Easyui Front End, JAVA Background Upload Attachments
The front end uses the easyui framework and the back end uses the JFinal framework of JAVA
Function description: Implement attachment upload function.The file upload path is:./upload (upload folder)/ID number/chronic disease code/upload attachment.
Detail requirements: multi-picture upload, after uploading, can be added and deleted twice in the foreground page
1. The front page shows: Fill in the ID number, select the chronic disease information, click the "Upload Attachment" button to select the file to upload
Front page code:

1 <tr>
2 <td class="pe-label"><span class="sp_waning">*</span>ID number:</td>
3 <td class="pe-content">
4 <input id="newchrApply_app05" name="newchrApply_app05" class="easyui-textbox">
5 </td>
6 <td class="pe-label">Apply for illness:</td>
7 <td class="pe-content">
8 <input id="newchrApply_app10" name="newchrApply_app10" class="easyui-combobox">
9 </tr>
10 <tr>
11 <td class="pe-label">Attachment upload:</td>
12 <td class="pe-content" colspan="3">
13 <span class="ui_button_primary"><label for="newchrApply_file1">Upload Attachments</label></span>
14 <input id="newchrApply_file1" name="newchrApply_file1" type="file" style="position:absolute;clip:rect(0 0 0 0);" multiple="multiple">
15 </td>
16 </tr>
17 <tr>
18 <td class="pe-label">Upload attachment name:</td>
19 <td class="pe-content" colspan="3">
20 <ul id='content'></ul>
21 </td>
22 </tr>
Form Display
2. Display the uploaded file information and delete button in the "Upload Attachment Name".
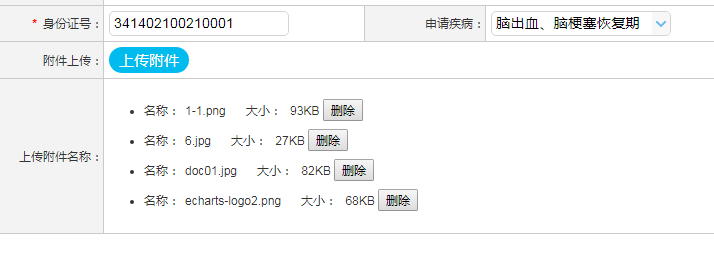

1 var chrApply_filesTemp = [];//Save Uploaded Attachments Collection
2 //Show upload file name
3 var test = document.getElementById('newchrApply_file1');
4 test.addEventListener('change', function() {
5 var t_files = this.files;
6 var p_idnum = $.trim($('#NewchrApply_app05').val(); //ID number
7 var p_icd01 = $('#newchrApply_app10').combobox('getValue');
8 if(p_idnum == '' || p_icd01 == '') {
9 $.messager.alert('Tips', 'Please enter your ID number or select a disease!', 'warning');
10 return;
11 }
12 var p_app01 = $.trim($('#newchrApply_app01').val());
13 if(p_app01 == '') {
14 var p_code = "SQ" + CreateCode(3);
15 $('#newchrApply_app01').val(p_code);
16 }
17 var str = '';
18 if(t_files.length > 0) {
19 var formData = new FormData();
20 for(var i = 0; i < t_files.length; i++) {
21 formData.append("file_cont" + i, t_files[i]);
22 }
23 formData.append("fileCount", t_files.length);
24 formData.append("app05", p_idnum);
25 formData.append("app10", p_icd01);
26 $.ajax({
27 url: '/ChrApply/UploadFiles',
28 type: 'POST',
29 data: formData,
30 processData: false,
31 contentType: false,
32 success: function(result) {
33 if(result.code > 0) {
34 var p_filesname = [];
35 if(chrApply_filesTemp.length > 0) {
36 for(var i = 0; i < chrApply_filesTemp.length; i++) {
37 if(p_filesname.indexOf(chrApply_filesTemp[i].name) == -1) {
38 p_filesname.push(chrApply_filesTemp[i].name);
39 }
40 }
41 }
42 var chrApply_filesUp = t_files; //New Uploaded File Collection
43 if(chrApply_filesUp.length > 0) {
44 for(var i = 0; i < chrApply_filesUp.length; i++) {
45 if(p_filesname.indexOf(chrApply_filesUp[i].name) == -1) {
46 chrApply_filesTemp.push({
47 'name': chrApply_filesUp[i].name,
48 'size': chrApply_filesUp[i].size
49 });
50 }
51 }
52 }
53 for(var i = 0, len = chrApply_filesTemp.length; i < len; i++) {
54 str += '<li id="li_' + i + '">Name:<span id="sp_name_' + i + '">' + chrApply_filesTemp[i].name + '</span> Size:<span id="sp_size_' + i + '"> ' + parseInt(chrApply_filesTemp[i].size / 1024) + 'KB</span>' +
55 ' <input id="delfj" type="button" value="delete" onclick="delAnnex(' + i + ')" ></li>';
56 }
57 document.getElementById('content').innerHTML = str;
58 } else {
59 $.messager.alert('Tips', result.msg, 'warning');
60 }
61 }
62 });
63 }
64 }, false);
Upload Attachments

1 //delete
2 function delAnnex(id) {
3 var del_idnum = $.trim($('#newchrApply_app05').val()); //ID number
4 var del_icd01 = $('#newchrApply_app10').combobox('getValue');
5 var del_name = document.getElementById("sp_name_" + id).innerText;
6
7 $.ajax({
8 url: '/ChrApply/DeleteAnnex',
9 type: 'POST',
10 data: {
11 'app05': del_idnum,//ID number
12 'app10': del_icd01,//Chronic Disease Coding
13 'ann01': del_name//Attachment Name
14 },
15 success: function(result) {
16 if(result.code > 0) {
17 // Delete elements from a collection
18 for(var i = 0; i < chrApply_filesTemp.length; i++) {
19 var flg = isEqual(chrApply_filesTemp[i].name.valueOf(), del_name.valueOf());
20 if(flg == true) {
21 chrApply_filesTemp.splice(i, 1);
22 }
23 }
24 var first = document.getElementById("li_" + id);
25 first.remove();
26 } else {
27 $.messager.alert('Tips', result.msg, 'warning');
28 }
29 }
30 });
31 }