=====
@Target
It is added to the Annotation through @ target to explain the object scope modified by Annotation: Annotation can be used for packages Types (classes, interfaces, enumerations, Annotation types), type members (methods, construction methods, member variables, values), method parameters and local variables (such as loop variables and catch parameters). Using @ target in the declaration of Annotation types can make it more clear what the modified target is, where it is used and what it is modified, as shown in the following common ways.
-
CONSTRUCTOR: used to describe the CONSTRUCTOR
-
FIELD: used to describe the FIELD
-
LOCAL_VARIABLE: used to describe local variables
-
METHOD: used to describe the METHOD
-
PACKAGE: used to describe a PACKAGE
-
PARAMETER: used to describe parameters
-
TYPE: used to describe class, interface (including annotation TYPE) or enum declaration
The following code
@Target({ElementType.FIELD,ElementType.METHOD}) //Use {} to enclose multiple, and then separate them with commas public @interface TargetTest { }
@Retention
This annotation represents a reserved state when we run or compile, indicating the length of time to retain annotations with annotation types.
-
SOURCE: valid in the SOURCE file (i.e. the SOURCE file is reserved). Discard it at compile time.
-
class: it is valid in the class file (i.e. class is reserved) and will not be added to the JVM
-
RUNTIME: valid at RUNTIME (i.e. reserved at RUNTIME). This is consistent with our common loading. It will be loaded into the JVM at RUNTIME. It is generally available through reflection. Generally, this is our common loading.
@Retention(RetentionPolicy.RUNTIME)//When using this annotation, only one attribute can be selected, and multiple attributes cannot be defined public @interface RetentionTest { }
@Documented
@Documented is used to describe that other types of annotation s should be used as a public API for annotated program members.
The following code
@Documented //Add document annotation @Target(ElementType.FIELD) @Retention(RetentionPolicy.RUNTIME) public @interface AnnotationTest { }
@Inherited:
@Inherited meta annotation is a tag annotation, @ inherited describes that a marked type is inherited. If an annotation type decorated with @ inherited is used for a class, the annotation will be used for the subclass of the class.
@Documented @Target(ElementType.FIELD) @Retention(RetentionPolicy.RUNTIME) @Inherited //Add inheritance annotation public @interface AnnotationTest { }
@Repeatable
This is an annotation feature added in Java 8. The value of @ Repeatable represents the containing annotation type of Repeatable annotation type.
@Target({ElementType.TYPE,ElementType.METHOD}) @Retention(RetentionPolicy.RUNTIME) public @interface Repeatables { RepeatablesTest[] value(); } @Repeatable(Repeatables.class) public @interface RepeatablesTest { String value() default "Ha ha ha"; }
example
==
We briefly introduce the five commonly used annotation elements of annotation, and solve them. We implement annotation through examples.
The code is as follows. We will test according to the above tips.
Before looking at the examples, let's look at some common methods.
-
Class.getAnnotations() gets all annotations, both self declared and inherited
-
Class. Getannotation (class < a > annotationclass) gets the specified annotation, which can be self declared or inherited
-
Class. Getdeclaraedannotations() gets the annotations declared by itself
-
Class.getDeclaredField(String name); // Gets the declaration field of the class
-
Class.getDeclaredMethods();// Returned is a Method [] array
@Documented @Target({ElementType.TYPE,ElementType.METHOD}) @Retention(RetentionPolicy.RUNTIME) public @interface AnnotationTest { String name() default "Xiao Ming"; //Indicates that Xiaoming is the default. }
@AnnotationTest() / / add annotations to this class
public class TestAnnotationMain {
public static void main(String[] args) { boolean hasAnnotation = TestAnnotationMain.class.isAnnotationPresent(AnnotationTest.class); if (hasAnnotation) { AnnotationTest annotation = TestAnnotationMain.class.getAnnotation(AnnotationTest.class); System.out.println(annotation.name()); } }
}
Printout results:  If we want to change name You can do this
@AnnotationTest(name = "Xiao Gang")
public class TestAnnotationMain {
public static void main(String[] args) { boolean hasAnnotation = TestAnnotationMain.class.isAnnotationPresent(AnnotationTest.class); if (hasAnnotation) { AnnotationTest annotation = TestAnnotationMain.class.getAnnotation(AnnotationTest.class); System.out.println(annotation.name()); } }
}
 If we want to assign a value to a class's attribute, we can do so 1.Create an annotation as follows
@Documented
@Retention(RetentionPolicy.RUNTIME)
public @interface AnnotationTest1 {
String value(); //value
}
2.Reference this master solution
public class Test {
@AnnotationTest1(value = "Xiao Yun") //Reference annotation for assignment public String name;
}
3.test
public class TestAnnotation1Main {
public static void main(String[] args) { try { Field name = Test.class.getDeclaredField("name");//Fields of this class name.setAccessible(true); AnnotationTest1 annotationTest1 = name.getAnnotation(AnnotationTest1.class);//Gets the annotation for the field if (annotationTest1 != null) { System.out.println(annotationTest1.value()); //Output value } } catch (NoSuchFieldException e) { e.printStackTrace(); } }
}
> Get the annotation class on the method, such as AnnotationTest2
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface AnnotationTest2 {
//todo has no methods or properties
}
public class Test { @AnnotationTest1(value = "Xiao Yun") public String name; @AnnotationTest2 //Objective to get the AnnotationTest2 public void fun() { System.out.println("Method execution"); } } ``` obtain ``` # **Reader welfare** **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** 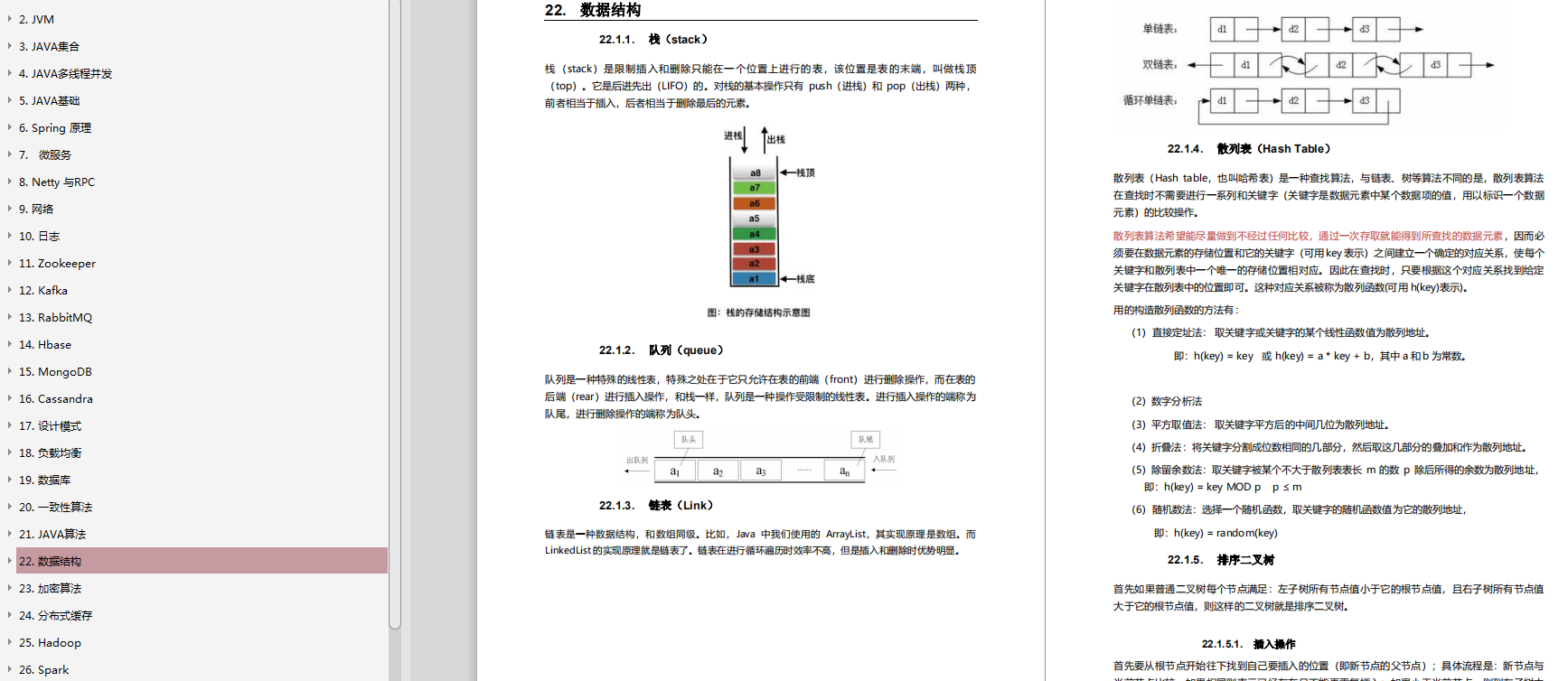 **More notes to share** 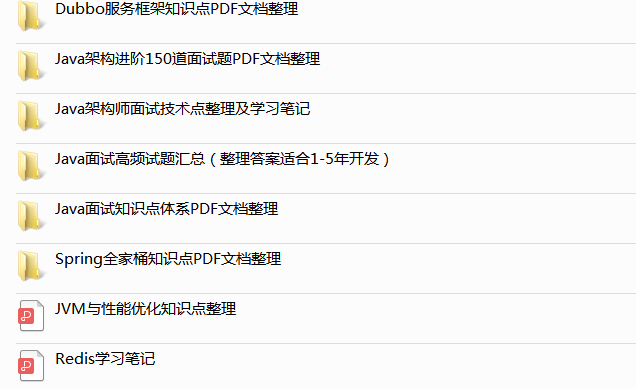 cloud") public String name; @AnnotationTest2 //Objective to get the AnnotationTest2 public void fun() { System.out.println("Method execution"); } } ``` obtain ``` # **Reader welfare** **[CodeChina Open source project: [first tier big factory] Java Analysis of interview questions+Core summary learning notes+Latest explanation Video]](https://codechina.csdn.net/m0_60958482/java-p7)** [External chain picture transfer...(img-1qpQB7tu-1630903078199)] **More notes to share** [External chain picture transfer...(img-MBVVU3uy-1630903078200)]