Introduction to basic use
- The service provider writes the implementation class based on the interface
- Keep Dubbo service interface (including registry address, protocol name and port) with Spring configuration
- The consumer cooperates with Spring and references the dubbo service interface (including: configuring the address of the registry)
- Start the spring container, get the bean and call the method
- See details Official website example
- It should be noted that the definition of and services in Dubbo:
- An interface can be called a service, and an interface implementation can also be called a service
- A service address can also be called a service address
- A complete service ID definition includes protocol, IP, port, interface name, grouping and version
- For example: dubbo://192.168.2.12:8080/user/getUserInfo:user_group:1.0.1
load balancing
- Both the consumer and the server can be configured, and the configuration is subject to the consumer
- Support algorithm
- Random (default)
- RoundRobin
- LeastActive (minimum number of active calls)
- ConsistentHash (requests with the same parameters are always sent to the unified provider)
- Custom extension
- Thinking: how is the least active count counted?
- Each consumer records the active attribute of the called server
- + 1 before the selected service call, - 1 after the result is received
- active is preferred to be the smallest, and the same is random
Service timeout
- Both server and consumer support configuration, but the effect is different
- Configured by the consumer, it indicates the timeout time of the calling server. If the timeout time is exceeded, an exception will be thrown
- If the server exceeds its configured timeout, only one police officer log will be printed
Cluster fault tolerance
- When the service provider invokes multiple instances of the service, it will handle the error message
- Failover: retry other instances. By default, it retries twice. It can be configured. By default
- Failfast: fast failure. The call will report an error immediately. It is usually used in non idempotent operation scenarios
- Failsafe: if the security fails and the exception is ignored directly, it is used for logging scenarios, for example
- It is used to record the failure in the following scenarios: scheduled, automatic, and failed
- Forking: call multiple instances in parallel. If more than one instance succeeds, it will return normally. It can also be configured. It is used for reading operations with high real-time requirements, but it wastes resources
- Broadcast: broadcast call, call one by one. If any station reports an error, it will report an error; For example, notify all instances to update local resource information such as local cache, or configure the failure ratio limit;
service degradation
- If the consumer calls the provider and reports an error, the measures taken;
- The difference between degradation and cluster fault tolerance: cluster is within the cluster, and degradation refers to within a single service provider;
- A non critical function can be masked through degradation, and the return strategy after degradation can be defined
- This mechanism is simply a mock idea, which is equivalent to a baffle
- Usage 1: mock=force:return+null. Return null value directly regardless of whether it is abnormal or not
- Usage 2: mock=fail:return+null. After the method call fails, it returns null
Local stub
- The name is very abstract, which is the Stub mechanism
- To put it bluntly, the consumer calls the provider logic by wrapping it locally
- You can do some operations before and after the call, such as parameter verification, result caching, etc
- This mechanism can also be used for fault tolerance of service invocation
package com.foo;
public class BarServiceStub implements BarService {
private final BarService barService;
// Constructor passes in the real remote proxy object
public BarServiceStub(BarService barService){
this.barService = barService;
}
public String sayHello(String name) {
// This code is executed on the client. You can do ThreadLocal local cache on the client, or pre verify whether the parameters are legal, and so on
try {
return barService.sayHello(name);
} catch (Exception e) {
// You can be fault tolerant and do anything AOP
return "Fault tolerant data";
}
}
}
Local camouflage
- The service degradation mentioned above
- See the official website for details: Local camouflage
parameter callback
- After the consumer invokes the service provider, it supports the service provider to call back a callback logic of the consumer
- Dubbo protocol is based on long connection, so if the same service provider method is called twice, it needs to be distinguished by key
- This function is equivalent to monitoring the processing of the service provider
Asynchronous call
- Directly understand it as Future
- Non blocking parallel call based on NIO
- The client can call multiple remote services in parallel without starting multithreading, which is less expensive than multithreading
Generalized call
- It is used when the client has no API interface and model class element
- Commonly used for framework integration
- For example, to implement a general service testing framework, all service implementations can be called through GenericService
import org.apache.dubbo.rpc.service.GenericService;
...
// Reference remote service
// This instance is very heavy. It encapsulates all connections with the registry and service providers. Please cache it
ReferenceConfig<GenericService> reference = new ReferenceConfig<GenericService>();
// Weakly typed interface name
reference.setInterface("com.xxx.XxxService");
reference.setVersion("1.0.0");
// Declared as a generalized interface
reference.setGeneric(true);
// Use org apache. dubbo. rpc. service. Genericservice can replace all interface references
GenericService genericService = reference.get();
// Basic types and Date,List,Map, etc. do not need to be converted and can be called directly
Object result = genericService.$invoke("sayHello", new String[] {"java.lang.String"}, new Object[] {"world"});
// The POJO parameter is represented by Map. If the return value is POJO, it will also be automatically converted to Map
Map<String, Object> person = new HashMap<String, Object>();
person.put("name", "xxx");
person.put("password", "yyy");
// If POJO is returned, it will be automatically converted to Map
Object result = genericService.$invoke("findPerson", new String[]
{"com.xxx.Person"}, new Object[]{person});
...
Generalized service
- The generic service implements the GenericService interface
- Implement the GenericService interface to handle all service requests
- It is mainly used when there is no API interface and model class element on the server
package com.foo;
public class MyGenericService implements GenericService {
public Object $invoke(String methodName, String[] parameterTypes, Object[] args) throws GenericException {
if ("sayHello".equals(methodName)) {
return "Welcome " + args[0];
}
}
}
REST
- A protocol supported by the new version of Dubbo
- For example, consumers do not use Dubbo and also want to call Dubbo services
- If a service has only REST protocol available, the service must define the access Path with @ Path annotation
- You can write services at one time and access them everywhere“
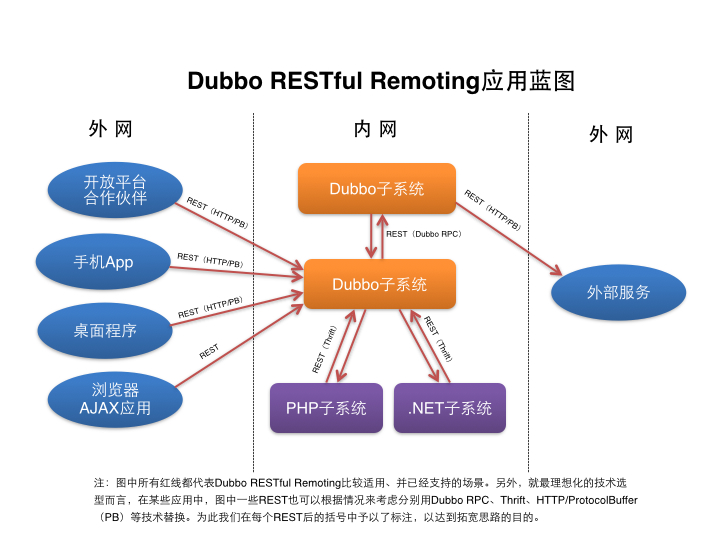
Management desk
- Dubbo admin, download the source code, package the startup interface, and use the springboot project
- dubbo supports configuring zk and redis as configuration centers
- Enter the management console to modify zk or other configuration center contents
- The overall functions provided are still improving
- Special function focus: dynamic configuration function, which can dynamically modify various service parameters
- Conditional routing configuration
- For consumers and providers
- Available means to configure routing rules
- Configurable blacklist policy
- It can be used to isolate different machine rooms
- Label routing
- It can be used for blue-green publishing or gray publishing
- Application instances can be labeled
- When consumers access with tags, they can find the corresponding providers according to the tags