, do... While. These three loops can be nested with each other. For example, apply a for loop to a for loop.
for(...) { for(...) {} }
Using a while loop in a while loop
while(...) { while(...) {} } }
Using a for loop in a while loop
while(...) { for(...) {} }
Print 9 * 9 multiplication table
#include <iostream> #include <iomanip> using namespace std; void main() { int i,j; i=1; j=1; for(i=1;j<10;i++) { for(j=1;j<i+1;j++) {cout<<setw(2)<<i<<"*"<<j<<"="<<setw(2)<<i*j; } cout<<endl; } }
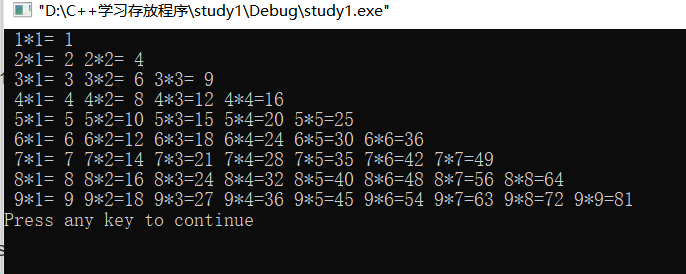
summary
This article explains the circular statements in C + +.
Author: Electric - Yu dengwu