String class
summary
String is widely used in Java programming. In Java, string belongs to object. Java provides string class to create and operate string.
characteristic
- Immutability: string is a constant and cannot be changed after creation
- Sharability: string literals are stored in the string pool and can be shared. The string pool is located in the method area of the heap
Create string
There are two ways:
-
String str = "China";
In this way, an object is generated and stored in the string pool.
package com.ibelifly.commonclass.string; public class Test1 { public static void main(String[] args) { String s1="China"; System.out.println(s1); } }
-
String str2=new String("China");
This method produces two objects, one in the heap and one in the string pool.
package com.ibelifly.commonclass.string; public class Test1 { public static void main(String[] args) { String s2=new String("China"); System.out.println(s2); } }
Note: Although this method will generate two objects, in fact, the objects in the heap are not assigned to China, but directly point to China in the constant pool. This process can be simply understood in the following figure:
Detailed explanation of non variability and sharing
-
Immutability
package com.ibelifly.commonclass.string; public class Test1 { public static void main(String[] args) { String s1="China"; System.out.println(s1); System.out.println(s1.hashCode()); s1="China"; System.out.println(s1); System.out.println(s1.hashCode()); } }
Note: because the string is a constant and cannot be changed after creation, when s1 is re assigned to China, it will not change the value of the original China, but will re create a China constant in the constant pool (string pool). Therefore, the memory address pointed to by s1 will change and the returned hash value will change. We can briefly understand this process with the following figure:
-
Sharing
package com.ibelifly.commonclass.string; public class Test3 { public static void main(String[] args) { String s1="China"; System.out.println(s1); System.out.println(s1.hashCode()); String s2="China"; System.out.println(s2); System.out.println(s2.hashCode()); } }
Note: after declaring an s1 variable and creating a Chinese object, declaring an s2 variable again and giving the same Chinese string, because there are Chinese objects in the constant pool, a new object will not be created for s2, but the address of the existing Chinese object will be assigned to s2, so the hash values returned by s1 and s2 are the same. This process can be simply understood in the following figure:
However, when using the new keyword to create a string, even if the assignment is the same string, the addresses pointed to by the two variables are different, for example:
package com.ibelifly.commonclass.string; public class Test4 { public static void main(String[] args) { String s1=new String("China"); String s2=new String("China"); System.out.println(s1==s2); } }
This is because using the new keyword to create a string will also create objects in the heap. Each new object will open up new memory space. However, since there are Chinese objects in the constant pool, two new objects in the heap point to the Chinese data in the same constant pool. Illustration:
1. length() method
summary
The length() method returns the length of a string.
grammar
public int length()
give an example
package com.ibelifly.commonclass.string; public class Test5 { public static void main(String[] args) { String s="I love China"; System.out.println(s.length()); } }
2. charAt() method
summary
The charAt() method returns the character at the specified index. The index range is from 0 to length() - 1.
grammar
public char charAt(int index)
Index – the index of the character.
give an example
package com.ibelifly.commonclass.string; public class Test5 { public static void main(String[] args) { String s="I love China"; System.out.println(s.charAt(0)); } }
Note: when the index range is out of bounds, a string index out of bounds exception StringIndexOutOfBoundsException will be thrown.
3. contains() method
summary
The contains() method is used to determine whether the string contains the specified character or string.
grammar
public boolean contains(CharSequence chars)
chars – the character or string to judge.
Return value
Returns true if it contains the specified character or string, otherwise false.
give an example
package com.ibelifly.commonclass.string; public class Test5 { public static void main(String[] args) { String s="I love China"; System.out.println(s.contains("China")); System.out.println(s.contains("China")); } }
4. toCharArray() method
summary
The toCharArray() method converts a string to a character array.
grammar
public char[] toCharArray()
Return value
Character array.
give an example
package com.ibelifly.commonclass.string; import java.lang.reflect.Array; import java.util.Arrays; public class Test5 { public static void main(String[] args) { String s="I love China"; System.out.println(s.toCharArray()); System.out.println(Arrays.toString(s.toCharArray())); } }
5. lastIndexOf(int ch) method
summary
The lastIndexOf() method has the following four forms:
- public int lastIndexOf(int ch): returns the index of the last occurrence of the specified character in this string. If there is no such character in this string, it returns - 1.
- public int lastIndexOf(int ch, int fromIndex): returns the index of the last occurrence of the specified character in this string. Reverse search starts from the specified index. If there is no such character in this string, return - 1.
- public int lastIndexOf(String str): returns the index of the last occurrence of the specified substring in this string. If there is no such string in this string, it returns - 1.
- public int lastIndexOf(String str, int fromIndex): returns the index of the last occurrence of the specified substring in this string. Reverse search starts from the specified index. If there is no such character in this string, return - 1.
Note: the reverse search mentioned above refers to that in the search process, the string is retrieved from right to left from the specified position, but in the returned result, it still starts from the starting position (0) and calculates the last position of the sub string (or character) from left to right up to the specified index.
grammar
public int lastIndexOf(int ch) or public int lastIndexOf(int ch, int fromIndex) or public int lastIndexOf(String str) or public int lastIndexOf(String str, int fromIndex)
ch – character.
fromIndex – the index location to start the search.
str – substring to search.
Return value
Specifies the index value of the substring at the first occurrence in the string.
give an example
package com.ibelifly.commonclass.string; import java.lang.reflect.Array; import java.util.Arrays; public class Test5 { public static void main(String[] args) { String s="I love China"; System.out.print("Find the last place where the character appears :"); System.out.println(s.lastIndexOf('I')); System.out.print("Find the last position of the character from the first position :"); System.out.println(s.lastIndexOf('I',1)); System.out.print("The last occurrence of the substring in China:"); System.out.println(s.lastIndexOf("China")); System.out.print("Search for the last occurrence of the substring in China from 2 positions :"); System.out.println(s.lastIndexOf("China",2)); } }
6. trim() method
summary
The trim() method is used to delete the leading and trailing whitespace of the string.
grammar
public String trim()
Return value
Delete a string with leading and trailing whitespace characters.
give an example
package com.ibelifly.commonclass.string; public class Test7 { public static void main(String[] args) { String s=" Hello world "; System.out.println(s.trim()); } }
7. toUpperCase() and toLowerCase() methods
summary
- The toUpperCase() method converts all characters of the string to uppercase.
- The toLowerCase() method converts all characters of the string to lowercase.
grammar
public String toUpperCase() public String toLowerCase()
Return value
Converted string.
give an example
package com.ibelifly.commonclass.string; public class Test7 { public static void main(String[] args) { String s="Hello World"; System.out.println(s.toUpperCase()); System.out.println(s.toLowerCase()); } }
8. endsWith() and startsWith() methods
summary
- The endsWith() method tests whether the string ends with the specified suffix.
- The startsWith() method detects whether the string starts with the specified prefix.
grammar
public boolean endsWith(String suffix) public boolean startsWith(String prefix)
Suffix – the suffix specified.
Prefix – the prefix specified.
Return value
endsWith(): returns true if the character sequence represented by the parameter is the suffix of the character sequence represented by this object; Otherwise, false is returned. Note that if the parameter is an empty String or equal to this String object (determined by the equals(Object) method), the result is true.
startsWith(): returns true if the string starts with the specified prefix; Otherwise, false is returned.
give an example
package com.ibelifly.commonclass.string; public class Test7 { public static void main(String[] args) { String s="HelloWorld.java"; System.out.println(s.endsWith(".java")); System.out.println(s.endsWith("")); System.out.println(s.endsWith("HelloWorld.java")); System.out.println(s.endsWith(".jav")); System.out.println(s.startsWith("h")); } }

9. replace() method
summary
The replace() method replaces all searchChar characters that appear in the string with the newChar character and returns the replaced new string.
grammar
public String replace(char searchChar, char newChar)
searchChar – original character.
newChar – new character.
Return value
New string generated after replacement.
give an example
package com.ibelifly.commonclass.string; public class Test8 { public static void main(String[] args) { String s="Java is the best programming language.Java is great."; System.out.println(s.replace("Java","Python")); } }
10. split() method
summary
The split() method splits the string based on matching the given regular expression.
Note:., $| And ***** and other escape characters must be added \.
**Note: * * multiple separators, you can use | as a hyphen.
grammar
public String[] split(String regex, int limit)
regex – regular expression separator.
limit – number of copies divided.
Return value
String array.
give an example
package com.ibelifly.commonclass.string; public class Test9 { public static void main(String[] args) { String s1 ="Welcome-to-learn-JAVA"; System.out.println("- Separator return value :" ); for (String str: s1.split("-")){ System.out.println(str); } System.out.println(""); System.out.println("- Separator sets the return value of the number of split copies :" ); for (String str: s1.split("-", 4)){ System.out.println(str); } System.out.println(""); String s2 ="www.JAVA.com"; System.out.println("Escape character return value :" ); for (String str: s2.split("\\.", 3)){ System.out.println(str); } System.out.println(""); String s3 = "account=? and uu =? or n=?"; System.out.println("Multiple separator return values :" ); for (String str: s3.split("and|or")){ System.out.println(str); } } }
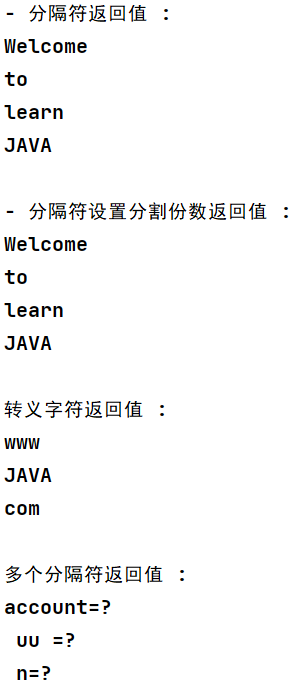
11. equals() and equalsIgnoreCase() methods
summary
- The equals() method compares the String with the specified object. The equals() method is overridden in the String class to compare whether the contents of two strings are equal.
- The equalsIgnoreCase() method is used to compare the string with the specified object, regardless of case.
grammar
public boolean equals(Object anObject) public boolean equalsIgnoreCase(String anotherString)
anObject – the object to compare with the string.
Return value
Returns true if the given object is equal to the string; Otherwise, false is returned.
give an example
package com.ibelifly.commonclass.string; public class Test10 { public static void main(String[] args) { String s1="hello"; String s2="HELLO"; System.out.println(s1.equals(s2)); System.out.println(s1.equalsIgnoreCase(s2)); } }
12. compareTo() method
summary
The compareTo() method compares two strings in dictionary order.
grammar
int compareTo(String anotherString)
Otherstring – the string to compare.
Return value
The return value is an integer. It first compares the size of the corresponding character (ASCII code order). If the first character and the first character of the parameter are different, the comparison ends and returns the length ASCII code difference between them. If the first character and the first character of the parameter are equal, the second character and the second character of the parameter are compared, and so on, Until one of the compared characters or the compared characters ends.
- If the parameter string is equal to this string, the return value is 0;
- If the string is less than the string parameter, a value less than 0 will be returned;
- If this string is greater than the string parameter, a value greater than 0 is returned.
explain:
If the first character is different from the first character of the parameter, the comparison ends and the ASCII difference of the first character is returned.
If the first character and the first character of the parameter are equal, compare the second character with the second character of the parameter, and so on until they are unequal, and return the ASCII code difference of the character. If two strings are not of the same length and the corresponding characters are exactly the same, the length difference between the two strings is returned.
give an example
package com.ibelifly.commonclass.string; public class Test11 { public static void main(String[] args) { String s1="abc"; String s2="bcd"; String s3="abc123"; System.out.println(s1.compareTo(s2)); System.out.println(s2.compareTo(s1)); System.out.println(s1.compareTo(s3)); } }
13. substring() method
summary
The substring() method returns a substring of a string.
grammar
public String substring(int beginIndex) or public String substring(int beginIndex, int endIndex)
beginIndex – the starting index (including), which starts from 0.
endIndex – end index (not included).
give an example
package com.ibelifly.commonclass.string; public class Test12 { public static void main(String[] args) { String s = "This is text"; System.out.println(s.substring(5)); System.out.println(s.substring(5, 10)); } }
Reference video: https://www.bilibili.com/video/BV1vt4y197nY?p=20
Reference article: https://www.runoob.com/java/java-string.html