Recently I started preparing for the Spring Entrance. I blogged to review C++ basic grammar and some interview questions.
1. Get the number of elements in the array
int arr[] = {1,2,3,4,5,6,7,8,9}; cout<<"The array has:"<<sizeof(arr)/sizeof(arr[0])<<endl; cout<<"The first address of the array:"<<arr<<endl; cout<<"The first address of the array element:"<<&arr[0]<<endl;
2. Inverse Array Order
int arr[] = {1,2,3,6,5,8}; int len = sizeof(arr)/sizeof(arr[0]),temp;//Gets the length of the array // cout<<len; int arr2[len]; for(int i = len-1;i>=0;i--) { arr2[(len-i)-1]=arr[i]; // cout<<len-i-1<<" "; } for(int i = 0;i<len;i++){ cout<<arr2[i]<<endl; }
3. Pointer and Reference
//On x86 operating systems, pointers account for 4 bytes and on 64-bit operating systems for 8 bytes int a = 10; int *p = &a; //The pointer must be initialized (pointed) or it will become a wild pointer *p = 1000; //The address where p points to a, *p stands for the content of the address, and modifying *p is equivalent to modifying a cout<<"At this time a The value was modified to:"<<a<<endl; cout<<"sizeof(int *)"<<sizeof(p)<<endl;
//Pointer Constant and Constant Pointer int a = 10; int b = 20; // constant pointer,Can only modify content, point to non-modifiable int * const p = &a; *p = 100; // Constant pointer, can only modify pointer, cannot modify content const int * p2 = &a; p2 = &b; // Mixed mode, can not be modified const int * const p3 = &a;
Memory: the pointer constant const modifies the address of p to a, while const points to unmodifiable
Constant pointer const modifies *p, *p is content, content cannot be modified
//Pointer and Array int arr[] = {1,2,3,4,5,6,7,8,9,10}; int *p = arr; cout<<"The first element of the array:"<<*p<<endl; p++; //One way to write it is P+4, because int s take up four bytes, but there is a potential danger that different processors may have different data types cout<<"The second element of the array:"<<*p<<endl;
Pointers also have a way to solve the problem of modifying parameters and a way to use references
void swap01(int *p1 , int *p2) { int temp = *p1; *p1 = *p2; *p2 = temp; }//Pointer Styleint a = 10,b=20; swap01(a,b);//Passing by reference is similar to passing by pointer cout<<a<<endl; cout<<b<<endl;
int a = 10; int b = 20; swap(&a,&b); cout<<a<<endl; cout<<b<<endl;
//By reference
But note that:
int& test01(){//Used to return static variable references static int a = 6; return a; }
int& ref = test01(); cout<<"ref:"<<ref<<endl; //Output 6 test01() = 666;//Use reference as return value, then function can be left modified cout<<"ref:"<<ref<<endl; //Output 666
4. Function overload
Three conditions need to be met:
void fun(int &a){ //Variable is called } void fun(const int &a){ //Constant is called } void func(int a){ }
5. Access rights to classes
1.public Accessible both inside and outside classes 2.protected Within a class, outside a class, children can't access the contents of the parent 3.private Within class may not, outside class may not You cannot access content in the parent struct Default is public class Default is private
The main difference between protected and private is evident in inheritance:
When using protected inheritance, derived classes have access to members within the class, while when using private inheritance, derived classes do not have access to members within the class
6. Various constructors for classes and classes
class Phone{ public: Phone(string name) :m_PName(name) { cout<<"phone"<<endl; } string m_PName; ~Phone(){ cout<<"Phone Destruction of"<<endl; } }; class Person{ ~Person(){ cout<<"Person Destruction of"<<endl; } Person(){ cout<<"person"<<endl; } string m_name; Phone m_phone; };
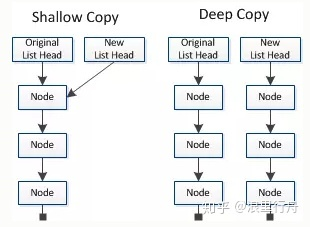
Hazard of shallow copies: When a destructor executes (the newly opened pointer points to the same space as the pointer), the heap data is released twice, causing the program to run