Preface
The official documents of Cocos Creator are very friendly, with two versions in English and Chinese.
[Strongly recommended] Beginners should read the official documents first. It also contains a lot of demo s.
Official Document Address: http://docs.cocos.com/creator/manual/zh/.
Today, I'd like to introduce you to the development process of Cocos Creator.
Next let's finish a simple drag-and-drop game.
Complete code
https://github.com/taoxhsmile/Cocos-Creator-demo1
development tool
- Cocos Creator
- Visual Studio Code
Visual Studio Code Configuration Document Address http://docs.cocos.com/creator/manual/zh/getting-started/coding-setup.html
text
1. Create a new project using Cocos Creator
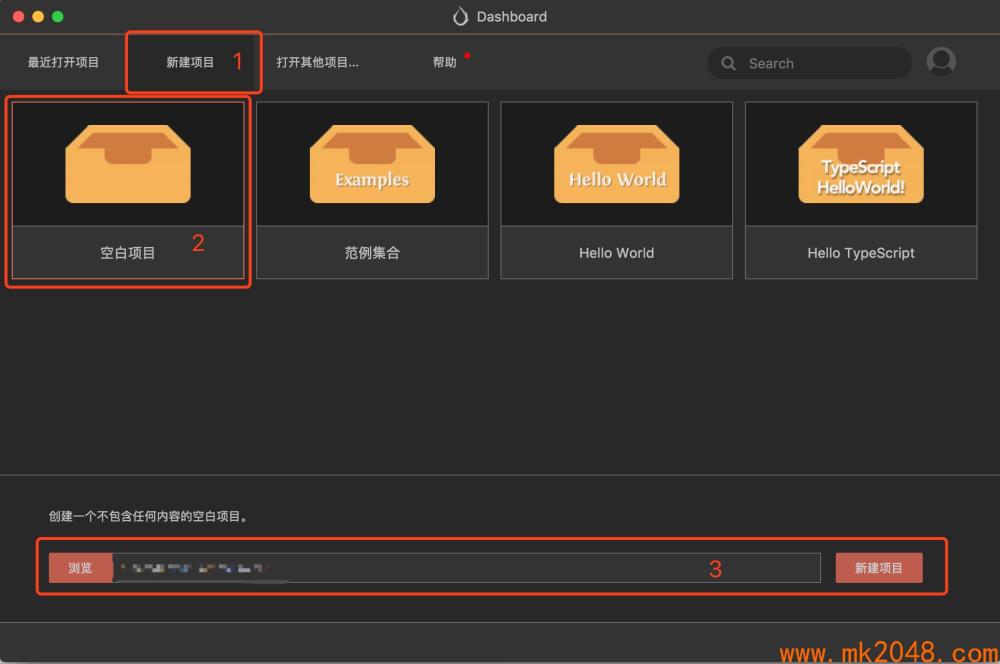
2. Create a scenario in assets named Drag

3. Double-click our scene.
4. Prepare a picture material and put it in assets.


6. Create a js file

7. Add js to the scenario

8. Open our project with Visual Studio Code

9. Write a script to add an attribute carNode to properties
*** properties: { carNode: { type: cc.Node, default: null } }, ***
10. Go back to Cocos Creator and find that the attributes just added appear in the editor.

11. Drag the car node to the location of the attribute value

12. Go back to Visual Studio Code and add drag events for car nodes
*** onLoad () { //Getting Car Node let { carNode } = this; //Adding variables to determine whether the user's mouse is currently pressed let mouseDown = false; //Record mouse click status when user clicks carNode.on(cc.Node.EventType.MOUSE_DOWN, (event)=>{ mouseDown = true; }); //Drag and drop only when the user presses the mouse carNode.on(cc.Node.EventType.MOUSE_MOVE, (event)=>{ if(!mouseDown) return; //Get the information of the last point from the mouse let delta = event.getDelta(); //Mobile Car Node carNode.x = carNode.x + delta.x; carNode.y = carNode.y + delta.y; }); //Restore state when mouse is raised carNode.on(cc.Node.EventType.MOUSE_UP, (event)=>{ mouseDown = false; }); }, ***
13. Cut back to Cocos Creator and click the "Run" button. Cocos Creator will help you open the browser and load the current game.

14. Try dragging the car to see if it's ready to move.
15. Some students may have found that the car will now be dragged outside the screen. We can try to add a restriction that the car can only be dragged inside the screen. Here is the implementation code:
*** onLoad () { //Getting Car Node let { carNode } = this; //Adding variables to determine whether the user's mouse is currently pressed let mouseDown = false; //Record mouse click status when user clicks carNode.on(cc.Node.EventType.MOUSE_DOWN, (event)=>{ mouseDown = true; }); //Drag and drop only when the user presses the mouse carNode.on(cc.Node.EventType.MOUSE_MOVE, (event)=>{ if(!mouseDown) return; //Get the information of the last point of the mouse distance let delta = event.getDelta(); //Adding qualifications let minX = -carNode.parent.width / 2 + carNode.width / 2; let maxX = carNode.parent.width / 2 - carNode.width / 2; let minY = -carNode.parent.height / 2 + carNode.height / 2; let maxY = carNode.parent.height / 2 - carNode.height / 2; let moveX = carNode.x + delta.x; let moveY = carNode.y + delta.y; //Controlling the range of movement if(moveX < minX){ moveX = minX; }else if(moveX > maxX){ moveX = maxX; } if(moveY < minY){ moveY = minY; }else if(moveY > maxY){ moveY = maxY; } //Mobile Car Node carNode.x = moveX; carNode.y = moveY; }); //Restore state when mouse is raised carNode.on(cc.Node.EventType.MOUSE_UP, (event)=>{ mouseDown = false; }); }, ***
16. Go back to Cocos Creator and click the "refresh" button. Then try again to see if the car can only be dragged on the screen now.
Attention points
- If you want to see the rendering on your mobile phone, you need to change the corresponding mouse event to touch event.
by:Tao