If you want to visit the website through HttpURLConnection, the website returns cookie information. The next time you visit the website through HttpURLConnection, you return cookie information to the website. You can use the following code.
CookieManager manager = new CookieManager(); CookieHandler.setDefault(manager);
Through these two lines of code, you can store the cookie information returned from the website. Next time you visit the website, you can automatically take the cookie information with you.
CookieManager can also set up CookiePolicy. The settings are as follows:
CookieManager manager = new CookieManager(); //Set cookie policy to accept only cookies from the server you are talking to, not cookies from other servers on the Internet manager.setCookiePolicy(CookiePolicy.ACCEPT_ORIGINAL_SERVER);
For more information on Cookie Policy: Analysis of CookiePolicy Principle
CookieHandler Source Code Analysis
public abstract class CookieHandler { private static CookieHandler cookieHandler; public synchronized static CookieHandler getDefault() { SecurityManager sm = System.getSecurityManager(); if (sm != null) { sm.checkPermission(SecurityConstants.GET_COOKIEHANDLER_PERMISSION); } return cookieHandler; } public synchronized static void setDefault(CookieHandler cHandler) { SecurityManager sm = System.getSecurityManager(); if (sm != null) { sm.checkPermission(SecurityConstants.SET_COOKIEHANDLER_PERMISSION); } cookieHandler = cHandler; } public abstract Map<String, List<String>> get(URI uri, Map<String, List<String>> requestHeaders) throws IOException; public abstract void put(URI uri, Map<String, List<String>> responseHeaders) throws IOException; }
CookieHandler is an abstract class that provides static setDefault methods internally.
And private static CookieHandler cookieHandler; static.
Subclasses need to implement get() and put() methods.
The get() method returns the uri-related cookie.
put() method is to store the uri-related cookie s.
The implementation class CookieManager of CookieHandler is provided in jdk1.6.
CookieManager Source Code Analysis
get() method
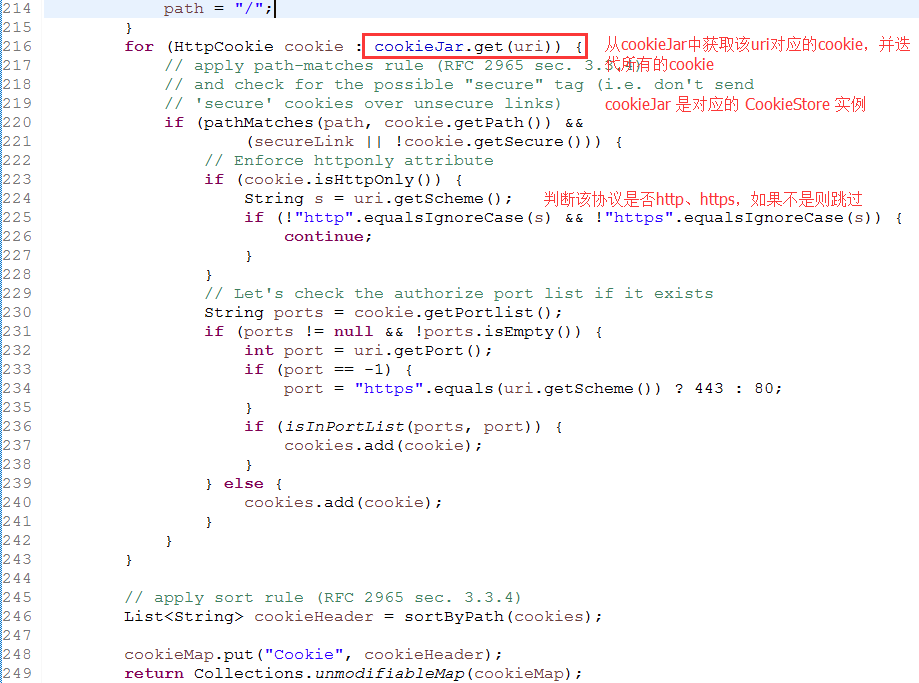
The CookieManager.get() method implements retrieving the cookie corresponding to the uri from the CookieStore.
put() method
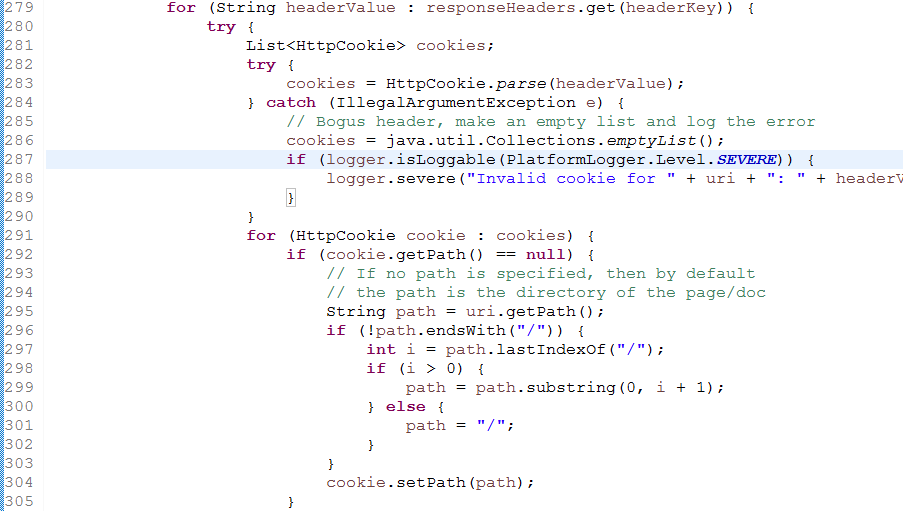
Firstly, the cookies in http header information are parsed and stored in List < HttpCookie > cookies.
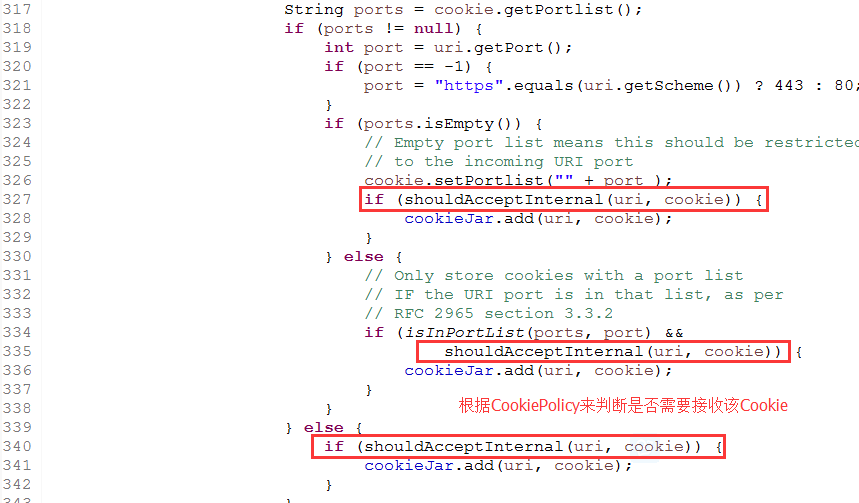
The cookie in the circular cookies is used to determine whether to receive the cookie information according to the set Cookie Policy.
If received, it is stored in CookieStore.
Cookie implementation mechanism
This way, every time HttpURLConnection is called to visit the website, the CookieHandler.getDefault() method is used to obtain the CookieManager instance (static method, global available).
Call the put method in CookieHandler from the cookie in the response header that parses http and store it in CookieStore.
Call the get method in CookieHandler to get the cookie of the uri response when visiting the website again and submit it to the site.
In this way, developers do not need to interfere with cookie information, and each visit to the site will automatically carry cookies.
Code examples
CookieHandler, CookieManager, CookieStore, HttpCookie are used in this example.
public class CookieManagerDemo { //Print cookie information public static void printCookie(CookieStore cookieStore){ List<HttpCookie> listCookie = cookieStore.getCookies(); listCookie.forEach(httpCookie -> { System.out.println("--------------------------------------"); System.out.println("class : "+httpCookie.getClass()); System.out.println("comment : "+httpCookie.getComment()); System.out.println("commentURL : "+httpCookie.getCommentURL()); System.out.println("discard : "+httpCookie.getDiscard()); System.out.println("domain : "+httpCookie.getDomain()); System.out.println("maxAge : "+httpCookie.getMaxAge()); System.out.println("name : "+httpCookie.getName()); System.out.println("path : "+httpCookie.getPath()); System.out.println("portlist : "+httpCookie.getPortlist()); System.out.println("secure : "+httpCookie.getSecure()); System.out.println("value : "+httpCookie.getValue()); System.out.println("version : "+httpCookie.getVersion()); System.out.println("httpCookie : "+httpCookie); }); } public static void requestURL() throws Exception{ URL url = new URL("http://192.168.3.249:9000/webDemo/index.jsp"); HttpURLConnection conn = (HttpURLConnection)url.openConnection(); String basic = Base64.getEncoder().encodeToString("infcn:123456".getBytes()); conn.setRequestProperty("Proxy-authorization", "Basic " + basic); BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream())); String line = null; while((line=br.readLine())!=null){ System.out.println(line); } br.close(); } public static void main(String[] args) throws Exception { CookieManager manager = new CookieManager(); //Set cookie policy to accept only cookies from the server you are talking to, not cookies from other servers on the Internet manager.setCookiePolicy(CookiePolicy.ACCEPT_ORIGINAL_SERVER); CookieHandler.setDefault(manager); printCookie(manager.getCookieStore()); //First request requestURL(); printCookie(manager.getCookieStore()); //Second request requestURL(); } }
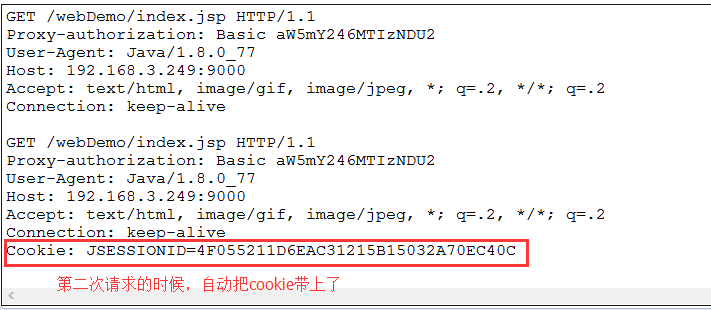
From the results of the package capture, it is found that Cookie information will be automatically carried when the site is visited the second time.