@TOC
Java data types
JAVA basic data type diagram
There are eight data types in java. The data type is java, and the data type is very important,
1. Java data types are divided into two categories: basic data types and reference types
2. The basic data types include 8 numerical types [byte, short, int, long, float, double] char and Boolean
3 reference type [class, interface, array]
2 integer variable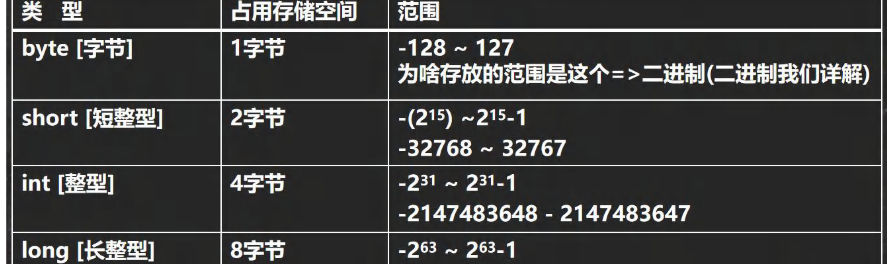
int variable name = initialized value
Usage details of int type
. int indicates that the type of the variable is an integer
2. The variable name is the identification of the variable. It is used later
3. In Java, = means assignment (different from Mathematics), which means setting an initial value for a variable
4. Initialization is optional, but it is recommended to explicitly initialize when creating variables
5. Finally, don't forget the semicolon, otherwise the compilation will fail
6. / / indicates comments. Comments are the explanatory part of the code and do not participate in compilation and operation
public class Wtd { public static void main(String[] args) { int b=30; int c=50; System.out.println(b); System.out.println(c); }
Use the following code to view the integer data range in Java:
public class Wtd { public static void main(String[] args) { int b=30; int c=50; System.out.println(Integer.MAX_VALUE); System.out.println(Integer.MIN_VALUE); } }
We can get the range
In java, integer variables are machine independent
Long integer variable
Long variable name = initial value
public class Wtd { public static void main(String[] args) { long b=30L; long c=50L; System.out.println(b); System.out.println(c); } }
Precautions for using long
- The basic syntax format is basically the same as that of creating int variables, except that the type is changed to long,
- The initialization setting value is 10l, which represents a long integer number. 10l is also OK,
- You can also initialize with 10. The type of 10 is int, and the type of 10L is long. It is better to use 10 L or 10 L
In Java, the long type takes up 8 bytes
Use the following code to view the scope
System.out.println(Long.MAX_VALUE); System.out.println(Long.MIN_VALUE);
4 double precision floating point variable
double variable name = initial value
public class Wtd { public static void main(String[] args) { double b=30; double c=50; System.out.println(b); System.out.println(c); } }
Single precision floating point variable
float variable name = initial value
public class Wtd { public static void main(String[] args) { float b=30; float c=50; System.out.println(b); System.out.println(c); } }
Character type variable
The character type can represent a single character. The character type is char. Char is two bytes (which can store Chinese characters). For multiple characters, we use string,
char c1 = 'a'; char c2 = '\t'; char c3 = 'Left'; char c4 = 97;
7-byte type variable
byte variable name = initial value
public class Wtd { public static void main(String[] args) { byte value = 0; System.out.println(value); } }
matters needing attention
- Byte type also represents an integer. It only occupies one byte and represents a small range (- 128 - > + 127)
- Byte types have nothing to do with character classes
Short integer variable
short variable name = initial value
public class Wtd { public static void main(String[] args) { short b=30; System.out.println(b); } }
matters needing attention:
- short takes up 2 bytes and represents a data range of - 32768 - > + 32767
- This indicates that the range is relatively small and is generally not recommended
Boolean type variable
Basic syntax format:
boolean variable name = initial value;
public class Wtd { public static void main(String[] args) { boolean value=true; System.out.println(value); } }
String type variable
Basic syntax format:
String variable name = "initial value";
matters needing attention:
- Java uses double quotation marks + several characters to represent string literals
- Different from the above types, String is not a basic type, but a reference type (explained later)
- Some specific characters in the string that are inconvenient to be directly represented need to be escaped
public class Wtd { public static void main(String[] args) { String name ="zxccbgsd"; System.out.println(name); } }
Scope of variable
That is, the range within which the variable can take effect. Generally, it is the code block (braces) where the variable definition is located
public class Wtd { public static void main(String[] args) { String name ="zxccbgsd"; System.out.println(name); } }
Naming rules for variables
Hard index:
- A variable name can only contain numbers, letters and underscores
- A number cannot begin
- Variable names are case sensitive. That is, Num and num are two different variables
Note: Although the Chinese dollar sign ($) is also allowed to name variables, it is strongly not recommended
Soft index: - Variable naming should be descriptive, see the meaning of name
- Variable names should not use pinyin (but not absolute)
- Nouns are recommended for the part of speech of variable names
- The small hump naming method is recommended for variable naming. When a variable name is composed of multiple words, the initials of other words are large except the first word
Write
Example of small hump naming:
int maxValue = 100;
String studentName = "Zhang San";
constant
Literal constant
Hex, octal, etc
Constant modified by final keyword
final int a = 10;
a = 20; // Compilation error. Hint: unable to assign value to final variable a
Constants cannot be modified while the program is running
Constant modified by final keyword
final int a = 10;
a = 20; // Compilation error. Hint: unable to assign value to final variable a
Constants cannot be modified while the program is running
Understand type conversion
The reverse process of automatic type conversion, which converts data types with large capacity into data types with small capacity. The cast character () should be added when using, but it may cause precision reduction or overflow. Pay special attention
Details needing attention
Understanding numerical improvement
Byte and byte are of the same type, but compilation errors occur. The reason is that although a and b are both bytes, calculating a + b will first both a and b
Promote to int, and then calculate, and the result is int, which is assigned to c, and the above error will occur
Because the CPU of the computer usually reads and writes data from the memory in units of 4 bytes, for the convenience of hardware implementation, such as byte and short
Summary of type promotion:
- For mixed operations of different types of data, those with a small range will be promoted to those with a large range
- For short and byte types smaller than 4 bytes, they will be promoted to 4 bytes of int before operation
public class Wtd { public static void main(String[] args) { byte a = 10; byte b = 20; byte c = (byte)(a + b); System.out.println(c); } }
Conversion between int and String
Convert int to String
public class Wtd { public static void main(String[] args) { int num = 10; // Method 1 String str1 = num + ""; // Method 2 String str2 = String.valueOf(num); } }
Convert String to int
public class Wtd { public static void main(String[] args) { String str = "100"; int num = Integer.parseInt(str); } }