Docker lifecycle and Dockerfile
Docker lifecycle
During the operation of the container, no matter what operation is carried out, once the container exits or restarts, the data in it will be cleared, which is the life cycle of the container.
Dockerfile and command line
directory structure
tke-lesson3 ├── Dockerfile ├── sources.list
sources.list file content
deb http://mirrors.cloud.tencent.com/ubuntu/ focal main restricted universe multiverse deb http://mirrors.cloud.tencent.com/ubuntu/ focal-security main restricted universe multiverse deb http://mirrors.cloud.tencent.com/ubuntu/ focal-updates main restricted universe multiverse deb-src http://mirrors.cloud.tencent.com/ubuntu/ focal main restricted universe multiverse deb-src http://mirrors.cloud.tencent.com/ubuntu/ focal-security main restricted universe multiverse deb-src http://mirrors.cloud.tencent.com/ubuntu/ focal-updates main restricted universe multiversedeb http://mirrors.cloud.tencent.com/ubuntu/ focal main restricted universe multiverse deb http://mirrors.cloud.tencent.com/ubuntu/ focal-security main restricted universe multiverse deb http://mirrors.cloud.tencent.com/ubuntu/ focal-updates main restricted universe multiverse deb-src http://mirrors.cloud.tencent.com/ubuntu/ focal main restricted universe multiverse deb-src http://mirrors.cloud.tencent.com/ubuntu/ focal-security main restricted universe multiverse deb-src http://mirrors.cloud.tencent.com/ubuntu/ focal-updates main restricted universe multiverse
Dockerfile file content
#Get the ubuntu image with version number latest FROM ubuntu:latest #Modify the image source address of apt COPY ./sources.list /etc/apt/ #Update local cache package RUN apt-get update && apt-get install -y python3
Right click in VSCode and select "open in integrated terminal" and enter the following command to package it into an image
docker build -t tke-lesson3 .
Create a new command line window, start the container named tke-lesson3, and enter interactive mode.
docker run -it --rm --name tke-lesson3 tke-lesson3
Then enter python 3 to enter the interactive mode of python.
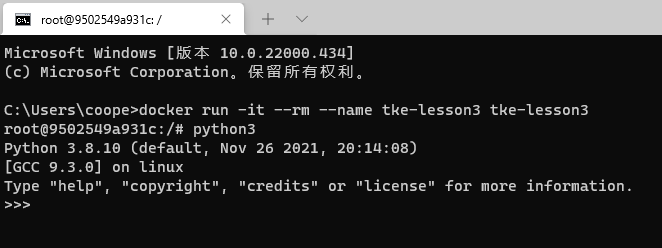
Mirror selection of programming environment
Container and programming environment
Through the docker images command, you can see that the size of the image just packaged is up to 169MB. This is because the Ubuntu system has many built-in software, resulting in a large package.
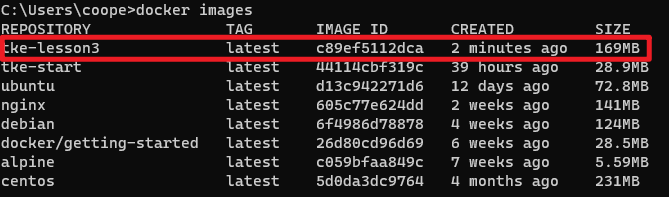
In the actual development, there may not be so many software and tools. You only need to select and install the necessary software. Therefore, it is more appropriate to choose lighter Alpine Linux for the later system image.
Official programming language image
Because alpine is too compact, many dependencies need to be installed to run the programming language project. However, various programming language versions of the system are also officially available.
- JavaScript Node: Official image of node
- Java's Open JDK: openjdk official image
- Python: Official image of python
- Go: Official image of golang
- PHP: PHP official image
- .Net : . net official image
The operating systems used by the official image may be different, such as the Linux operating system distribution alpine, bullseye, stretch, buster, and windows systems; The chip architecture is amd64, arm32v6, arm32v7, arm64v8, ppc64le, s390x, etc; Different development environment versions (e.g. Nodejs 17.1.0); And slim has multiple tag s to choose from.
Directly use the command docker pull node to pull the image under the lastest tag, and lastest is only the version number name, not necessarily the latest, so it is best to use a fixed tag.
Use of official images
docker pull python:3.11.0a4-bullseye docker run -it --rm --name tke-python python:3.11.0a4-bullseye #Write the version number later, or you will download lastest
Build Python 3.0 manually 10 mirroring
Dockerfile file:
FROM python:3.10.0-bullseye
Terminal input under current directory:
docker build -t tke-python .
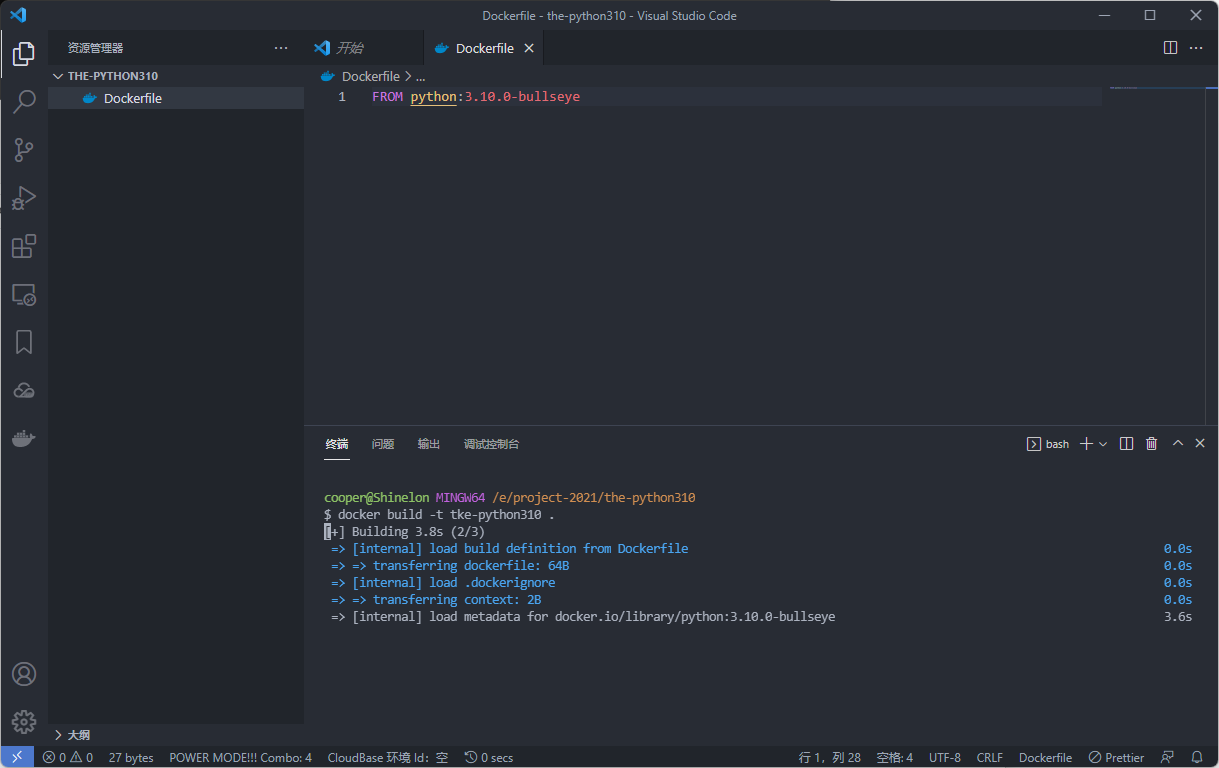
Using Docker to learn programming language
Python project
directory structure
python-start ├── Dockerfile └── index.py
index.py
name = input("Please enter your name:") company = input("Please enter your company name:") title = input("Please enter your position:") email = input("Please enter your email address:") info = f""" The results you entered are as follows: honorific{name},Hello, you work in{company}Corporate{title}, We will send you an email{email}Keep in touch with you. """ print(info)
Dockerfile
#Pull to Python 3 development environment FROM python:3.10.0-bullseye #Set working directory WORKDIR /usr/src/app #Copy local directory files to working directory COPY . . #Execute command CMD [ "python", "./index.py" ]
Package image
docker build -t python-start:1.0.1 .
Enter interactive mode
docker run -it --rm --name python-start python-start:1.0.1
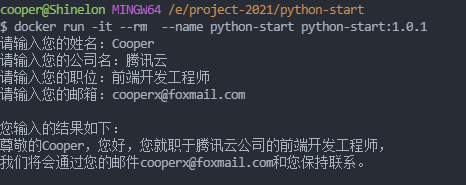
Package manager and Docker
directory structure
express-start ├── Dockerfile └── package.json └── index.js └── .dockerignore
package.json
{ "name": "TKE express Basic case", "description": "use Docker To deploy back-end applications", "version": "1.0.0", "main": "index.js", "scripts": { "start": "node index.js" }, "dependencies": { "express": "^4.17.1" } }
index.js
const express = require('express') const app = express() const port = 3000 app.get('/', (req, res) => { res.send('Hello, you opened the home page of the website') }) app.listen(port, () => { console.log(`The port you are opening is: http://localhost:${port}`) })
Dockerfile
FROM node:17-slim WORKDIR /usr/src/app COPY package.json ./ RUN npm install --only=production COPY . ./ CMD [ "npm", "start"]
Terminal under current directory
docker build -t node-start:1.0.1 . docker run -dp 3005:3000 --name node-start node-start:1.0.1
Visit localhost:3005 and you can see the web page.
summary
There are many practical projects in today's chapter. I experienced the process of deploying projects in docker using python and nodejs. Every time you create a container image, the speed will be slow, so you need to add sources List to speed up. Using docker to run programming language projects is lighter than the traditional server environment. Moreover, different docker containers are isolated from each other and do not affect each other. It is very convenient for practical development.