As we all know, learning programming is a very boring thing, especially when you can't get the results you want when running the program and still can't solve it after some debugging. I believe every programmer has encountered this situation, which is also the necessary stage for the birth of a great programmer.
Sadly, the same mistakes continue to happen. But luck followed. Here, I put together the eight most common errors encountered by new programmers for everyone to learn
one Check equality using single "="
char x='Y'; while(x='Y') { //... cout<<"Continue? (Y/N)"; cin>>x; }
The above code will be an endless loop ~ ~ as free as the wind~~~
The code uses an equal sign to check whether the loop conditions are equal. In fact, the program assigns the value on the right of the expression to the variable on the left when executing, which is actually the assignment of the variable. In this case, the value is' Y ', which is treated as true. Therefore, the cycle will never end. Therefore, to solve the above problems, the following changes need to be made:
- Use = = to check for equality;
- To avoid accidental assignment, place the variable on the right side of the expression. If you accidentally use an equal sign, there will be a compilation error, because you can't assign the value to something other than the variable.
char x='Y'; while('Y'==x) { //... cout<<"Continue? (Y/N)"; cin>>x; }
two Variable assignment
#include <iostream> using namespace std; int main() { int a, b; int sum=a+b; cout<<"input two numbers to add: "; cin>>a; cin>>b; cout<<"The sum is: "<<sum; return 0; }
After the above code is run, the results are as follows:
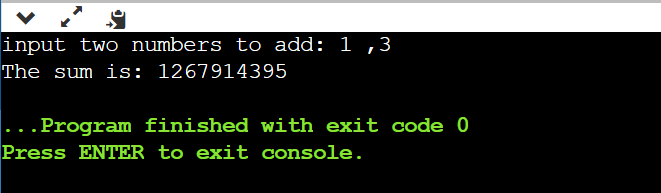
Clearly assigned values to both a and b, why does sum still get an abnormal value? Let's see what happened in the program. Generally, beginners think that the function of variables is like an equation - if a variable is assigned to the operation results of several other variables, when these variables change, such as a and b in the code, the value (sum) of the variable will also change. But this is not the case. In C + +, once a variable is assigned a value, the value of the variable will not change unless it is re assigned. Therefore, in the above program, since uninitialized variables are used to assign values to sum at the beginning, sum will also get a random value. Although a and b are assigned later, sum will not be changed.
three Variable not declared
#include <iostream> using namespace std; int main() { cin>>x; cout<<x; return 0; }
When compiling, the compiler will report an error. Because the compiler doesn't know what we mean by x, we must declare it when we use it. As follows:
#include <iostream> using namespace std; int main() { int x=0; cin>>x; cout<<x; return 0; }
four Variable not initialized
#include <iostream> using namespace std; int main() { int count; while(count<100) { cout<<count<<";"; count++; } return 0; }
When the above code is executed, it cannot enter the while loop as expected, because in C + +, integer variables will not be assigned 0 by default. In the above code, count can be any value in the range of int. For example, it might be 121, in which case the condition of the while loop will never be true. The output of the program may be a number from - 99 to 99.
Remember: variables must be initialized!!!
five Function not defined
int main() { add(); } void add() { //... }
An error will be reported during compilation. It is clear that the add function is defined later. Why is it said that the add function is not defined~
When compiling code, the compiler does not know what add() stands for unless it is told in advance. If it is told that there is a function called add after using it, it will be confused. Therefore, you must define the prototype of the function or the whole definition of the function when you use the function for the first time. For example:
void add() { //... } int main() { add(); }
six Extra semicolon
Most of these problems occur in the for loop, such as:
#include <iostream> using namespace std; int main() { int x; for(x=0; x<100; x++); cout<<x; return 0; }
The above code is expected to output values from 0 to 99, but after actual operation, the output is: 100; The reason is that there is an extra semicolon after the for statement. This will change the original logic of the code. Therefore, when programming, remember that semicolons cannot appear after if statements, loops, or function definitions. If you place one of these locations, your program will not work properly.
seven array bounds overflow
#include <iostream> using namespace std; int main() { int array[10]; for(int i=1; i<=10; i++) cout<<array[i]; return 0; }
In C + +, the array index starts from 0. For example, if you have an array of 10 elements, the first element is at position 0 and the last element is at position 9.
int array[10]; for(int =1; i<10; i++) cout<<array[i];
There are other problems in the above code, such as the array is not initialized, so the printed value is actually a random value when outputting.
eight Incorrect use of "& &" and "|"
#include <iostream> using namespace std; int main() { int value; do { //... value=10; }while(!(value==10) || !(value==20)); return 0; }
The original intention of the code implementation should be to end the loop when value is equal to 10 or value is equal to 20. However, it is found that the loop statement will always be executed even if value is equal to 10. From the analysis of while expression. It is impossible to have value equal to 10 and 20 at the same time. If you want to meet the end condition of the above loop, you need to modify the expression to:! (value==10) && ! (value==20) or! ((value = = 10) | (value==20)) can meet the exit conditions. If you have any questions about this condition, you can review discrete mathematics by yourself.