Day08 static keyword and inheritance
1. Implementation of people class and test class
package com.lagou.Day08; /** * Programming the encapsulation of People class */ public class People { //1. Privatize the member variable and use the private keyword to modify it private String name; private int age; private String country; //3. in the construction method, we call the set method to judge the reasonable value. public People(){} public People(String name,int age,String country){ setName(name); setAge(age); setCountry(country); } //2. Provide public get and set methods, and judge the reasonable value in the method body public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age>0&&age<150){ this.age = age; }else { System.out.println("Age unreasonable!!!"); } } public String getCountry() { return country; } public void setCountry(String country) { this.country = country; } public void show(){ System.out.println("I am"+getName()+",this year"+getAge()+"Years old, from"+getCountry()); } }
package com.lagou.Day08; public class Test { public static void main(String[] args) { People p1 = new People("Fei Zhang",30,"China"); p1.show(); People p2 = new People("Guan Yu",35,"China"); p2.show(); } }
Print results I'm Zhang Fei,30 years old, from China I'm Guan Yu,35 years old, from China
2. Basic concept of static keyword
package com.lagou.Day08; /** * Programming the encapsulation of People class */ public class People { //1. Privatize the member variable and use the private keyword to modify it private String name; private int age; //private String country; public static String country; //3. in the construction method, we call the set method to judge the reasonable value. public People(){} public People(String name,int age,String country){ setName(name); setAge(age); setCountry(country); } //2. Provide public get and set methods, and judge the reasonable value in the method body public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age>0&&age<150){ this.age = age; }else { System.out.println("Age unreasonable!!!"); } } public String getCountry() { return country; } public void setCountry(String country) { this.country = country; } public void show(){ System.out.println("I am"+getName()+",this year"+getAge()+"Years old, from"+getCountry()); } }
package com.lagou.Day08; public class Test { public static void main(String[] args) { People p1 = new People("Fei Zhang",30,"China"); p1.show(); People p2 = new People("Guan Yu",35,"China"); p2.show(); p1.setCountry("Shu Kingdom"); p1.show(); p2.show(); } }
I'm Zhang Fei,30 years old, from China I'm Guan Yu,35 years old, from China I'm Zhang Fei,He is 30 years old and comes from Shu I'm Guan Yu,He is 35 years old and comes from Shu
- Use the static keyword to modify the member variable to indicate the meaning of static. At this time, the member variable is promoted from the object level to the class level, that is, there is only one copy of the whole class and shared by all objects. The member variable is ready with the loading of the class, regardless of whether to create an object.
- static keyword modified members can use references But the class name is recommended The way.
3. Usage of static keyword
4. Building blocks and static code blocks
- Building blocks: code blocks directly enclosed by {} in the class body.
- Building blocks are executed once without creating an object
- Static code block: a building block decorated with the static keyword
- Static code blocks are executed only once
package com.lagou.Day08; /** * Programming to achieve the use of building blocks and static code blocks */ public class Demo01 { //Function: unified initialization of member variables { System.out.println("Tectonic block!"); } //Function: load the driver package of the database, etc static { System.out.println("Static code block"); } //Custom construction method public Demo01(){ System.out.println("Construction method"); } public static void main(String[] args) { Demo01 d = new Demo01(); Demo01 d1 = new Demo01(); } }
Static code block Tectonic block! Construction method Tectonic block! Construction method
5. Detailed explanation of main method
- Syntax format: public static void main(String[] args) {}
- parameter
package com.lagou.Day08; /** * main Method testing */ public class Demo02 { public static void main(String[] args) { System.out.println("The number of elements in the parameter array is:" + args.length); System.out.println("Pass to main The actual parameters of the method are:"); for (int i=0;i<args.length;i++){ System.out.println("Subscript"+i+"The value of the formal parameter variable is:"+args[i]); } } }
main Method to pass parameters, java Demo02 Zhang Fei and Guan Yu
6. Framework implementation of singleton and SingletonTest classes
Title: 1.Programming implementation Singleton Class encapsulation 2.Programming implementation SingleTest Class pair Singleton Class for testing, requirements main Method can get and function to get an object of this class.
package com.lagou.Day08; /** * Write and implement the encapsulation of Singleton class */ public class Singleton { //1. Privatization construction method, using private private Singleton(){ } }
package com.lagou.Day08; /** * Test of programming Singleton class */ public class SingletonTest { public static void main(String[] args) { //1. Declare a Singleton class reference to the object of this type //Singleton s1 = new Singleton(); //Singleton s2 = new Singleton(); //System.out.println(s1 == s2);//false } }
When the construction method is privatized, main Method can no longer create objects.
7. Complete implementation of the framework of singleton and SingletonTest classes
package com.lagou.Day08; /** * Write and implement the encapsulation of Singleton class */ public class Singleton { //2. Declare that the reference of this type points to the object of this type private static Singleton sin = new Singleton(); public static Singleton getSin() { return sin; } public static void setSin(Singleton sin) { Singleton.sin = sin; } //1. Privatization construction method, using private private Singleton(){ } }
package com.lagou.Day08; /** * Test of programming Singleton class */ public class SingletonTest { public static void main(String[] args) { //Singleton refers to a class of this type //Singleton s1 = new Singleton(); //Singleton s2 = new Singleton(); //System.out.println(s1 == s2);//false Singleton s1 = Singleton.getSin(); Singleton s2 = Singleton.getSin(); System.out.println(s1 == s2);//true } }
8. Perform process and memory structure analysis
9. Single case design mode
-
In some special cases, when a class provides only one object, such a class is called a singleton class, and the process and idea of designing a singleton is called a singleton design pattern.
-
Implementation flow of singleton design pattern
- The privatization construction method is modified with the private keyword
- Declare that the reference of this class type points to the object of this class type, and use the private static keyword to modify it
- Provide a public get method, which is responsible for returning the object, and use the public static keyword to modify it.
-
Implementation of singleton design pattern
- There are two ways to implement the singleton design pattern: hungry and lazy. Hungry sweat is recommended in future development
// Come in and eat, hungry man style 1.private static Singleton sin = new Singleton(); // When calling the interface, lazy 2.private static Singleton sin = null; public static Singleton getInstance(){ if(null == sin){ sin = new Singleton(); } return sin; }
Origin and concept of inheritance
- When multiple classes have the same features and behaviors, the same content can be extracted to form a public class, so that multiple classes can attract the existing features and behaviors in the public class, while in multiple types, it is only necessary to write their own unique features and behaviors, which is called inheritance.
- In the Java language, the extends keyword is used to represent the inheritance relationship.
- The use of inheritance improves the reusability, maintainability and scalability of the code, which is the prerequisite of polypeptide.
11. Meaning of succession
package com.lagou.Day08; /** * Programming the encapsulation of Person class */ public class Person { //1. Privatize the member variable and use the private keyword to modify it private String name; private int age; //3. in the construction method, we call the set method to judge the reasonable value. public Person(){} public Person(String name,int age){ setName(name); setAge(age); } //2. Provide public get and set methods and judge the reasonable value in the method body public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age>0 && age<150){ this.age = age; }else { System.out.println("Age unreasonable!!!"); } } // Custom member method to print features public void show(){ System.out.println("I am"+getName()+",this year"+getAge()+"Years old!"); } //The custom member method describes the behavior of the user public void eat(String food){ System.out.println(food+"Really delicious!"); } //Custom member methods describe the behavior of entertainment public void play(String game){ System.out.println(game+"What fun!"); } }
package com.lagou.Day08; /** * Custom Worker inherits Person */ public class Worker extends Person{ }
package com.lagou.Day08; public class WorkerTest { public static void main(String[] args) { // 1. Use parameterless method to construct Worker type objects and print features Worker w1 = new Worker(); w1.show();//I'm null. I'm 0 years old this year! } }
12. Characteristics of succession 1
- Subclasses cannot inherit the constructor and private methods of the parent class, but private member variables can be inherited, but they cannot be accessed directly.
package com.lagou.Day08; /** * Custom Worker inherits Person */ public class Worker extends Person{ private int salary; public Worker(){} public Worker(String name,int age,int salary){ setName(name); setAge(age); setSalary(salary); } public int getSalary() { return salary; } public void setSalary(int salary) { if (salary >= 2200){ this.salary = salary; }else { System.out.println("The salary is unreasonable!!!"); } } // Custom member methods describe the behavior of the work public void work(){ System.out.println("Today's bricks are a little hot"); } }
package com.lagou.Day08; public class WorkerTest { public static void main(String[] args) { // 1. Use parameterless method to construct Worker type objects and print features Worker w1 = new Worker(); w1.show();//I'm null. I'm 0 years old this year! System.out.println("------------------"); // 2. Use parametric method to construct Worker type objects and print features Worker w2 = new Worker("Fei Zhang",30,3000); w2.show();//I'm Zhang Fei. I'm 30 years old! w2.eat("Bean sprouts");//Bean sprouts are delicious! w2.play("Glory of Kings");//King Rong Yao is so fun! w2.work();//Today's bricks are a little hot } }
13. Characteristics of succession 2
- No matter how the subclass object is constructed, the parameterless construction method of the parent class will be automatically called to initialize the member variables inherited from the parent class, which is equivalent to adding the effect of code super() in the first line of the construction method.
package com.lagou.Day08; /** * Programming the encapsulation of Person class */ public class Person { //1. Privatize the member variable and use the private keyword to modify it private String name; private int age; //3. in the construction method, we call the set method to judge the reasonable value. public Person(){ System.out.println("Person()"); } public Person(String name,int age){ System.out.println("Person(String,int)"); setName(name); setAge(age); } //2. Provide public get and set methods and judge the reasonable value in the method body public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age>0 && age<150){ this.age = age; }else { System.out.println("Age unreasonable!!!"); } } // Custom member method to print features public void show(){ System.out.println("I am"+getName()+",this year"+getAge()+"Years old!"); } //The custom member method describes the behavior of the user public void eat(String food){ System.out.println(food+"Really delicious!"); } //Custom member methods describe the behavior of entertainment public void play(String game){ System.out.println(game+"What fun!"); } }
package com.lagou.Day08; /** * Custom Worker inherits Person */ public class Worker extends Person{ private int salary; public Worker(){ super();//It refers to calling the parameterless construction method of the parent class. If it is not added, the compiler will add it automatically System.out.println("Worker()"); } public Worker(String name,int age,int salary){ super(name,age);//Represents a parameterized constructor that calls the parent class System.out.println("Worker(String,int,int)"); //setName(name); //setAge(age); setSalary(salary); } public int getSalary() { return salary; } public void setSalary(int salary) { if (salary >= 2200){ this.salary = salary; }else { System.out.println("The salary is unreasonable!!!"); } } // Custom member methods describe the behavior of the work public void work(){ System.out.println("Today's bricks are a little hot"); } }
package com.lagou.Day08; public class WorkerTest { public static void main(String[] args) { // 1. Use parameterless method to construct Worker type objects and print features Worker w1 = new Worker(); w1.show();//I'm null. I'm 0 years old this year! System.out.println("------------------"); // 2. Use parametric method to construct Worker type objects and print features Worker w2 = new Worker("Fei Zhang",30,3000); w2.show();//I'm Zhang Fei. I'm 30 years old! w2.eat("Bean sprouts");//Bean sprouts are delicious! w2.play("Glory of Kings");//King Rong Yao is so fun! w2.work();//Today's bricks are a little hot } }
Person() Worker() I am null,I'm 0 years old! ------------------ Person(String,int) Worker(String,int,int) I'm Zhang Fei. I'm 30 years old! Bean sprouts are delicious! King Rong Yao is so fun! Today's bricks are a little hot
14. Characteristics of succession 3 and 4
- The use of inheritance must meet the logical relationship: the subclass is a parent class, that is, inheritance cannot be abused.
- The Java language only supports single inheritance, not multiple inheritance, that is, a subclass can only have one parent class, but a parent class can have multiple subclasses.
15. Concept and use of method rewriting
- When the method inherited from the parent class does not meet the needs of the child class, it is necessary to override a method in the child class like the parent class to override the version inherited from the parent class. This method is called method override
package com.lagou.Day08; /** * Programming the encapsulation of Person class */ public class Person { //1. Privatize the member variable and use the private keyword to modify it private String name; private int age; //3. in the construction method, we call the set method to judge the reasonable value. public Person(){ System.out.println("Person()"); } public Person(String name,int age){ System.out.println("Person(String,int)"); setName(name); setAge(age); } //2. Provide public get and set methods and judge the reasonable value in the method body public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { if (age>0 && age<150){ this.age = age; }else { System.out.println("Age unreasonable!!!"); } } // Custom member method to print features public void show(){ System.out.println("I am"+getName()+",this year"+getAge()+"Years old!"); } //The custom member method describes the behavior of the user public void eat(String food){ System.out.println(food+"Really delicious!"); } //Custom member methods describe the behavior of entertainment public void play(String game){ System.out.println(game+"What fun!"); } }
package com.lagou.Day08; /** * Custom Worker inherits Person */ public class Worker extends Person{ private int salary; public Worker(){ super();//It refers to calling the parameterless construction method of the parent class. If it is not added, the compiler will add it automatically System.out.println("Worker()"); } public Worker(String name,int age,int salary){ super(name,age);//Represents a parameterized constructor that calls the parent class System.out.println("Worker(String,int,int)"); //setName(name); //setAge(age); setSalary(salary); } public int getSalary() { return salary; } public void setSalary(int salary) { if (salary >= 2200){ this.salary = salary; }else { System.out.println("The salary is unreasonable!!!"); } } // Custom member methods describe the behavior of the work public void work(){ System.out.println("Today's bricks are a little hot"); } // The custom show method overrides the version inherited from the parent class @Override public void show(){ super.show();// It means calling the show method of the parent class to print the name and age of the parent class System.out.println("My salary is:"+getSalary()); } }
package com.lagou.Day08; public class WorkerTest { public static void main(String[] args) { // 1. Use parameterless method to construct Worker type objects and print features Worker w1 = new Worker(); w1.show(); System.out.println("------------------"); // 2. Use parametric method to construct Worker type objects and print features Worker w2 = new Worker("Fei Zhang",30,3000); w2.show(); w2.eat("Bean sprouts"); w2.play("Glory of Kings"); w2.work(); } }
16. Principle of method rewriting
- The method name, parameter list and return value type are required to be the same. Subclass types are allowed to be returned from Java 5.
- It is required that the access rights of methods cannot be reduced, but can be the same or larger.
- Requires that the method cannot throw a larger exception (exception mechanism).
17. Implementation of animal class
package com.lagou.Day08; /** * 1.Program to realize the encapsulation of Animal class. The features include: name and coat color. It is required to provide a method to print all features. * 2.Program to realize the encapsulation of Dog class and inherit Animal class. The characteristics of this class include: the number of teeth. It is required to provide a method to print all characteristics. * 3.The DogTest class is implemented by programming. In the main method, the non parametric and parametric methods are used to construct Dog type objects and print features. */ public class Animal { private String name; private String color; public Animal() { } public Animal(String name, String color) { setName(name); setColor(color); } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getColor() { return color; } public void setColor(String color) { this.color = color; } public void show(){ System.out.println("name:"+getName()+",Color:"+getColor()); } }
18. Implementation of DAG class and DogTest class
package com.lagou.Day08; public class Dog extends Animal{ private int tooth; public Dog(){} public Dog(String name,String color,int tooth){ super(name,color);//Represents a parameterized constructor that calls the parent class setTooth(tooth); } public int getTooth() { return tooth; } public void setTooth(int tooth) { if (tooth>0){ this.tooth = tooth; }else { System.out.println("Unreasonable number of teeth"); } } @Override public void show() { super.show(); System.out.println("The number of teeth is:"+getTooth()); } }
package com.lagou.Day08; public class DogTest { public static void main(String[] args) { Dog d1 = new Dog(); d1.show();//Name: null, color: null Dog d2 = new Dog("Wangcai","white",10); d2.show();//Name: Wangcai, color: white } }
name: null,Color: null Number of teeth: 0 Name: Wangcai,Color: white Number of teeth: 10
19. Test points for building blocks and static code blocks
package com.lagou.Day08; /** *The implementation sequence of the previous explanation is as follows */ public class SuperTest { { System.out.println("SuperTest Class!"); //Second execution } static { System.out.println("SuperTest Static code block in class!"); //First execution } public SuperTest(){ System.out.println("SuperTest Constructor in class!"); //Last execution } public static void main(String[] args) { //Construct objects with no parameters SuperTest st = new SuperTest(); } }
package com.lagou.Day08; public class SubSuperTest extends SuperTest{ { System.out.println("=====SubSuperTest Class!"); //Second execution } static { System.out.println("=====SubSuperTest Static code block in class!"); //First execution } public SubSuperTest(){ System.out.println("=====SubSuperTest Constructor in class!"); //Last execution } public static void main(String[] args) { //Construct objects with no parameters SubSuperTest sst = new SubSuperTest(); } }
- First execute the static code block of the parent class, and then execute the static code block of the child class
- Execute the construction block of the parent class and the constructor body of the parent class
- Execute the construction block of the subclass and the constructor body of the subclass
20. Definition of permission modifier and package
Modifier | This category | Classes in the same package | Subclass | Other classes |
---|---|---|---|---|
public | Can access | Can access | Can access | Can access |
protected | Can access | Can access | Can access | Cannot access |
default | Can access | Can access | Cannot access | Cannot access |
private | Can access | Cannot access | Cannot access | Cannot access |
- Members decorated with public can be used anywhere
- Members decorated with private can only be used inside this class
- Usually, member methods are decorated with public keywords, and member variables are decorated with private keywords.
21. Function of final modifier classes and methods
- Final means "final and unchangeable", which can modify classes, member methods and member variables
package com.lagou.Day08; /** * 1.final Keyword modification class is reflected in that this class cannot be inherited * - It is mainly used to prevent abuse of inheritance, such as Java Lang. string class, etc */ public final class FinalClass { }
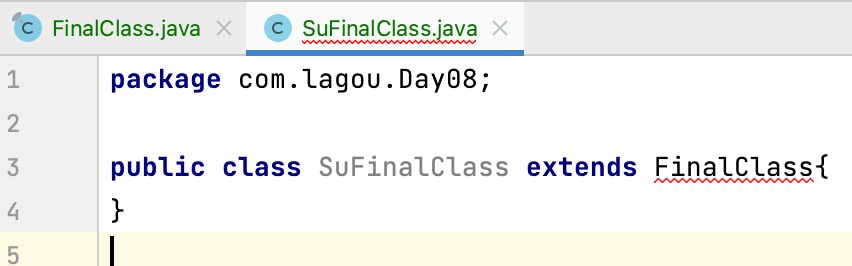
package com.lagou.Day08; /** * 1.final Keyword modification class is reflected in that this class cannot be inherited * - It is mainly used to prevent abuse of inheritance, such as Java Lang. string class, etc * 2.final Keyword modified member method is reflected in that the method cannot be overridden but can be inherited * - It is mainly used to prevent inadvertent rewriting, such as Java text. format method in dateformat class, etc */ public /*final*/ class FinalClass { public final void show(){ System.out.println("FinalClass Class show method!"); } }

22. Function of final modifier member variable
- The member variable of final modification must be initialized first
package com.lagou.Day08; /** * final Keyword modifies a member variable, which must be initialized and cannot be changed. * -It is mainly used to prevent inadvertent changes, such as Java Max in lang.Thread class_ Priority et al */ public class FinalMemberTest { private final int cnt = 1;//Display initialization public static void main(String[] args) { //Declare the FinalMemberTest type but refer to the object pointing to this class FinalMemberTest fmt = new FinalMemberTest(); //Print the value of the member variable System.out.println("fmt.cnt="+fmt.cnt);//1 } }
- Initialization in building block
package com.lagou.Day08; /** * final Keyword modifies a member variable, which must be initialized and cannot be changed. * -It is mainly used to prevent inadvertent changes, such as Java Max in lang.Thread class_ Priority et al */ public class FinalMemberTest { private final int cnt;//Display initialization { cnt = 2;//Building block initialization } public static void main(String[] args) { //Declare the FinalMemberTest type but refer to the object pointing to this class FinalMemberTest fmt = new FinalMemberTest(); //Print the value of the member variable System.out.println("fmt.cnt="+fmt.cnt);//2 } }
- Initialization construction method
package com.lagou.Day08; /** * final Keyword modifies a member variable, which must be initialized and cannot be changed. * -It is mainly used to prevent inadvertent changes, such as Java Max in lang.Thread class_ Priority et al */ public class FinalMemberTest { private final int cnt; public FinalMemberTest(){ cnt = 3;//Constructor initialization } public static void main(String[] args) { //Declare the FinalMemberTest type but refer to the object pointing to this class FinalMemberTest fmt = new FinalMemberTest(); //Print the value of the member variable System.out.println("fmt.cnt="+fmt.cnt);//3 } }