Time: August 31, 2021
1. Procedure flow chart
- Concept: a way to express program control structure
- It is used for program analysis and process description of key parts. It is composed of a series of graphics, flow lines and text descriptions.
graphical | notes |
---|
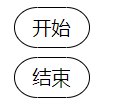 | Start stop box: indicates the beginning or end of program logic |
 | Processing box: represents a group of processing procedures, corresponding to the program logic executed in sequence |
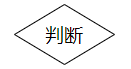 | Judgment box: indicates a judgment condition and selects different execution paths according to the judgment results |
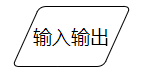 | Input / output box: indicates the data input or result output in the program |
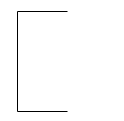 | Comment box: indicates the comment of the program |
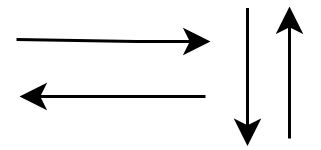 | Flow direction line: represents the control flow of the program, and the execution path of the program is expressed by a straight line or curve with arrow |
 | Connection point: indicates the connection method of multiple flow charts. It is often used to form multiple smaller flow charts into larger flow charts |
2. Program control structure foundation
name | notes | flow chart |
---|
Sequential structure | The program is executed in linear order | 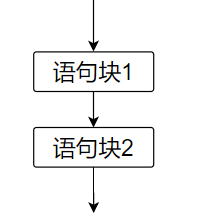 |
Branching structure | The program selects different forward execution according to the conditional judgment results | 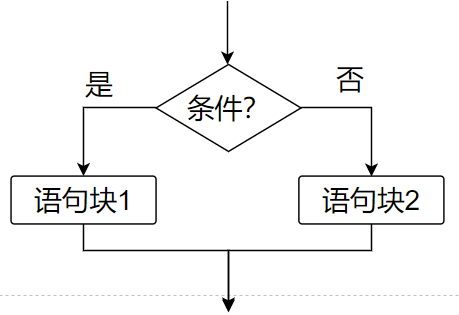 |
Cyclic structure | The program executes backward according to the condition judgment result | 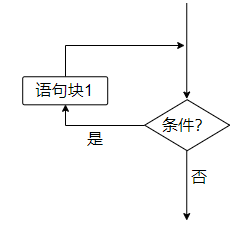 |
2.1 branch structure of program
2.1. 1. Single branch structure: if
grammar | flow chart |
---|
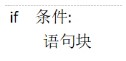 | 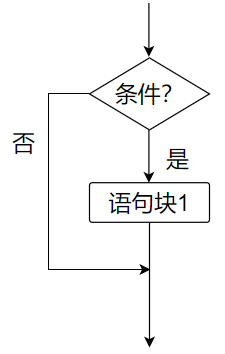 |
- A condition is a statement block that produces a True or False result. When the result is True, the statement block is executed, otherwise the statement block is skipped.
# Judge whether the number entered by the user is even
num = eval(input('Please enter an integer:'))
if num % 2 ==0:
print('This is an even number')
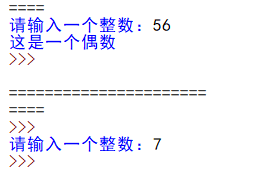
- If there are multiple conditions, multiple conditions are logically combined by and or. And represents the relationship between multiple conditions and, or represents the relationship between multiple conditions or
# Judge the characteristics of the number entered by the user
num = eval(input('Please enter an integer:'))
if num%2==0 and num%3==0:
print('This is a number divisible by 2 and 3')
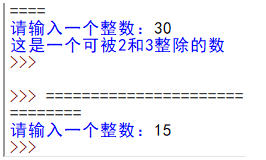
2.1. 2. Double branch structure: if else
grammar | flow chart |
---|
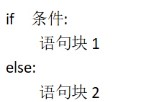 | 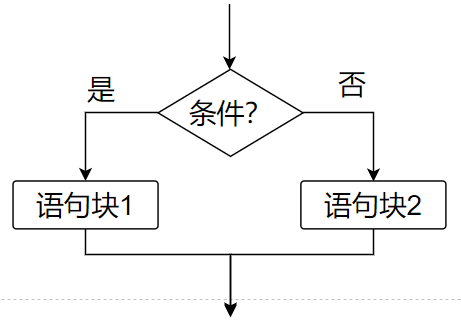 |
# Judge whether the number entered by the user is even
num = eval(input('Please enter an integer:'))
if num % 2 ==0:
print('This is an even number')
else:
print('This is not an even number')
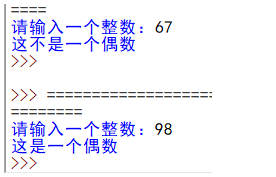
There is also a concise expression of double branch structure, which is abbreviated as conditional expression
- Syntax: expression 1 if conditional else expression 2
# Judge whether the number entered by the user is even
num = eval(input('Please enter an integer:'))
result='yes'if num%2==0 and num%3==0 else'no'
print('this{}A number divisible by 2 and 3'.format(result))
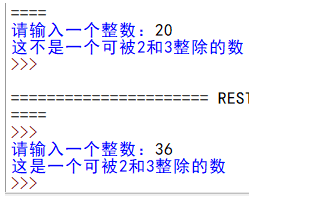
- Differences between expressions and statements:
An expression is a code fragment that generates or evaluates a new data value. It is not a complete statement itself
Example: 99 + 1 is an expression, and a = 99 + 1 is a statement
2.1. 3 multi branch structure: if elif else
grammar | flow chart |
---|
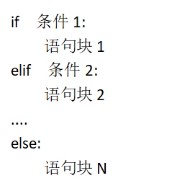 | 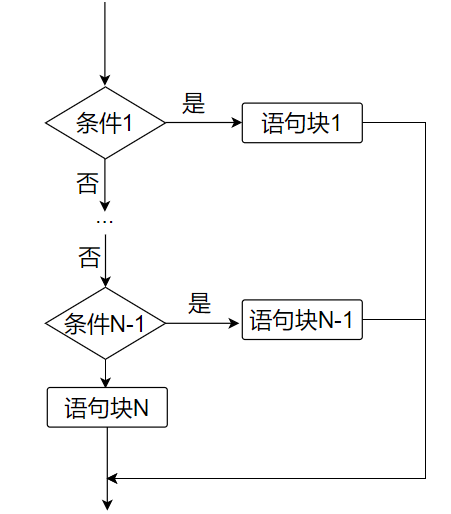 |
- When using multi branch structure to write code, we should pay attention to the sequence of multiple logical conditions
- 70, 80 and 90 are greater than 60, the branch ends at the beginning, and the logic is wrong
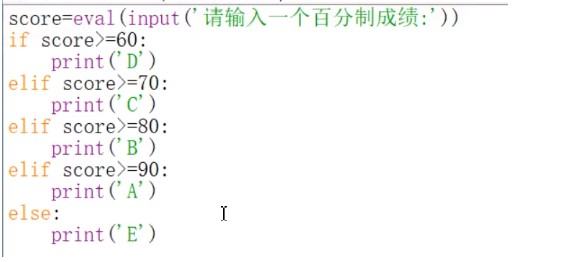
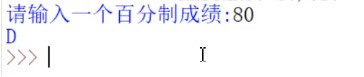
score = int(float(input('Please enter your score:')))
if 100 >= score > 90:
print('A')
elif score > 80:
print('B')
elif score > 70:
print('C')
elif score >= 60:
print('D')
elif score >= 0:
print('fail,')
else:
print('The result is illegal')
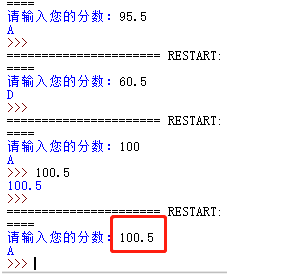
2.1. 4 judgment conditions and combination
- The judgment condition in the branch structure can use any statement or function that can produce True or False.
- In python, for any non-zero value, the Boolean value of non empty data type is True, and the Boolean value of 0 or empty type is False
Operator | Operator meaning |
---|
< | less than |
<= | Less than or equal to |
>= | Greater than or equal to |
> | greater than |
== | be equal to |
!= | Not equal to |
- python uses and, or, not to perform logical operations or combinations on conditions
- And logic and, each sub condition is true, and the final result is true
- Or logic or, one of the sub conditions is true, and the final result is true
- Not logic is not, the sub condition is false, and the final result is true
2.2 loop structure of program
2.2. 1 traversal loop: for
- Use the keyword for to extract each element of the traversal structure in turn for processing
- It can be understood that the elements are extracted from the traversal structure, placed in the loop variable, and the statement block is executed once for each extracted element.
grammar | flow chart |
---|
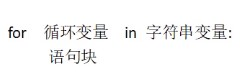 | 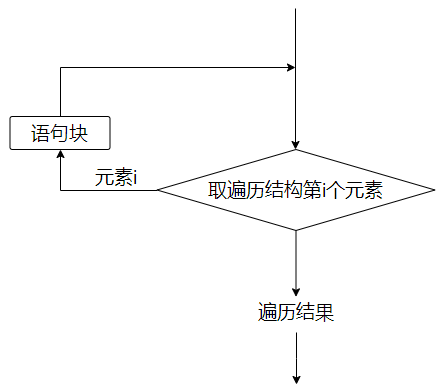 |
for Cyclic variable in String variable:
Statement block
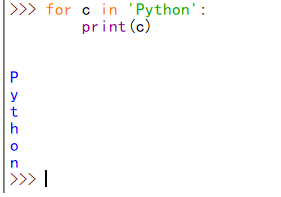
- Using the range() function, you can specify the number of cycles of the statement block:
for Cyclic variable in range(Number of cycles):
Statement block
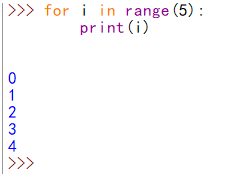
- Traversal loop extension: for else structure
- When the for loop executes normally, the program will continue to execute the contents of the else statement. Else statements end only after the loop executes normally.
for s in 'Python':
print('Loop execution:'+s)
else:
s = 'The cycle ends normally'
print(s)
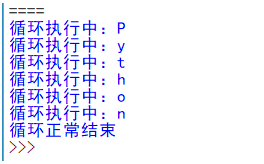
2.2. 2 infinite loop: while
- Use the keyword while to execute the program according to the judgment conditions
grammar | flow chart |
---|
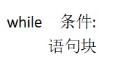 | 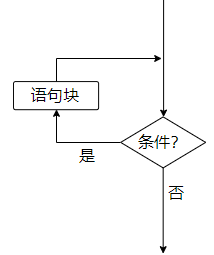 |
- When the program executes the while statement, if the judgment condition is True, execute the loop body statement, and return the condition for judging the while statement again after the statement is completed;
- When the condition is False, the loop terminates and subsequent statements indented at the same level as while are executed.
n = 0
while n<23:
print(n)
n = n+5
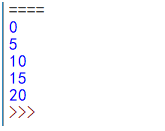
- Infinite loop expansion: while else
while condition:
Statement block 1
else:
Statement block 2
# s = 'python'
# num = 0
s,num = 'Python',0
while num < len(s):
print('Loop execution:',s[num])
num += 1
else:
s = 'The cycle ends normally'
print(s)
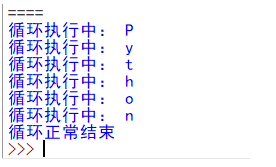
2.2. 3 cycle control: break and continue
- The loop structure has two auxiliary loop control Keywords: break and continue
- Break is used to jump out of the innermost for or while loop, break away from the subsequent program of the loop and continue to execute the subsequent code of the loop
while True:
s = input('input Q Can exit')
if s == 'Q':
break
print(s)
print('Exit program')
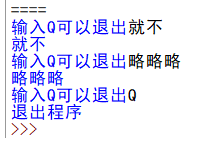
- If there are two or more loops, break exits the innermost loop
while True:
s = input('input Q Can exit')
if s == 'Q':
break # Exit the while loop
for c in s:
if c == 'q':
break # Exit for loop
print(c)
print('Exit program')

- continue is used to end the current loop, that is, to jump out of the following unexecuted statements in the loop body, but not out of the current loop, as follows: the output of "y" is skipped, but not out of the loop
for s in 'Python':
if s == 'y':
continue
print(s,end='')
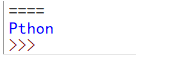
for s in 'Python':
if s == 'y':
break
print(s,end='')
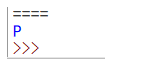
- Summary:
- The continue statement only ends this cycle and does not affect subsequent cycles
- The break statement jumps out of the loop and affects subsequent loops
3. Program exception handling
- python programs generally have certain requirements for input, but the actual input does not meet the requirements from time to time, which may lead to the operation error of the program.
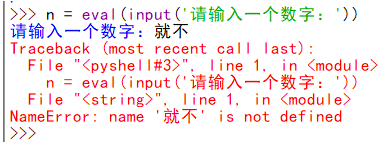
analysis:
- Because the eval() function is used, an error will be reported if the user does not enter a number
- There are many possibilities for such errors caused by input and expectation mismatches
- In order to ensure the stability of program operation, such operation errors should be captured and reasonably controlled by the program
- Syntax:
try:
Statement block 1
except:
Statement block 2
Parsing: statement block 1 is the program content normally executed. When an exception occurs during the execution of this statement block, statement block 2 after except will be executed
try:
n=eval(input('Enter an integer:'))
print('The third power of the input number is:',n**3)
except:
print('Illegal input, please re-enter an integer!')
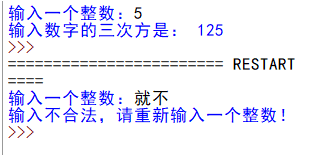
- In addition to input, exception handling can also handle running exceptions in program execution
for i in range(5):
print(10/i,end= '')
Parsing: traverse from 0, 0 cannot be used as divisor, operation error
try:
for i in range(10):
print(10/i)
except:
print('Divisor cannot be 0')
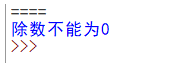
- The program can also capture specific error types for processing.
try:
a = eval(input('Please enter the divisor:'))
b = eval(input('Please enter divisor:'))
print(a/b)
except ZeroDivisionError:
print('0 Cannot be a divisor')
except:
print('Other errors!')
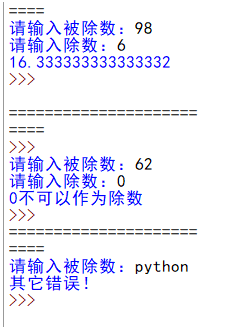
4. Example analysis: number guessing game
- Write a "number guessing game" program, randomly generate a number between 1-100, and then ask the user to guess the number repeatedly. For each answer, only answer "guess big" or "guess small" until it is correct, and output the user's guess times.
- In order to generate random numbers, you need to use python's random number standard library random.
# Guess the number
import random
target=random.randint(1,100)
count=0
while True:
guess=eval(input('Please enter a guess integer (1)-100 Between)'))
count+=1
if guess>target:
print('Guess big')
elif guess<target:
print('Guess it's small')
else:
print('You guessed right')
break
print('The number of guesses in this round is:',count)
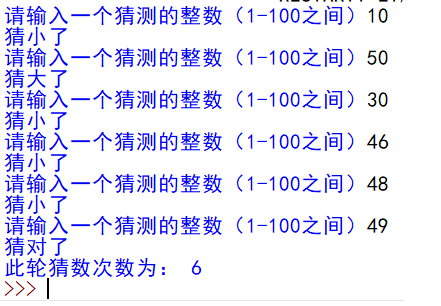
- Because the eval() method is used to obtain user input, if the user enters a non numeric value, resulting in a running error, the program will exit. In order to increase the robustness of the program, an exception handling mechanism needs to be added.
# Guess the number
import random
target=random.randint(1,100)
count=0
while True:
try:
guess=eval(input('Please enter a guess integer (1)-100 Between)'))
except:
print('Input error, please re-enter')
continue
count+=1
if guess>target:
print('Guess big')
elif guess<target:
print('Guess it's small')
else:
print('You guessed right')
break
print('The number of guesses in this round is:',count)
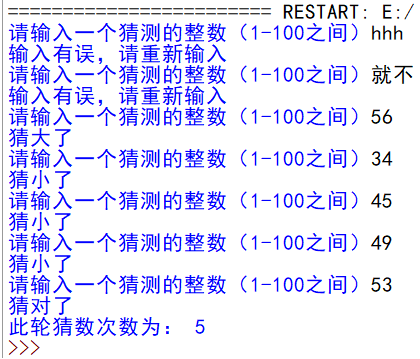