Demand problem
In daily work, most of the back-end django requests sent from the front end are returned in json format, and some are returned in view by template.
It's really convenient to return the view in the template, but django can only return data in json format if it involves dynamic and static separation and ajax requests.
So here comes a problem: how to put the data queried by django from the database model class back to the front end in json format.
Then what if the front end gets and reads the returned data?
Environmental description
- The front end uses jquery to send ajax requests
- python 3.7.2
- django 2.1.7
Example description
This example first writes a simple page to send an ajax request, and then the back-end divides how to return multiple rows of data. If you return a query object, give an example.
Front end code
First, write a simple front-end page test_ ajax. The html is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <script src="/static/js/jquery-3.0.0.min.js"></script> <script> $(function () { $('#search_server').click(function () { // Get server name var server_name = $('#server_name').val(); console.log('server_name = ' + server_name); // Send ajax post request $.ajax({ url: "/assetinfo/test_ajax", type: 'POST', data: { "tag": "search_project", "server_name": server_name, }, dataType: "json", async: false, // The function successfully called by the request success: function (data) { console.log(data); }, // The function called when the request is in error error: function () { alert("Failed to send query item data"); } }) }) }) </script> </head> <body> <input type="text" id="server_name"> <button id="search_server">Submit</button> </body> </html>
The function is very simple, just get the content of the input box, and then click the submit button to send an ajax post request.
The background directly queries the server information, and then returns multiple json data
The implementation class view code is as follows:
from django.core import serializers from django.http import HttpResponse from assetinfo.models import ServerInfo # ex: /assetinfo/test_ajax class TestAjax(View): def get(self,request): """display html page""" return render(request,'assetinfo/test_ajax.html') def post(self,request): """Receive processing ajax of post request""" servers = ServerInfo.objects.all() # Query server information json_data = serializers.serialize('json', servers) # json serialize query results return HttpResponse(json_data, content_type="application/json") # Return json data
In the background code, I did not get the parameters of the post request and then query the parameters. This only demonstrates how to return json format data.
The way to get the parameters of the post request is very simple, which is still request POST. Just get ('parameter name ').
The browser test functions are as follows: "
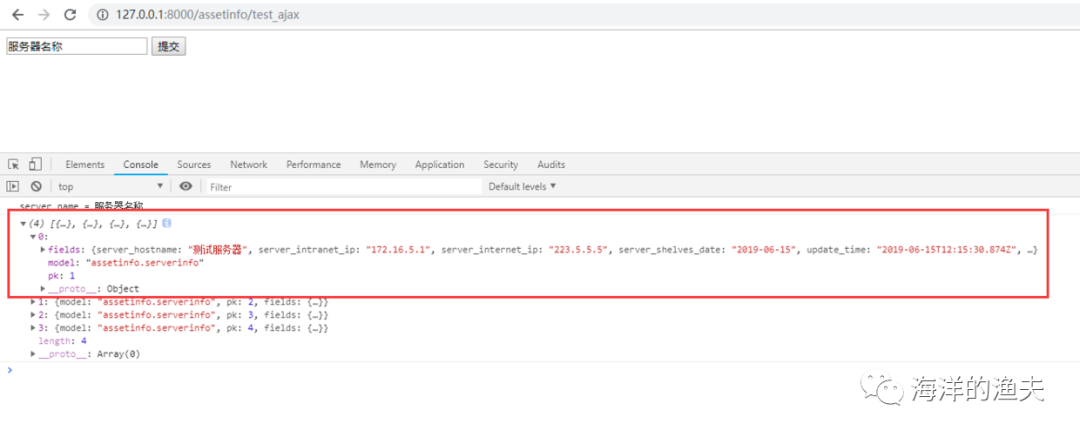
You can see the result data returned from the back end from the browser console.
However, it is not good to directly return without task constraints with the front end. Let's add Format Constraints for interaction with the front end.
Data format returned by front and rear constraints
{"resCode": '0', "message": 'success',"data": []}
According to this constraint format, the query results should be placed in the data array. Let's change the back-end view code.
The backend returns json data in constraint format
from django.core import serializers from django.http.response import JsonResponse from assetinfo.models import ServerInfo # ex: /assetinfo/test_ajax class TestAjax(View): def get(self,request): """display html page""" return render(request,'assetinfo/test_ajax.html') def post(self,request): """Receive processing ajax of post request""" # Return format agreed with the front end result = {"resCode": '0', "message": 'success',"data": []} # Query server information servers = ServerInfo.objects.all() # Serialize to Python object result["data"] = serializers.serialize('python', servers) return JsonResponse(result)
The browser test is as follows: "
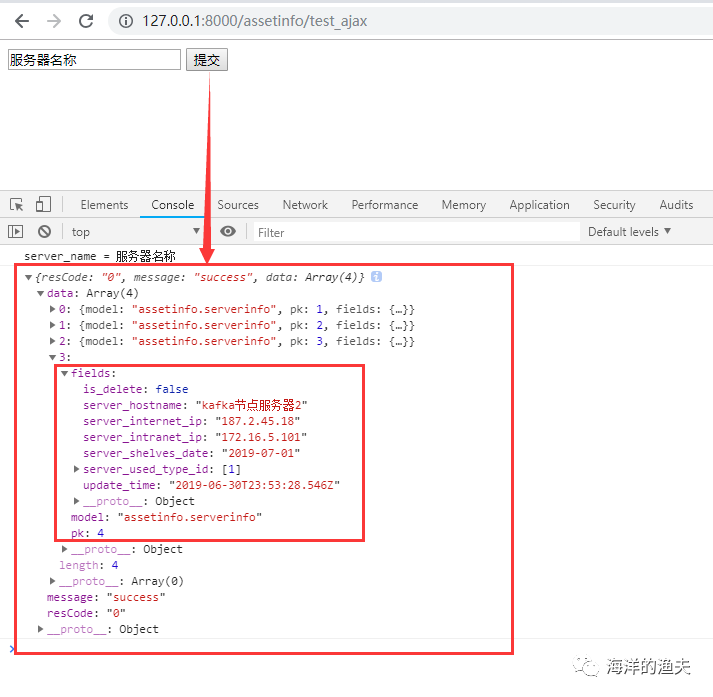
If the child returns to the front end in this way, each data object contains three objects: fields, model and pk, representing the field, model and primary key respectively. I prefer a dictionary object that only contains all fields.
The backend modifies each model object and converts it into a dict dictionary object
from django.core import serializers from django.http.response import JsonResponse from django.forms.models import model_to_dict # ex: /assetinfo/test_ajax class TestAjax(View): def get(self,request): """display html page""" return render(request,'assetinfo/test_ajax.html') def post(self,request): """Receive processing ajax of post request""" # Return format agreed with the front end result = {"resCode": '0', "message": 'success',"data": []} # Query server information servers = ServerInfo.objects.all() # Convert the model objects to dict dictionary one by one, and then set them to the list of data for server in servers: server = model_to_dict(server) # model object to dict dictionary server['server_used_type_id'] = serializers.serialize('python', server['server_used_type_id']) # Foreign key model objects need to be serialized or not passed result["data"].append(server) return JsonResponse(result)
The browser test is as follows: "
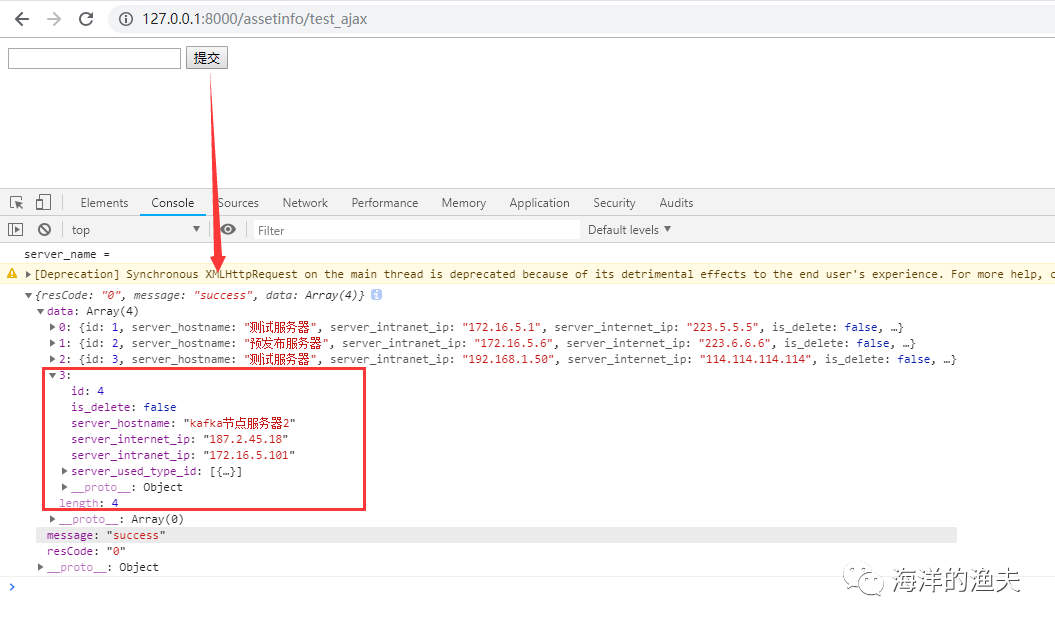
You can see that the dictionary object is passed to the front end.
Finally, an example of front-end js traversing json format data is given.
Example of json format data returned by front-end traversal
<script> $(function () { $('#search_server').click(function () { .... // Send ajax post request $.ajax({ url: "/assetinfo/test_ajax", type: 'POST', data: { "tag": "search_project", "server_name": server_name, }, dataType: "json", async: false, // The function successfully called by the request success: function (res) { {#console.log(res.data);#} // Traversal data information for(var i=0;i<res.data.length;i++){ console.log(res.data[i]); console.log(res.data[i]['server_hostname']); console.log(res.data[i]['server_internet_ip']); console.log(res.data[i]['server_intranet_ip']); } }, // The function called when the request is in error error: function () { alert("Failed to send query item data"); } }) }) }) </script>
The browser displays as follows: "
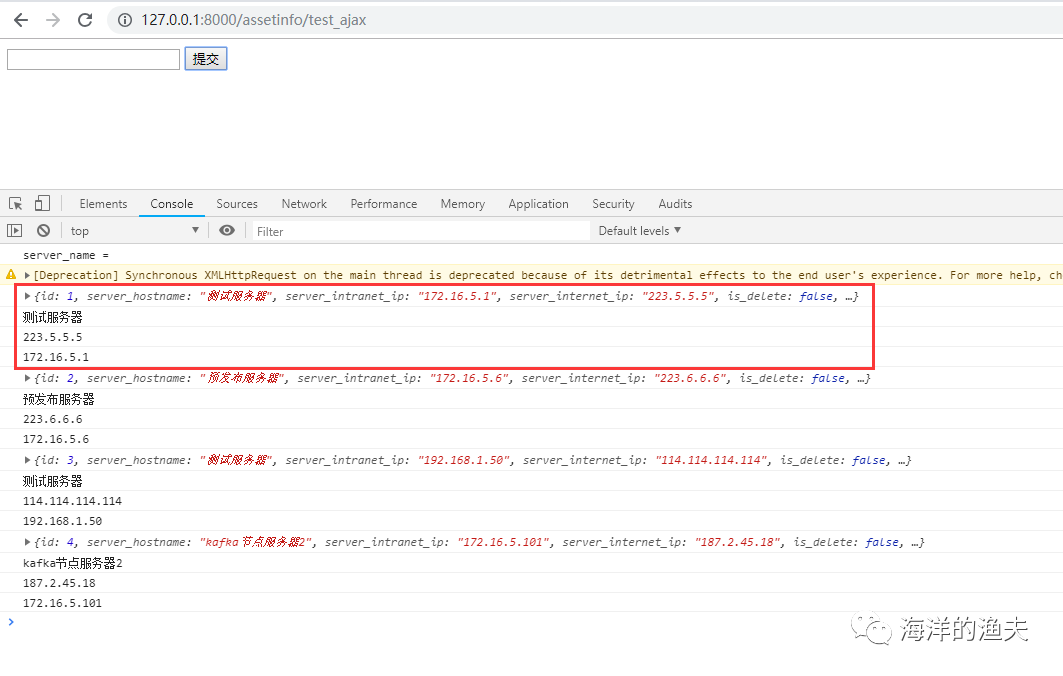