Effect
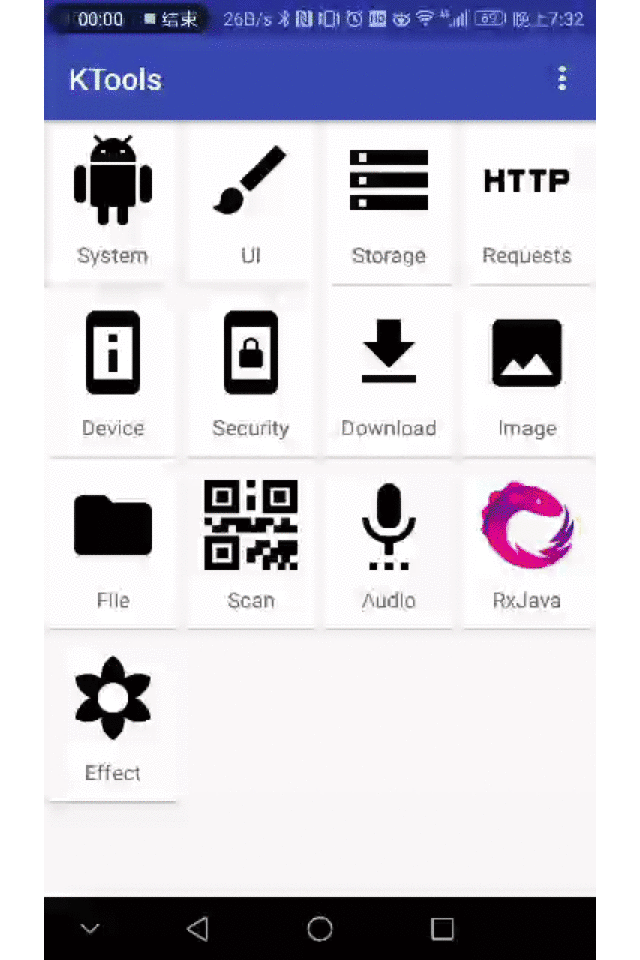
Taiji.gif
Step breakdown
Regular figures like Taiji are usually decomposed into basic figures by mathematical knowledge, such as line segment, circle, rectangle, sector, polygon, etc., and then drawn step by step.
- Decomposing Taiji diagram
@Override protected void onDraw(Canvas canvas) { int width = getWidth(); int height = getHeight(); //Pan the canvas to the middle of the View canvas.translate(width / 2, height / 2); //Background color canvas.drawColor(Color.GRAY); //Rotation, where mDegrees are associated with animation canvas.rotate(mDegrees,0,0); //Two semicircles int radius = Math.min(width, height) / 2 - 40; RectF rectF = new RectF(-radius, -radius, radius, radius); canvas.drawArc(rectF, 90, 180, true, mPaintBlack); canvas.drawArc(rectF, -90, 180, true, mPaintWhite); //Two small circles int smallRadius = radius / 2; canvas.drawCircle(0, -smallRadius, smallRadius, mPaintBlack); canvas.drawCircle(0, smallRadius, smallRadius, mPaintWhite); //Two little dots int dotRadius = smallRadius / 4; canvas.drawCircle(0, -smallRadius, dotRadius, mPaintWhite); canvas.drawCircle(0, smallRadius, dotRadius, mPaintBlack); }
- Use animation to constantly change one attribute of custom View
/* * ,Add animation to make Taiji move * */ public void startRotate() { ValueAnimator animator = ValueAnimator.ofInt(0,360); animator.setDuration(2000); animator.setInterpolator(new LinearInterpolator()); animator.setRepeatCount(ValueAnimator.INFINITE); animator.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() { @Override public void onAnimationUpdate(ValueAnimator animation) { mDegrees = (int) animation.getAnimatedValue(); invalidate(); } }); animator.start(); }
This effect is relatively simple, so we won't explain it more.
It is recommended not to use the method of sending Handler for the implementation effect of Animation, but to use Animation directly.