winform is an old technology. I feel a little toothless (I started to contact. net from winform nearly 20 years ago); blazor, a new favorite in Microsoft's technology circle, is being taken care of. What sparks will there be after this technology collision between the old and the young?
. net v6.0.0-preview.3. It brings the premise for the combination of winform and blazor. https://github.com/axzxs2001/Asp.NetCoreExperiment/tree/master/Asp.NetCoreExperiment/Blazor/BlazorWinForm I wrote a simple demo to introduce blazor into the winform form form.
Let's see what it looks like first:
It is a simple example of querying drugs with mnemonic code. The input box query button and the style of table are bootstrap 5 0, the outer layer is a winform Form.
The specific implementation depends on the difference of the project file csproj: SDK should be replaced by Microsoft NET. Sdk. Razor, and then introduce these packages through nuget.
<Project Sdk="Microsoft.NET.Sdk.Razor">
<PropertyGroup>
<OutputType>WinExe</OutputType>
<TargetFramework>net6.0-windows</TargetFramework>
<UseWindowsForms>true</UseWindowsForms>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Dapper" Version="2.0.78" />
<PackageReference Include="Microsoft.AspNetCore.Components.WebView.WindowsForms" Version="6.0.0-preview.3.21201.13" />
<PackageReference Include="Microsoft.Extensions.DependencyInjection" Version="6.0.0-preview.3.21201.4" />
<PackageReference Include="Microsoft.NET.Sdk.Razor" Version="5.0.0-preview.8.20414.8" />
<PackageReference Include="System.Data.SqlClient" Version="4.8.2" />
</ItemGroup>
<ItemGroup>
<Content Update="wwwroot\app.css">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</Content>
<Content Update="wwwroot\Query.razor">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</Content>
<Content Update="wwwroot\css\bootstrap.min.css">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</Content>
<Content Update="wwwroot\index.html">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</Content>
<Content Update="wwwroot\js\bootstrap.min.js">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</Content>
</ItemGroup>
<ItemGroup>
<None Update="wwwroot\app.css">
<CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory>
</None>
<None Update="wwwroot\Query.razor">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</None>
<None Update="wwwroot\index.html">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</None>
</ItemGroup>
</Project>
I know from csproj that I added the wwwroot folder and some front-end files:
index.html is a template page, which introduces some css and js
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no" />
<title>Blazor app</title>
<base href="/" />
<link href="{PROJECT NAME}.styles.css" rel="stylesheet" />
<link href="app.css" rel="stylesheet" />
<link href="css/bootstrap.min.css" rel="stylesheet" />
</head>
<body>
<div id="app" class="container"></div>
<div id="blazor-error-ui">
An unhandled error has occurred.
<a href="" class="reload">Reload</a>
<a class="dismiss">🗙</a>
</div>
<script src="_framework/blazor.webview.js"></script>
<script src="js/bootstrap.min.js"></script>
</body>
</html>
css and js are not mentioned. They are front-end files. The focus is on query Razor, which realizes the main business logic, business data + UI performance
@using Microsoft.AspNetCore.Components.Web
@using Dapper
@using System.Data.SqlClient;
<div class="row">
<div class="col-1"></div>
<div class="col-10">
<div class="input-group mb-3">
<input type="text" class="form-control" id="zjm" placeholder="Please enter mnemonic code" @bind="ZJM" aria-describedby="button-addon2">
<button class="btn btn-outline-secondary" type="button" @onclick="GetGoods" id="button-addon2">query</button>
</div>
</div>
<div class="col-1"></div>
</div>
@code {
private string ZJM { get; set; }
List<Goods> list = new List<Goods>();
void GetGoods()
{
using (var con = new SqlConnection(System.Configuration.ConfigurationManager.ConnectionStrings["sqlcon"].ConnectionString))
{
if (string.IsNullOrWhiteSpace(ZJM))
{
list = con.Query<Goods>("select top 30 spid,spmch,shpchd,shpgg from spkfk ").ToList();
}
else
{
list = con.Query<Goods>("select top 30 spid,spmch,shpchd,shpgg from spkfk where zjm like @zjm ",new {zjm="%"+ZJM+"%" }).ToList();
}
}
}
}
<div class="row">
<table class="table table-striped table-hover">
<thead>
<tr class="table-dark">
<th scope="col">number</th>
<th scope="col">name</th>
<th scope="col">Place of Origin</th>
<th scope="col">Specifications</th>
</tr>
</thead>
<tbody>
@foreach (var item in list)
{
<tr>
<td>@item.spid</td>
<td>@item.spmch</td>
<td>@item.shpchd</td>
<td>@item.shpgg</td>
</tr>
}
</tbody>
</table>
</div>
How to introduce it into the form? This is a bit like the introduction of a webview in the previous winform, which is replaced by BlazorWebView.
using BlazorWinForm.wwwroot;
using Microsoft.AspNetCore.Components.WebView.WindowsForms;
using Microsoft.Extensions.DependencyInjection;
using System.Windows.Forms;
namespace BlazorWinForm
{
public partial class frmMain : Form
{
public frmMain()
{
InitializeComponent();
var serviceCollection = new ServiceCollection();
serviceCollection.AddBlazorWebView();
var blazor = new BlazorWebView()
{
Dock = DockStyle.Fill,
HostPage = "wwwroot/index.html",
Services = serviceCollection.BuildServiceProvider(),
};
blazor.AutoScroll = false;
blazor.RootComponents.Add<Query>("#app");
Controls.Add(blazor);
}
}
class Goods
{
public string spid { get; set; }
public string spmch { get; set; }
public string shpchd { get; set; }
public string shpgg { get; set; }
}
}
Overall, this kind of programming combines winform + c#+ HTML, CSS and JS, that is, it introduces rich front-end framework to make winform more flexible and powerful, and does not lose the control of cs architecture over the current computer (many industries connect the driver of proprietary devices through exe). winform's UI was previously enhanced through three-party UI controls such as devaxpress. Although there is WPF, it still turns in a circle drawn by Microsoft itself, and blazor brings a rich front-end library. In addition, unlike the previous winform embedded webview, the c#, in blazor, can easily interact with winform, communicate in the same language and communicate directly. For example, you can easily create a new form in the page of blazor.
Blazor into winform, is it chicken ribs or innovation? It should be that different people see different people, and wise people see different things.
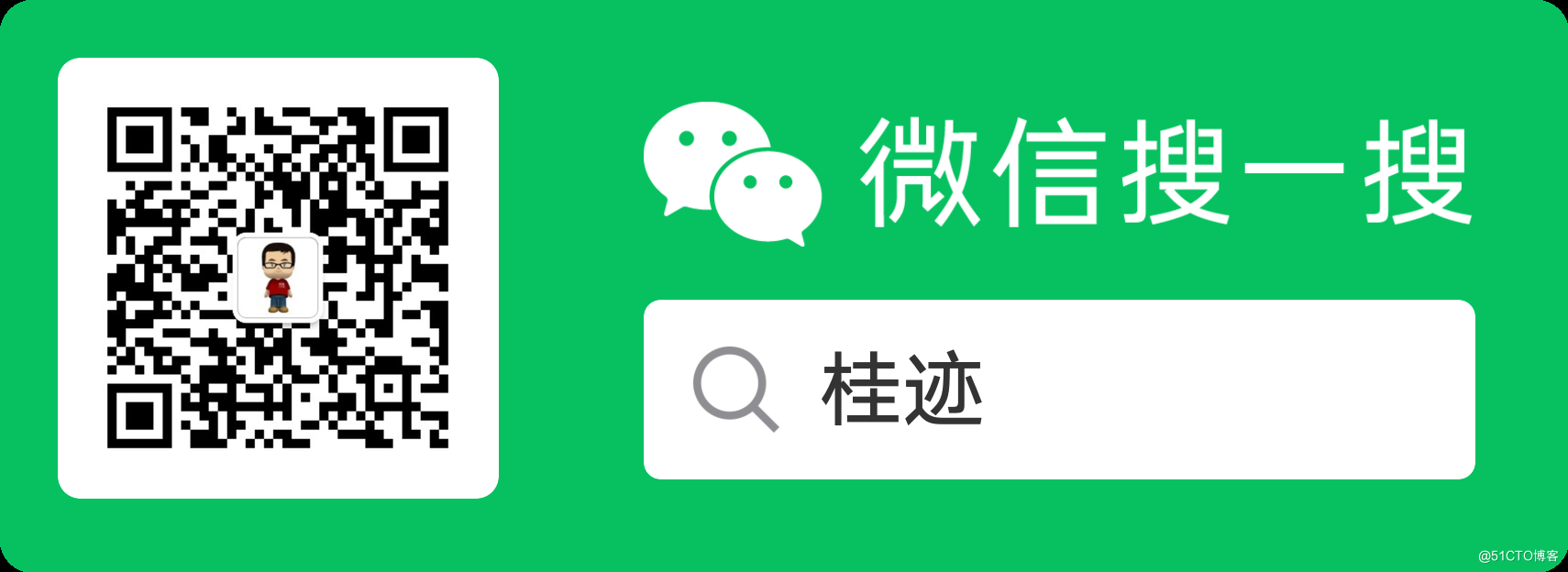