What is the bridging mode?
Bridge pattern is to decouple abstraction and realization so that they can be independent. This design pattern belongs to structural design pattern. What is meant by decoupling abstraction and realization can be understood as abstracting function points. How to realize functions depends on different requirements, but the abstract function points (interfaces) have been bridged to the original types and only focus on and implementation. The original types and abstract function points can be changed freely, and the bridge in the middle has been built.
In fact, the bridging mode is not only using class inheritance, but also focusing on using class aggregation to bridge and aggregate (inject) the abstract function points into the base class.
Benefits of bridging mode
What problems are generally used to solve?
The main reason is that there are many types of function nodes. Function nodes with multiple dimensions change independently and have no correlation. They can be managed according to dimensions. For example, there are two dimensions, and each dimension has three implementations, but there is no association between different dimensions. If we do it according to the pairwise association between dimensions, the number of implementation classes alone has 2 * 3 = 6 classes, which is inappropriate and coupled together.
Taking computers as an example, they will be divided into different brands, such as Dell and Lenovo, as well as desktops and notebooks. Then there will be many different categories, and the functions may be repeated. In view of this, we have to peel off the repeated functions and maintain the abstracted common function points by bridging.
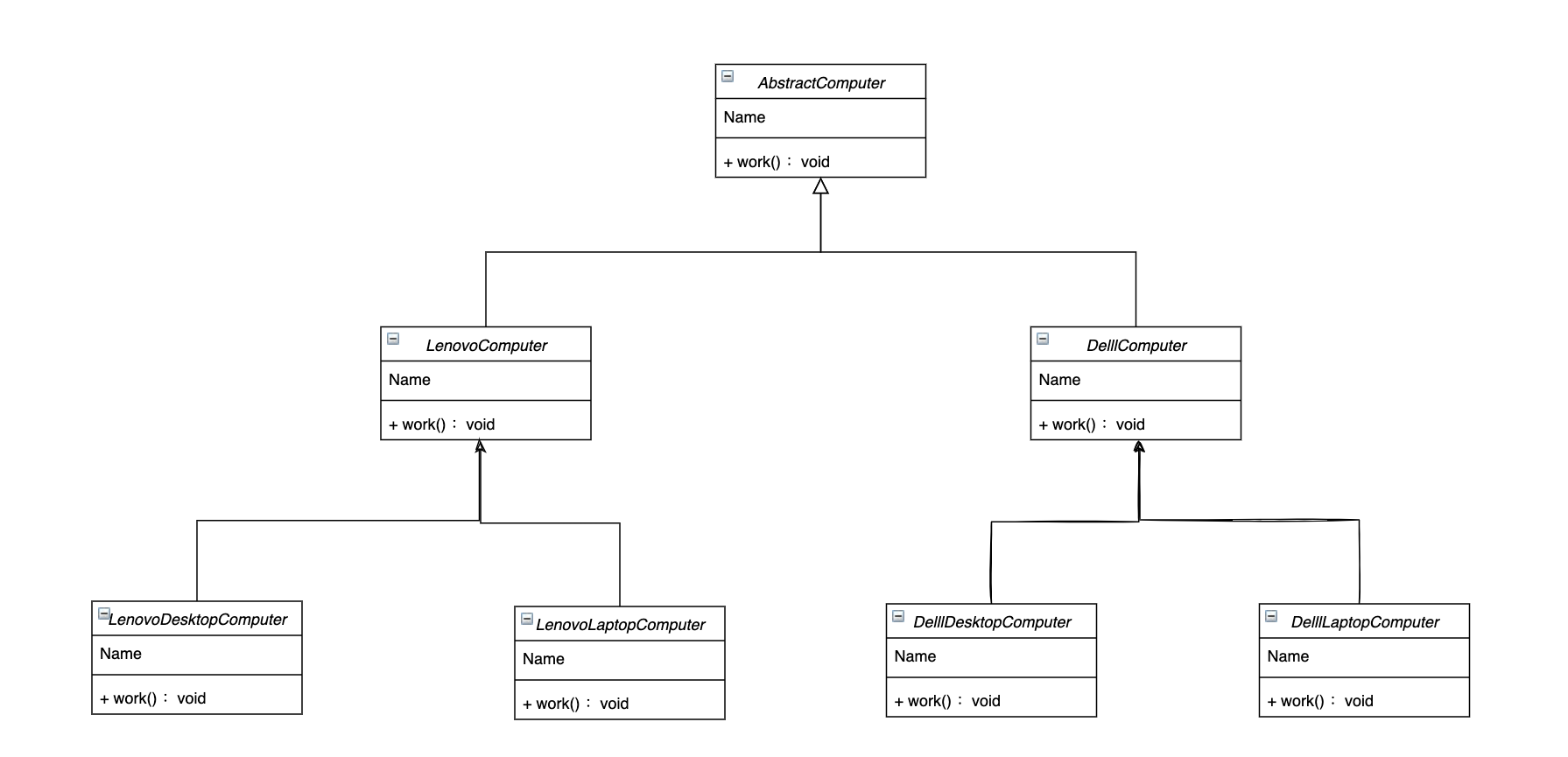
If you add another brand, such as ASUS, you have to add two classes, which is obviously inappropriate. Many functions of different classes may be repeated.
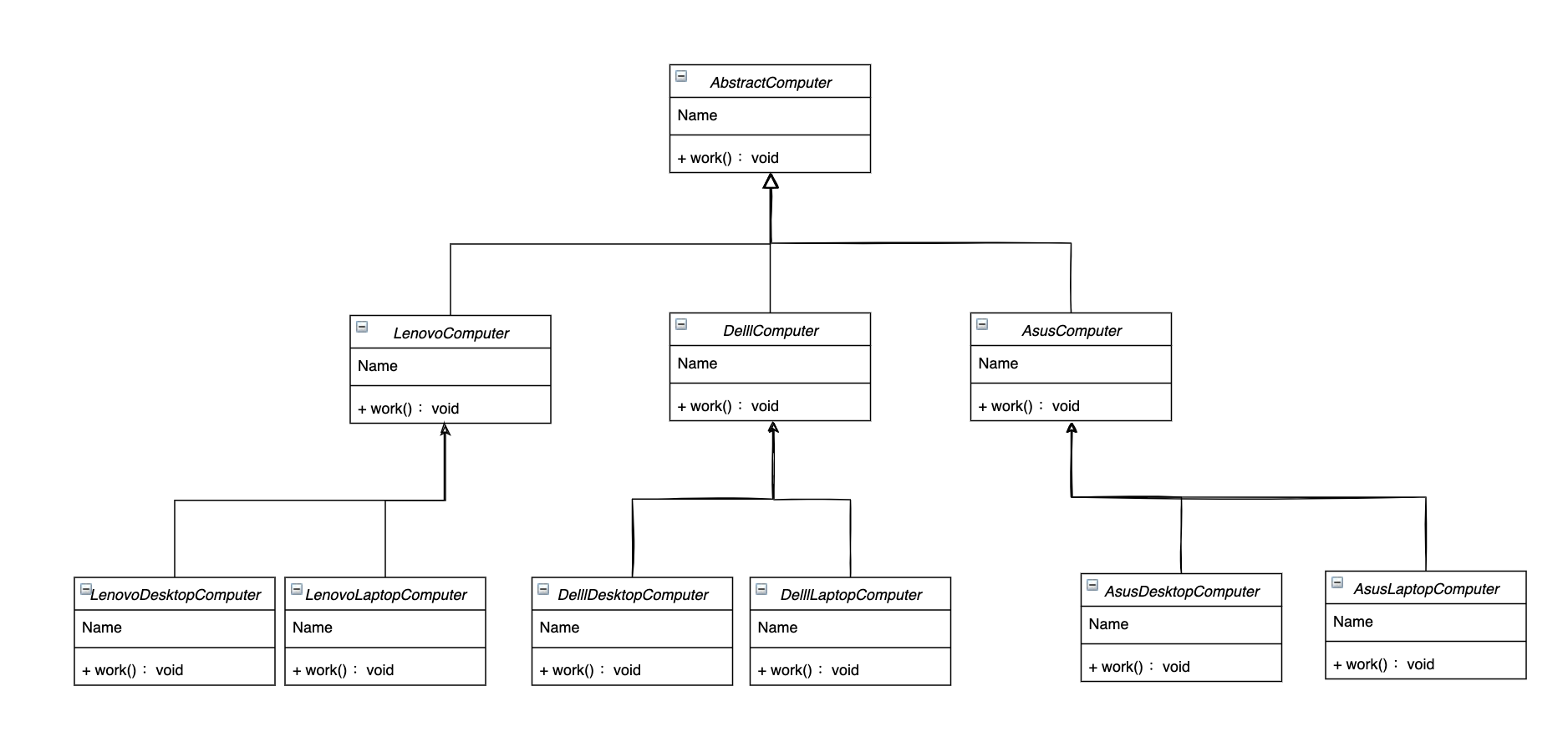
So what about the bridge mode? The bridging mode extracts two different dimensions of desktop and notebook, which is equivalent to being maintained as a general attribute.
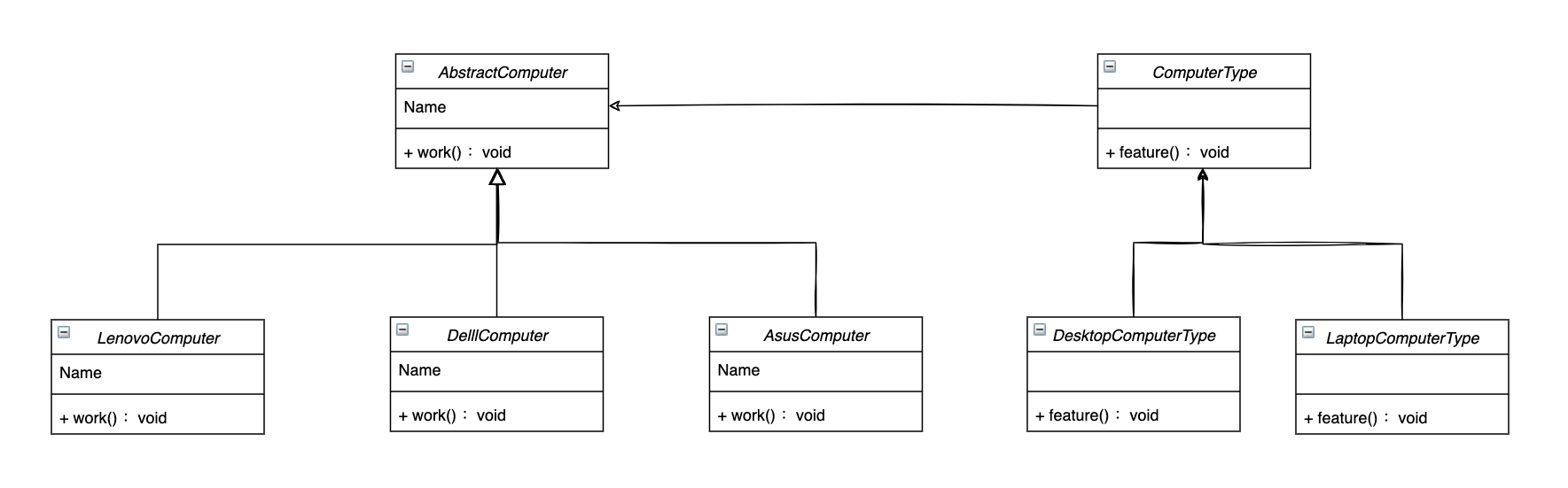
Code Demo demo
First, define an abstract computer class AbstractComputer, in which one attribute is ComputerType, indicating the type of computer:
public abstract class AbstractComputer { protected ComputerType type; public void setType(ComputerType type) { this.type = type; } public abstract void work(); }
Define three types of computers: Lenovo computer, ASUS computer, and DellComputer:
public class LenovoComputer extends AbstractComputer{ @Override public void work() { System.out.print("association"); type.feature(); } }
public class AsusComputer extends AbstractComputer{ @Override public void work() { System.out.print("ASUS"); type.feature(); } }
public class DellComputer extends AbstractComputer{ @Override public void work() { System.out.print("Dale"); type.feature(); } }
The dimension of computer type also needs an abstract class ComputerType, which has a function description method feature():
public abstract class ComputerType { // Functional characteristics public abstract void feature(); }
In the dimension of computer type, we define desktop and notebook computers:
public class DesktopComputerType extends ComputerType{ @Override public void feature() { System.out.println(" Desktop: strong performance and expansibility"); } }
public class LaptopComputerType extends ComputerType{ @Override public void feature() { System.out.println(" Laptop: small and portable, office is no problem"); } }
Test it. When we need different collocations, we only need to set one dimension into the object to aggregate different brands and types of computers:
public class BridgeTest { public static void main(String[] args) { ComputerType desktop = new DesktopComputerType(); LenovoComputer lenovoComputer = new LenovoComputer(); lenovoComputer.setType(desktop); lenovoComputer.work(); ComputerType laptop = new LaptopComputerType(); DellComputer dellComputer = new DellComputer(); dellComputer.setType(laptop); dellComputer.work(); } }
Test results:
Lenovo desktop: powerful performance and strong expansibility Dell laptop: small and portable, office is no problem
To sum up
In essence, bridging mode is to abstract different dimensions or functions and aggregate them into objects as attributes, rather than through inheritance. This reduces the number of classes to a certain extent, but if the changes between different dimensions are related, you need to make various special judgments when using them. It is easy to cause confusion and should not be used. (emphasis: use combination / aggregation relationship to replace inheritance relationship)
JDBC, students who have been involved in Java should know that this is an API for Java unified access to databases, which can operate Mysql,Oracle, etc. the main design mode used is also the bridging mode. If you are interested, you can learn about the source code of Driver driver class management.