1, Adjacency table
1. Why do I need adjacency tables?
A: when you encounter a sparse graph, if you use the adjacency matrix to store it, the time complexity will be O(n^2) and the space complexity will be O(n^2). In fact, this is very uneconomical, because you have a lot of space unused, so you have the storage method of adjacency table
2. What is the adjacency table?
A: you can treat it as a linked list. It uses the form of pointing to find your next side.
3. Complexity of adjacency table
A: time complexity: O(n + e), space complexity: O(e), e depends on the number of edges
4. Function of adjacency table
A: you can quickly know the in degree and out degree of a vertex through linear traversal. Here I refer to a directed graph. Of course, an undirected graph can also calculate the degree
2, Construction of adjacency table
Given a set of data
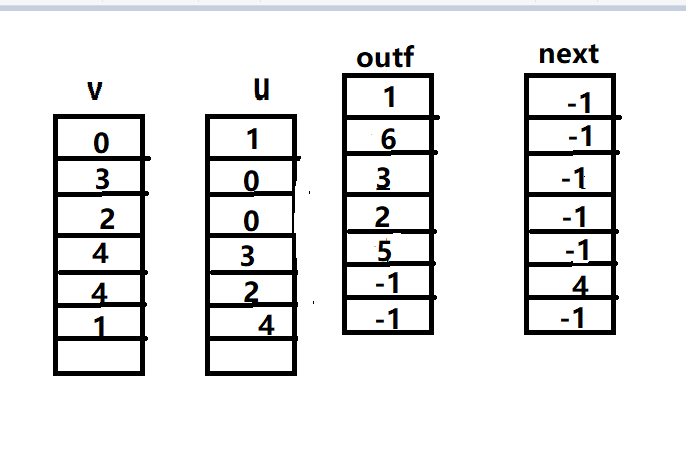
III. inverse adjacency table
The idea of inverse adjacency table is the same as that of adjacency table. Inverse adjacency table is used to calculate the in degree of a point, while adjacency table is used to calculate the out degree of a point.
4, Code implementation
1 #include "bits/stdc++.h" 2 using namespace std; 3 int inf[110],outf[110]; 4 int inext[110],outnext[110];//in Represents penetration, out Representative degree 5 int v[110],u[110]; 6 int main() 7 { 8 for(int i = 1;i <= 100;i++) 9 inf[i] = -1,outf[i] = -1; 10 int n,m; 11 cin >> n >> m;//n It's the top point, m Is the number of sides 12 int vi,ui; 13 for(int i = 1;i <= m;i++){ 14 cin >> v[i] >> u[i];//vi-->ui It's out, ui-->vi It's penetration 15 outnext[i] = outf[v[i]];//outf An adjacency table is created 16 outf[v[i]] = i; 17 inext[i] = inf[u[i]];//inf An inverse adjacency table is established 18 inf[u[i]] = i; 19 } 20 for(int i = 1;i <= n;i++){ 21 int ink,outk; 22 ink = inf[i]; 23 outk = outf[i]; 24 if(ink != -1) 25 cout << u[ink] << "\nin:" << endl; 26 //Yidi i The edge whose node No. is in degree is 27 while(ink != -1){ 28 cout << v[ink] << "-->" << u[ink] << endl; 29 ink = inext[ink]; 30 } 31 if(outk != -1) 32 cout << "out:" << endl; 33 //Yidi i The edge with node No. as out degree is 34 while(outk != -1){ 35 cout << v[outk] << "-->" << u[outk] << endl; 36 outk = outnext[outk]; 37 } 38 cout << "\n\n"; 39 } 40 return 0; 41 }