1, Method reference
(1) Experience method reference
- When using Lambda expressions, the code we actually pass in is a solution: operate with parameters
- Then consider a case: if the same scheme already exists in the operation scheme specified in Lambda, is it necessary to write duplicate logic?
- The answer must be no need
- How do we use the existing scheme? Method reference, we use the existing scheme through method reference
/*
Requirements:
1.Define an interface (Printable): define an abstract method inside: void printString(String s);
2.Define a test class (PrintableDemo) and provide two methods in the test class
One method is: userPrintable(Printable p)
One method is the main method, and the usePrintable method is called in the main method.
*/
interface Printable{
void printString(String s);
}
public class PrintableDemo{
public static void main(String[] args) {
//Calling the usePrintable method in the main method
//Lambda
userPrintable(s -> System.out.println(s));
//Method reference:
userPrintable(System.out::println);
//What can be deduced is what can be omitted
}
private static void userPrintable(Printable p){
p.printString("Love life,love Java");
}
}
(2) Method reference
1. Method reference
- : this symbol is a reference operator, and the expression in which it is located is called a method reference.
2. Review our code in the experience method reference
- Lambda expression: useprintable (s - > system. Out. Println (s));
Analysis: after getting the parameter s, it is passed to system. Com through Lambda expression out. Println method to handle - Method reference: usePrintable(System.out::println);
Analysis: directly use system The println method in out replaces Lambda, and the code is more concise
3. Derivation and omission
- If Lambda is used, according to the principle of "derivable is omitted", there is no need to specify the parameter type or overload form, and they will be deduced automatically
- If method reference is used, it can also be deduced according to the context
- Method references are Lambda's twin brothers
(3) Method references supported by Lambda expressions
1. Reference class method
(1) A reference class method is actually a static method of a reference class
- Format: Class Name:: static method
- Example: Integer::parseInt
- Convert the data type of this class: String int (string int) to public int
(2) Exercise:
- Define an interface (Converter), which defines an abstract method
int convert(String s); - Define a test class (ConverterDemo) and provide two methods in the test class
① One method is: useConverter(Converter c)
Second, one method is the main method, and the useConverter method is called in the main method.
interface Converter{
int convert(String s);
}
public class ConverterDemo{
public static void main(String[] args) {
//Lambda
useConverter(s -> Integer.parseInt(s));
//Reference class method
useConverter(Integer::parseInt);
//When a Lambda expression is replaced by a class method, all its formal parameters are passed to the static method as parameters
//Pass the s parameter in Lambda to the static method parseInt, that is, if there are multiple parameters in Lambda, there are multiple corresponding static methods
}
private static void useConverter(Converter c){
int number = c.convert("666");
System.out.println(number);
}
}
2. Instance method of reference object
(1) The instance method of a reference object actually refers to the member method in the class
- Format: object:: member method
- Example: "HelloWorld"::toUpperCase
- The method in the String class: public String toUpperCase() converts all characters of this String to uppercase
(2) Practice
- Define a class (PrintString), which defines a method
public void printUpper(String s): change the string parameter into uppercase data, and then output it on the console - Define an interface (Printer), which defines an abstract method
void printUpperCase(String s) - Define a test class (PrinterDemo) and provide two methods in the test class
① One method is: usePrinter(Printer p)
Second, one method is the main method, and the usePrinter method is called in the main method.
class PrintString{
//Change the string parameter to uppercase data, and then output it to the console
public void printUpper(String s){
String result = s.toUpperCase();
System.out.println(result);
}
}
interface Printer{
void printUpperCase(String s);
}
public class PrinterDemo{
public static void main(String[] args) {
//Calling the usePrinter method in the main method
// usePrinter((String s) ->{
// //String result = s.toUpperCase();
// //System.out.println(result);
//
// //System.out.println(s.toUpperCase());
// });
//Lambda
usePrinter(s -> System.out.println(s.toUpperCase()));
//Instance method of reference object
PrintString ps = new PrintString();
usePrinter(ps::printUpper);
//When a Lambda expression is replaced by an instance method of an object, all its formal parameters are passed to the method as parameters
//The formal parameters s in the Lambda expression need to be passed to the printUpper method as parameters
}
private static void usePrinter(Printer p){
p.printUpperCase("HelloWorld");
}
}
3. Instance method of reference class
(1) An instance method of a reference class is actually a member method of a reference class
- Format: Class Name:: member method
- Example: String::substring
① Methods in String class: public String substring (int beginIndex,int endIndex)
② . intercept the string from beginIndex to endIndex. Returns a substring whose length is endIndex beginIndex
(2) Practice
- Mystring defines an abstract method:
String mySubString(String s,int x,int y); - Define a test class (MyStringDemo) and provide two methods in the test class
① One method is: use mystring (mystring my)
Second, one method is the main method, and the useMyString method is called in the main method.
interface MyString{
String mySubString(String s, int x, int y);
}
public class MyStringDemo {
public static void main(String[] args) {
//Calling the useMyString method in the main method
//Lambda
useMyString((String s,int x, int y) ->{
return s.substring(x,y);
});
useMyString((s,x,y) -> s.substring(x,y));
//Instance method of reference class
useMyString(String::substring);
//When a Lambda expression is replaced by an instance method of a class
//The first parameter is the caller
//All the following parameters are passed to the method as parameters
}
private static void useMyString(MyString my){
String s = my.mySubString("HelloWorld", 2, 5);
System.out.println(s);
}
}
4. Reference constructor
(1) A reference constructor is actually a reference constructor
- Format: Class Name:: new
- Example: Student::new
(2) Practice
- Define a class (Student) with two member variables (name,age)
It also provides parameterless construction methods and parameter construction methods, as well as get and set methods corresponding to member variables - Define an interface (StudentBuilder), which defines an abstract method
Student build(String name,int age); - Define a test class (StudentDemo) and provide two methods in the test class
① One method is: use studentbuilder (studentbuilder s)
Second, one method is the main method, and the useStudentBuilder method is called in the main method.
class Student{
private String name;
private int age;
public Student() {
}
public Student(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
interface StudentBuilder{
Student build(String name,int age);
}
public class StudentDemo {
public static void main(String[] args) {
//Calling the useStudentBuilder method in the main method
useStudentBuilder((String name, int age)->{
return new Student(name,age);
});
//Lambda
useStudentBuilder(((name, age) -> new Student(name,age)));
//Reference constructor
useStudentBuilder(Student::new);
//When a Lambda expression is replaced by a constructor, all its formal parameters are passed to the constructor as parameters
}
private static void useStudentBuilder(StudentBuilder sb){
Student s = sb.build("Lin Qingxia", 30);
System.out.println(s.getName()+","+s.getAge());
}
}
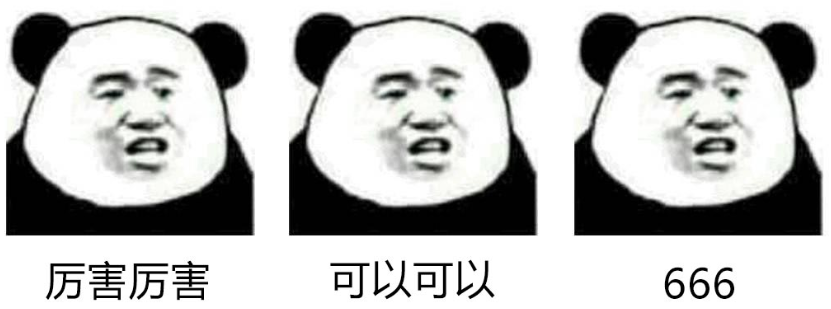