In this article, I will focus on edge based and region based segmentation techniques. Before entering the details, we need to understand what segmentation is and how it works.
division
Image segmentation is a technology that divides digital images into various image objects.
Each pixel in a region (image object) is similar in some attributes, such as color, intensity, position or texture, which can reduce the complexity of the image for analysis. With the help of segmentation, hidden information can also be detected from the image.
The segmentation algorithm processes two basic attributes of an image:
- Strength values, such as discontinuities (boundary method)
- Similarity (regional method)
In the figure below, we see an image characterized by chairs, tables, windows, etc. We can get these objects separately by segmentation. The middle image has a chair, a table and a window as our segmentation image objects. In the rightmost image, instance segmentation is used by marking the image object.
After using Python for machine learning, segmentation becomes very easy.
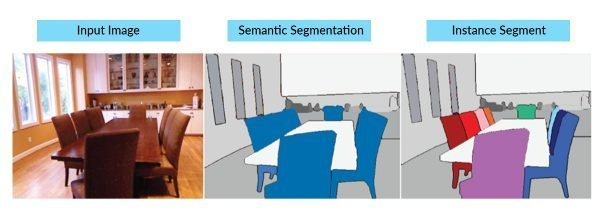
Necessity of image segmentation
The image is divided into different image objects, from which information is obtained, and then labels are used to train various ML models to solve business problems. One example is a face recognition system, which automatically marks attendance through segmentation.
Another application of segmentation is in the medical field, to carry out efficient and rapid diagnosis after detecting serious diseases such as tumor and cancer, and to view the patterns in medical images generated by radiography, MRI, thermal imaging, endoscopy, cell and tissue ultrasound. Image segmentation also has great applications in robotics and other fields.
Image classification is a popular segmentation application. The algorithm can only capture the required components from the image. It is easy to implement image segmentation in Python to obtain fast results.
Edge based segmentation
In this method, the boundaries of regions are very different from each other and from the background, which allows boundary detection based on local discontinuities of intensity (gray level).
In other words, it is the process of locating edges in the image. This is a very important step in understanding image features, because we know that edges are composed of meaningful features and have important information.
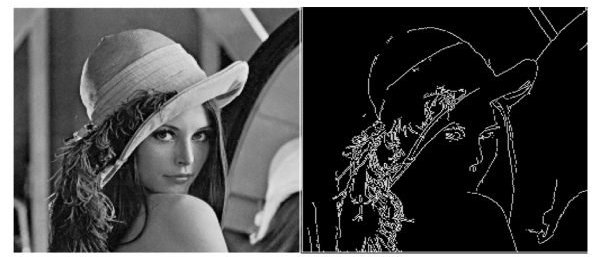
Region based segmentation
This method includes dividing the image into similar regions according to a specific set of criteria.
The region based segmentation technology relates to an algorithm, which divides the image into various components with similar pixel characteristics. The technology searches for small blocks or large blocks in the input image for segmentation.
It will add more pixels to the selected block, or further reduce the block points to smaller segments and merge them with other smaller block points. Therefore, there are two more basic technologies based on this method: region growth and region merging and segmentation.
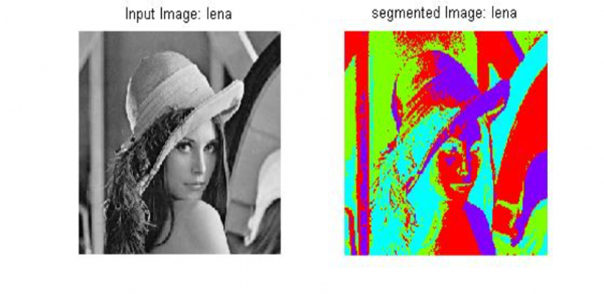
Main applications of segmentation
- Major disease detection
- Face recognition system
- Autopilot
- Robotics
Python implementation
import numpy as np import matplotlib.pyplot as plt from skimage import data coins = data.coins() hist = np.histogram(coins, bins=np.arange(0, 256)) fig, (ax1) = plt.subplots() ax1.imshow(coins, cmap=plt.cm.gray,interpolation='nearest')
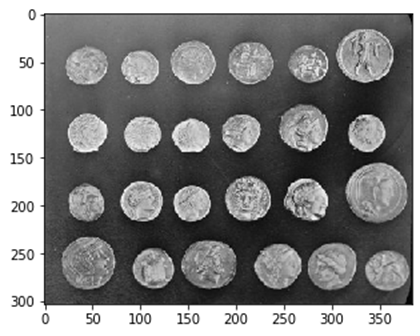
Edge based segmentation
from skimage.feature import canny edges = canny(coins/255.) fig, ax = plt.subplots(figsize=(4, 3)) ax.imshow(edges, cmap=plt.cm.gray, interpolation='nearest') ax.axis('off') ax.set_title('Canny detector') Text(0.5, 1.0, 'Canny detector')
In this code, we use canny library, which is a popular edge detection algorithm to detect the edge of the input image.
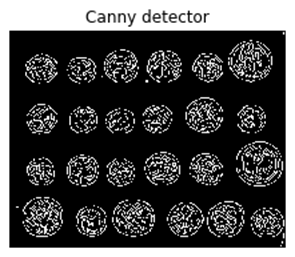
Small spurious objects can be easily removed by setting the minimum size of valid objects:
from scipy import ndimage as ndi fill_coins = ndi.binary_fill_holes(edges) fig, ax = plt.subplots(figsize=(4, 3)) ax.imshow(fill_coins, cmap=plt.cm.gray, interpolation='nearest') ax.axis('off') ax.set_title('Filling the holes') Text(0.5, 1.0, 'Filling the holes')
We use ndimage as ndi, which means an n-dimensional image. It is a sub module for processing image processing operations, such as input, output, clipping, filtering, etc.
Ndi.binary_fill_holes are used to fill n-dimensional binary array holes and intrusion holes connected to the boundary.
Region based segmentation
from skimage.filters import sobel elevation_map = sobel(coins) fig, ax = plt.subplots(figsize=(4, 3)) ax.imshow(elevation_map, cmap=plt.cm.gray, interpolation='nearest') ax.axis('off') ax.set_title('elevation_map') Text(0.5, 1.0, 'elevation_map')
Here, we start from skimage Filters import sobel module, which is used to find the edge in the input image.
Sobel transform can also help us find the vertical and horizontal edges in the input image.
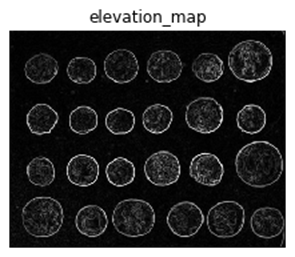
conclusion
This article uses Python implementation to explain segmentation and its two important technologies (edge based segmentation and region based segmentation) in detail.