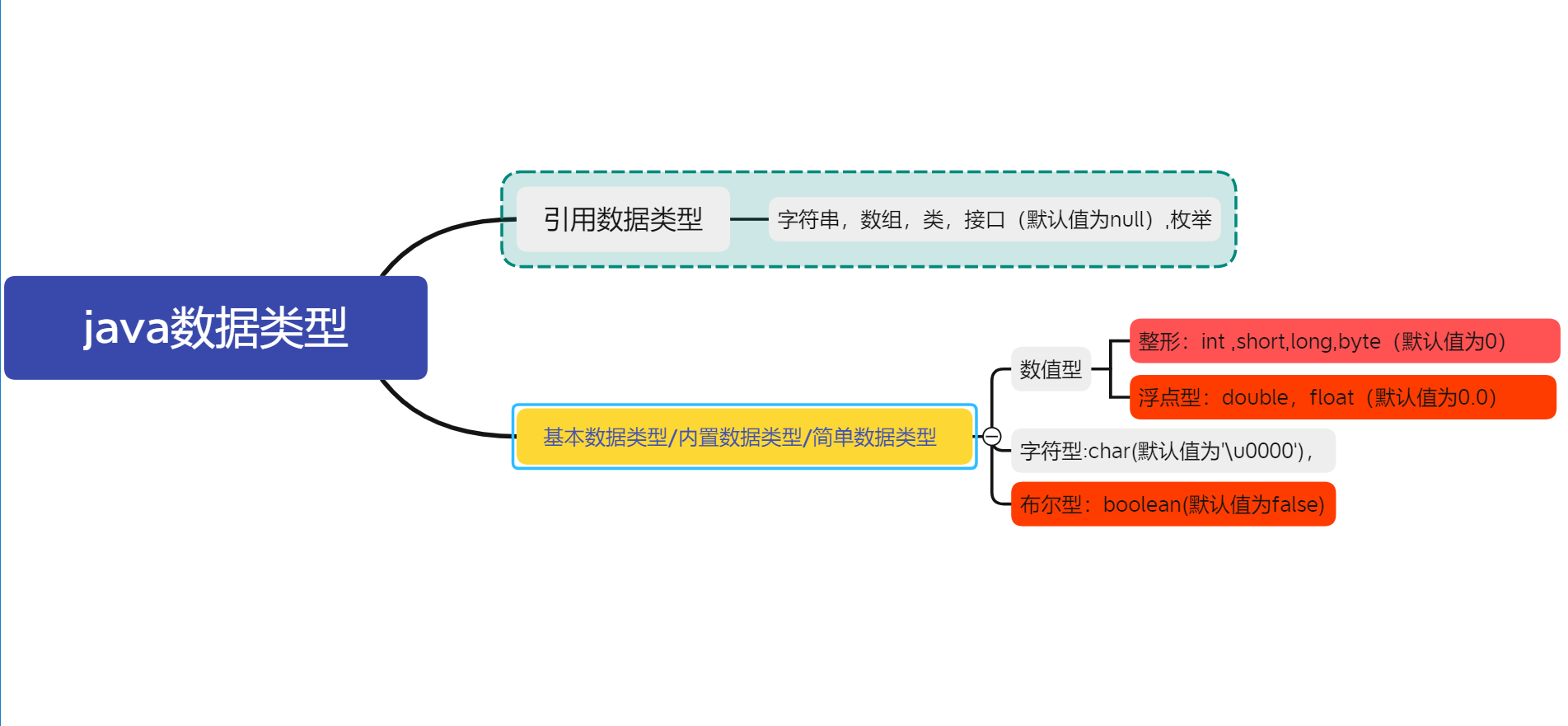
✏️ Variables and types
Variable refers to a quantity used to store variable data. It is created before the program runs to open up a memory space to store some data Type is to divide the types of variables. Variables of different types are used to store different data and have different attributes, These variables are created on the computer's internal memory, and the program runs on the internal memory. The audio, pictures and videos we usually produce are stored on the external memory of the computer. The external memory is simply our hard disk, u disk, optical disk and other devices. Now computers work according to von Neumann system. Let's take a brief look at von Neumann system structure:
Introduction to basic data types:
data type | Bytes occupied | Default value | Value range | Wrapper class |
---|---|---|---|---|
byte | 1 | 0 | -2^7— 2^7-1 | Byte |
short | 2 | 0 | -2^15— 2^15-1 | Short |
int | 4 | 0 | -2^31— 2^31-1 | Integer |
long | 8 | 0 | -2^63— 2^63-1 | Long |
float | 4 | 0.0 | -2^31— 2^31-1 | Float |
double | 8 | 0.0 | -2^63— 2^63-1 | Double |
char | 1 | empty | 0—2^16-1 | Character |
boolean | The JVM standard does not specify the size, and there is no explicit size | false | true or false | Boolean |
Remember the table above, and save the screenshot below (HA HA)
✏️ Integer variable (key)
Basic syntax format
int Variable name = Initial value;
Code example: int num = 10; // Define an integer variable System.out.println(num) ;
matters needing attention:
- int indicates that the type of the variable is an integer
- The variable name is the identification of the variable Variables are used later by this name
- In Java, = means assignment (different from Mathematics), which means setting an initial value for a variable
- Initialization is optional, but it is recommended that variables be explicitly initialized when they are created Local variables must be initialized when they are used. If they are not initialized, the compilation cannot pass.
- In java, an Int variable takes up 4 bytes It is not directly related to the number of bits of the machine used. Codes with Int variables can run as long as they are installed on the machine where the jvm is installed., In this way, java is highly portable.
What are bytes?
Bytes are the basic unit of space in a computer Computers use binary to represent data We consider eight bits as one byte Including 1KB
= 1024 Byte, 1MB = 1024 KB, 1GB = 1024 MB. In java, an int variable takes up 4 bytes. There is no unsigned integer in java, but it is signed (such as short,long,byte).
You can view the value range of the corresponding data by using the packaging class of the corresponding type. The packaging class is actually an upgraded version of the original data type. The data types defined by them are consistent. The integer data range in Java can be printed through the following code:
System.out.println(Integer.MAX_VALUE); // Maximum value of int System.out.println(Integer.MIN_VALUE); // Minimum value of int
🎇🔍✒️ When assigning a value to any data type, the literal value must not exceed the value range of this type, otherwise overflow will occur and compilation errors will occur. Secondly, for any type of data, the maximum value plus one becomes the minimum value, and the minimum value plus one becomes the maximum value. This is a rule. You can remember that you can refer to the figure I drew above when memorizing.
public static void main(String[] args) { int maxValue = Integer.MAX_VALUE; System.out.println(maxValue);//Maximum int a=Integer.MAX_VALUE+1;; System.out.println(a); int b=2147483647+1; //int c=2147483648; Code compilation error System.out.println(maxValue); int minValue = Integer.MIN_VALUE; System.out.println(minValue-1); }
The operation results are as follows:
✏️ Long integer variable
Basic syntax format:
long Variable name = Initial value;
Code example:
long num = 10L; // Define a long integer variable, and the initial value can be written as 10l (lowercase L, not number 1) System.out.println(num) ;
matters needing attention:
- The basic syntax format is basically the same as that of creating int variables, except that the type is changed to long
- The initialization setting value is 10l, which represents a long integer number 10l is also OK
- You can also initialize with 10. The type of 10 is int, and the type of 10L is long. It is better to use 10 L or 10 L
Long integer data range in Java, which is far beyond the representation range of int Enough for most scenes
System.out.println(Long.MAX_VALUE); System.out.println(Long.MIN_VALUE) // Operation results 9223372036854775807 -9223372036854775808
✏️ Double precision floating point variable (key)
Basic syntax format
double Variable name = Initial value;
Code example:
double num = 1.0; System.out.println(num)
In Java, the value of int divided by int is still int (the decimal part will be discarded directly)
int a = 1; int b = 2; System.out.println(a / b); // results of enforcement 0
If you want to get decimals, you need to use double type calculation
double a = 1.0; double b = 2.0; System.out.println(a / b); // results of enforcement 0.5
Although the double in java is also 8 bytes, the memory layout of floating-point numbers is very different from that of integers, and the data range cannot be simply expressed in the form of 2 ^ n** float accurately represents 6 decimal places, double accurately represents 11 decimal places, * * decimal can only be accurate to a few digits, and exceeding the exact value is inaccurate.
double num = 1.1; System.out.println(num * num) // results of enforcement 1.2100000000000002
✏️ Single precision floating point variable
Basic format:
float Variable name = Initial value;
Code example:
float num = 1.0f; // Writing 1.0F is also OK System.out.println(num);
Float type occupies four bytes in Java and also complies with IEEE 754 standard Due to the small range of data accuracy, double is preferred for floating-point numbers in engineering, and float is not recommended
💣💥❕ For data of float type, the letters following the data type cannot be omitted. The default type of floating-point data is double. If omitted, an error will be reported if there is a type difference during definition, while the default type of shaping is int, and the letters following can be omitted, as shown in the following code;
float num = 1.0; //Compilation error, can be written as 1.0F or 1.0F System.out.println(num); long num=4;
✏️ Character type variable
Basic format:
char Variable name = Initial value;
Code example:
char ch = 'A'; char cp="good"
matters needing attention:
- Java uses single quotation marks + single letters to represent character literals
- A character in a computer is essentially an integer ASCII is used to represent characters in C language, while Unicode is used to represent characters in Java Therefore, a character occupies two bytes and represents more types of characters, including Chinese.
- 💣💥❕ char represents an unsigned type in java, that is, it cannot store negative numbers. There are no unsigned types, but positive numbers.
✏️ Byte type variable
Basic syntax format:
byte Variable name = Initial value;
Code example:
byte value = 0; System.out.println(value);
matters needing attention:
💣💥❕ 1. The byte type also represents an integer It only occupies one byte, and the size of the representation is the same as that of the char type representation of c language.
2. Byte type and character type are irrelevant
✏️ Short integer variable
Basic syntax format:
short Variable name = Initial value;
Code example:
short value = 0; System.out.println(value);
matters needing attention:
- short takes up 2 bytes and represents a data range of - 32768 - > + 32767 (- 2 ^ 15-2 ^ 15).
- The scope of this representation is relatively small. It is generally not recommended. In practice, the use of int is greater than that of short.
✏️ Boolean type variable
Basic syntax format:
boolean Variable name = Initial value;
Code example:
boolean value = true; System.out.println(value);
matters needing attention:
> 1. Boolean variables have only two values. True means true and false means false. It cannot be converted with other types.
2. Java boolean type and int cannot be converted to each other. There is no such usage as 1 for true and 0 for false
3. boolean type. Some JVM s occupy 1 byte, and some occupy 1 bit. This is not explicitly specified
✏️ String type variable (emphasis)
Put some characters together to form a string, which belongs to the reference data type.
Basic syntax format:
String Variable name = "Initial value"; //The variable name here is a reference variable, which stores the address of the string, and the variable points to the string object
Code example:
String name = "zhangsan"; System.out.println(name);
matters needing attention:
- Java uses double quotation marks + several characters to represent string literals
- Different from the above types, String is not a basic type, but a reference type (which will be explained in the blog later)
- Some specific characters in the string that are inconvenient to be directly represented need to be escaped
Escape character | explain |
---|---|
\n | Line feed |
\t | Horizontal tab |
\' | Single quotation mark |
\" | Double quotation mark |
\\ | Anti inclined rod |
Common escape characters:
Examples of escape characters:
// Create a string My name is "Zhang San" String name = "My name is \"Zhang San\"";//The middle two quotation marks are parsed as escape characters, and the outer two quotation marks contain a string
💣💥❕ The + operation of string indicates string splicing. After string and any type of data are spliced with a plus sign, the result is also a string
String a = "hello"; String b = "world"; String c = a + b; System.out.println(c);
The result is:
💣💥❕ String and integer can also be used for splicing: when a string exists in a + expression, string splicing is performed:
String str = "result = "; int a = 10; int b = 20; String result = str + a + b+"hello"; System.out.println(result); // The result is result = 1020hello
💣💥❕ When splicing, it should be noted that if there is no character in front of the number, the number will be spliced after addition
String str = "result = "; int a = 10; int b = 20; String result = a + b+str + "hello"; System.out.println(result); String result1="world"+a + b+str + "hello"; System.out.println(result1);
As shown in the figure:
✏️ Scope of variables and naming rules of variables
That is, the range within which the variable can take effect. Generally, it is the code block (braces) where the variable definition is located. If it is defined inside the code block, there will be an error in external access. The local variable is defined in the function body, and its scope of action is within the function body.
class Test { public static void main(String[] args) { { int x = 10; System.out.println(x); // Compilation passed; } System.out.println(x); // Compilation failed, variable x } }
Naming rules: hard indicators:
- A variable name can only contain numbers, letters, underscores and dollar symbols
- A number cannot begin
- Variable names are case sensitive That is, Num and num are two different variables Note: Although the Chinese dollar sign ($) is also allowed to name variables in syntax, such soft indicators are strongly not recommended:
- Variable naming should be descriptive, see the meaning of name
- Variable names should not use pinyin (but not absolute)
- Nouns are recommended for the part of speech of variable names
- The small hump naming method is recommended for variable naming. When a variable name is composed of multiple words, the first letters of other words are capitalized except the first word. The large hump naming is used to name the class interface, and the first letters of each word are capitalized: example of large and small hump naming:
int zuidazhi//You can't name it that way int maxValue = 100; String studentName = "Zhang San"; public TestDemo//Class naming
✏️ constant
The variables discussed above are variables of various rules. Each type of variable also corresponds to a constant of the same type. For example, the constant corresponding to int type is a constant of int type Constant means that the runtime type cannot be changed.
Constants are mainly embodied in the following two forms:
✏️ literal constant
- 10 // int literal constant (decimal)
- 010 // int literal constant (octal) starts with the number 0 010 is the decimal 8
- 0x10 // int literal constant (HEX) starts with the number 0x 0x10 is the decimal 16
- 10L // long literal constant You can also write 10l (lowercase L)
- 1.0 // double literal constant You can also write 1.0D or 1.0D
- 1.5e2 // double literal constant Scientific notation Equivalent to 1.5 * 10 ^ 2
- 1.0f // float literal constant, or 1.0F
- true // boolen literal constant, and also false
- 'a' / / char literal constant. There can only be one character in single quotation marks
- "abc" / / String literal constant. There can be multiple characters in double quotation marks
.
✏️ Constant modified by final keyword
Modify a variable with final in java and convert it into a constant. The constant cannot be modified during program operation, 💣💥❕ Generally, variable names are also expressed in uppercase letters.
final int a = 10; a = 20; // Compilation error Tip: unable to assign value to final variable a final int SIZE ; SIZE = 99; System.out.println(SIZE);
✏️ Type conversion and value promotion
Type conversion
As a strongly typed programming language, Java teaches strict verification when variables of different types are assigned to each other Let's look at the following code scenarios:
int and long/double are assigned to each other
int a = 10; long b = 20; a = b; // Compilation error, prompt may lose precision Then the code will not be executed b = a; // The compilation passed ------------------------------- int a = 10; double b = 1.0; a = b; // Compilation error, prompt may lose precision b = a; // Compiler pass
Long indicates a larger range. You can assign int to long, but you can't assign long to int
Double represents a larger range. You can assign int to double, but you can't assign double to int
💣💥❕ Conclusion: the assignment between variables of different numerical types indicates that the type with smaller range can be implicitly converted to the type with larger range, otherwise it is not
int and boolean are assigned to each other
int a = 10; boolean b = true; b = a; // Compilation error, prompt incompatible types a = b; // Compilation error, prompt incompatible types
Conclusion: there are only two values for boolean variables, true for true and false for false int and boolean are two unrelated types and cannot be assigned to each other
The int literal constant assigns a value to byte
byte a = 100; // Compile passed byte b = 256; // An error is reported during compilation, indicating that conversion from int to byte may be lost
Note: the data range represented by byte is - 128 - + 127, 256 has exceeded the range, and 100 is still within the range
Conclusion: when using literal constant assignment, Java will automatically perform some checks to determine whether the assignment is reasonable
Conversion between int and String
Convert int to String
int num = 10; // Method 1 String str1 = num + ""; // Method 2 String str2 = String.valueOf(num);//Convert using valueOf function
Convert String to int
String str = "100"; int num = Integer.parseInt(str);//Convert using parseInt function System.out.println(num);//The result is 100
Use cast
int a = 0; double b = 10.5; a = (int)b; int a = 10; boolean b = false; b = (boolean)a; // Compilation error, prompt incompatible types
Conclusion:
- The double type can be forcibly converted to int. by using (type)
Casting may result in loss of precision As in the example just now, after the assignment, 10.5 becomes 10, and the part after the decimal point is ignored - Forced type conversion is not always successful. Irrelevant types cannot be forced (such as boolean and other types)
Type conversion summary
1. If the accuracy of the two data representations is different, it should be converted to the data type with high accuracy, not to the direction with low accuracy.
3. Assignment between variables of different numeric types indicates that types with smaller range can be implicitly converted to types with larger range
4. If you need to assign a type with a large range to a type with a small range, you need to force type conversion, but the precision may be lost
5. When assigning a literal constant, Java will automatically check the number range
Numerical promotion
Mixed operation of int and long
int a = 10; long b = 20; int c = a + b; // Compilation error, prompt that converting long to int will lose precision long d = a + b; // The compilation passed
Conclusion: when int and long are mixed, int will be promoted to long, and the result is still of long type. You need to use variables of long type to
Receive results If you do not want to use Int to receive the result, you need to use cast to convert to Int type
Operation of byte and byte
byte a = 10; byte b = 20; byte c = a + b; System.out.println(c);
Conclusion: byte and byte are of the same type, but there are compilation errors The reason is that although both a and b are bytes, the calculation of a + b will first promote both a and b to int, and then calculate. The result is also int, which is assigned to c, and the above error will occur
💣💥❕ Because the CPU of a computer usually reads and writes data from memory in units of 4 bytes For the convenience of hardware implementation, types less than 4 bytes, such as byte and short, will be promoted to int first, and then participate in the calculation
Correct writing:
byte a = 10; byte b = 20; byte c = (byte)(a + b); System.out.println(c);
Summary of type promotion:
- For mixed operations of different types of data, those with a small range will be promoted to those with a large range
- For short and byte types smaller than 4 bytes, they will be promoted to 4-byte int s before operation
📖 operator
📖 Arithmetic operator
Basic four operators + - * /%
int / int the result is still int. if you want to get a decimal, use double to calculate
int a = 1; int b = 2; System.out.println(a / b); // The result is 0
%Represents remainder. You can find modules not only for int, but also for double
System.out.println(11.5 % 2.0); // Operation results //1.5
* 🔺❗👀 The incremental assignment operator + = - = / =% =. The compound operator is characterized by automatic type conversion for us.
As+=
int a = 10; a += 1; // Equivalent to a = a + 1 System.out.println(a);//Result 11 short c=2; c+=1;//Automatic type conversion = (short) (c + 1);
🔺❗👀 Auto increment / Auto decrement operator + + –
The pre + + is added with 1 before use, and the post + + is used first to increase by 1 (the pre post is to see the position of + + before and after the variable). It is the same as the language
//Post public static void main(String[] args) { int a = 10; int b = a++;// b = a a = a+1; System.out.println(a);//11 System.out.println(b);//10 //Front /* int a = 10;*/ /* int b = ++a;//a = a+1 b = a;*/ /* System.out.println(a);//11*/ /* System.out.println(b);//11*/ }
🔺❗👀 However, there is a special case. For example, the result of the following code is different from that of c. The result is 10, and there is 11 in c (powerful. The explanation of this problem depends on the assembly code, which we won't discuss here)
public static void main(String[] args) { int a = 10; a = a++; System.out.println(a); }
📖 Relational operator
There are six relational operators: = =! = < > < = >=
int a = 10; int b = 20; System.out.println(a == b); System.out.println(a != b); System.out.println(a < b); System.out.println(a > b); System.out.println(a <= b); System.out.println(a >= b);
🔺❗👀 Note: the return values of the expressions of relational operators are all boolean types. When we write some conditional statements, we judge that the conditions are boolean values after relational operation, rather than the difference between 0 and non-0.
📖 Logical operators (emphasis)
There are three main logical operators:
&& || !
Logic and&&
Rule: both operands are true, and the result is true; otherwise, the result is false
int a = 10; int b = 20; int c = 30; System.out.println(a < b && b < c);
Logical or||
Rule: if both operands are false, the result is false, otherwise the result is true
int a = 10; int b = 20; int c = 30; System.out.println(a < b || b < c);
Logical non!
Rule: the operand is true and the result is false; The operand is false and the result is true (this is a unary operator with only one operand)
int a = 10; int b = 20; System.out.println(!a < b);
Short-circuit evaluation
- For & &, if the left expression value is false, the overall value of the expression must be false, and the right expression does not need to be evaluated
- For |, if the left expression value is true, the overall value of the expression must be true, and the right expression does not need to be evaluated
//We all know that calculating 10 / 0 will cause the program to throw an exception However, the above code works normally, indicating that 10 / 0 is not really evaluated System.out.println(10 > 20 && 10 / 0 == 0); // Print false System.out.println(10 < 20 || 10 / 0 == 0); // Print true
📖 Bitwise Operators
There are four bitwise operators: & | ~^
🔺❗👀 Bit operations represent operations on binary bits In computers, binary is used to represent data (binary bit is a sequence composed of 01). Bitwise operation is to calculate in turn according to each bit of binary bit, and the operation is the complement of data
Bitwise AND &: if both binary bits are 1, the result is 1, otherwise the result is 0
int a = 10; int b = 20; System.out.println(a & b);//The result is 0
For bitwise operation, 10 and 20 need to be converted into binary, 1010 and 1010 respectively (the original inverse complement of positive numbers is the same), as shown in the figure:
Bitwise or |: if both binary bits are 0, the result is 0, otherwise the result is 1
int a=-1; int b=2; System.out.println(a|b);
🔺❗👀 Note: when the operands of & and | are integers (int, short, long, byte), they represent bitwise operation, and when the operands are boolean, they represent logical operation
Reverse by bit ~: the place of operation is the binary complement of a number. If the bit is 0, it will be converted to 1. If the bit is 1, it will be converted to 0. The operation is also a binary bit.
Bitwise exclusive or ^: if the binary bits of two numbers are the same, the result is 0, and if they are different, the result is 1
int a = 1; int b = 2; System.out.printf("%d\n", a ^ b);//0011 result is 3
📖 Shift operation (understand)
There are three shift operators, all of which operate according to the complement of binary bits < > > > >
Move left < <: don't use the leftmost position, and fill 0 on the rightmost position
Shift right > >: the rightmost bit is not needed, and the leftmost sign bit is filled (positive number is filled with 0, negative number is filled with 1)
Unsigned shift right > > >: the rightmost bit is not needed, and the leftmost bit is filled with 0, It's the same as the right side, but the complement is 0
be careful:
- Shift left by 1 bit, equivalent to the original number * 2 Shift N bits to the left, which is equivalent to the nth power of the original number * 2
- Shift 1 bit to the right, equivalent to the original number / 2 Shift N bits to the right, which is equivalent to the nth power of the original number / 2
- Because the shift efficiency of computer calculation is higher than that of multiplication and division, when a code exactly multiplies and divides to the nth power of 2, it can be replaced by shift operation
- Moving negative digits or shifting too many digits is meaningless
📖 Conditional operator
There is only one conditional operator, and it is also the only ternary operator in Java
Expression 1? Expression 2: expression 3
When the value of expression 1 is true, the value of the whole expression is the value of expression 2; When the value of expression 1 is false, the value of the entire expression is the value of expression 3
// Find the maximum of two integers int a = 10; int b = 20; int max = a > b ? a : b;
📖 Summary
- %Operations can also be calculated for double in Java
- It is necessary to distinguish between pre auto increment and post auto increment
- Because Java is a strongly typed language, it is strict for type checking. Therefore, operands such as & & must be boolean
- We should distinguish when & and | represent bitwise operation and when they represent logical operation
On the whole, the basic rules of Java operators are basically the same as those of C language
sense 🦀👍🏻🍹 Hold, ❤️ You