Compilation and call of boost library under Windows platform [turn]
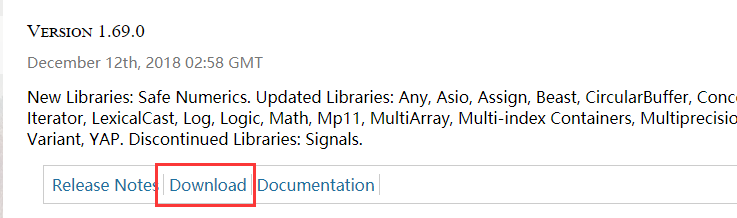
- Download a compressed package
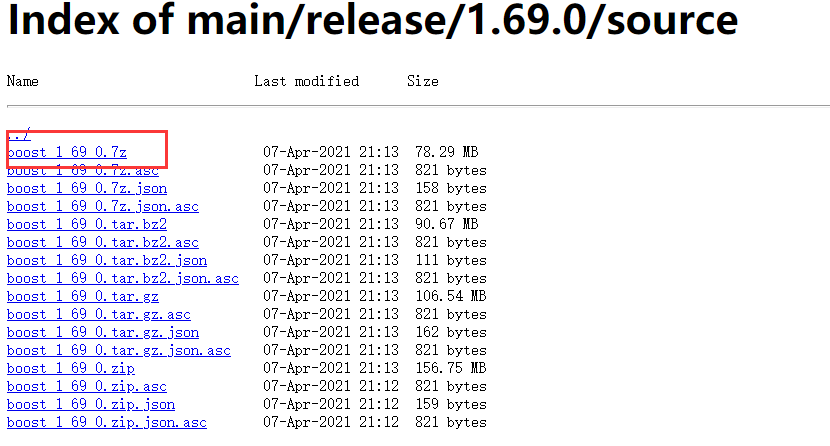
compile
- Open the command line window of VS
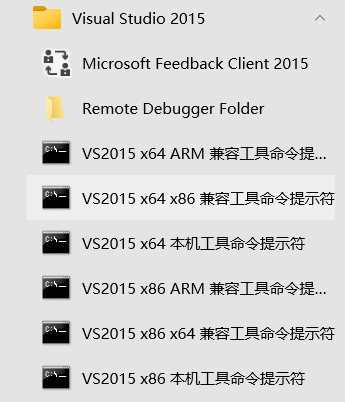
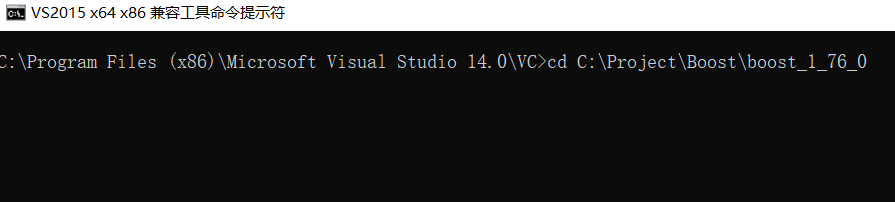
- The command line window executes bootstrap bat
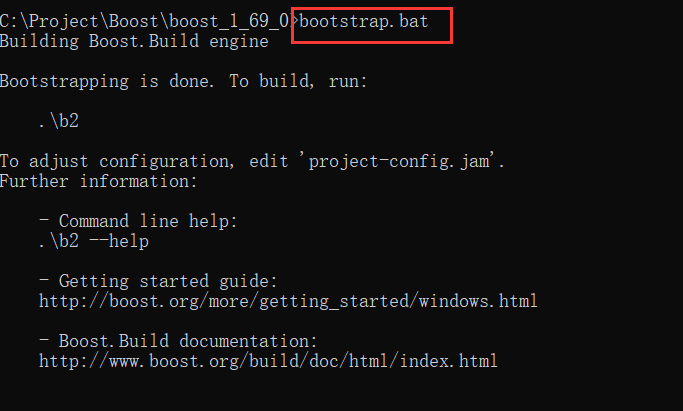
- Run bootstrap. From the command line Bat, will produce bjam in the root directory exe,b2. Exe (upgraded version of bjam), project config jam,bootstrap.log four files
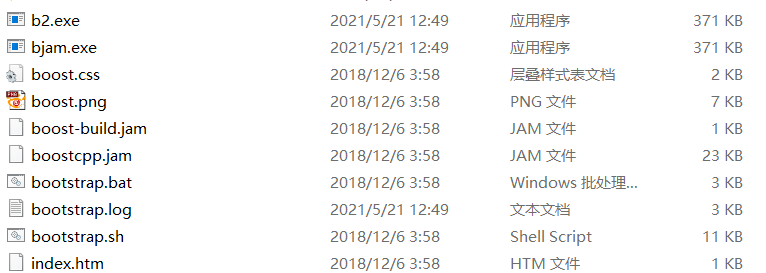
- The command line required to compile the bjam Library
//Example - compile a boost library other than the boost::python Library
bjam stage --toolset=msvc-14.0 --without-python --stagedir="C:\Project\Boost" link=static runtime-link=shared threading=multi address-model=32
- A lib directory will be generated under C:\Project\Boost, which is the Lib Library of the compiled boost
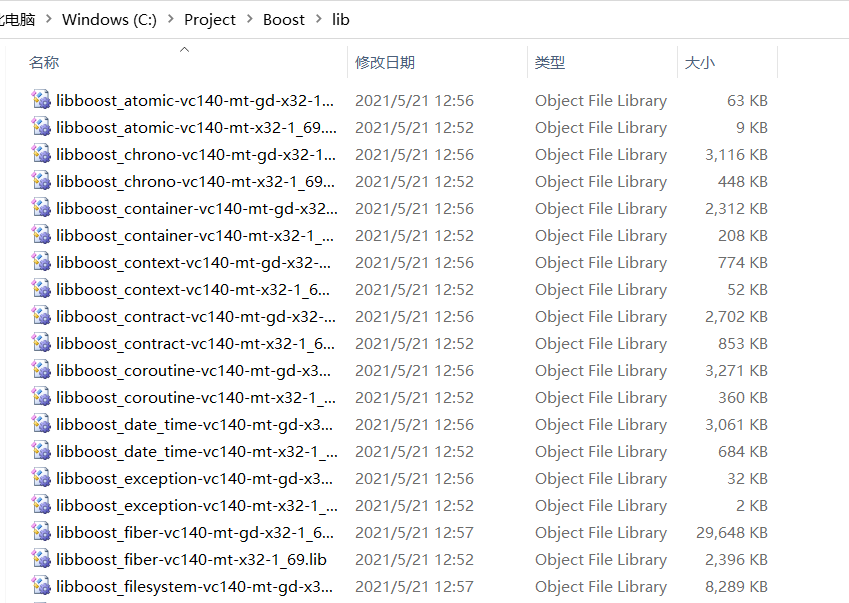
bjam compilation parameter description
- stage/install:stage means that only libraries are generated, and install will also generate the include directory containing header files, but the compilation time is long; The default is stage.
- --stagedir/prefix: stagedir is used during stage and prefix is used during install, indicating the path of the compiled generated file.
bjam install --toolset=msvc-14.0 --without-python --prefix="C:\Project\Boost" link=static runtime-link=shared threading=multi address-model=64
- --Build type: complete compile all boost libraries; The default is complete.
bjam stage --toolset=msvc-14.0 --build-type=complete --stagedir="C:\Project\Boost" link=static runtime-link=shared threading=multi address-model=64
- --without/with: select which libraries to not compile / compile. The default is to compile all.
- The command to view the boost library is bjam -- show libraries
//boost::python lib
bjam stage --toolset=msvc-14.0 --with-python --stagedir="C:\Project\Boost" link=static threading=multi address-model=64
- --toolset: Specifies the compiler, which is optional, such as borland, gcc, msvc-14.0 (VS2025), etc.
- Link: generate dynamic link library / static link library.
- static only generates lib files.
- shared generates lib files and dll files.
bjam stage --toolset=msvc-14.0 --build-type=complete --stagedir="C:\Project\Boost" link=shared runtime-link=shared threading=multi address-model=64
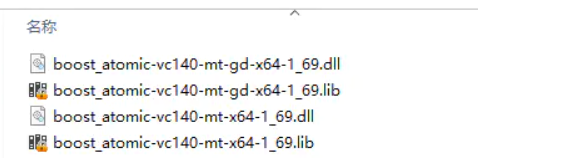
- Runtime link: dynamically / statically link C/C + + runtime libraries. There are also two methods: shared and static.
- threading: single / multithreaded compilation. Now it's basically multi-mode.
- Address model: 64 bit platform or 32-bit platform. If it is not filled in, the libraries of both platforms will be compiled.
- debug/release: debug version, release version. If you don't fill in, both versions of the library will be compiled.
bjam stage --toolset=msvc-14.0 --with-atomic --stagedir="C:\Project\Boost" link=static threading=multi address-model=64 debug
Naming characteristics of boost library
//link=static runtime-link=shared
libboost_atomic-vc140-mt-gd-x64-1_69.lib
libboost_atomic-vc140-mt-x64-1_69.lib
//link=static runtime-link=static
libboost_atomic-vc140-mt-sgd-x64-1_69.lib
libboost_atomic-vc140-mt-s-x64-1_69.lib
//link=shared runtime-link=shared
boost_atomic-vc140-mt-gd-x64-1_69.dll
boost_atomic-vc140-mt-gd-x64-1_69.lib
boost_atomic-vc140-mt-x64-1_69.dll
boost_atomic-vc140-mt-x64-1_69.lib
- Beginning_ Library name - compiler version - [multithreading] - [static version] [debug version] - platform - version number
- Beginning: the version starting with "lib" is "link=static" (static link library version without dll), while the version starting directly with "boost" is "link=shared" (dynamic link library version, including lib and dll).
- Library name: followed by the name of boost library (such as date_time Library).
- Compiler version: use "-" instead of the underscore "" between and the library name Separation (e.g. - vc140).
- [multithreading]: the version with "mt" is "threading=multi", and the version without this item is "threading=single".
- [static version]: the version with "s" is "runtime link = static". Without this item, it is "runtime link = shared".
- [debug version]: those with "gd" are the debug version, while those without "gd" are the release version.
- Platform: x64 or x32
- Version number: all libraries contain the end of the version number of the boost library
Combination of link parameter and runtime link
link | runtime-link | Operation requirements | Apply project settings |
---|
static | static | libboost_atomic-vc140-mt-sgd-x64-1_69.lib libboost_atomic-vc140-mt-s-x64-1_69.lib | /MTd/MT |
static | shared | libboost_atomic-vc140-mt-gd-x64-1_69.lib libboost_atomic-vc140-mt-x64-1_69.lib | /MDd/MD |
shared | shared | boost_atomic-vc140-mt-gd-x64-1_69.dll boost_atomic-vc140-mt-gd-x64-1_69.lib boost_atomic-vc140-mt-x64-1_69.dll boost_atomic-vc140-mt-x64-1_69.lib | |
shared | static | There is no such combination | |
Usage configuration of VS Project
- VS establishing win32 console project
- Project settings - Properties (current solution)
- VC + + directories:: include directory plus boost root directory
- VC + + directories:: Library directories plus lib directory compiled by boost
- If you connect the boost::python and boost::numpy libraries in a static way, you need to add a static ID before including these two modules
#define BOOST_PYTHON_STATIC_LIB
#define BOOST_NUMPY_STATIC_LIB
#include <boost/python.hpp>
#include <boost/python/numpy/ndarray.hpp>
Examples
#include <iostream>
#include <boost/format.hpp>
#include <iomanip>
int main()
{
std::cout << boost::format("%s:%d+%d=%d\n") % "sum" % 1 % 2 % (1 + 2);
boost::format fmt("(%1% + %2%) * %2% = %3%\n");
fmt % 2 % 5 %((2+5)*5);
std::cout << fmt.str();
fmt.parse("%|05d|\n%|-8.3|f\n%| 10S|\n%|05X|\n");
std::cout << fmt % 62 % 2.236 % "123456789" % 48;
fmt.clear();
//std::cout << fmt.str(); //error after call clear function
std::cout << fmt % 56 % 1.125 % "987654321" % 32;
boost::format fmtPro("%1% %2% %3% %2% %1% \n");
std::cout << fmtPro % 1 % 2 % 3;
fmtPro.bind_arg(2, 10);
std::cout << fmtPro % 1 % 3;
fmtPro.clear();
std::cout << fmtPro % boost::io::group(std::showbase, std::oct, 111) % 333;
fmtPro.clear_binds();
fmtPro.modify_item(1, boost::io::group(std::hex, std::right, std::showbase, std::setw(8), std::setfill('*')));
std::cout << fmtPro % 49 % 20 % 100;
std::cout << 1 / 2;
return 0;
}
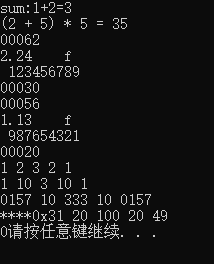