cookies and session s
- Session - from opening the browser to visit a website to closing the browser to end the visit, it is called a session
- The HTTP protocol is stateless, which makes it difficult to maintain the session state
- Imagine shopping on e-commerce websites if you don't keep the conversation state Experience?
Session and session are the two technologies for storing session
cookies
- cookies are storage space stored on the client browser
- Chrome browser may view and operate all cookie values on the browser side through application > > storage > > Cookies of developer tools
- Firefox browser may store - > cookies through developer tools
cookies are stored in the browser in the form of key value pairs. Keys and values are stored in the form of ASCII strings (not Chinese strings)
The data in cookies is stored and isolated by domain and cannot be accessed between different domains
The internal data of cookies will be carried to the server every time you visit this website. If the cookies are too large, the response speed will be reduced
- Setting the COOKIE of the browser in Django must be completed through the HttpResponse object
Add and modify COOKIE
HttpResponse.set_cookie(key, value='', max_age=None, expires=None)
Key: the name of the cookie
Value: the value of the cookie
max_age: cookie lifetime, in seconds
expires: specific expiration time
When Max is not specified_ Age and expires, this data is invalid when the browser is closed
- Delete COOKIE
HttpResponse.delete_cookie(key)
The specified Cookie key is deleted. If the key does not exist, nothing happens.
- Get cookie
Pass request The COOKIES bound Dictionary (dict) obtains the COOKIES data of the client
value = request.COOKIES.get('cookies name', 'Default value') print("cookies name = ", value)
- Examples
The following examples are called in the view function.
Add cookie
# Add key for browser as my_ A cookie with a value of 123 and an expiration time of 1 hour responds = HttpResponse("Added my_var1,The value is 123") responds.set_cookie('my_var1', 123, 3600) return responds
- Modify cookie
# Add key for browser as my_var1, the modified value is 456, and the expiration time is 2 hours responds = HttpResponse("Modified my_var1,The value is 456") responds.set_cookie('my_var1', 456, 3600*2) return responds
- Delete cookie
# Delete browser key as my_ cookie for VAR1 responds = HttpResponse("Deleted my_var1") responds.delete_cookie('my_var1') return responds
- Get cookie
# Get my in browser_ Value corresponding to var variable value = request.COOKIES.get('my_var1', 'No value!') print("cookie my_var1 = ", value) return HttpResponse("my_var1:" + value)
session
Session, also known as session control, is to open up a space on the server to retain important data when the browser interacts with the server
- Implementation mode
To use session, you need to start the cookie on the browser client and store the sessionid in the cookie
Each client can have an independent Session on the server
Note: this data will not be shared among different requesters, and it corresponds to the requester one by one
- Configuring Session in Django
In settings Py file
To installed_ Add to the apps list:
INSTALLED_APPS = [ # Enable sessions app 'django.contrib.sessions', ]
- Add to the midview list:
MIDDLEWARE = [ # Enable Session Middleware 'django.contrib.sessions.middleware.SessionMiddleware', ]
- Basic operation of session:
session for an object of SessionStore type similar to a dictionary, it can be operated in a dictionary like manner
session can only store data that can be serialized, such as dictionaries, lists, etc.
1. Save the session value to the server
request.session['KEY'] = VALUE
2. Get the value of session
- VALUE = request.session['KEY']
- VALUE = request.session.get('KEY ', default)
3. Delete the value of session
del request.session['KEY']`
- In settings session settings in PY
- SESSION_COOKIE_AGE
Function: specify the duration of saving sessionid in cookies (2 weeks by default), as follows:
SESSION_COOKIE_AGE = 60 * 60 * 24 * 7 * 2
- SESSION_EXPIRE_AT_BROWSER_CLOSE = True
Set that the session will be invalid as long as the browser is closed (the default is False)
- Note: when using session, you need to migrate the database, otherwise an error will occur
python3 manage.py migrate
django Primordial session Question: 1,django_session Table is a single table design; And the data volume of this table continues to increase [deleted intentionally by the browser] sessionid&Expired data not deleted] 2,It can be executed every night python3 manage.py clearsessions [This command deletes expired session [data]
Cookies vs session
Storage location: C- In browser s- Server[ mysql] Security: C - unsafe s- Relatively safe Ignore C still S , Do not store sensitive data [password]
cache
What is caching?
Cache is a kind of media that can read data faster. It also refers to other storage methods that can speed up data reading. It is generally used to store temporary data. The commonly used medium is the memory with fast reading speed
Why cache?
View rendering has a certain cost. For pages with low-frequency changes, cache technology can be considered to reduce the actual rendering times
case analysis
from django.shortcuts import render def index(request): # Rendering with high time complexity book_list = Book.objects.all() #->It is assumed here that it takes 2s return render(request, 'index.html', locals())
Optimization thought
given a URL, try finding that page in the cache if the page is in the cache: return the cached page else: generate the page save the generated page in the cache (for next time) return the generated page
Use cache scenarios: blog list page, e-commerce product details page, cache navigation and footer
Setting cache in Django
Django provides a variety of caching methods. If you need to use it, you need to use it in settings Py
- Change the configuration of database cache mysite7 to migrate and add the cache configuration item createcachetable
Django can store its cached data in your database
CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.db.DatabaseCache', 'LOCATION': 'my_cache_table', 'TIMEOUT': 300, #The unit of cache saving time is seconds. The default value is 300, 'OPTIONS':{ 'MAX_ENTRIES': 300, #Maximum number of cached data 'CULL_FREQUENCY': 2,#Delete 1/x of cache data when the number of cache entries reaches the maximum } } }
Create cache table
python3 manage.py createcachetable
- File system cache
CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.filebased.FileBasedCache', 'LOCATION': '/var/tmp/django_cache',#This is the path to the folder #'LOCATION': 'c:\test\cache',#Example under windows } }
- Local memory cache
CACHES = { 'default': { 'BACKEND': 'django.core.cache.backends.locmem.LocMemCache', 'LOCATION': 'unique-snowflake' } }
Using cache in Django
- Use in View
- Use in routing URL s
- Use in template
Using cache in View
from django.views.decorators.cache import cache_page @cache_page(30) -> Company s def my_view(request): ...
Use in routing
from django.views.decorators.cache import cache_page urlpatterns = [ path('foo/', cache_page(60)(my_view) ), ]
Use in template
{% load cache %} {% cache 500 sidebar username %} .. sidebar for logged in user .. {% endcache %}
- Cache api
- Function: local cache partial results
- use:
#Specify configuration import from django.core.cache import caches cache1 = caches['myalias'] cache2 = caches['myalias_2'] #The default configuration [default item in the configuration] introduced by default is equivalent to caches['default '] from django.core.cache import cache #General command set #key: string type #value: Python object #Timeout: cache storage time. The default value is settings Py caches corresponds to the configured timeout #Return value: None cache.set('my_key', 'myvalue', 30) #General command get #Return value: the specific value of the key. If there is no data, return None cache.get('my_key') #The default value can be added. If it is not obtained, the default value will be returned cache.get('my_key', 'default value') #The general command add can be set successfully only when the key does not exist #Return value True or False cache.add('my_key', 'value') #If my_ If the key already exists, the assignment is invalid #General command get_or_set if no data is obtained, perform the set operation #Return the value of key cache.get_or_set('my_key', 'value', 10) #General command get_many(key_list) set_many(dict,timeout) #Return value set_many: returns the key array that failed to insert # get_many: Dictionary of retrieved key and value >>> cache.set_many({'a': 1, 'b': 2, 'c': 3}) >>> cache.get_many(['a', 'b', 'c']) {'a': 1, 'b': 2, 'c': 3} #General command delete #Return value None cache.delete('my_key') #General command delete_many #Return value the number of data pieces successfully deleted cache.delete_many(['a', 'b', 'c'])
Cache in browser
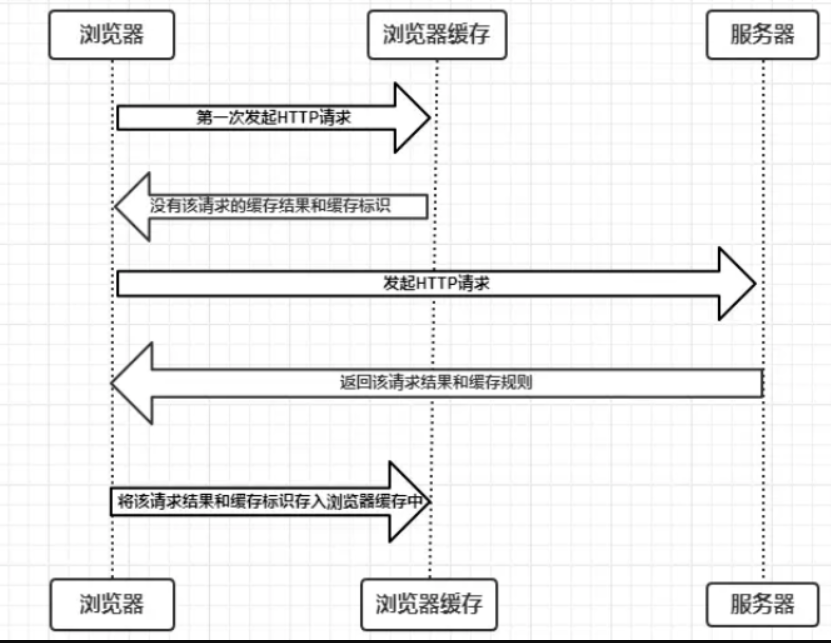
Browser cache classification:
Strong cache
No request will be sent to the server and resources will be read directly from the cache
1,Expires
Cache expiration time is used to specify the expiration time of resources. It is a specific time point on the server side
Expires:Thu, 02 Apr 2030 05:14:08 GMT
Expires is a product of HTTP/1. It is limited by the local time. If the local time is modified, the cache may become invalid
2, Cache-Control
In HTTP/1.1, cache control is mainly used to control web page caching. For example, when cache control: Max age = 120 represents 120 seconds after the request creation time, the cache is invalid
Negotiation cache
Negotiation cache is a process in which the browser sends a request to the server with the cache ID after the forced cache expires, and the server determines whether to use the cache according to the cache ID
- Last modified and if modified since On the first visit, the server returns Last-Modified: Fri, 22 Jul 2016 01:47:00 GMT The browser will carry the header if modified since the next request. The value is last modified After receiving the request, the server compares the results. If the resource has not changed, it returns 304; otherwise, it returns 200 and returns the new resource to the browser Disadvantages: it can only be accurate to seconds, which is prone to multiple modifications in a single second and can not be detected
- ETag and if none match Etag is a unique identifier (generated by the server) of the current resource file returned by the server when responding to the request. As long as the resource changes, Etag will be regenerated The process is the same as above
Compare last modified vs Etag
- Different accuracy - Etag high
- Performance - last modify high
- Priority - Etag high